// Sarah Luongo
// Section A
// This code aims to tell time via a buttefly, with the body filling to blue every second, purple dots being added every minute, and the background changing every hour.
var r = 12;
var g = 16;
var b = 30;
function setup() {
createCanvas(400, 400);
}
function draw() {
// Background changes different shades of blue every hour
H = hour();
// Black - medium blue from 12 AM - 7 AM
if (H<8){
background(r*H, g*H, b*H);
// Medium blue - light blue from 8 AM - 4 PM
} else if (H >= 8 & H <= 16) {
background(r*H, g*H, 245*H);
// Light blue - dark blue from 5 PM - 11 PM
} else {
background(192 - (r+13)*(H-16), 255 - (g+20)*(H-16), 255 - b*(H-16));
}
translate(width/2, height/2);
// Pink wings for the butterfly
fill(255, 50, 123);
butterflyW();
// Body of the butterfly
fill(0); // Black
ellipse(0, 0, 6, 100);
// Body filling to a blue color every second
fill(120, 150, 160);
var S = map(second(), 0, 59, 0, 100);
ellipse(0, 0, 6, S);
// Purple dots added every minute
fill(75, 0, 110);
rotate(radians(45));
for (var j = 0; j < minute(); j++) {
// Bottom right wing
if(j <= 15) {
circle(j*12+13, 0, 10);
// Top left wing
} else if(j <= 30) {
circle(-(j-15)*12-7,0,10);
// Bottom left wing
} else if (j <= 45) {
rotate(radians(90));
circle((j-30)*12+7,0,10);
rotate(radians(-90));
// Top right wing
} else {
rotate(radians(90));
circle(-(j-45)*12-7,0,10);
rotate(radians(-90));
}
}
}
function butterflyW() {
var da = PI/100;
beginShape();
for (var i = 0; i <= 2*PI; i += da) {
var w = sin(2*i) * width/2;
var x = w * cos(i);
var y = w * sin(i);
vertex(x, y);
}
endShape(CLOSE);
}
I wanted to do a butterfly to tell time for this project because I was inspired by one of the many faces on my apple watch. Also, I used to love catching butterflies when I was younger (and letting them go of course). I think there are a lot of beautiful butterflies out there. I would’ve like to do a more exquisite butterfly, but I felt it was better to try and have the assignment requirements down before designing anything too crazy. I was happy to at least show a little design to the butterfly with the addition of purple dots every minute.
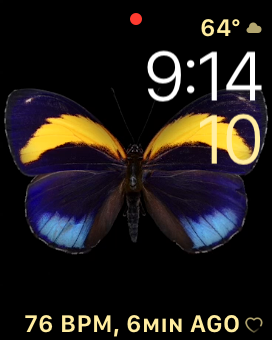