For my abstract clock, I was inspired by scenes of rainy days. First, I did a rough sketch of the various visual elements that I wanted to comprise the scene. Then, I made notes on the sketch of various elements that I wanted to be reactive to time. I decided that the color of the clouds should be reactive to the hour; as such, the cloud color gets darker with each hour of the day. Additionally, I made the sky change color for a range of hours during the day. The rain is generated depending on how many seconds have elapsed in the minute (e.g. 30 seconds into a minute, 30 lines of rain appear). Lastly, the width of the umbrella increases with each minute and resets at the start of each hour.
var rainX = [];
var rainY = [];
var buildingStart = [];
function setup() {
createCanvas(480, 480);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
for (i = 0; i < 10; i++){
buildingStart[i] = random(height/7,height/2);
}
}
function draw() {
noStroke();
if (hour() < 6){
background(117,128,181);
} else if(hour() >= 6 & hour() < 19){
background(68,117,181);
} else if (hour() == 19 ){
//sunset colors
background(160,24,85);
noStroke();
fill(237,100,21,150);
rect(0,0,width,3*height/4);
} else {
background(8,6,48);
}
cityscape();
//ground
fill(76,75,62);
rect(0,360,width,height);
fill(0,100);
rect(0,360,width,110);
baby();
rock();
rain();
cloud();
push();
translate(130,330);
rotate(radians(-30));
umbrella();
pop();
}
function cloud() {
fill(255 - (10*(hour())));
var circleX = 0;
var circleY = 0;
noStroke();
//cloud gets darker with each hour
ellipse(width/2,0,width,height/8);
//for loop was slowing down browser so I wrote out each circle
circle(circleX,circleY,height/3);
circle(circleX + 48,circleY - 10,height/3);
circle(circleX + 48*2,circleY,height/3);
circle(circleX + 48*3,circleY,height/3);
circle(circleX + 48*4,circleY,height/3);
circle(circleX + 48*5,circleY + 10,height/3);
circle(circleX + 48*6,circleY + 20,height/3);
circle(circleX + 48*7,circleY + 10,height/3);
circle(circleX + 48*8,circleY,height/3);
circle(circleX + 48*9,circleY,height/3);
circle(circleX + 480,circleY - 15,height/3);
}
function rain() {
for(i = 0; i <= second(); i++){
rainX[i] = random(0,480);
rainY[i] = 0;
stroke(175,199,216);
line(rainX[i],rainY[i],rainX[i],height - 10);
}
}
function baby() {
//shadow
fill(0,200);
ellipse(90,430,220,50);
//swaddling
fill(221,173,218);
rect(80,400, 60, 40);
circle(80,420,40);
circle(140,420,40);
//face
fill(173,156,133);
circle(130,420,38);
//eyes
if (hour() >= 20){
stroke(0);
strokeWeight(1.5);
line(130,405,130,415);
line(130,425,130,435);
} else {
fill(0);
circle(130,410,5);
circle(130,430,5);
}
}
function umbrella() {
//top of umbrella
fill(102,24,33);
arc(0,0,150 + minute(),90,PI,0,CHORD); //umbrella width increases with each minute
fill(25,16,10);
rect(0,0,3,100);
//handle
beginShape();
vertex(0,100);
curveVertex(0,110);
curveVertex(-5,115);
vertex(-10,105);
vertex(-10,108);
curveVertex(-5,118);
curveVertex(3,110);
vertex(3,100);
endShape(CLOSE);
//top
triangle(-1,-40,0,-60,1,-40);
}
function rock() {
fill(31,31,35);
quad(0,430,10,400,45,380,60,430);
fill(255,30);
triangle(10,400,45,380,30,420);
}
function cityscape() {
for(i = 0; i < 10; i++){
fill(0,100);
rect(48*i,buildingStart[i], 48, height);
}
}
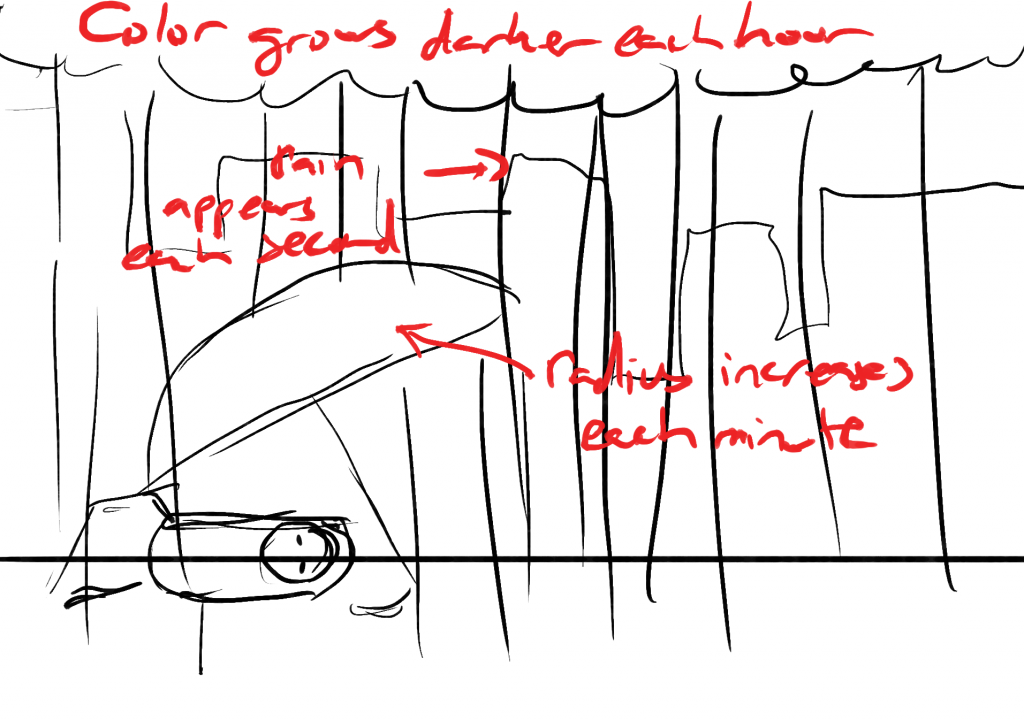