I had a rough week and was unable to complete this code 🙁 It was supposed to be a rocket traveling through space with stars generating in the background and asteroids randomly generating to travel parallel to the ship to give the impression of speed. The two static objects were the rocket and the earth. I drew them myself, trying to get a laugh. But since the code doesn’t work, it just seems overwhelmingly pathetic.
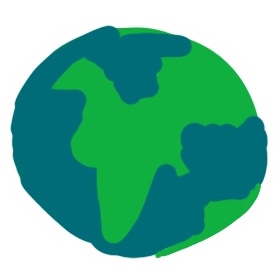
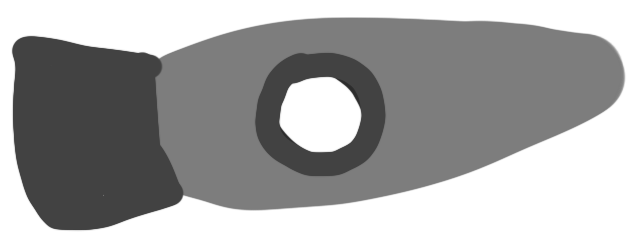
//jubbies
var stars = [];
var asteroids = [];
var rocket;
var counter = 0;
function preload() {
rocket = loadImage("https://i.imgur.com/YMS5MRv.png");
earth = loadImage("https://i.imgur.com/7N4avKI.png");
}
function setup() {
createCanvas(400, 250);
frameRate(12);
//setting up the stars - priming the array
for (var i = 0; i < 140; i++) {
var sx = random(width);
var sy = random(height);
var sWidth = random(20);
stars[i] = makeStars(rx, ry, 2, sWidth);
}
//setting up the asteroids - priming the array
for (var i = 0; i < 4; i++) {
var rx = random(width);
var ry = random(150);
asteroids[i] = makeAsteroids(rx, ry);
}
}
function draw() {
background('black');
//EARTH (BEHIND ALL)
image(earth, 270, 40, 50, 50);
//STARS
updateAndDisplayStars();
removeStarsOutOfView();
addNewStars();
//ASTEROIDS
updateAndDisplayAsteroid();
removeAsteroidsOutOfView();
addNewAsteroids();
//ROCKET
image(rocket, 30, 140, 150, 80);
counter++;
}
//STARS- ALL FUNCTIONS
function updateAndDisplayStars(){
for (var i = 0; i < stars.length; i++){
stars[i].stepFunction();
stars[i].drawFunction();
}
}
function removeStarsOutOfView(){
//dropping off old stars
var starsToKeep = [];
for (var i = 0; i < stars.length; i++){
if (stars[i].x + stars[i].width > 0) {
starsToKeep.push(stars[i]);
}
}
stars = starsToKeep;
}
function addNewStars(){
var newstars = 0.455
if (random(0, 1) < newstars) {
stars.push(makeStars(255, 255, 3, random(1, 10));
}
}
function starsStep() {
this.x -= this.dx;
}
function starsDraw() {
stroke(this.c);
strokeWeight(this.size);
point(this.x, this.y);
}
function makeStars(sx, sy, sdx, sWidth) {
var s = {x: sx, y: sy, dx: sdx,
width: sWidth,
c: fill(255),
drawFunction: starsDraw, stepFunction: starsStep
}
return s
}