// Yoo Jin Kim
// 15104 - section D
// yoojink1@andrew.cmu.edu
//Project-05
function setup() {
createCanvas(490, 490);
noLoop();
}
function draw() {
background(145,204,236);
noStroke();
//repetition of butter on plate as wallpaper
for (var x = 0; x < width; x += 80) {
for (var y = 0; y < height; y += 80 ) {
push();
translate(x, y);
drawButter();
pop();
}
}
//repetition of milk as wallpaper
for (var x = 0; x < width; x += 80) {
for (var y = 0; y < height; y += 80 ) {
push();
translate(x - 60, y - 60);
drawMilk();
pop();
}
}
//orange juice
fill(255,140,0);
ellipse(197, 189, 12, 3);
quad(191, 189, 203, 189, 203, 202, 191, 202);
ellipse(197, 202, 12, 3);
}
//milk drawing
function drawMilk() {
//milk cup - gray
fill(207, 212, 214);
ellipse(97, 79, 14, 3);
quad(90, 79, 104, 79, 104, 103, 90, 103);
ellipse(97, 103, 14, 3);
//milk - white
fill(255);
ellipse(97, 89, 12, 3);
quad(91, 89, 103, 89, 103, 102, 91, 102);
ellipse(97, 102, 12, 3);
}
//butter drawing
function drawButter() {
//plate dark side - light gray
fill(212, 211, 206);
quad(66, 49, 75, 55, 51, 63, 42, 55);
//plate right light side - white
fill(233,229,234);
quad(75, 55, 75, 56, 51, 64, 51, 63);
//plate left light side - white
quad(42, 55, 51, 63, 51, 64, 42, 56);
//butter light side - light yellow
fill(255, 229, 155);
quad(49, 50, 67, 45, 71, 49, 53, 55);
//butter light side (cut piece)
quad(49, 56, 53, 61, 46, 62, 42, 57);
//butter medium dark side - medium dark yellow
fill(236,195,93);
quad(49, 50, 53, 55, 53, 61, 49, 56);
//butter medium side (cut piece)
quad(42, 57, 46, 61, 46, 62, 42, 58);
//butter dark side - dark yellow
fill(218,177,72);
quad(71, 49, 71, 55, 53, 61, 53, 55);
//butter dark side (cut piece)
quad(46, 61, 53, 60, 53, 61, 46, 62);
}
I personally love the butter and milk emojis so I wanted to make a wallpaper containing these emojis. It looks quite simple from far away, but I tried to detail the butter as much as I could to replicate the emoji in my iPhone as closely as possible. I added a slight randomness of orange juice for visual playfulness.
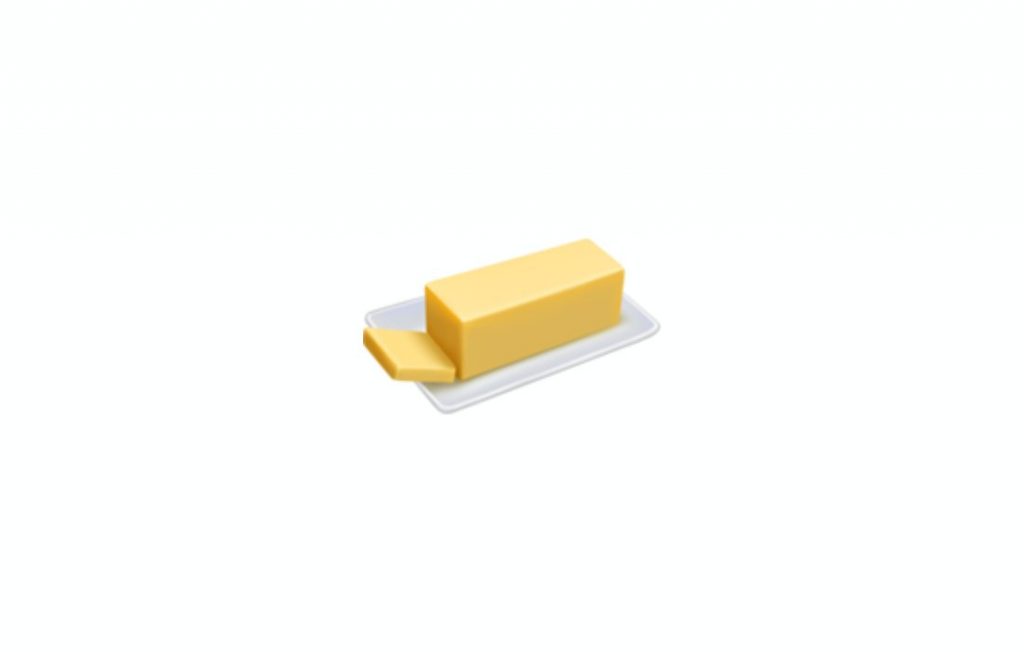