function setup() {
createCanvas(600, 500);
}
function draw() {
background(214, 204, 135);
//stars
for(var x = 25; x <= 600; x += 150) {
for(var y = 0; y <= 500; y += 160) {
push();
translate(x, y);
poopiB(x, y);
pop();
}
}
//pizza
for(var x = -50; x <=600; x += 150) {
for(var y = -50; y <= 500; y += 160) {
push();
translate(x, y);
pizza(x, y);
pop();
}
}
}
function poopiB(x, y) {
//head
stroke(0);
fill(255, 254, 143)
rect(35, 70, 25, 70, 17)
//hat
fill(0);
rect(32, 65, 31, 8)
rect(38, 35, 20, 30)
//hat detail
fill(35, 105, 94)
rect(38, 60, 20, 5)
//eyes
fill(255);
circle(35, 95, 18, 18);
circle(60, 95, 18, 18);
//pupils
strokeWeight(3);
fill(0);
point(35, 95);
point(60, 95);
//nose
strokeWeight(1);
noFill();
beginShape();
curveVertex(44.5, 102);
curveVertex(44.5, 102);
curveVertex(45.5, 112);
curveVertex(47.5, 115);
curveVertex(49.5, 112);
curveVertex(50.5, 102);
curveVertex(50.5, 102);
endShape();
//mouth
fill(105, 35, 37);
beginShape();
curveVertex(39, 118);
curveVertex(39, 118);
curveVertex(56, 118);
curveVertex(56, 121);
curveVertex(54, 122.5);
curveVertex(47.5, 125);
curveVertex(40.5, 122.5);
curveVertex(39.5, 121);
curveVertex(39, 119);
curveVertex(39, 119);
endShape();
fill(202, 66, 68);
beginShape();
curveVertex(44.5, 124);
curveVertex(44.5, 124);
curveVertex(45, 122);
curveVertex(46, 121);
curveVertex(49, 120.5);
curveVertex(52, 123.5);
curveVertex(51, 124.5);
endShape();
}
function pizza(x, y) {
//crust
stroke(0);
fill(208, 166, 116);
triangle(28, 75, 60, 55, 85, 85);
quad(24, 69.5, 85, 80, 85, 85, 24, 75);
quad(24, 69.5, 33, 70.5, 60, 50, 58, 47);
//cheese
fill(255, 248, 194);
triangle(28, 70, 60, 50, 85, 80);
//pepperoni
fill(223, 78, 73);
circle(45, 67, 8, 8);
circle(68, 70, 8, 8);
circle(59, 60, 8, 8);
}
I love Rick and Morty, and I wanted to create a wallpaper of the additional characters that show up in certain episodes. One of my favorite characters is Mr. Poopybutthole. My wallpaper is inspired by a scene in the show where he is also surrounded by pizza.
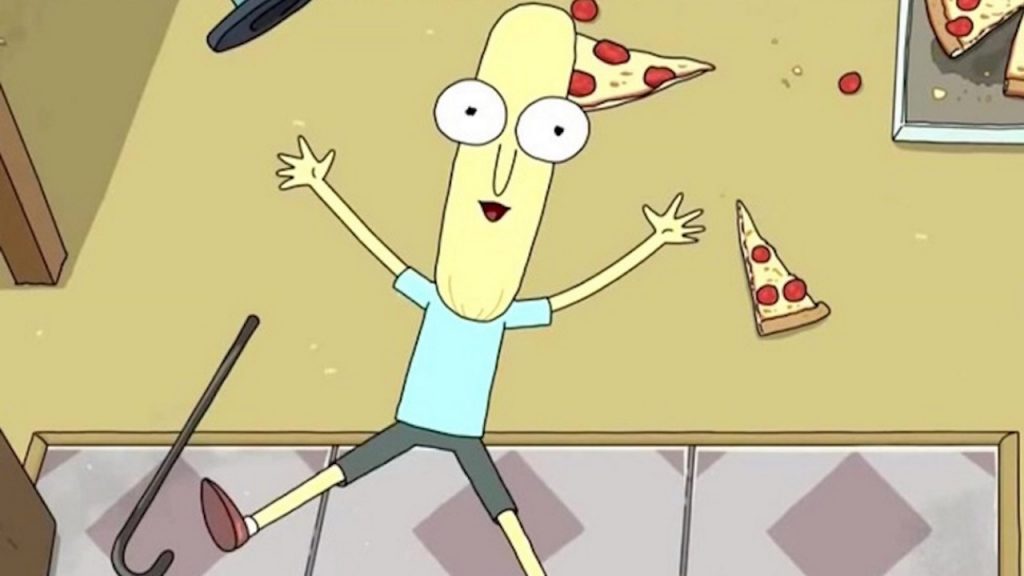