//Xinyi Du
//15104 Section B
var eyes = 20;
var mouth = 20;
var face = 100;
var ears = 20;
var r // red
var g // green
var b // blue
var hairDeco = 20; //hair decorations
var hair = 30; //hair
var cloth = 200;
function setup() {
createCanvas(300, 300);
}
function draw() {
background(80);
noStroke();
// clothing
fill(255-r, 255-g, 255-b);
triangle(width / 2, height / 2, width/2-cloth/2, height/2+cloth, width/2+cloth/2, height/2+cloth);
fill(255, 229, 204);
ellipse(width / 2, height / 2, face, face); // face
fill(255, 229, 204);
ellipse(width / 2 - face / 2, height / 2, ears, ears); // left ear
fill(255, 229, 204);
ellipse(width / 2 + face / 2, height / 2, ears, ears); // right ear
fill(255, 102, 102);
ellipse(width/2, height/2+face/4, mouth,); // mouth
var eyeL = width/2 - face/4; // left eye
var eyeR = width/2 + face/4; // right eye
fill(64, 64, 64);
ellipse(eyeL, height/2, eyes, eyes+5);
ellipse(eyeR, height/2, eyes, eyes+5);
//hairstyle
fill(r, g, b);
ellipse(width/2, height/2-face/2, hairDeco, hairDeco); // hair decorations 1
fill(r, g, b);
// hair 1
var x1 = width/2-hair/2;
var y1 = height/2-face/2-hairDeco-hair;
var x2 = width/2+hair/2;
var y2 = height/2-face/2-hairDeco-hair;
var x3 = width/2;
var y3 = height/2-face/2-hairDeco/2;
triangle(x1, y1, x2, y2, x3, y3);
fill(r-30, g-30, b-30);
ellipse(width/2-face/4, height/2-face/3, hairDeco, hairDeco); // hair decorations 2
ellipse(width/2+face/4, height/2-face/3, hairDeco, hairDeco); // hair decorations 3
// hair 2
var x1 = width/2-face/4;
var y1 = height/2-face/3-hairDeco/2;
var x2 = width/2-face/4-hair/2;
var y2 = height/2-face/3-hairDeco/2-hair;
var x3 = width/2-face/4+hair/2;
var y3 = height/2-face/3-hairDeco/2-hair;
triangle(x1, y1, x2, y2, x3, y3);
// hair 3
var x1 = width/2+face/4;
var y1 = height/2-face/3-hairDeco/2;
var x2 = width/2+face/4-hair/2;
var y2 = height/2-face/3-hairDeco/2-hair;
var x3 = width/2+face/4+hair/2;
var y3 = height/2-face/3-hairDeco/2-hair;
triangle(x1, y1, x2, y2, x3, y3);
}
function mousePressed() {
face = random(80, 150);
eyes = random(10, 20);
ears = random(12, 30);
mouth = random(10, 25);
hairDeco = random(10, 30);
hair = random(20, 50);
r = random(10, 250);
g = random(10, 250);
b = random(10, 250);
cloth = random(150, 300);
//
}
Month: September 2022
Looking Outwards – Holger Lippmann
The art series I was most intrigued by was noiseScape IV by Holger Lippmann (2021).
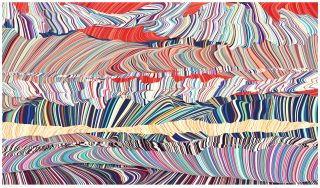
link to website: http://www.lumicon.de/wp/?p=3914
This series of generative art is simple in the way its structured visually, but its still very interesting. The first thing that sticks out to me is the fact that each piece is stratified into horizontal layers. In each layer are squiggly vertical lines. The way the vertical lines are drawn reminds me of a cascading hilly landscape.
In some of the pieces I notice that some areas of the canvas are left blank which create cloud like shapes that float around on the canvas.
I’m not sure how it would have been coded but I’d guess that
the artist maybe defined the boundaries of each layer and the
computer “semi randomly” drew lines between those boundaries. The reason why I say “semi randomly” is because there seems to be a logic behind how each lines are drawn after all each line somewhat follows the path of the adjacent lines. The artist must’ve also defined the colors as the colors of the lines are harmonious.
Project – 02
sketch
//Leo Deng Section A
var eyeWidth = 20;
var eyeHeight = 20;
var faceWidth = 140;
var faceHeight = 160;
var LeyebrowX = 115;
var LeyebrowY = 125;
var ReyebrowXx = 185;
var ReyebrowYy = 125;
var mouthX = (300 / 2);
var mouthY = (300/2 + 40);
var mouthW = 30;
var mouthH = 10;
var mouthStart = 90;
var mouthEnd = 195;
var on = -1;
var R = 255;
var G = 215;
var B = 0;
function setup() {
createCanvas(300, 300);
}
function draw() {
background(233,150,122);
strokeWeight(3);
stroke(175,238,238);
if (on == -1) { //color flip from yellow to orange
fill(R, G, B);
} else if (on == 1) {
fill(R, G - 100, 0);
}
triangle(0, 0, 125, 145, 145, 100); //pointy design elements
triangle(150, 0, 100, 145, 200, 145);
triangle(299, 0, 185, 145, 100, 145);
triangle(299, 150, 165, 100, 165, 195);
triangle(0, 150, 135, 100, 135, 195);
triangle(0, 299, 125, 155, 145, 200);
triangle(150, 299, 100, 155, 200, 155);
triangle(299, 299, 185, 155, 100, 155);
ellipse(width / 2, height / 2, faceWidth, faceHeight);
var eyeLX = width / 2 - faceWidth * 0.25; //local eye variables
var eyeRX = width / 2 + faceWidth * 0.25;
ellipse(eyeLX, height / 2, eyeWidth, eyeHeight);
ellipse(eyeRX, height / 2, eyeWidth, eyeHeight);
line(LeyebrowX, LeyebrowY, 135, 125); //eyebrows
line(165, 125, ReyebrowXx, ReyebrowYy);
ellipse(eyeLX, height / 2, eyeWidth * (2 / 3), eyeHeight); //eyes
ellipse(eyeRX, height / 2, eyeWidth * (2 / 3), eyeHeight);
arc(mouthX, mouthY, mouthW, mouthH, mouthStart, mouthEnd, PIE); //mouth
}
function mousePressed() {
on = on * -1;
// when the user clicks, these variables are reassigned to make the face look angry
faceWidth = (faceWidth + 50);
eyeWidth = (eyeWidth + 10);
LeyebrowX = (LeyebrowX - 10);
LeyebrowY = (LeyebrowY - 10);
ReyebrowXx = (ReyebrowXx + 10);
ReyebrowYy = (ReyebrowYy - 10);
mouthX = (mouthX - 10);
mouthH = (mouthH + 10);
if(faceWidth > 190) {
// next 3 clicks changes from mischevious face to unsure face
faceWidth = (faceWidth - 60);
eyeWidth = (eyeWidth - 10);
LeyebrowX = (LeyebrowX + 15);
LeyebrowY = (LeyebrowY + 15);
ReyebrowXx = (ReyebrowXx - 15);
ReyebrowYy = (ReyebrowYy + 15);
mouthX = (mouthX + 5);
mouthW = (mouthW + 10);
mouthH = (mouthH - 10);
mouthStart = 0;
mouthEnd = PI + QUARTER_PI;
}
if(faceWidth < 160) {
//return to original face
eyeWidth = 20;
eyeHeight = 20;
faceWidth = 140;
faceHeight = 160;
LeyebrowX = 115;
LeyebrowY = 125;
ReyebrowXx = 185;
ReyebrowYy = 125;
mouthX = (300 / 2);
mouthY = (300/2 + 40);
mouthW = 30;
mouthH = 10;
mouthStart = 90;
mouthEnd = 195;
on = -1;
R = 255;
G = 215;
B = 0;
}
}
Project-03-Dynamic-Drawing
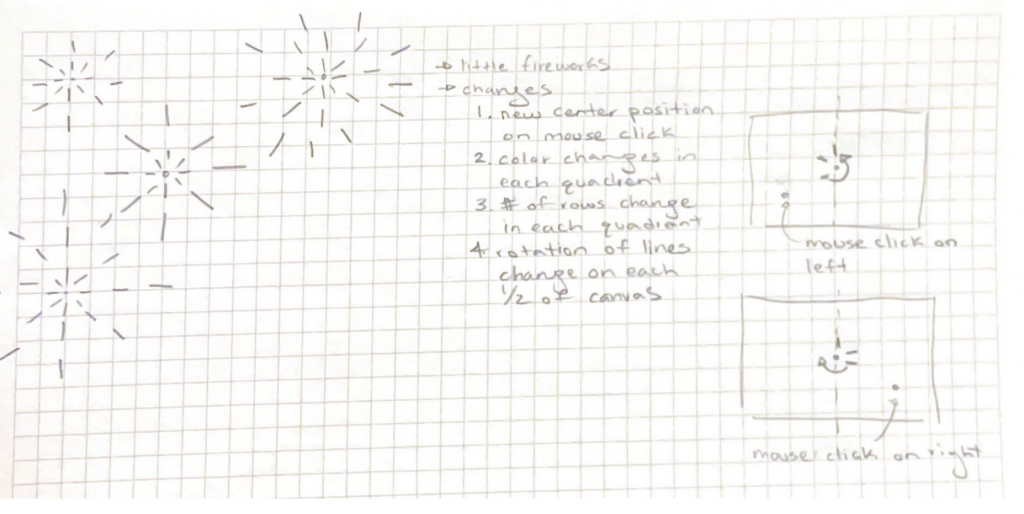
I began this project by writing down my main actions for the animation and what conditions caused them to change. After I got one part working, I slowly added more conditions and commands, ensuring they worked before continuing.
The animation starts with a simple circle of lines. The user can click on the screen to make the second row of lines appear. If the user clicks on the left half then the direction that the lines are drawn changes. Depending on the quadrant the user clicks in the number of rows and color will change.
// Emily Franco
// efranco
// Section C
//line distance from center
var fromCenter = 5;
//x and y positions of pts for lines
var ptX = 0;
var ptY = 0;
//default line length
var length = 5;
//current rotation degree
var deg = 0;
var rot = 5;
var dir = 1;
var degTwo = 0;
//tracks how many times mouse is clicked
var clicked = 0;
//tracks number of circle sets
var cirSet = 0;
//stores mouseX and mouseY when clicked
var mX =0;
var mY = 0;
//store previous tranlation to subtract from when new center is clicked
var transX;
var transY;
//num of rows around circle
var rows = 6;
//colors
var r = 255;
var g = 255;
var b = 0;
//color increment
var colorInt = 20;
function setup() {
createCanvas(600, 450);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
frameRate (70);
background ("black");
}
function draw() {
//change origin to center of canvas
transX = width/2;
transY = height/2;
translate (transX,transY);
//if mouse is clicke five times now center is
//at mouse position
if (cirSet==1){
translate (mX-transX,mY-transY);
transX = mX-transX;
transY = mY-transY;
}
//only reset at start of each set
if(clicked == rows){
//reset values
fromCenter = 5;
length = 5;
clicked = 0;
}
if (deg <= 360){
//rotate lines
rotate (radians(deg));
deg += rot*dir;
//if mouse is in left half turn clockwise
//if mouse is in right hald turn counterclockwise
//draw lines towards center of canvas
stroke (g,r,b);
line (ptX+fromCenter,ptY,ptX+fromCenter+length,ptY);
}
//line "chases white lines" and draws over them
push();
stroke (b,g,r);
rotate (radians(degTwo));
degTwo += rot*dir;
line (ptX+fromCenter,ptY,ptX+fromCenter+length,ptY);
//rect (ptX-1,ptY-length-fromCenter-1,2,length+2);
pop();
}
function mousePressed (){
//increase distance of lines from center
fromCenter += length + 10;
//increase length of lines
length+=10;
//if mouse clicked left of center draw counter clockwise
if (mouseX<=width/2){
dir = -1;
}else if (mouseX>=width/2){
dir = 1;
}
//if circle is complete set degree back to zero
//this will let next row start where previous row ended for only white lines
if (Math.abs(deg)>=360){
deg = 0;
}
//add one to clicked each time mouse pressed
clicked += 1;
//store x and y position for new center
if (clicked==rows){
cirSet = 1;
mX = mouseX;
mY = mouseY;
}
//number of rows per circle changed on first click of each set
if (clicked==1){
//number of rows per circle set changes in depending
//on the quadrant clicked in
if (mouseX<=width/2 & mouseY<=height/2){
rows = 3;
//blue
r = 255;
g = 0;
b = 0;
}if (mouseX>=width/2 & mouseY<=height/2){
rows = 4;
//yellow
r = 255;
g = 255;
b = 0;
}if (mouseX>=width/2 & mouseY>=height/2){
rows = 2;
//green
r = 128;
g = 255;
b = 0;
}if (mouseX<=width/2 & mouseY>=height/2){
rows = 6;
//orange
r = 255;
g = 128;
b = 0;
}
}
}
Project 2 – Variable Face
Inspired by the popular Sumikk Gurashi characters from Japanese company San-X, this project explores the variable faces of cute 2D characters in the context of a simple text-based meme format. The occurrence of each feature (ie. body color, lack/ type of ear, mouth, whiskers, arm position, text) are determined by their own unique variables, allowing for a wide scope of character generation.
// Tsz Wing Clover Chau
// Section E
function setup() {
createCanvas(640, 480);
background(220);
}
var counter = 0;
var ears = false;
var earsUp = false;
var r = 0;
var rArms = 0;
var rEars = 0;
var rMouth = 0;
var rWhiskers = 0;
var rText = 0;
let c = [255, 255, 255];
let t = ["Is for me?", "A positive weed", "Am i even\na penguin?", "Made of 1% meat, \n99% fat"];
function mouseClicked(){
r = random([0,1,2,3]);
rEars = random([0,1,2,3]);
rArms = random([0,1,2,3]);
rMouth = random([0,1,2,3]);
rWhiskers = random([0,1,2,3]);
rText = random([0,1,2,3]);
print("%s", r);
counter += 1;
if (counter > 3){ // wrapping bounds
counter = 0;
}
if (r%2 == 0){
ears = true;
} else {
ears = false;
}
if (r == 2) {
whiskers = true;
} else {
whiskers = false;
}
}
function draw() {
background(220);
scale(1.45 *0.93);
ellipseMode(CENTER);
rectMode(CENTER);
var cX = width/2;
var cY = height/2;
var sizeX = width/3;
var sizeY = width/3.75;
if (r == 0){
c = [255, 255, 255]; // shirokuma
} else if (r == 1){
c = [202, 240, 248]; // tokage
} else if (r == 2){
c = [254, 250, 224]; // neko
} else {
c = [217, 224, 163]; // penguin
}
var offsetX = width/35;
var offsetY = height/10;
//BODY
fill(c);
rect(cX + offsetX, cY + (offsetY*2), sizeX - offsetX, height - (cY + offsetY), 70);
ellipse(cX, cY, sizeX, sizeY);
noStroke();
rect(cX + offsetX, cY + (offsetY*2)-2, sizeX - offsetX-2, height - (cY + offsetY)-5, 70);
stroke(0);
strokeWeight(3);
beginShape();
curveVertex(cX - sizeX/1.75, cY - sizeY*0.9); //1st control point
curveVertex(cX + sizeX/2.5, cY - offsetY*1.07);
curveVertex(cX + sizeX/1.5, cY + (offsetY*2));
curveVertex(cX + sizeX/3.75, height - offsetY*1.02);
curveVertex(cX - sizeX/1.75, cY + sizeY*0.7); //2nd control point
endShape();
//ARMS
if (rArms == 0){
arc(cX, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3 * PI / 2, OPEN);
arc(cX - offsetY, cY + offsetY*1.5, offsetY, offsetX*1.2, 3*PI / 2, PI / 2, OPEN);
} else if (rArms %3 == 0){
arc(cX - offsetY, cY + offsetY*1.5, offsetY, offsetX*1.2, 3*PI / 2, PI / 2, OPEN);
} else if (rArms == 1){
arc(cX, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3 * PI / 2, OPEN);
} else {
arc(cX, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3*PI / 2, OPEN);
arc(cX - offsetX*4.25, cY + offsetY*1.5, offsetY, offsetX*1.2, PI / 2, 3*PI / 2, OPEN);
}
//EYES
push();
fill(0,0,0);
ellipse(cX - offsetY*1.2, cY - offsetY/6, offsetX/2, offsetX/3);
ellipse(cX + offsetY*0.2, cY - offsetY/6, offsetX/2, offsetX/3);
pop();
//NOSE / MOUTH
push();
if (rMouth %3 == 0){ // beak
fill(255, 220, 116);
ellipse(cX - offsetY/2, cY+offsetX - offsetY/6, offsetY/1.5, offsetX);
line(cX - offsetY/1.25, cY + offsetY/5, cX - offsetY/5, cY + offsetY/5);
} else if (rMouth %2 == 0) { // snout
fill(255);
strokeWeight(2);
rect(cX - offsetY/2, cY+offsetX*1.15, offsetY*0.85, offsetX*1.4, 120);
fill(0);
ellipse(cX - offsetY/2, cY+offsetX - offsetY/6, offsetY/3, offsetX/1.75);
} else {
fill(0);
ellipse(cX - offsetY/2, cY+offsetX - offsetY/6, offsetY/3, offsetX/1.75);
}
pop();
//EARS
fill(c);
if (rEars %2 == 0){ // bear
push();
rotate(radians(15));
arc(cX + offsetY*1.8, cY - sizeY, offsetY*1.1, offsetY, -PI*0.95, PI*0.05, OPEN);
fill(252, 213, 206);
ellipse(cX + offsetY*1.8, cY - sizeY*1.02, offsetY*0.45, offsetY/4);
pop();
push();
rotate(radians(-20));
arc(cX - sizeX/1.5, cY + offsetX/1.75, offsetY*1.1, offsetY, -PI*1.1, -PI*0.03, OPEN);
fill(252, 213, 206);
ellipse(cX - sizeX/1.5, cY + offsetX/2.2, offsetY*0.45, offsetY/4);
pop();
} else if (rEars%3 == 0){ // cat
push();
rotate(radians(-20));
arc(cX - sizeX*0.85, cY + offsetX*1.5, offsetY*1.35, offsetY*1.15, -PI*0.7, -PI*0.1, PIE);
noStroke();
arc(cX - sizeX*0.837, cY + offsetX*1.6, offsetY*1.25, offsetY*1.05, -PI*0.7, -PI*0.1, PIE);
stroke(0);
arc(cX - sizeX*0.25, cY + offsetX*3.85, offsetY*1.35, offsetY*1.15, -PI*0.7, -PI*0.1, PIE);
noStroke();
arc(cX - sizeX*0.235, cY + offsetX*3.95, offsetY*1.25, offsetY*1.05, -PI*0.7, -PI*0.1, PIE);
pop();
}
//WHISKERS
if (rWhiskers %3 == 0 ){
line(cX - offsetY*2.4, cY, cX - offsetX*5.5, cY + offsetY*0.05);
line(cX - offsetY*2.4, cY + offsetX/2, cX - offsetX*5.5, cY + offsetX/2 - offsetY*0.05);
line(cX + offsetY, cY, cX + offsetX*2, cY + offsetY*0.05);
line(cX + offsetY, cY + offsetX/2, cX + offsetX*2, cY + offsetX/2 - offsetY*0.05);
}
push();
textSize(18);
noStroke();
fill(255,255, 255);
text(t[rText], width/14, height/2);
pop();
}
Looking Outwards 02: Generative Art
Transiterate
Holger Lippman
2022
Spring Storm
Holger Lippman
2020-2021
The projects I have chosen are Holger Lippmann’s “Transiterate” (2022) and “Spring Storm” (2020-2021). “Transiterate” is an animated looping NFT consisting of 100 individual unique frames. Lippmann’s site describes it as “a gradual, repeating subdivision (iteration) of rhythmically consecutive color tables”, which translates to a large circle with constantly shifting and transforming subdivisions whose coloring move up and down the color wheel.
Meanwhile, “Spring Storm” is a series of prints composed of red and white swirls on a wavy background. The compositions were generated via layered Perlin noise, resulting in a highly dynamic yet varied series of artworks. The integration of code and other digital means as a core part of a lucrative artistic workflow is highly admirable.
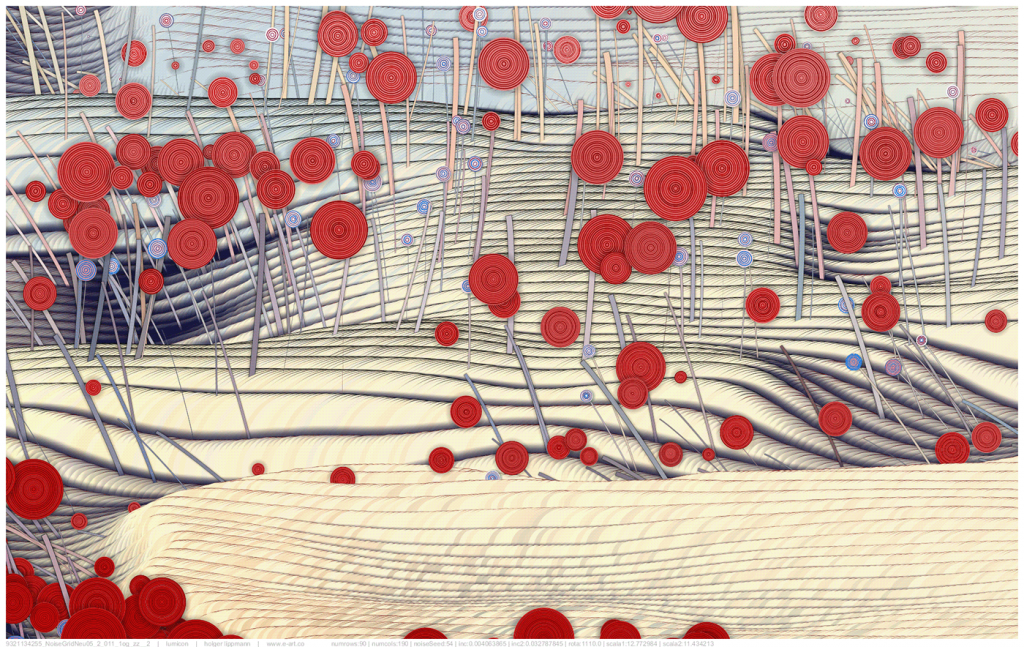
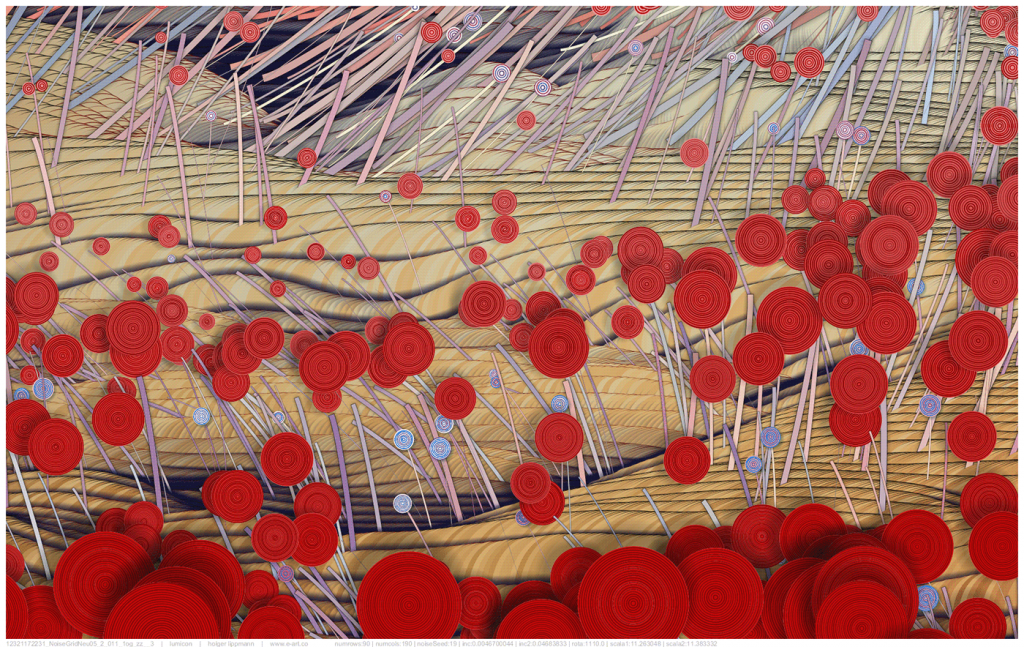
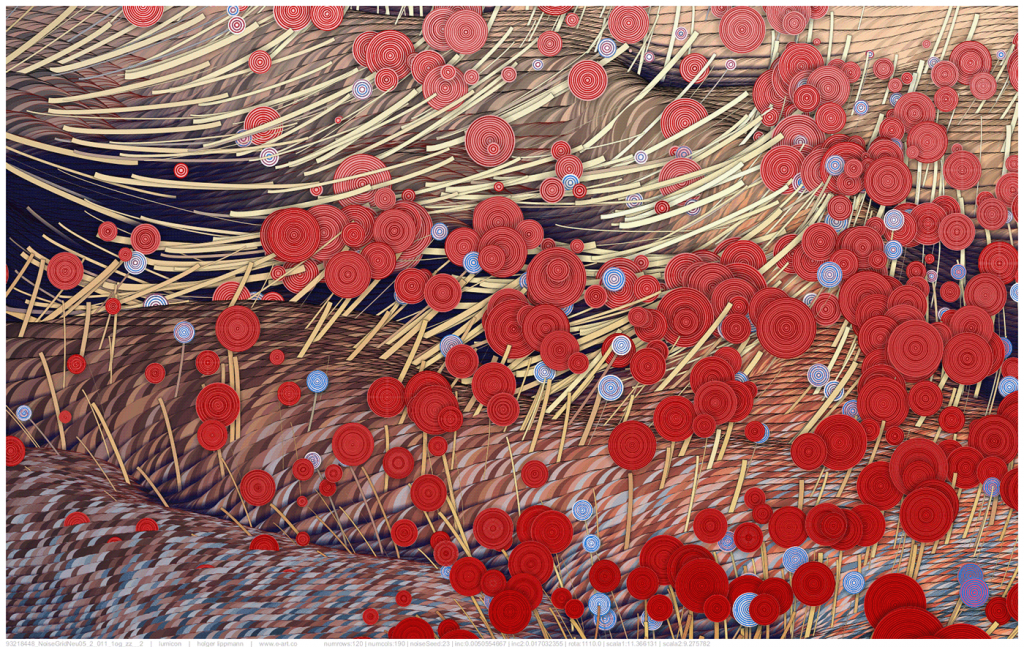
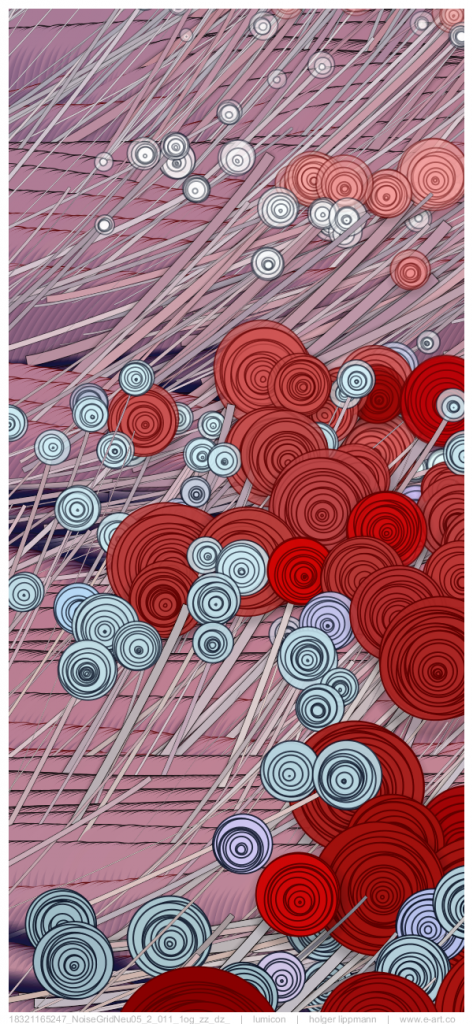
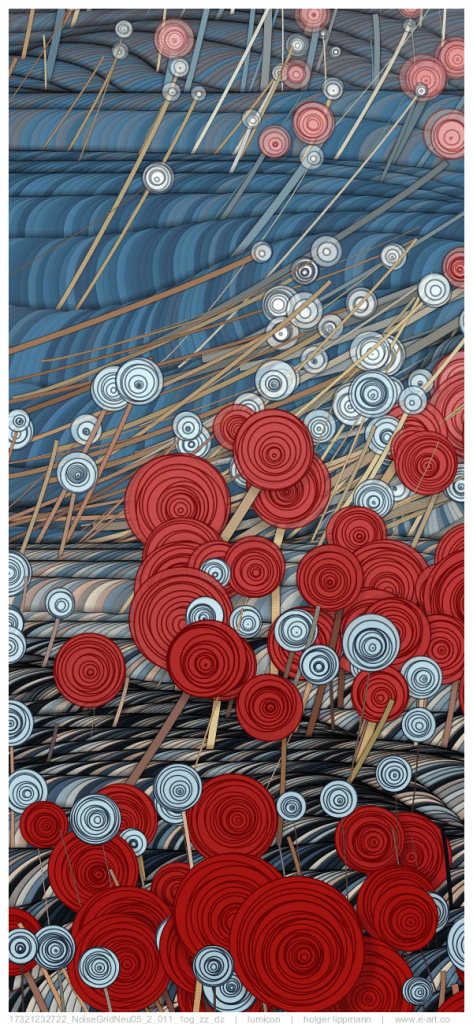
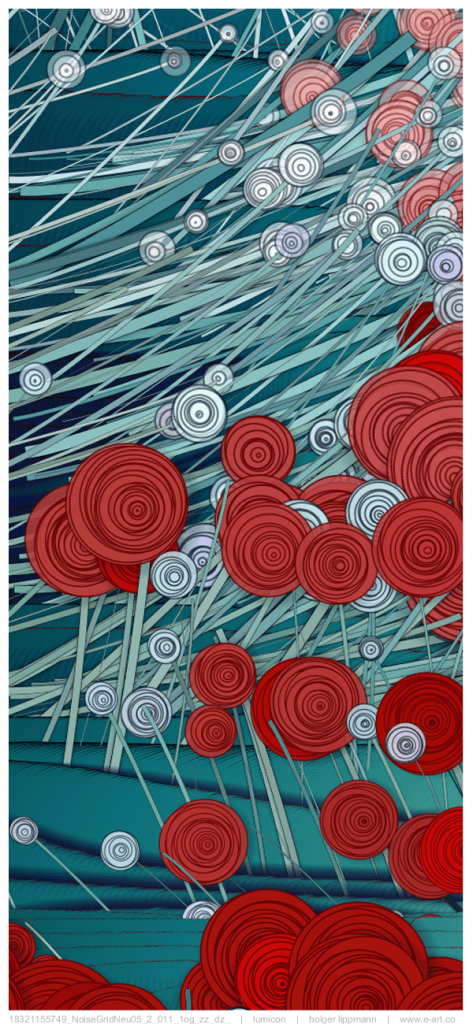
Lippmann’s work is described as falling “somewhere in between artistic disassociation and realistic reproduction, between old values and modern perspectives”. His abstract compositions are reminiscent of the atmosphere and sensorial experience of various natural phenomena. He incorporates various algorithms, particularly RNG, which allows him to instantly generate “an abundance of possibilities”, which he feels is missing in traditional forms of media.
Links:
https://holgerlippmann.io/
https://www.lumas.com/artist/holger_lippmann/
http://e-art.co/
Looking Outwards-02
I admire the Flow Fields, reinterpretation of Vincent van Gogh’s most famous works, by LIA in 2018. I like how it takes such a famous artwork, my favorite is the Sunflower reinvention, and puts a unique spin on it because of the artist’s sensibilities. In the case of the sunflowers, it is how the lines move and flow as if they are blossoming. The blossoming movement is the artist’s variation on the original Sunflower’s lines but through a generative art technique called vector fields or flow fields. This technique reveals lines and loops that happen in magnetic and energy fields. There are endless variations to the ways the lines can move. The artist also uses Van Gogh’s color palette as a base to try to bring the viewer closer to the original. I also admire how different sections of the work can go through different motions at the same time. The timing of this and how each movement goes together with the others to form the larger picture is part of how the artist comes through in the work. However, I think there was still more room for the artist to add in their own personality. Perhaps by changing up the variations of the lines.
LIA | Flow Field #2 – Reinterpreting Sunflowers by Vincent Van Gogh | Sedition (seditionart.com)
LO 2: Casey Reas
I found this piece of generative art by Casey Reas, Untitled 5 to be rather inspirational. On a lesser point, the
presentation of this work is fantastic. It goes to show that the art itself is everything: the context in which you
view it (the appearance of the website is rather bare and calls back to older internet aesthetics), the title
(or in this case, the lack of one is unsettling), and the music that is shown with the work of art (the music is similarly
unpredictable).
I suppose that this work was generated by finding an average of domestic images and messing with the generation
of the average in order to distort the images.
Given that the piece’s emphasis is meant to be unsettling, the randomization of the distortions, with their
unpredictability, further adds to the creepiness of the work, making it a wonderful case study on as to how
generative art can make a work more potent. Some may be under the impression that generative art is
interesting, but not an entirely viable form of creating art when all art could be created entirely within the
autonomy of the artist, though the unpredictability of this work is aided by its generative nature.
Reas.com Is a Database for Casey Reas, http://reas.com/untitled_5/.
Project 2: Variable Face
// Ana Furtado
// Section E
var noseSize = 20;
var r = 139;
var g = 69;
var b = 19; // r g b is going to be randomlly
//generating blues for corneas when mouse is pressed
var a = 105; // y of more hair left strand 1
var b = 105; // y of more hair left strand 2
var x = 405; //y of more hair right strand 1
var y = 405; //y of more hair right strand 2
var eyeLeft = 190;
var eyeRight = 290;
function setup() {
createCanvas(480, 640);
background(255, 210, 223); // pink
}
function draw() {
stroke(84, 18, 18); // reddish brown
strokeWeight(30);
line(175, 160, 120, 620); // back hair left
line(175, 160, 155, 620);
line(305, 160, 365, 620); // back hair right
line(305, 160, 315, 620);
stroke(0, 0, 0); // black
strokeWeight(1);
fill(0, 206, 209); // light blue
ellipse(140, 330, 30, 30); // earrings
ellipse(340, 330, 30, 30);
fill(255, 228, 196); // skin
ellipse(240, 540, 75, 400); // neck
fill(255, 228, 196); // skin
ellipse(240, 320, 200, 400); // face
fill(255, 255, 255); // white
ellipse(190, 270, 50, 100); // left eye
ellipse(290, 270, 50, 100); // right eye
fill(r, g, b); // brown
ellipse(190, 270, 25, 50); // left cornea
ellipse(290, 270, 25, 50); // right cornea
fill(0);
ellipse(eyeLeft, 270, 10, 20); // left pupil
ellipse(eyeRight, 270, 10, 20); // right pupil
fill(255, 228, 196); // skin
circle(240, 320, noseSize, noseSize); // nose
//line(190, 200, 190, 125);
//line(210, 200, 210, 125);
fill(220, 20, 60); // red
ellipse(240, 420, 110, 50) // mouth
line(185, 420, 295, 420);
stroke(84, 18, 18); // reddish brown
strokeWeight(1);
fill(84, 18, 18); // reddish brown
ellipse(240, 160, 150, 80); // hair top
strokeWeight(10);
line(160, 160, 90, 620); // hair left
line(160, 160, 115, 620);
line(160, 160, a, 620);
line(160, 160, b, 620);
line(310, 160, 415, 620); // hair right
line(310, 160, 390, 620);
line(310, 160, x, 620);
line(310, 160, y, 620);
noStroke();
fill(r, g, b); // bow
ellipse(305, 150, 20);
ellipse(325, 150, 20);
}
function mousePressed() {
// when the user clicks, these variables are reassigned
// to random values within specified ranges. For example,
// 'noseSize' gets a random value between 10 and 30.
// eye color changes as bow color changes to match
// more left and right hair
// left and right pupils move
noseSize = random(10, 30);
r = random(0, 100);
g = random(0, 250);
b = random(200, 250);
a = random(0, 175);
b = random(0, 175);
x = random(305,620);
y = random(305,620);
eyeLeft = random(177.5, 202.5);
eyeRight = random(277.5, 302.5);
}
I started with the same proportions as the face for project 1. I modified it by changing the eye color, hair, and adding a bow. The pupils also move at the press of the mouse. The most challenging part was to get the pupils to move and understanding the difference between mouseIsPressed and mousePressed in practice.
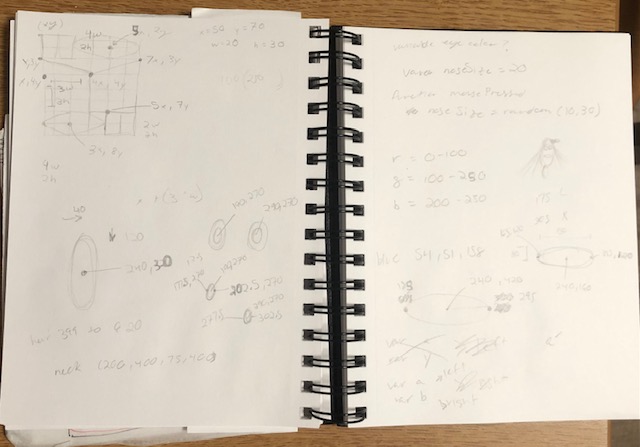