For my project, I wanted to create a composition based on an illustration idea I had, so I created an illustration first in Illustrator and then exported the layers as images. In my project, I was able to practice using images and sound together and ended up using 7 images and 5 sounds total (meow, car, bicycle, wind, chirping). I wanted to make part of the sketch interactive, so I made the sun follow the user’s mouse. My story is as follows:
It is fall and the sun is setting. A cat sits on the windowsill and meows, watching the traffic outside. A car passes by, then a bicycle. The wind blows and leaves rustle. Birds chirp in the distance.
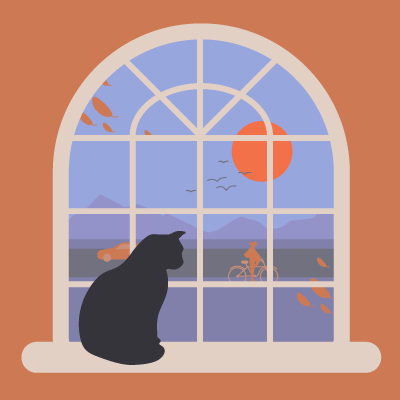
//Storyline: A cat sits on the windowsill and meows, watching
//traffic outside. A car passes by, then a bicycle. It is late
//fall and the sun is setting. Birds chirp in the distance.
//images
var cat;
var wind;
var leaf;
var bike;
var car;
var bird;
var mountains;
//sounds
var meow;
var engine;
var spinning;
var windblows;
var chirping;
//moving distances
var dx = 0;
var dy = 0;
function preload() {
//loads images
cat = loadImage("https://i.imgur.com/4Jqrrll.png");
wind = loadImage("https://i.imgur.com/jYEWld7.png");
leaf = loadImage("https://i.imgur.com/1rd8ozl.png");
bike = loadImage("https://i.imgur.com/u3DeUeL.png");
car = loadImage("https://i.imgur.com/4F1DaeZ.png");
bird = loadImage("https://i.imgur.com/aEcelIZ.png");
mountains = loadImage("https://i.imgur.com/kdIC817.png");
//loads sounds
meow = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/meow.wav");
engine = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/engine.wav");
spinning = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/spinning.wav");
windblows = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/windblows.wav");
chirping = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/chirping.wav");
}
function setup() {
createCanvas(400, 400);
frameRate(1);
useSound();
cat.loadPixels();
wind.loadPixels();
leaf.loadPixels();
bike.loadPixels();
car.loadPixels();
bird.loadPixels();
mountains.loadPixels();
}
function soundSetup() {
//setup for audio generation
meow.setVolume(1);
engine.setVolume(0.2);
spinning.setVolume(0.5);
windblows.setVolume(0.5);
chirping.setVolume(0.75);
}
function draw() {
background(204, 121, 84);
//plays sounds at correct times
switch (frameCount) {
case 4: meow.play(); break;
case 7: engine.play(); break;
case 12: spinning.play(); break;
case 18: windblows.play(); break;
case 23: chirping.play(); break;
}
//sky
noStroke();
fill(151, 166, 221);
ellipse(200, 180, 287);
//sun and mountains
fill(242, 113, 73);
ellipse(mouseX, mouseY, 60);
image(mountains, 57, 194);
//car drives by
if (frameCount >= 6 & frameCount < 13) {
image(car, 240-dx, 235);
dx+=25;
}
//bicycle passes by
if (frameCount >= 11 & frameCount < 20) {
image(bike, 15+dx, 240);
dx+=30;
}
//wind blows leaves
if (frameCount >= 17 & frameCount < 22) {
image(leaf, 80, 80+dy);
image(leaf, 280, 200+dy);
dy+=40;
}
//birds fly in distance
if (frameCount >= 23 & frameCount < 27) {
image(bird, 216, 187);
image(bird, 230, 175);
image(bird, 232, 150);
image(bird, 150, 155);
}
//window and cat
image(wind, 0, 0);
image(cat, 80, 230);
}