var buildings = [];
var mountains = [];
var trees = [];
var noiseParam = 0;
var noiseStep = 0.01;
var people = [];
var img;
function preload(){
//calling the superman image
img = loadImage("https://i.imgur.com/21p2JQR.png");
}
function setup() {
createCanvas(480, 480);
imageMode(CENTER);
// create an initial collection of buildings
for (var i = 0; i < 10; i++){
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
// mountains
for (var i = 0; i <= width/4; i++) {
var value = map(noise(noiseParam), 0, 1, height * 0.2, height * 0.9);
mountains.push(value);
noiseParam += noiseStep;
}
}
function draw() {
background(204, 241, 255);
//drawing clouds
cloud(50, 70);
push();
scale(0.7);
cloud(480, 150);
pop();
drawMountains(); //drawing the mountains
displayStatusString();
displayHorizon();
//drawing the buildings
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
image(img, 250, 150, img.width * 0.2, img.height * 0.2); //superman
//drawing the trees
updateAndDisplayTrees();
removeTreesThatHaveSlippedOutOfView();
addNewTreesWithSomeRandomProbability();
//drawing the people
updateAndDisplayPeople();
removePeopleThatHaveSlippedOutOfView();
addNewPeopleWithSomeRandomProbability();
}
function cloud(x, y){
//drawing the cloud
push();
translate(x, y);
noStroke();
fill(255, 237, 209, 95);
ellipse(0, 10, 70, 50);
ellipse(25, 0, 90, 60);
ellipse(50, 10, 80, 45);
pop();
}
//Buildings
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
//removing the buildings
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep; // remember the surviving buildings
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.015;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
// method to update position of building every frame
function buildingMove() {
this.x += this.speed;
}
// draw the building and some windows
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
fill(59, 107, 130);
stroke(100);
push();
translate(this.x, height - 40);
rect(0, -bHeight, this.breadth, bHeight);
stroke(220);
fill(207, 226, 232);
if (bHeight > 55){
for (var i = 0; i < this.nFloors; i++) {
rect(5, -15 - (i * floorHeight), this.breadth - 40, 10);
rect(35, -15 - (i * floorHeight), this.breadth - 40, 10);
}
}else{
fill(255, 246, 230);
rect(0, -bHeight, this.breadth, bHeight);
noStroke();
fill(240, 182, 74);
triangle(-10,-bHeight,this.breadth/2,-bHeight-30,this.breadth+10,-bHeight);
fill(150, 100, 45);
rect(20, 0, this.breadth - 40, -20);
}
pop();
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 60,
speed: -3.5,
nFloors: round(random(2,8)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
function drawMountains(){
mountains.shift();
var value = map(noise(noiseParam), 0, 1, height * 0.2, height * 0.9);
mountains.push(value);
noiseParam += noiseStep;
stroke(135, 173, 141);
fill(135, 173, 141);
beginShape();
vertex(0, height);
for (var i = 0; i < width/4; i++) {
vertex(i*5, mountains[i]);
vertex((i+1)*5, mountains[i+1]);
}
vertex(width, height);
endShape();
}
//drawing trees
function updateAndDisplayTrees(){
for (var i = 0; i < trees.length; i++){
trees[i].move();
trees[i].display();
}
}
function removeTreesThatHaveSlippedOutOfView(){
var treesToKeep = [];
for (var i = 0; i < trees.length; i++){
if (trees[i].x + trees[i].breadth > 0) {
treesToKeep.push(trees[i]);
}
}
trees = treesToKeep;
}
function addNewTreesWithSomeRandomProbability() {
var newTreeLikelihood = 0.025;
if (random(0,1) < newTreeLikelihood) {
trees.push(makeTree(width));
}
}
function treeMove() {
this.x += this.speed;
}
function makeTree(birthLocationX) {
var trees = {x: birthLocationX,
breadth: 20,
speed: -3.5,
treeHeight: round(random(50,100)),
move: treeMove,
display: treeDisplay}
return trees;
}
function treeDisplay() {
push();
translate(this.x, height - 30);
noStroke();
fill(107, 83, 71);
rect(-5, 0, 10, 15); //tree trunk
fill(66, 161, 61);
//leaves
triangle(-this.breadth+10, -30, 0, -this.treeHeight, this.breadth-10, -30);
triangle(-this.breadth+5, 0, 0, -this.treeHeight*0.7, this.breadth-5, 0);
pop();
}
//Drawing people
function updateAndDisplayPeople(){
for (var i = 0; i < people.length; i++){
people[i].move();
people[i].display();
}
}
function removePeopleThatHaveSlippedOutOfView(){
var peopleToKeep = [];
for (var i = 0; i < people.length; i++){
if (people[i].x + people[i].breadth > 0) {
peopleToKeep.push(people[i]);
}
}
people = peopleToKeep;
}
function addNewPeopleWithSomeRandomProbability() {
var newPeopleLikelihood = 0.005;
if (random(0,1) < newPeopleLikelihood) {
people.push(makePeople(width));
}
}
function peopleMove() {
this.x += this.speed;
}
function peopleDisplay() {
push();
translate(this.x, height - 25);
noStroke();
fill(0);
ellipse(0, -20, 40, 50); //head
rect(-35, 0, 70, 50, 80); //body
push();
rotate(radians(-7));
ellipse(25, -15, 15, 110); //arm
pop();
pop();
}
function makePeople(birthLocationX) {
var ppl = {x: birthLocationX,
breadth: round(random(5, 10)),
speed: -3.5,
pHeight: round(random(20,25)),
move: peopleMove,
display: peopleDisplay}
return ppl;
}
function displayHorizon(){
fill(53, 97, 44);
rect (0,height-50, width, height-50);
}
function displayStatusString(){
noStroke();
fill(0);
}
Category: SectionC
Looking Outwards 11: A Focus on Women Practitioners
Emily Gobeille is a designer who specializes in merging technology and design to create an immersive design experience. She spent her years studying many disciplines, including web, prints, motion graphics, games, and installations. She also is one of the founders of Designer I/O, which specializes in the design and development of interactive installations. The company’s clients and partners usually include children museums.
I decided to look at one of her Designer I/O projects called Mimic. It is an “interactive installation that allows visitors to engage in a dialogue with a robot arm through gesture.” The robot can track the people’s movements and also has the ability to react to individuals actions accordingly. The robot can break down its impressions into three feelings: trust, curiosity, and interest, which will affect the robot’s responses. Depending on the individual’s movement and emotions, Mimic will react to many people simultaneously, changing its behavior. I personally find this interactive robot interesting for its skill to acknowledge the viewer’s reactions.
LO: Stephanie Dinkins
I first found Stephanie Dinkins while doing research for one of our previous Looking Outwards assignments and found that her work was very inspirational. As a Black woman transmedia (computational) artist, Dinkins explores the intersection between storytelling, treasured history, and the amplification of voices through her art. Her work often circles around topics of gender (in both culture-specific and broader societal contexts), being a Black person in America, and equity for communities of color.
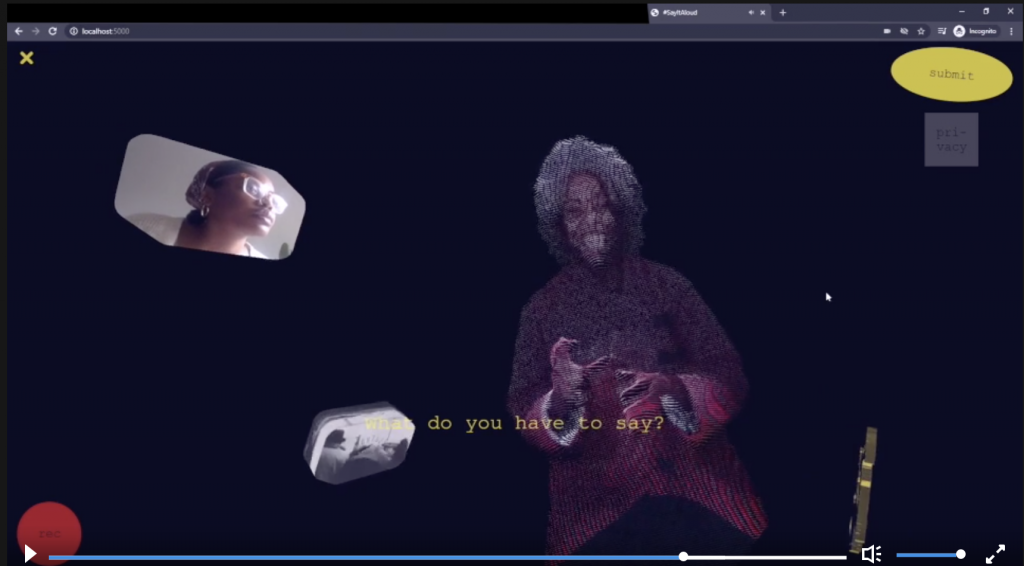
One of her most recent works, #SayItAloud, is a WebXR experience driven by motivations of fighting injustices in the world but at the same time, creating things to solidify those impacts. The user is led through this experience by a central persona, Professor Commander Justice and the interaction is enhanced by the ability for viewers to add their own thoughts and experiences through the form of video submissions.
Project 11: Generative Landscape
For this project, I went with a simple scrolling sunset city & mountain view. The sun has a second ring around it just because I felt that it gave it more of a setting sun feeling. Then the buildings have flashing lights because I thought about how dorm/student living buildings when you look at them from the outside. Everyone seems to have different color LED lights lighting up their rooms in all different colors.
//Helen Cheng
//helenc1@andrew.cmu.edu
//Project 11
//Section C
var buildings = [];
var hill = [];
var noiseParam = 0;
var noiseStep = 0.01;
var n;
var hillPoint;
function setup() {
createCanvas(640, 240);
// create an initial collection of buildings
for (var i = 0; i < 10; i++){
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
//creates initial set of mountains
for (i=0; i<=width/5; i++) {
n = noise(noiseParam);
hillPoint = map(n, 0, 1, 0, height);
hill.push(hillPoint);
noiseParam += noiseStep;
}
frameRate(10);
}
function draw() {
background(93, 150, 168);
//setting sun
fill(245, 188, 159);
circle(width/2, height/2, 100);
fill(250, 211, 125);
circle(width/2, height/2, 50);
drawHills();
displayHorizon();
updateAndDisplayBuildings();
removeBuildings();
addNewBuildings();
}
function drawHills() {
var x = 0;
beginShape();
vertex(0, height);
stroke(0);
fill(126, 168, 151);
//draws hill shape
for (i=0; i<width/5; i++) {
vertex(5*i, hill[i]);
}
//appends new hill point and removes first
hill.shift();
vertex(width, height);
n = noise(noiseParam);
hillPoint = map(n, 0, 1, 0, height);
hill.push(hillPoint);
noiseParam += noiseStep;
endShape(CLOSE);
}
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildings(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep;
}
function addNewBuildings() {
var newBuildingLikelihood = 0.005;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
// method to update position of building every frame
function buildingMove() {
this.x += this.speed;
}
// draw the building and some windows
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
stroke(0);
fill(245, 229, 201);
push();
translate(this.x, height - 40);
rect(0, -bHeight, this.breadth, bHeight);
stroke(200);
for (var i = 0; i < this.nFloors; i++) {
fill(color(random(255), random(255), random(255)));
rect(5, -15 - (i * floorHeight), this.breadth - 10, 10);
}
pop();
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: 50,
speed: -2.0,
nFloors: round(random(2,9)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
function displayHorizon(){
stroke(0);
fill(202, 245, 201);
rect(0,height-50, width, height);
}
Project 11: A worm dreaming
I wanted to do a little animation/landscape of a worm’s dream 🙂 Flashy colors and big flowers!
var backgroundX = 600;
var trip = [];
var noiseParam = 0;
var noiseStep = 0.02;
var x1 = 250;
function preload() {
colorBackground = loadImage("https://i.imgur.com/XE9vWWB.png")
worm = loadImage("https://i.imgur.com/CYR27Ru.png");
redFlower = loadImage("https://i.imgur.com/R5vVLaL.png");
yellowFlower = loadImage("https://i.imgur.com/ogHwP78.png");
blueFlower = loadImage("https://i.imgur.com/faPKcYb.png");
backgroundHouse = loadImage("https://i.imgur.com/DgMNstF.png");
backgroundTower = loadImage("https://i.imgur.com/AuMqECF.png");
}
function setup() {
createCanvas(450, 300);
for (var i = 0; i<width/5 +1; i++) {
var n = noise(noiseParam);
var value = map(n,0,1,0,height+50);
trip.push(value);
noiseParam+=noiseStep;
}
}
function draw() {
// backdrop
image(colorBackground,width/2,height/2);
dream();
imageMode(CENTER);
image(blueFlower, x1, 105, 250, 250);
image(redFlower, x1 - 170, 137, 67, 126);
image(yellowFlower, x1 + 130, 105.5, 210, 210);
image(worm, 94, 190.5, 188, 163);
backgroundX -= 2;
x1 -= 2;
// resetting foreground and background elements
if (backgroundX <= -125) {
backgroundX = 900;
}
if (x1 <= -160) {
x1 = 825
}
}
function dream() {
trip.shift();
var n = noise(noiseParam);
var value = map(n,0,1,0,height+50);
trip.push(value);
noiseParam += noiseStep;
beginShape();
vertex(0,height);
for(var i =0; i <= width/5; i++) {
fill(random(150,200),103,random(100,200));
strokeWeight(4);
vertex((i*5),trip[i]);
}
vertex(width,height);
endShape();
}
LO11: Women Practitioners
Mimi Son – Lit Tree
Mimi Son’s installation called “Lit Tree” was a work that I found very interesting. A small potted tree is augmented with video projection, which allows different lights, patterns, and visualizations appear through the branches and leaves of the tree. It can also interact with the visitors, and the work symbolizes the relationship between humankind and nature. I found the work interesting because Son tried to recreate how humans intervene and disrupt nature.
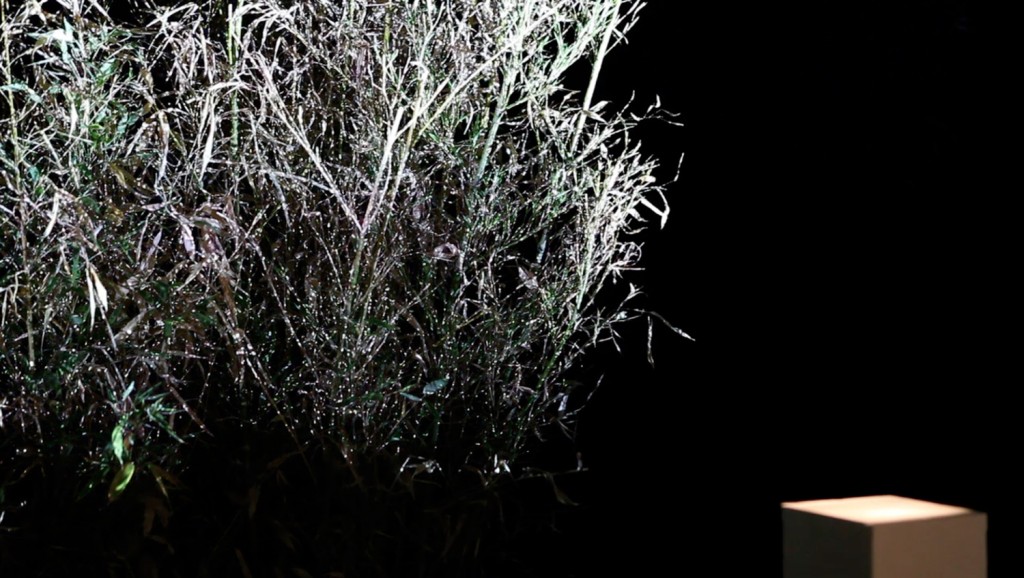
Mimi Son founded a Korean creative studio in called “Kimchi and Chips”in 2009. The goal of her studio is to connect the arts, sciences and philosophy through their research-based approach. She works in South Korea, but has created projects that are installed all over the world. She also became the first Korean artist to win the Award of Distinction at Ars Electronica, which is an award that signifies importance of work within the field of media art.
Looking Outwards – 11
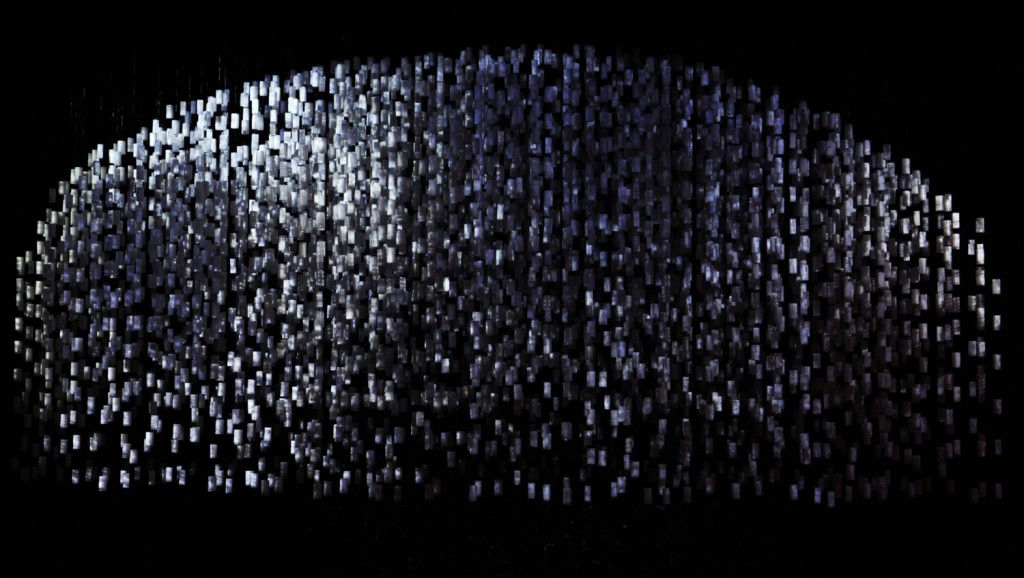
For this Looking Outwards, I decided to write about the piece Assembly and one of the women involved in its creation. This piece features 5,500 white polymer blocks hanging from the ceiling. Through projectors, each block is illuminated with digital light. What I admire about this project is the use of both physical and digital instruments. I’m especially attracted to the way that light is implemented in this piece, creating a wave like animation. Mimi Son is the co-founder of the Seoul based art studio responsible for this artwork. She studied Interaction Design in Copenhagen, Denmark and got her MA in London, England. Along with working as an art director, she also teaches Interactive Storytelling in Korea.
Project – 11 – Landscape
//Dreami Chambers; Section C; dreamic@andrew.cmu.edu; Assignment-11-Project
var stars = []
var planets = []
var moon = []
var noiseParam = 0
var noiseStep = 0.1
var sky
var astronaut
var planet
var meteor
var mx = 300
var my = 0
var mdx
var mdy
var ax = 0
var ay = 100
var ady = 1
var frameCount = 0
function preload() {
sky=loadImage("https://i.imgur.com/zfE0rbY.jpg")
astronaut=loadImage("https://i.imgur.com/0GaKn9k.png")
planet=loadImage("https://i.imgur.com/UCrpkmB.png")
meteor=loadImage("https://i.imgur.com/gcfvaqR.png")
}
function setup() {
createCanvas(400, 300)
//meteor velocity
mdx = random(-10,-15)
mdy = random(10,15)
//stars setup
for (var i = 0; i < 100; i++) {
var rx = random(width)
var ry = random(height)
var rdx = random(4)
stars[i] = makeStars(rx, ry, 2, rdx)
}
//planets setup
for (var j = 0; j < 2; j++) {
var rx = random(width)
var ry = random(150)
planets[j] = makePlanets(rx, ry)
}
//moon surface setup
for (var k = 0; k < width; k++){
var n = noise(noiseParam)
var value = map(n, 0, 1, 10, 50)
moon.push(value)
noiseParam += noiseStep
}
frameRate(10)
}
function draw() {
image(sky, 0, 0, 400, 400)
//draws stars
starUpdate()
starsRemove()
starsAdd()
//draws planets
planetUpdate()
planetsRemove()
planetsAdd()
//draws moving meteor
drawMeteor(mx, my, mdx, mdy)
//draws moon surface
drawMoon()
//draws astronaut
drawAstronaut(ax, ay)
//moves meteors across screen
mx+=mdx
my+=mdy
//moves astronaut up and down
ay+=ady
if (ay > 110){
ady*=-1
}
if (ay < 90){
ady*=-1
}
frameCount++
}
function drawAstronaut(x, y){
image(astronaut, x, y, 55, 60)
}
function drawMoon(){
stroke(200, 180, 200)
strokeWeight(200)
moon.shift()
var n = noise(noiseParam)
var value = map(n, 0, 1, 10, 50)
moon.push(value)
noiseParam += noiseStep
for (var i=0; i < width; i++){
line(i*15, 300+moon[i], (i+1)*15, 300+moon[i+1])
}
}
function drawMeteor(x, y, dx, dy){
image(meteor, x, y, 50, 45)
}
//star functions
function starUpdate(){
for (var i = 0; i < stars.length; i++){
stars[i].stepFunction()
stars[i].drawFunction()
}
}
function starsRemove(){
starsToKeep = []
for (var i = 0; i < stars.length; i++){
if (stars[i].x + stars[i].size > 0){
starsToKeep.push(stars[i])
}
}
stars = starsToKeep
}
function starsAdd(){
var starProb = 0.5
if (random(1) < starProb){
stars.push(makeStars(random(width, 410), random(200), 2, random(4)))
}
}
function starStep() {
this.x -= this.dx
}
function starDraw() {
stroke(this.c)
strokeWeight(this.size)
point(this.x, this.y)
}
function makeStars(px, py, pdx, ps) {
var s = {x: px, y: py, dx: pdx,
size: ps, c: color(random(150, 230),random(100, 140),random(200, 255)),
drawFunction: starDraw, stepFunction: starStep
}
return s
}
//planet functions
function planetUpdate(){
for (var i = 0; i < 2; i++){
planets[i].stepFunction()
planets[i].drawFunction()
}
}
function planetsRemove(){
planetsToKeep = []
for (var i = 0; i < planets.length; i++){
if (planets[i].x + planets[i].size > 0){
planetsToKeep.push(planets[i])
}
}
planets = planetsToKeep
}
function planetsAdd(){
var planetsProb = 0.3
if (random(0, 1) < planetsProb){
planets.push(makePlanets(random(width, 450), random(150)))
}
}
function planetStep() {
this.x -= this.dx
}
function planetDraw() {
image(planet, this.x, this.y, this.size, this.size)
}
function makePlanets(px, py) {
var p = {x: px, y: py, dx: 2,
size: random(10,60), c: color(random(200,200),random(140,170),random(140,170)),
drawFunction: planetDraw, stepFunction: planetStep
}
return p
}
For this project, I wanted to create a landscape capturing space. I used clip art for a few of the objects here. The meteor moves past the screen at the beginning of the animation. The objects that move with the camera are the stars, the planets, and the moon surface. The stars are created by randomly placing points with different sizes and slightly different colors. The planets have random sizes and locations as well. For the moon surface, I referred to the stock market example that we did in lab previously. I had originally planned to add craters to the moon, but decided not to for the sake of time. Here is my sketch for this project:

Project 11
To start with this project I wanted to make my landscape the outside of a plane window. I wanted to make it seem like someone was sitting in an airplane looking at mountain tops and a sunset as clouds and birds go by.
var grass=[];
var noiseParam=0;
var noiseStep=0.04;
var x=[];
var cloud={x:200,xx:200,y:0,yy:0,r:50,v:-2,
v2:-2.75};
var robin={x:100,y:25,dx:-2,dy:28,c:255,z:0,r:212,g:124,b:47,
rr:48,gg:46,bb:59,r3:105,g3:107,b3:102};
function setup() {
createCanvas(400, 400); //setting up the grass
for(var i=0; i<width/5+1; i++){
var n=noise(noiseParam);
var value=map(n,0,1,0,height-100);
grass.push(value);
noiseParam+=noiseStep;
}
frameRate(40);
}
function draw() {
var c2=color(253,94,83);
var c1=color(83,242,253);
sunset(0,0,width,height,c1,c2,"C"); //background
mountains(); //call mountains
noFill();
stroke(0);
bird(); //call bird
clouds(); //call clouds
push();
strokeWeight(0);
rect(100,100,200,200); //draw inside of plane
fill(64,64,64);
rect(0,0,width,75);
rect(0,325,width,75);
rect(0,75,100,250);
rect(300,75,100,250);
pop();
wing(); //draw wings
push();
noStroke(); //draw window gray lining
fill(186,194,202);
rect(75,50,25,300);
rect(100,50,200,25);
rect(100,325,200,25);
rect(300,50,25,300);
pop();
line(110,65,290,65); //window shade pull down
}
function sunset(x,y,w,h,c1,c2,a) {
noFill();
if (a=="C") { //color gradient of background
for(var i=y;i<=y+h;i++){
var cl=map(i,y,y+h,0,1);
var color=lerpColor(c1,c2,cl);
stroke(color);
line(x,i,x+w,i);
}
}
fill(253,94,83);
circle(200,325,150); //draw the sun
}
function wing(){ //drawing the planes wing
push();
fill(34,33,54);
beginShape();
vertex(300,250);
vertex(201,225);
vertex(191,225);
vertex(300,300);
vertex(300,250);
endShape(CLOSE);
fill(83,60,61);
beginShape();
vertex(198,225);
vertex(185,215);
vertex(180,215);
vertex(191,225)
endShape();
fill(52,34,32);
beginShape();
vertex(191,225);
vertex(189,235);
vertex(192,235);
vertex(201,225);
endShape(CLOSE);
pop();
}
function clouds(){
push();
fill(255); //cloud white
noStroke();
translate(width/2,height/2);
cloud.xx+=cloud.v2;
cloud.x+=cloud.v;
circle(cloud.x,cloud.y,cloud.r); //top cloud
circle(cloud.x+25,cloud.y,cloud.r);
circle(cloud.x+12.5,cloud.y-25,cloud.r);
circle(cloud.xx-100, cloud.yy+150,cloud.r); //bottom cloud
circle(cloud.xx-75,cloud.yy+150,cloud.r);
circle(cloud.xx-87.5,cloud.yy+125,cloud.r);
cloud.xx+=cloud.v2;
cloud.x+=cloud.v;
if(cloud.xx<-200){ //makes clouds reappear on left side of screen
cloud.xx=400;
cloud.yy=random(-100,100);
};
if(cloud.x<-200){
cloud.x=400;
cloud.y=random(-100,100);
}
pop();
}
function bird(){ //bird helper function
push();
fill(robin.r,robin.g,robin.b);
circle(robin.x,robin.y,50);
fill(robin.rr,robin.gg,robin.bb);
circle(robin.x-25,robin.y-13,25);
fill(robin.r3,robin.g3,robin.b3);
triangle(robin.x+35,robin.y+5,robin.x-5,
robin.y+5,robin.x+25,robin.y-25);
fill(robin.c,robin.c,robin.z);
triangle(robin.x-38,robin.y-8,robin.x-43,
robin.y-8,robin.x-38,robin.y-13);
robin.x+=robin.dx;
if(robin.x<-200){ //makes clouds reappear on right side of screen
robin.x=400;
robin.y=random(100,300);
};
pop();
}
function mountains(){
if(grass.length>80){ //allow the mountains to move
grass.shift();
for(var i=0;i<width/5+1;i++){
var n=noise(noiseParam);
var value=map(n,0,1,0,1.25*height);
grass.push(value);
noiseParam+=noiseStep;
push();
strokeWeight(3);
x[i]=5*i;
pop();
}
}
fill(178, 168, 255);
beginShape(); //set up the shape of the mountains
for(var i=0; i<width; i++){
vertex(i*5,grass[i]);
}
vertex(width,height);
vertex(0,height);
endShape(CLOSE);
}
Looking Outwards 11
A project that I found interesting was Text Rain by Camille Utterback. I found this interesting because the piece was permanently installed in 2000, very early for graphic art that works with human motions. I like this project because it allows people to manipulate fake words with their motions. The creator of this piece, Camille Utterback is an internationally acclaimed artist and pioneer in the field of digital and interactive art. Utterback earned her BA in Art from Williams College, and her Masters degree from The Interactive Telecommunications Program at NYU’s Tisch School of the Arts. She has many pieces in private and public collections, including The Smithsonian and Hewlett Packard.
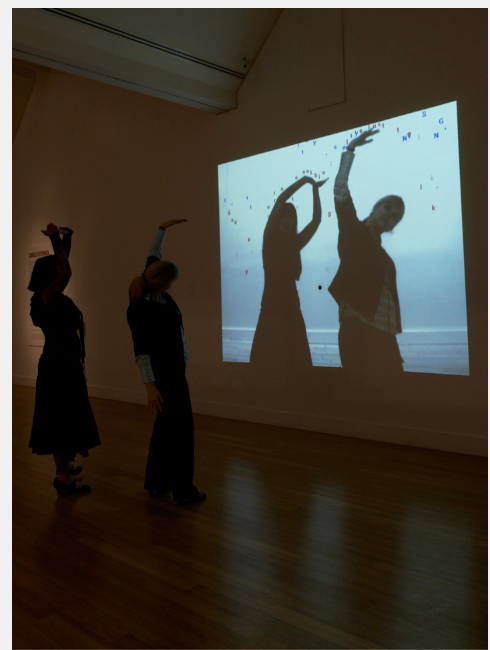