function setup() {
createCanvas(480, 480);
background(240, 213, 214);
}
function draw() {
background(240, 213, 214);
noStroke();
push();
rectMode(CENTER);
//dark pink shadow
fill(208, 186, 187);
ellipse(240, 435, 165, 30);
//plant pot
fill(186, 107, 60);
rect(240, 405, 75, 65);
rect(240, 360, 88, 20);
//plant pot shading
fill(165, 95, 52);
rect(240, 372, 75, 4);
pop();
//soil filling up represents seconds
fill(146, 85, 46);
var s = second();
rect(205, 435, 70, -s);
//ombre of pink tracking 10 minute intervals
//0 - 10 minute mark
fill(243, 209, 210);
rect(0, 245, 480, -20);
//10 - 20 minute mark
fill(238, 206, 208);
rect(0, 225, 480, -20);
//20 - 30 minute mark
fill(234, 202, 204);
rect(0, 205, 480, -20);
//30 - 40 minute mark
fill(230, 198, 201);
rect(0, 185, 480, -20);
//40 - 50 minute mark
fill(224, 194, 196);
rect(0, 165, 480, -20);
//50 - 60 minute mark
fill(220, 190, 195);
rect(0, 145, 480, -20);
//resetting the background past the 60 minute mark
fill(216, 187, 190);
rect(0, 125, 480, -150);
//plant stem growing represents minutes
fill(30, 71, 42);
var m = minute();
rect(237, 350, 5, -50-m*3);
//plant leaves
push();
fill(30, 71, 42);
translate(224, 320);
rotate(radians(-60));
ellipse(0, 0, 18, 43);
pop();
push();
fill(30, 71, 42);
translate(256, 320);
rotate(radians(60));
ellipse(0, 0, 18, 43);
pop();
//flower petals represents hours
var h = hour();
var hours = map(m, 0, 60, 260, 390);
if (h < 24 & h > 12){
h -= 12;
}
if (h == 0){
h = 12;
}
for (var i = 0; i < h; i++){
push();
translate(240, height - 0.9*hours);
rotate(radians(30*i));
//flower petals
fill(233, 160, 167);
ellipse(0, 30, 20, 55);
//flower center
fill(249, 213, 139);
ellipse(0, 0, 30, 30);
pop();
}
}
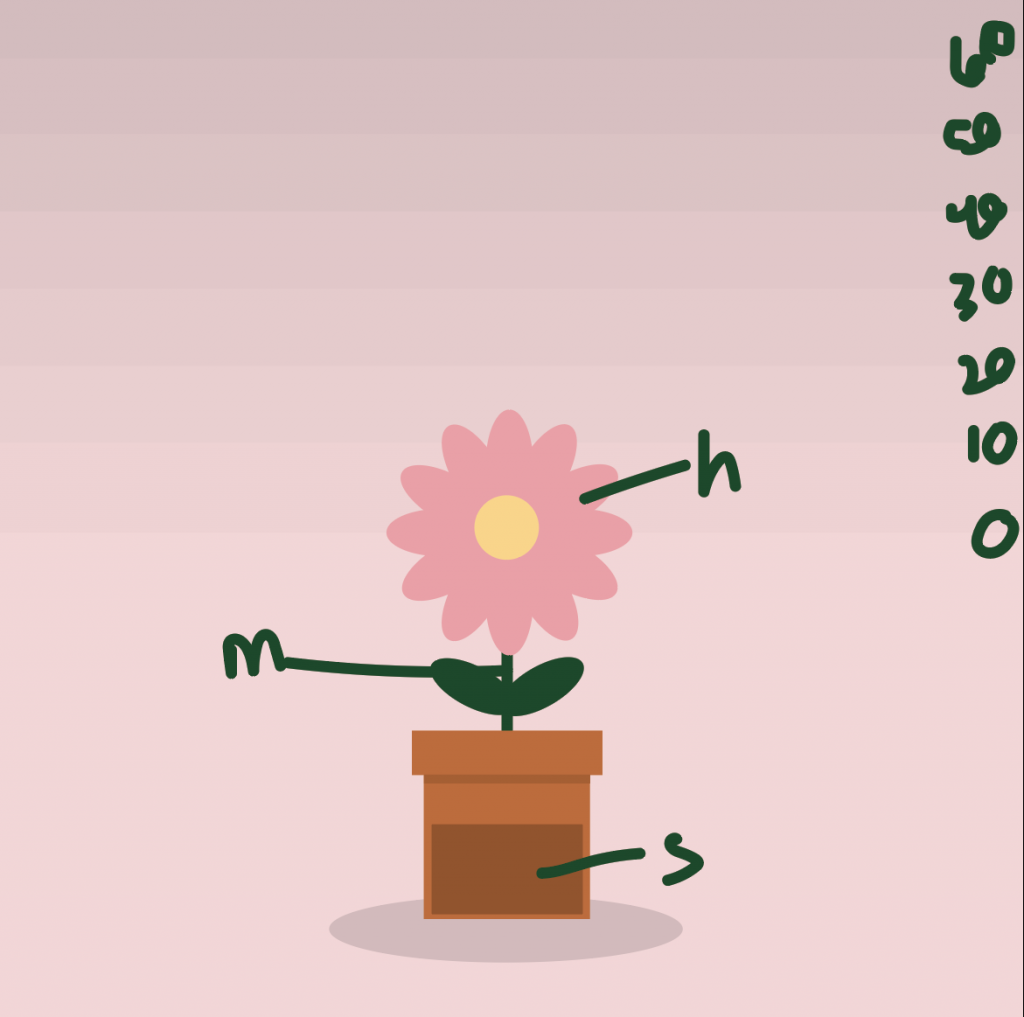
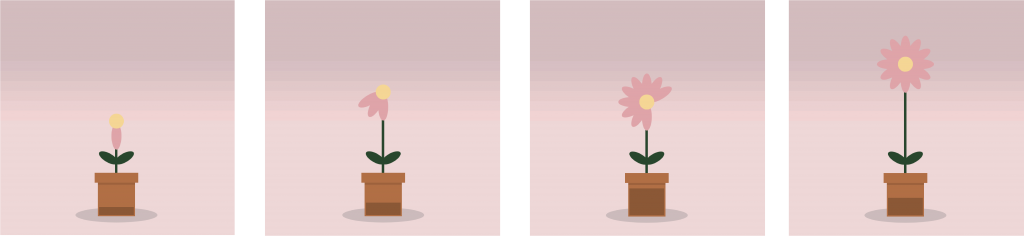
I wanted to create an abstract clock based on a plant growing over time. The pot filling up with soil represents seconds, the plant stem growing represents minutes, and the number of petals represents hours. The ombre of pink rectangles in the background track the minutes based on the position of the yellow center in 10 minute intervals. I had to make a few changes to the design based on where the center landed, which was challenging but once I figured out the map function it was fun to create!