var c; //color
var hourcolor; //color of the hour flower
var mincolor; //color of the minute flower
var seccolor; //color of the second flower
var wintercolor = '#ffffff'; //color of the winter ground
var springcolor = '#c6ed4c'; //color of the spring ground
var summercolor = '#29d359'; //color of the summer ground
var fallcolor = '#f26f16'; //color fo the fall ground
function setup() {
createCanvas(480, 480);
hourcolor = color(255,72,72,200); //red
mincolor = color(255,46,131,200); //pink
seccolor = color(123,52,214,200); //purple
}
function draw() {
background('#5e99ef'); //blue sky
push();
flower(110,320-10*hour(),hourcolor); //hour flower
pop();
push();
flower(230,320-4*minute(),mincolor); //minute flower
pop();
push();
flower(350,320-4*second(),seccolor); //second flower
pop();
noStroke();
if (month() > 2 & month() <= 5) { //when it is spring--March, April, May
fill(springcolor);
rect(0,width-70,width,70);
} else if (month() > 5 & month() <= 8 ) { //when it is summer--June, July, August
fill(summercolor);
rect(0,width-70,width,70);
} else if (month() > 8 & month() <= 11) { //when it is fall--September, October, November
fill(fallcolor);
rect(0,width-70,width,70);
} else { //when it is winter--December, January, February
fill(wintercolor);
rect(0,width-70,width,70);
}
}
function flower(x,y,c) { //flower structure
translate(x,y);
noStroke();
fill('#86e55a'); //green
rect(-2,0,5,500); //flower stem
ellipse(-10,100,20,10); //stem leaf
ellipse(10,150,20,10); //stem leaf
ellipse(-10,200,20,10); //stem leaf
ellipse(10,250,20,10); //stem leaf
fill(c);
for (var i = 0; i < 5; i ++) { //flower petals
ellipse(0, 0, 20, 70);
rotate(PI/5);
}
fill('#ffd961'); //yellow
ellipse(0,0,30,30); //flower middle circle
}
Author: jae
Looking Outwards 06
Jae Son
Section C
Looking Outwards 06
I found Marius Watz’s “Abstract01.js” interesting. Using processing.js, he created this piece of computational art. I think it might be “pseudo-random” because of the composition. The composition/visual of the shape when mouse clicked is random yet has somewhat consistent shape. To make it complicated and random but organized at the same time, I think Watz has used probability distributions to bias randomness to get this kind of composition. I admire the randomness and organized system that coexist in this artwork. I like the interactivity of it, too. It draws the shape when I click the mouse, and I like how interactive input and randomness are both present in this artwork.
Looking Outwards 05: 3D Computer Graphics
Jae Son
Section C
Looking Outwards 05: 3D Computer Graphics
Olympthings by Branko Kolarevik and Ryan Cook was particularly interesting to me. It is a conceptual architecture of a tower. It is a conceptual work in which curates tower that is a repurposed structure of things changed through time. According to Kolarevik and Cook, “The interior is defined through advection and perlin noise algorithms which help to create openings and pathways for people to access. Variations of these algorithms are repeated throughout each structural layer to create a blurring effect when viewed from afar.” It is a theoretical work, so I am not sure of what kind of algorithms they used to generate this, but I suppose it will be something that is looped. I admire its creativity and imagination, and its beautifully executed composition and details. Massive amounts of series of lines are intertwined to create the tower architecture, and I admire this sensibilities in the detail and complex structure.
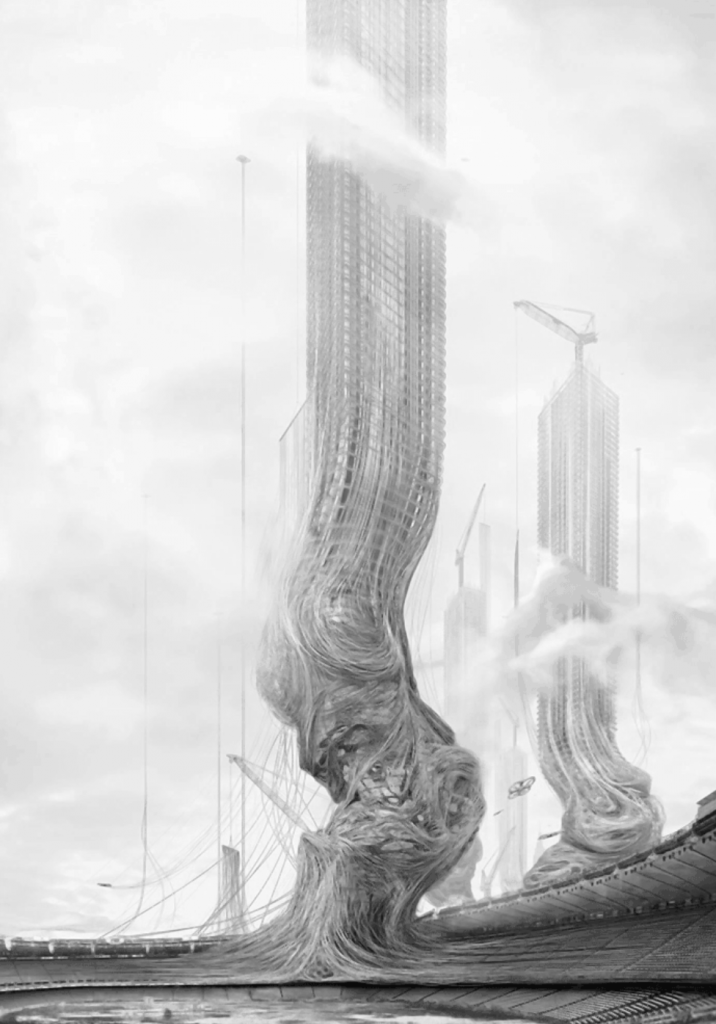
Project-05-Wallpaper
//Jae Son, Section C, hyunjaes@andrew.cmu.edu,Project-05-Wallpaper
function setup() {
createCanvas(600, 400);
background(220);
}
function draw() {
background(247,242,210); //beige background
//columns of yellow square that have 2 squares
for(y = 100; y <= 300 ; y += 200){
for(x=0; x <= 600 ; x += 200){
push();
yellowbox(x,y);
pop();
}
}
//columns of yellow square that have 3 squares
for (y = 0 ; y <= 400; y+=200){
for (x=100; x < 600 ; x +=200) {
push();
yellowbox(x,y);
pop();
}
}
//green line that is rotated 7*PI/4
for (y = 80; y >= -165; y -= 75){
for (x = 142; x <= 568; x +=142){
push();
greenline(x,y);
pop();
}
}
//green line that is rotated PI/4
for (x = -142; x <= 284; x +=142){
push();
greenline2(x,340);
pop();
}
//columns of yellow square that have 2 squares
for (y = 95; y <= 295; y += 200){
for (x = -12; x <= 588; x +=200){
push();
heart(x,y);
pop();
}
}
//columns of heart shapes that have 3 heart shapes
for (y = -5; y <= 395; y +=200 ){
for(x=88; x<=488; x +=200){
push();
heart(x,y);
pop();
}
}
}
//yellow sqaure
function yellowbox(x,y) {
noStroke();
rectMode(CENTER);
translate(x,y);
rotate(PI/4);
fill(248,228,87);
rect(0,0,90,90);
}
//green line
function greenline(x,y){
noStroke();
rectMode(CENTER);
rotate(PI/4);
translate(x,y);
fill(180,223,90);
rect(0,0,16,680);
}
//green line
function greenline2(x,y){
noStroke();
rectMode(CENTER);
rotate(7*PI/4);
translate(x,y);
fill(180,223,90);
rect(0,0,16,750);
}
//heart shape
function heart(x,y){
noStroke();
translate(x,y);
fill(237,132,103);
ellipse(0,0,34,34);
ellipse(25,0,34,34);
triangle(-14,10,12,37,38.5,10);
}
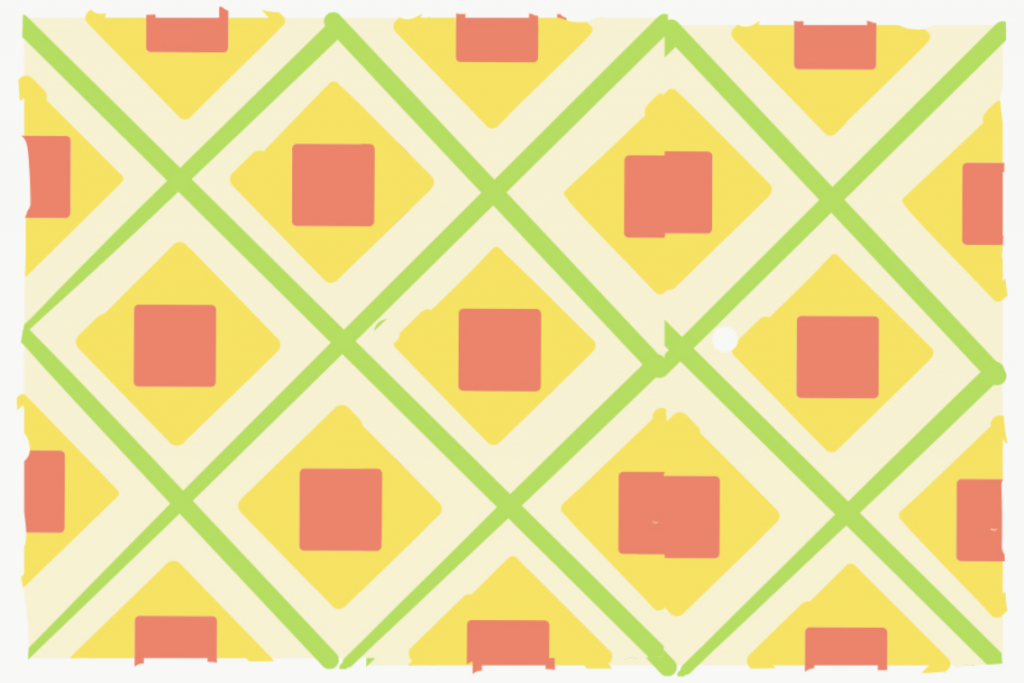
LookingOutwards-04
Laetitia Sonami’s signature instrument, the Lady’s Glove, was particulary interesting to me. It is fitted with a vast array of sensors which track the motions of her dance, and those movements shape the music. It is very interesting and admirable that she takes the physical movements and process them digitally to generate the sound into the physical world again. It is very unconventional and innovative. The signals go through Sensorlab at STEIM Institute, and are mapped onto MAX-MSP software. Also, the mapping and sonic material changes in every composition. Signals control sound parameters and processes. They can also control motors, light bulbs, and video too. It is very interesting how intuitive body movement would result into digitally processed sound art.
Project-04-String-Art
I created a string art in which consists of lines that are sort of symmetric and floating lines according to the position of mouse.
//Jae Son, Section C
function setup() {
createCanvas(400, 300);
background(220);
}
function draw() {
background(55,40,39);
for (var i = 0; i <= 250; i+=2){
strokeWeight(1);
//yellow line -left
stroke(240,227,30);
line(i*2, height, 0, 3*i);
//purple line -left
stroke(104,99,154);
line(i*5, height, 0, 3*i);
//pink line -left
stroke(181,134,132);
line(i*8, height, 0, 3*i);
//yellow line -right
stroke(240,227,30);
line(width,i*2,3*i,0);
//purple line -right
stroke(104,99,154);
line(width,i*5,3*i,0);
//pink line -right
stroke(181,134,132);
line(width,i*8,3*i,0);
}
for (var i = 0; i <= 300; i++) {
strokeWeight(0.5);
//moving orange line
stroke(249,211,140);
line(mouseX,i*3,i*2,mouseY);
//moving turquoise line
stroke(148,224,226);
line(i*3,mouseY,mouseX,i);
}
}
Looking Outwards 3
Looking at Andrew Kudless’s works was very inspiring. Its complexity in its form, unity, and the pattern is very beautifully constructed. I love how computationally generated pattern can result in an amazing physical object. I am not sure of what kind of algorithms were used to generate these works, but I guess it would be the recursive one which generates pattern. I love how they are beautifully designed yet conceptual and abstract that it evokes curiosity. I was surprised how there are multiple layers of parameters that are used to create those works. One of his works, Honeycomb Morphologies, is beautifully executed. It “explores integration strategies for a particular industrially produced material system for use in architectural applications.” I love how it is theoretical, numerical, and patterned, yet it has architectural system to it.
https://www.matsys.design/honeycomb-morphologies
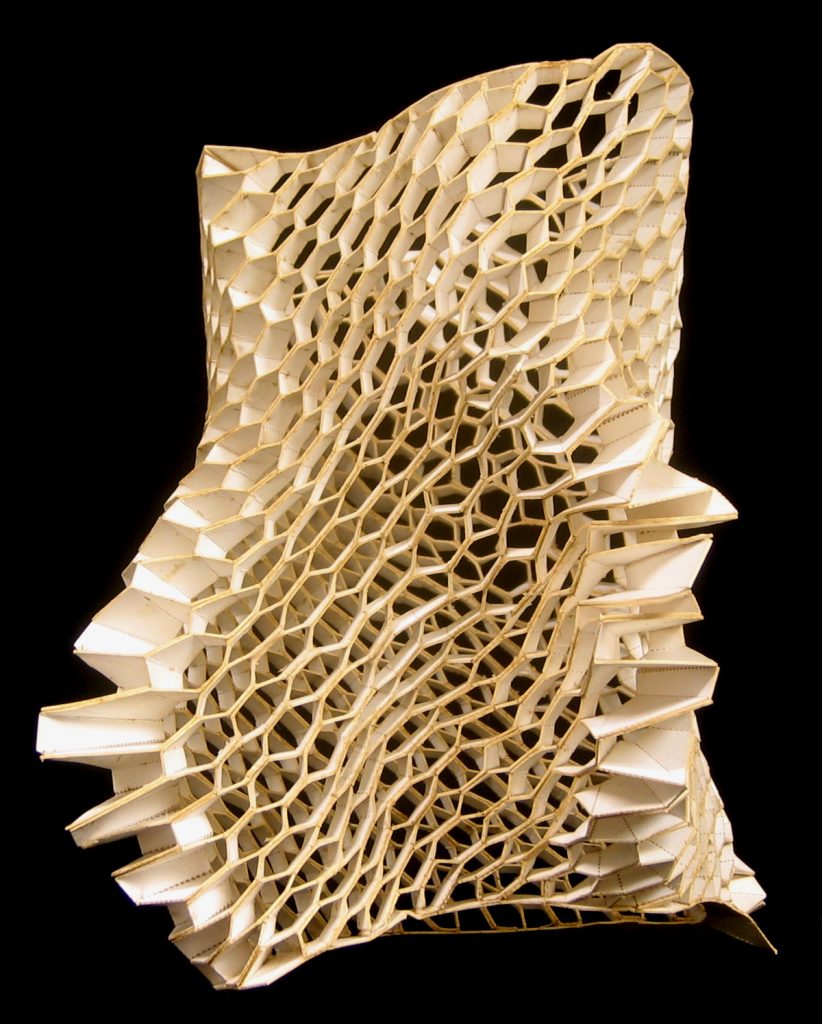
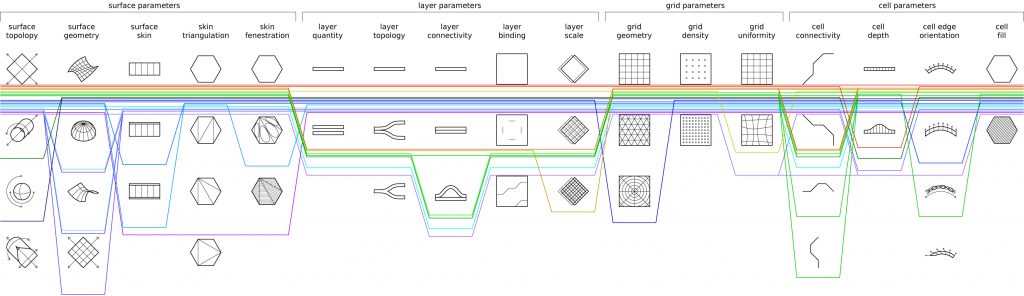
Project-3-Dynamic Drawing
I chose to show the clock and time with the day/night change, along with the alarm bell going on and off.
//Jae Son, section C
var x = 0;
var y = 0;
var angle = 0;
function setup() {
createCanvas(640, 450);
}
function draw() {
if(mouseX<320){ //when mouse is on the left side
background(151,215,240); //it is during the day - light blue background
noStroke();
fill(255,150,60);
ellipse(mouseX,80,100,100); //orange sun is up
} else { //when mouse is on the right side
background(31,41,84); //it is during the night - dark blue background
noStroke();
fill(255,227,90);
ellipse(mouseX,80,100,100); //yellow moon is up
}
fill(46,178,187);
noStroke();
quad(230,360,250,360,270,300,250,300); //clock leg
quad(380,322,400,322,410,360,390,360); //clock leg
push();
translate(250,130);
rotate(PI*3/4);
rect(0,0,25,35); //bridge between the clock body and the bell
translate(-70,-110);
rotate(PI/2);
rect(-5,25,25,35); //bridge between the clock body and the bell
pop();
arc(220,125,mouseY/3.5,mouseY/3.5,PI*3/4,PI*7/4); //alarm is on when mouse is on the bottom - bell is bigger
arc(415,125,mouseY/3.5,mouseY/3.5,PI*5/4,PI/4) //alarm is on when mouse is on the bottom - bell is bigger
ellipse(320,225,250,250); //clock body frame
fill(255);
ellipse(320,225,220,220); //clock body
fill(46,178,187);
ellipse(320,225,20,20); //clock center
push();
fill(46,178,187);
translate(318,225);
rotate(radians(30)+mouseX/100); //clock hour hand rotates clockwise
rect(0,0,5,80);
pop();
}
Project 2 – Variable Faces
var facewidth = 200;
var faceheight = 200;
var lefteary = 149; //y coordinate of the left ear
var righteary = 149; //y coordinate of the right ear
var eyebrowx1 = 295; //x coordinate of the left eyebrow
var eyebrowy1 = 230; //y coordinate of the left eyebrow
var eyebrowx2 = 345; //x coordinate of the right eyebrow
var eyebrowy2 = 230; //y coordinate of the right eyebrow
var eyewidth = 15;
var eyeheight = 15;
var mouthwidth = 15;
var mouthheight = 11;
var cheekwidth = 65;
var cheekheight = 65;
function setup() {
createCanvas(640, 480);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(255,243,169);
noStroke();
fill(255,197,197);
ellipse(266,lefteary,70,172); //left ear
ellipse(373,righteary,70,172); //right ear
ellipse(320,267,facewidth,faceheight); //face
fill(255,171,195);
ellipse(250,291,cheekwidth,cheekheight);//left cheek
ellipse(389,291,cheekwidth,cheekheight); //right cheek
fill(107,43,43);
ellipse(286,251,eyewidth,eyeheight);//left eye
ellipse(353,251,eyewidth,eyeheight); //right eye
rect(313,268,mouthwidth,mouthheight); //mouth
strokeWeight(5);
stroke(107,43,43);
line(277,230,eyebrowx1,eyebrowy1); //eyebrow left
line(eyebrowx2,eyebrowy2,362,230); //eyebrow right
}
function mousePressed(){
eyebrowy1 = random(225,235);
eyebrowy2 = random(225,235);
eyewidth = random(5,30);
eyeheight = random(5,30);
mouthwidth = random(5,30);
mouthheight = random(5,30);
cheekwidth = random(40,70);
cheekheight = random(20,70);
lefteary = random(120,170);
righteary = random(120,170);
}
LO2- Generative Art
I really liked Allison Parrish’s Compasses.
http://sync.abue.io/issues/190705ap_sync2_27_compasses.pdf
It “has two parts: a “speller,” which spells words based on how they sound, and a “sounder-out,” which sounds out words based on how they’re spelled. In the process of sounding out a word, the “sounder-out” produces a fixed-length numerical vector, known as a “hidden state,” which is essentially a condensed representation of a word’s phonetics. The “speller” can then use the phonetic information contained in this hidden state to produce a plausible spelling of the word.” (http://portfolio.decontextualize.com/) It really drew my eye on how it’s reproducing what has been produced. I like how it’s poetic and artistic yet very dry at the same time because of its mechanical nature. I have no idea of how she created the algorithm to generate this work, but I guess it’s some kind of machine learning. I liked how she took the multiple layers of the words and regenerate different forms of the words.
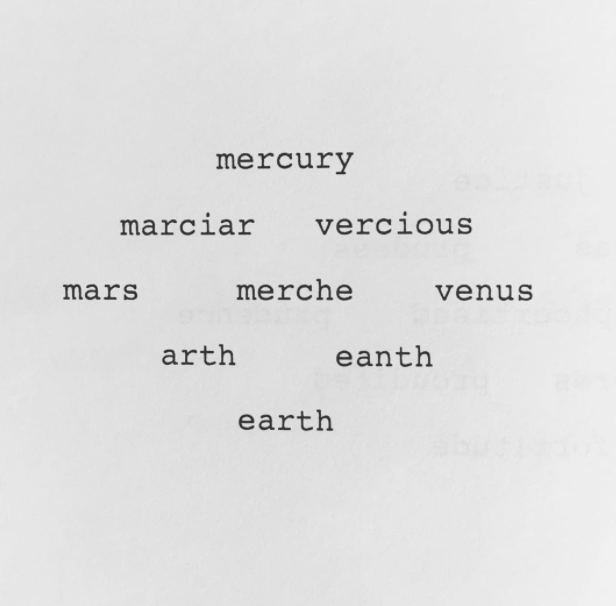