Virus Run
For my final project, I have created a side-scrolling game in which the player takes on the role of a coronavirus particle. As obstacles such as masks and hand sanitizer fly from right to left, the player must use the space bar to make the virus “jump” and avoid these obstacles. The longer the user holds down the key, the higher the virus floats, until it reaches the max height. If any of the obstacles are touched, the game stops. The score is then calculated based on the frame count. I was inspired by the dinosaur game which people can play when trying to use google with no internet connection, and if I had more time, I would have liked to make it so that the longer the game goes on, the more obstacles there are and the faster they move.
Some Screenshots of what the full canvas looks like:
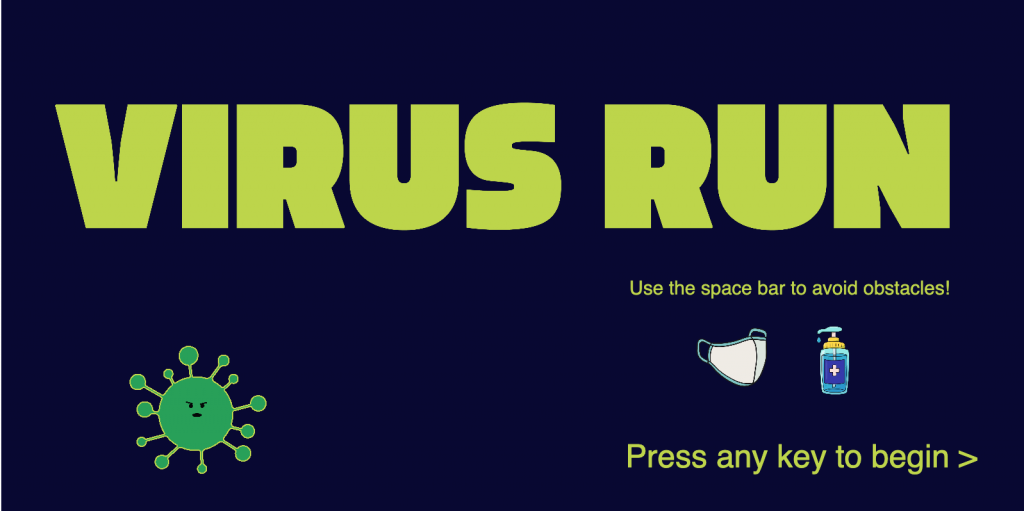
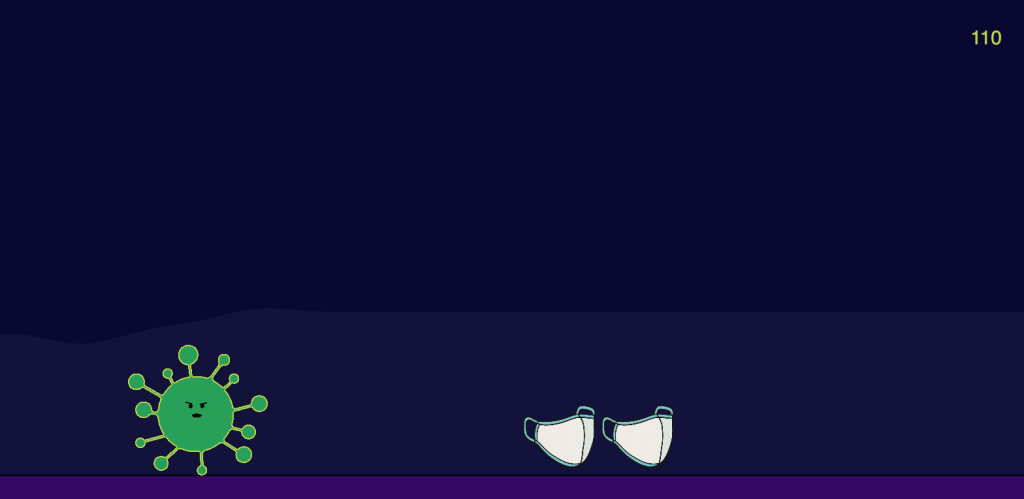
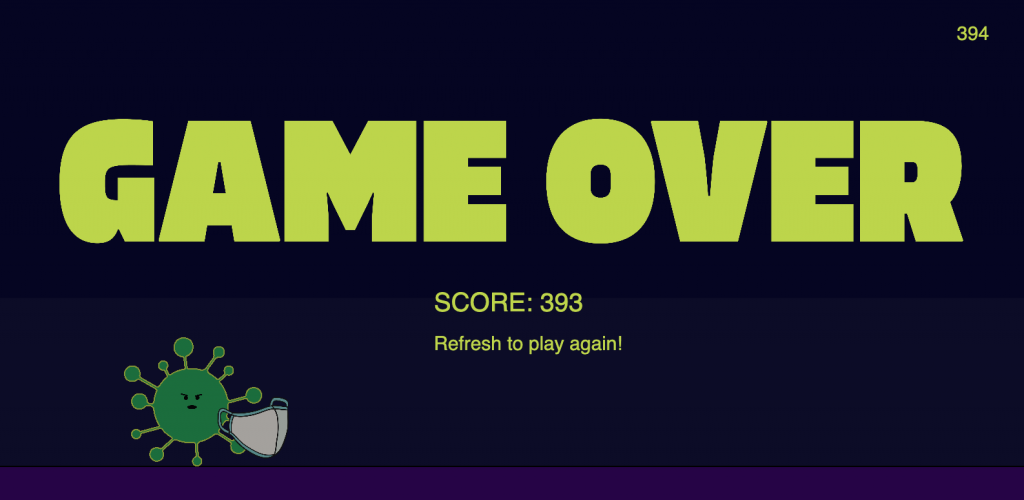
var imgV; // image of virus
var imgM; // image of mask obstacle
var imgHS; // image of hand sanitizer
var imgStart; // image for starting screen
var imgGO; // image for game over
var mask = []; // array for masks on ground level
var mx = 800 // x coord of mask
var handSan = []; // array for hand sanitizer flying overhead
var hsx = 800 // x coord of hand sanitizer
var virusX = 100; // permanent x position of virus
var virusY = 270; // starting y position of virus
var virusR = 70; // radius of virus
var virusDy = 0; // change in height of virus when jumping
var score = 0; //counts score based on frames
var start = true; // checks to see if starting page is showing
var hills = []; // making moving hills as a background element
var noiseParam = 0;
var noiseStep = 0.02;
function preload(){
imgV = loadImage("https://i.imgur.com/k04oKtW.png");
imgM = loadImage("https://i.imgur.com/v2CRQLt.png");
imgHS = loadImage("https://i.imgur.com/iFAELeQ.png");
imgGO = loadImage("https://i.imgur.com/ORHbmPV.png");
imgStart = loadImage("https://i.imgur.com/OUL56Za.png");
}
function setup(){
createCanvas(800, 400);
//making hills
for(var i = 0; i <= width/5; i++){
var n = noise(noiseParam);
hills[i];
var value = map(n, 0, 1, 0, height/2);
hills.push(value);
noiseParam += 0.5*noiseStep;
}
}
function draw(){
if (start == true){
startingPage();
} else {
background(8, 6, 51); // dark blue background
drawHills();
fill(55, 0, 104); // purple floor
rect(0, 370, 800, 30);
image(imgV, virusX, virusY, 107, 100); // image of virus
if(keyIsDown(32)){
virusY -= 10;
// while the space bar is down, the virus moves upward
} else if (virusY < 270){
virusY +=10;
// when the space bar is let go while the virus is in the air,
//the virus moves down
} else { virusY = 270; }
// otherwise, the virus stays on the ground
if (virusY < 40)
{ virusY += 10}
createNewMask();
displayMask();
createNewHandSan();
displayHandSan();
checkIfGameOver();
score += 1;
//display score count in the top right corner during the game
textSize(15);
fill(190, 215, 62);
text(score, 745, 40);
}
}
function startingPage(){ // starting page with instructions
push();
fill(8, 6, 51);
rect(-1, -1, 801, 801);
fill(190, 215, 62);
textSize(25);
text('Press any key to begin >', 487, 365);
textSize(15);
text('Use the space bar to avoid obstacles!', 490, 230);
pop();
image(imgStart, 40, 80, 700, 100);
image(imgM, 540, 250, 60, 58);
image(imgHS, 620, 247, 62, 70);
image(imgV, virusX, virusY, 107, 100);
}
function keyPressed(){
if(start == true){ start = false; } // game begins
}
//drawing hills (background element)
function drawHills(){
push();
translate(0, 130)
hills.shift();
var n = noise(noiseParam);
var value = map(n, 0, 1, 0, height/2);
hills.push(value);
// draws shape for sand
beginShape();
vertex(0, height);
for(var i = 0; i <= width/5; i++){
//filling the hill color
fill(255, 255, 255, 10);
noStroke();
//vertex function to fill the hills
vertex((i * 5), hills[i]);
vertex((i + 1) * 5, hills[i + 1]);
}
vertex(width, height);
endShape(CLOSE);
pop();
}
function checkIfGameOver (){
for (i = 0;i < mask.length;i++)
{
if ((dist(mask[i].x, 400, virusX, 400) <= virusR)
// tests if the mask comes within a certain distance of the virus
& virusY == 270){
gameOver(); // the screen goes darker
noLoop(); // the game stops
}
}
for (i = 0;i < handSan.length;i++){
if ((dist(handSan[i].x, handSan[i].y, virusX, virusY) <= virusR)){
//tests if the hand sanitizer comes within a certain distance of the virus
gameOver(); // the screen goes darker
noLoop(); // the game stops
}
}
}
// BEGIN CODE FOR MASK OBSTACLES
function displayMask(){ // update mask positions and display them
for(var i = 0; i < mask.length; i++){
mask[i].move();
mask[i].draw();
}
}
function createNewMask(){
// makes new masks with some random probability
if(random(0, 1) < 0.008){
mask.push(makeMask(800));
}
}
function moveMask(){
this.x -= 10;
}
function drawMask(){
mask.push();
image(imgM, this.x, this.y, 60, 60);
}
// function to make mask obstacle object
function makeMask(){
var m = {x: mx,
y: 310,
move: moveMask,
draw: drawMask
}
return m;
}
// BEGIN CODE FOR HAND SANITIZER OBSTACLES
function displayHandSan(){ // update hand sanitizer positions and display them
for(var i = 0; i < handSan.length; i++){
handSan[i].move();
handSan[i].draw();
}
}
function createNewHandSan(){
// makes new masks with some random probability
if(random(0, 1) < 0.005){
handSan.push(makeHandSan(800));
}
}
function moveHandSan(){
this.x -= 10;
}
function drawHandSan(){
handSan.push();
image(imgHS, this.x, this.y, 80, 90);
}
// function to make mask obstacle object
function makeHandSan(){
var h = {x: hsx,
y: random(30, 100),
move: moveHandSan,
draw: drawHandSan
}
return h;
}
function gameOver(){
// screen displayed when the virus hits an obstacle
push();
fill(0, 0, 0, 80);
rect(0, 0, 800, 400);
pop();
image(imgGO, 50, 100, 700, 100);
push();
fill(190, 215, 62);
noStroke();
textSize(20);
text('SCORE:' + ' ' + score, 340, 250);
textSize(15);
text('Refresh to play again!', 340, 280)
pop();
}