I wanted to create a replication of the portrait that could be either detailed and accurate or abstract. To do this I made my image get created by squares and circles that are created by a loop. The size gets changed by the x-position of the mouse (left is less abstract and right is more abstract).
sketchDownload
//function that loads image of my face
function preload() {
lucas = loadImage("https://i.imgur.com/M9dRkNS.png");
}
function setup() {
//canvas is the size of the resized image
createCanvas(850/2.29, 480);
background(0);
//stores pixels from the image
lucas.loadPixels();
//resizes image to keep proportions
lucas.resize(850/2.29, 480);
frameRate(100);
}
function draw() {
//changes position of the circles and squares in the array
var modifier = 10;
//constrains the size of the circles and squares
var restrict = map(mouseX, 0, width, 5, modifier*2);
for(var i = 0; i < width; i++){
for(var j = 0; j < height; j++){
//obtains the pixel color at a given x and y of the picture
var pixelColor = lucas.get(i*modifier, j*modifier);
noStroke();
//fills with the color from the pixel
fill(pixelColor);
//creates circles and squares that will replicate the image
circle(i*modifier, j*modifier, random(restrict));
square(i*modifier, j*modifier, random(restrict));
}
}
}
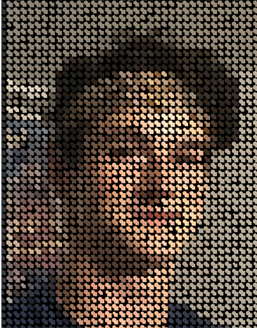
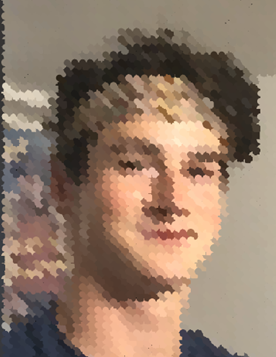
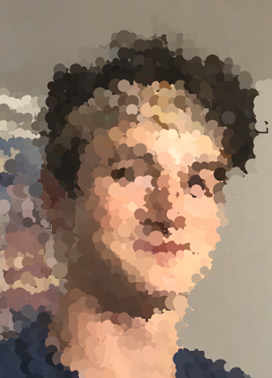