sketchDownload
/*
* Amy Lee
* amyl2
* Section B
*/
var count = 0;
var grayvalue = 255;
function preload() {
// loading image files
sheep = loadImage("https://i.imgur.com/ASPgqzI.png");
sheep2 = loadImage("https://i.imgur.com/E1rZIt0.png")
rabbit = loadImage("https://i.imgur.com/R9besKF.png");
turtle = loadImage("https://i.imgur.com/KzuH03l.png");
turtlefam = loadImage("https://i.imgur.com/6Wo9cT4.png?1")
musician = loadImage("https://i.imgur.com/NAApLxI.png");
musicnotes = loadImage("https://i.imgur.com/Q1cKMk2.png");
// loading sound files
gasp = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/gasp.wav");
cheer = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/cheer.wav");
guitar = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/guitar1.wav");
countdown = loadSound("https://courses.ideate.cmu.edu/15-104/f2020/wp-content/uploads/2020/11/countdown.wav");
}
function soundSetup() {
// Setup for audio generation
gasp.setVolume(.4);
cheer.setVolume(.6);
guitar.setVolume(.3);
countdown.setVolume(.5);
}
function setup() {
createCanvas(500, 600);
frameRate(15);
useSound();
// Set up text parameter
textAlign(CENTER, CENTER);
// Creating character objects
rab = new Object();
rab.image = rabbit;
rab.x = 60;
rab.y = 460;
rab.dy = 2;
rab.width = 200;
rab.height = 150;
rab.draw = rabbitDraw;
turt = new Object();
turt.image = turtle;
turt.x = 250;
turt.y = 450;
turt.dy = 1;
turt.width = 200;
turt.height = 150;
turt.draw = turtleDraw;
turtfam = new Object();
turtfam.image = turtlefam;
turtfam.x = 200;
turtfam.y = 700;
turtfam.dy = 2;
turtfam.width = 250;
turtfam.height = 150;
turtfam.draw = turtlefamDraw;
muse = new Object();
muse.image = musician;
muse.x = -100;
muse.y = 200;
muse.width = 330;
muse.height = 260;
muse.draw = musicianDraw;
// Normal sheep
she = new Object();
she.image = sheep;
she.x = 360;
she.y = 150;
she.dx = 1;
she.width = 200;
she.height = 150;
she.draw = sheepDraw;
// Shocked sheep
she2 = new Object();
she2.image = sheep2;
she2.x = 360;
she2.y = 150;
she2.width = 200;
she2.height = 150;
she2.draw = sheep2Draw;
notes = new Object();
notes.image = musicnotes;
notes.x = 80;
notes.y = 170;
notes.width = 40;
notes.height = 30;
notes.draw = musicnotesDraw;
}
function draw() {
background(125,45,45);
// Random speckles on race track
push();
for (var i = 0; i < 100; i ++){
fill(0);
ellipse(random(0,600),random(0,600),1.5,1.5);
}
pop();
// Starting Line and Dividers
fill(255);
noStroke();
rect(248,0,4,600);
rect(80,0,4,600);
rect(420,0,4,600);
rect(0,470,500,4);
// Checkered Finish Line
for(var y = 20; y <= 40; y += 20){
for (var x = 0; x <= 500; x += 20){
fill(grayvalue);
rect(x, y, 20, 20);
grayvalue = 255 - grayvalue;
}
grayvalue = 255 - grayvalue;
}
// Write "FINISH" near finish line
textSize(50);
fill(0);
text("F I N I S H", 250, 100);
fill(255);
text("1",100,500);
text("2",280,500);
print(frameCount);
rab.draw();
turt.draw();
muse.draw();
// Storyline
// Calling sounds at specific counts here:
if (count == 0){
countdown.play();
}
if (count == 75){
guitar.play();
}
if (count == 120){
gasp.play();
}
if (count == 450){
cheer.play();
}
if (count >= 0){
she.draw();
muse.draw();
}
// Make music notes move
if (count > 70 & count <= 660){
notes.draw();
notes.x += random(-2,2);
notes.y += random(-2,2);
if (notes.x <= 78 || notes.x >= 82){
notes.x = 80;
}
if (notes.y <= 168 || notes.y >= 172){
notes.y = 170;
}
}
// Moving images at specific counts
// Turtle and rabbit begin racing
if (count > 50 & count <= 60){
turt.y -= turt.dy;
rab.y -= rab.dy;
she.draw();
muse.draw();
}
// Musician starts playing, rabbit gets distracted and stops
// Turtle keeps going
if (count > 60 & count <= 80){
rab.y -= rab.dy;
turt.y -= turt.dy;
she.draw();
}
// turtle keeps going
// rabbit still distracted
if (count > 80 & count <= 110){
turt.y -= turt.dy;
rab.y -= rab.dy;
if (rab.y = 300){
textSize(13);
text("i love music",200,280);
rab.y = 300;
}
she.draw();
}
// Sheep shows up, gasps at surprise
// Turtle wins
if (count > 110 & count<= 120){
turt.y -= turt.dy;
she.draw();
}
if (count > 120){
textSize(13);
text("*shocked*",465,170);
turt.y -= turt.dy
if (turt.y <= 10){
turt.y = 10;
turtfam.draw();
turtfam.y -= turtfam.dy;
if (turtfam.y <= 100){
turtfam.y = 100;
}
}
she2.draw();
}
// Confetti celebration for when turtle wins
if (count >= 450){
confetti();
}
count ++;
}
function turtleDraw(){
image(turtle, this.x,this.y, this.width, this.height);
}
function rabbitDraw(){
image(rabbit,this.x,this.y, this.width, this.height);
}
function sheepDraw(){
image(sheep, this.x, this.y, this.width, this.height);
}
function sheep2Draw(){
image(sheep2, this.x, this.y, this.width, this.height);
}
function musicianDraw(){
image(musician, this.x, this.y, this.width, this.height);
}
function musicnotesDraw(){
image(musicnotes, this.x,this.y,this.width, this.height);
}
function turtlefamDraw(){
image(turtlefam, this.x,this.y,this.width, this.height);
}
function confetti(){
var cy = 0;
var cDy = 3;
for( i = 0; i <= 300; i++){
fill(random(255),random(255),random(255));
ellipse(random(width),cy, 4,4);
cy += cDy;
}
}
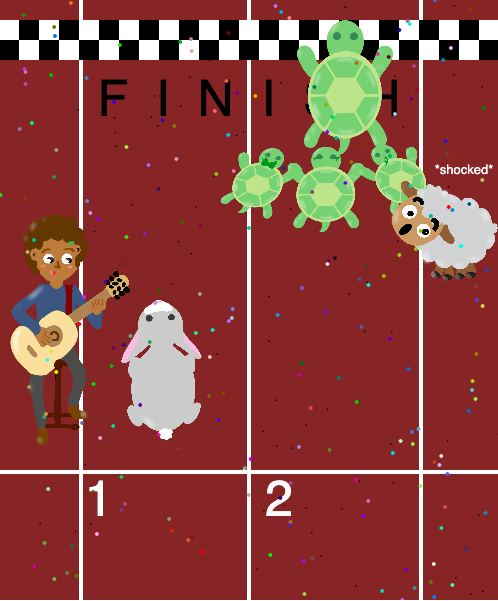
For my sonic story, I wanted to illustrate the class Tortoise and the Hare race. In this story, the rabbit is distracted by the music and stops racing, while the turtle takes it slow and continues to race. In the end, his win is celebrated by confetti and his family joining to congratulate him.
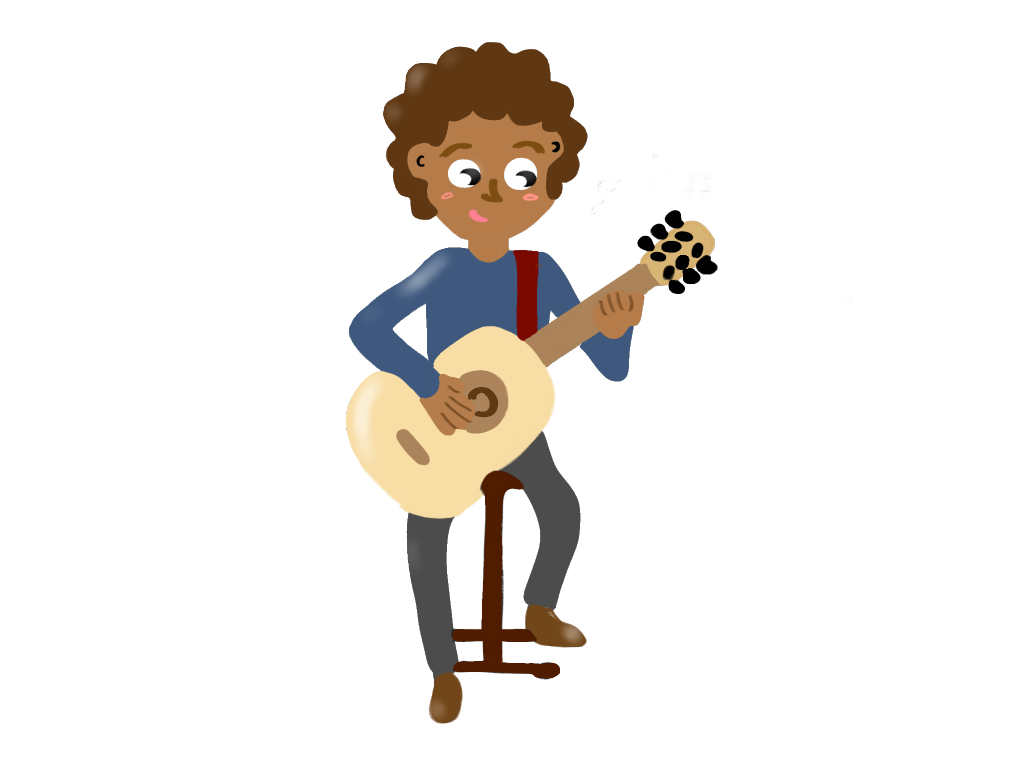
I also drew the characters separately in the “Sketchbook” app in order to get the transparent background for this project.