For my project, I animated a short story about a frog on a lily pad on a lake. The story is simply about a lonely frog who gets excited to eat a fly that comes alone (because it was hungry), and gets sad when a nearby fish swims away. There is thunder and lightning that turns the sky dark, and the frog wishes the fish had stayed with him. This project was pretty difficult because there were many things to account for, like all of the coordinates, movements, sounds, and captions. I had to refresh my canvas multiple times to make sure everything was playing out the way I wanted it to. I was tempted to import images into my code, but wanted to challenge myself, so I decided to create all the images and shapes/objects myself.
My 4 sounds consist of the following: a loud thunder that echoes, a rippling/swishing noise of water, a loud croaking of a frog, and a (very irritating) buzzing of a fly. I used the buzzing sound of the fly to make sure the viewer could understand that the shape I had created was some sort of bug/fly. With the frog, I wanted to make sure its croaking was heard after it ate the fly to show some emotion. With the loud thunder, I used it to make the story more dark and scary, followed by a sudden darkening of the sky. With the water noises, I used that to make the night sounds seem louder and make it more clear that the fish had swam away.
//Annie Kim
//anniekim@andrew.cmu.edu
//SectionB
//anniekim-10-project
/*
For my program, this is the general story line:
A lonely frog who is on a lilypad in the middle
of the water, is there with a fish. A fly comes near
the frog, and the frog eats it. Luckily, the frog is
happy because it was getting hungry, however it is not
so lucky for the fly. Then suddenly, lightning strikes,
and thunder echoes through the sky, and the sky turns dark.
The fish swims away in fear, and the frog is left alone again.
*/
var fly; //audio file names
var frogcroak;
var thunder;
var water;
var bug = {
x: 450, y: 180,
width: 45, height: 25
}
var lily = {
x: 110, y: 320,
width: 200, height: 90
}
var lily1 = {
x: 110, y: 320,
width: 200, height: 90
}
var tongue_move = {
x: 240, y: 220
}
var cloud = {
x: 50, y: 75
}
var crab = {
x: 400, y: 370,
}
var fish = {
x: 225, y: 440,
}
function preload() {
// call loadImage() and loadSound() for all media files here
fly = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/fly-1.wav")
frogcroak = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/frogcroak.wav")
thunder = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/thunder.wav")
water = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/water.wav")
}
function setup() {
// you can change the next 2 lines:
createCanvas(450, 480);
useSound();
frameRate(1);
}
function soundSetup() { // setup for audio generation
// you can replace any of this with your own audio code:
fly.setVolume(0.25);
frogcroak.setVolume(0.75);
thunder.setVolume(0.75);
water.setVolume(0.8);
}
function draw() {
//sky color
background(217, 243, 245);
//water
fill(150, 214, 250);
noStroke();
rect(0, 250, 450, 230);
//calling fish object
fishy();
//lilypad1 ~stagnant, doesnt move
strokeWeight(2);
stroke(100, 175, 0);
fill(150, 225, 0);
ellipse(lily1.x, lily1.y, lily1.width, lily1.height);
fill(150, 214, 250); //making cut into lily pad
noStroke();
triangle(lily1.x, lily1.y, lily1.x - 100, lily1.y + 15, lily1.x - 50, lily1.y + 60);
//calling cloud object
clouds();
//calling froggy object
froggy();
//fly & tongue movement + sound
if (frameCount >= 1 && frameCount <= 7) {
flying();
fill(0);
textSize(17);
strokeWeight(1);
textFont('HelveticaBold');
text("The fly accidentally gets too close to the hungry frog.", 10, 100);
tongue();
fly.play();
}
if (frameCount == 7) {
fly.stop(); //stops sound once fly disappears
}
if (frameCount >= 9 & frameCount <= 13) {
frogcroak.play();
}
if (frameCount == 13) {
frogcroak.stop();
}
if (frameCount == 25) {
background(0);
textSize(40);
fill(255);
strokeWeight(1);
textFont('HelveticaBold');
text("The End", 150, 240);
water.stop();
noLoop();
}
}
function flying() {
//creating 1st fly wing
fill(255);
stroke(0);
ellipse(bug.x + 10, bug.y - 12, bug.width - 15, bug.height - 10);
//creating fly body
fill(0);
noStroke();
ellipse(bug.x, bug.y, bug.width, bug.height);
//creating 2nd fly wing
fill(255);
stroke(0);
ellipse(bug.x + 12, bug.y - 10, bug.width - 15, bug.height - 10);
//making fly move
bug.x = bug.x - 65;
if (bug.x <= 200) {
bug.x = 200;
}
}
function froggy() {
//light green color
fill(117, 176, 111);
//legs
stroke(0);
strokeWeight(3);
ellipse(lily.x - 15, lily.y - 43, 35, 55);
ellipse(lily.x + 65, lily.y - 43, 35, 55);
//body
ellipse(lily.x + 25, lily.y - 50, 80, 90);
//feet
fill(117, 176, 111);
//arc(lily1.x - 3, lily1.y - 5, 40, 25, PI, 0);
arc(lily.x + 4, lily.y - 5, 40, 30, PI, 0, CHORD);
arc(lily.x + 46, lily.y - 5, 40, 30, PI, 0, CHORD);
//head
stroke(0);
ellipse(lily.x + 25, lily.y - 105, 100, 80);
//smile
fill(100, 0, 0);
arc(lily.x + 25, lily.y - 100, 70, 40, 0, PI, CHORD);
//ears
fill(117, 176, 111);
circle(lily.x, lily.y - 140, 38);
circle(lily.x + 47, lily.y - 140, 38);
noStroke();
circle(lily.x + 4, lily.y - 132, 36);
circle(lily.x + 43, lily.y - 132, 36);
//white part of eyes
stroke(0);
strokeWeight(2);
fill(255);
circle(lily.x + 47, lily.y - 140, 23);
circle(lily.x, lily.y - 140, 23);
//black of eyes
fill(0);
circle(lily.x + 47, lily.y - 140, 10);
circle(lily.x, lily.y - 140, 10);
//this will make the frog jump
if (frameCount >= 9 & frameCount <= 10) {
lily.y -= 150;
textSize(20);
strokeWeight(1);
textFont('HelveticaBold');
fill(0);
text("The frog jumps with joy because it was hungry.", 15, 380);
}
if (frameCount >= 10 & frameCount <= 11) {
lily.y += 150;
}
}
function tongue() {
strokeWeight(17);
stroke(100, 0, 0);
line(tongue_move.x, tongue_move.y - 10, 135, 230);
//moving tongue to catch fly
tongue_move.y -= 5;
tongue_move.x -= 5;
if (tongue_move.y <= 200) {
tongue_move.y = 200;
}
if (tongue_move.x <= 220) {
tongue_move.x = 220;
}
}
function clouds() {
if (frameCount >= 14 & frameCount <= 18) {
darksky();
lightning();
textSize(28);
fill(0);
textFont('HelveticaBold');
text("The sky shakes with thunder.", 100, 390);
thunder.play();
}
if (frameCount >= 19 & frameCount <= 25) {
darksky();
thunder.stop();
water.play();
}
fill(255);
//left cloud
circle(cloud.x, cloud.y, 100);
circle(cloud.x - 45, cloud.y, 70);
circle(cloud.x + 55, cloud.y, 55);
//right cloud
circle(cloud.x + 300, cloud.y - 30, 100);
circle(cloud.x + 255, cloud.y - 30, 70);
circle(cloud.x + 355, cloud.y - 30, 55);
//clouds moving
cloud.x += 5;
}
function lightning() {
fill(255, 255, 0);
quad(cloud.x, cloud.y, cloud.x - 20, cloud.y + 70, cloud.x + 10, cloud.y + 90, cloud.x + 30, cloud.y + 20);
quad(cloud.x + 2, cloud.y + 75, cloud.x - 10, cloud.y + 145, cloud.x + 12, cloud.y + 165, cloud.x + 32, cloud.y + 95);
}
function darksky() {
fill(160);
rect(0, 0, 450, 250);
}
function fishy() {
//body and tail
fill(237, 104, 74);
strokeWeight(1);
stroke(255, 0, 0);
ellipse(fish.x - 38, fish.y - 5, 17, 30);
ellipse(fish.x - 38, fish.y + 5, 17, 30);
ellipse(fish.x - 8, fish.y - 26, 30, 25);
ellipse(fish.x, fish.y, 70, 60);
//face of fish
fill(255, 122, 92);
ellipse(fish.x + 10, fish.y, 53, 54);
//eyes of fish
fill(255);
stroke(0);
circle(fish.x + 9, fish.y - 5, 18, 18);
circle(fish.x + 27, fish.y - 5, 18, 18);
fill(0);
circle(fish.x + 9, fish.y - 5, 6, 6);
circle(fish.x + 27, fish.y - 5, 6, 6);
//bottom fin
fill(237, 104, 74);
stroke(155, 0, 0);
triangle(fish.x, fish.y + 22, fish.x - 20, fish.y + 23, fish.x - 4, fish.y + 38);
//smile
noFill();
arc(fish.x + 18, fish.y + 10, 20, 10, 0, PI);
//fish is moving off of the canvas
if (frameCount >= 19 & frameCount <= 25) {
fish.x += 55;
fish.y -= 36;
fill(0);
textFont('HelveticaBold');
textSize(20);
text("The frog wishes the fish would stay.", 50, 450);
text("The frog doesn't want to be alone in the storm.", 50, 400);
}
}
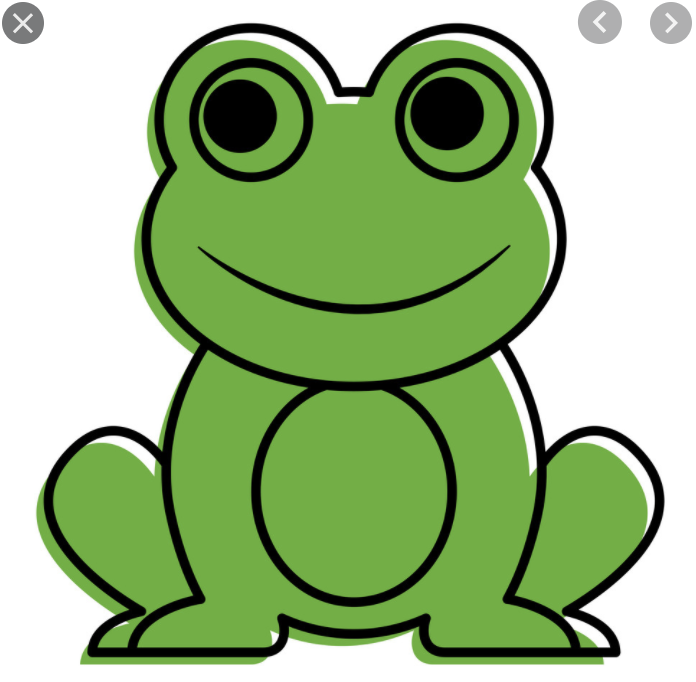