// Kyli Hilaire - Project 05
// khilaire@andrew.cmu.edu
// Section B
var x = 0;
var y = 0;
var beeWidth = 30;
var beeLength = 38;
var stripeY = 0;
function setup() {
createCanvas(400, 300);
background(247, 183, 191);
}
function draw() {
bgStripes();
// draw background stripes
push();
translate(-45, -18);
// move bees in the canvas
for(x = 0; x <= 500; x += 75) {
for(y = 0; y <= 400; y += 75) {
bee();
}
} pop();
// draw bees
for(x = 0; x <= 500; x += 75){
for(y = 0; y <= 400; y += 75){
greenCircles();
}
}
// draw green circles
}
function bee() {
beeWings();
beeBody();
}
function beeBody() {
stroke(245, 242, 158);
strokeWeight(1);
fill(255, 255, 188);
ellipse(x + 50, y + 50, beeWidth, beeLength);
// yellow oval
stroke(80);
strokeWeight(4);
line(x + 39, y + 42, x + 61, y + 42);
line(x + 38, y + 52, x + 62, y + 52);
line(x + 41, y + 62, x + 59, y + 62);
// draw bee stripes
noStroke();
fill(95)
triangle(x + 44, y + 68, x + 56, y + 68, x + 50, y + 74);
// stinger
}
function beeWings() {
noStroke();
fill(255, 255, 240);
arc(x + 50, y + 50, 65, 50, 11*PI/6, PI/6);
arc(x + 50, y + 50, 65, 50, 5*PI/6, 7*PI/6);
// bee wing arcs
stroke(0);
strokeWeight(1);
line(x + 50, y + 50, x + 75, y + 50);
line(x + 25, y + 50, x + 50, y + 50);
}
function bgStripes() {
for(let i = 0; i <= 300; i += 10) {
stroke(255, 251, 236);
strokeWeight(0.75);
line(0, stripeY + 2, 400, stripeY + 2);
stripeY += 16
// space lines 16px apart
}
}
function greenCircles() {
noStroke();
fill(216, 238, 219);
circle(x + 14, y + 61, 5);
circle(x + 20, y + 54, 8);
circle(x + 22, y + 63, 3);
// bottom right of bee
circle(x + 60, y + 10, 5);
circle(x + 65, y + 7, 3);
// upper left of bee
stroke(216, 238, 219);
strokeWeight(2);
point(x + 61, y + 3);
// small point upper left
}
Category: SectionB
3D Art / Filip Hondas
I find Filip Hodas’ ability to take characters and images we’re so familiar with out of the worlds to which they belong super fascinating. The Super Mario mushroom and the Gameboy building are some of my favorite of his works.
As someone who struggles with realism using paint/graphite, I’m particularly impressed by his ability to create things that are very much in the world but still have this surreal glow about them. I can’t imagine trying to make work like this at all, let alone on a computer.
I struggled to find a comprehensive online catalog of his work, but this article provides a decent overview of his style.
https://www.thecoolector.com/filip-hodas-artwork/
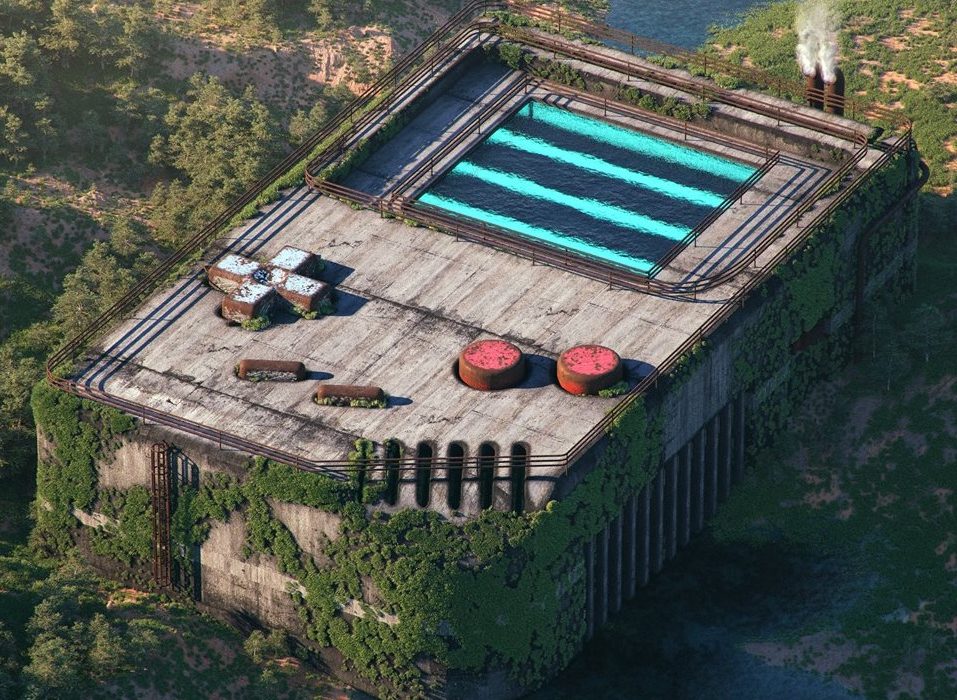
Project 5: Wallpaper, Section B
Flower of Life Wallpaper
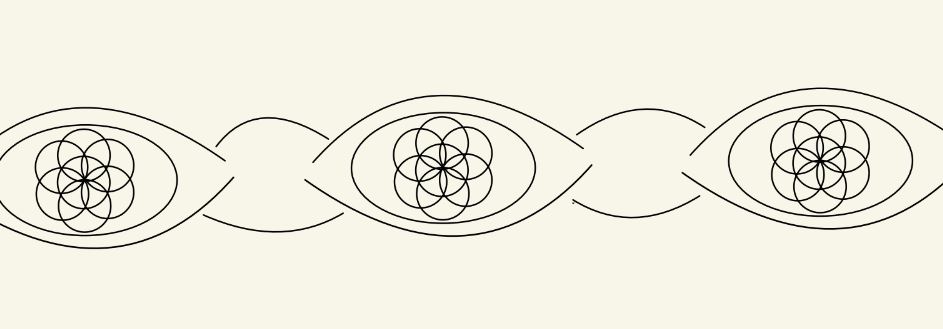
/* Evan Stuhlfire
** estuhlfi@andrew.cmu.edu, section B
** Project 05: Wallpaper */
var dot = 5;
var bigDot = 10;
function setup() {
createCanvas(600, 400);
background(224, 223, 214);
rectMode(CENTER);
}
function draw() {
var rowHeight = 150;
var colCount = 1;
var circleDiam = 50;
var circleOffset = 10;
var cosAmplitude = 40; // height of cos wave
var sinAmplitude = 60; // height of sin wave
var sinInc = 5; // increase for sin offset
// Iterate over row to create rows of sin and cos waves
for(var row = 0; row < height + rowHeight; row += rowHeight) {
var colCounter = 0; // reset column counter for new row
// iterate over theta to draw cos waves in background
for(var theta = 0; theta < width; theta++) {
stroke(49, 54, 56, 45);
circle(theta, row + cosAmplitude * cos(radians(theta)), .2);
circle(theta, row + cosAmplitude * -cos(radians(theta)), .2);
}
// iterate over theta to draw sin waves
for(var theta = 0; theta < width + circleDiam; theta++) {
stroke(49, 54, 56, 70);
fill(49, 54, 56, 70);
// draw sin waves as circles as theta moves across canvas
// increment the sinAmplitude to create layered affect
// decrease diam of circles to decrease line weight
circle(theta, row + sinAmplitude * sin(radians(theta)), 2);
circle(theta, row + (sinAmplitude + sinInc) *
sin(radians(theta)), 1);
circle(theta, row + (sinAmplitude + 2 * sinInc) *
sin(radians(theta)), .2);
circle(theta, row + sinAmplitude * -sin(radians(theta)), 2);
circle(theta, row + (sinAmplitude + sinInc) *
-sin(radians(theta)), 1);
circle(theta, row + (sinAmplitude + 2 * sinInc) *
-sin(radians(theta)), .2);
// every 90 degrees draw a design
if(theta % 90 == 0) {
colCounter++;
// when inside the sin waves draw circle of life
if(colCounter % 2 == 0) {
stroke(49, 54, 56, 175);
fill(224, 223, 214);
drawLife(theta, row);
} else {
// draw ellipses at intersection of sin waves
stroke(49, 54, 56, 50);
fill(224, 223, 214);
// draws concentric ellipses
for(var i = circleDiam; i >= 15; i -= 15){
ellipse(theta, row, i + circleOffset, i);
}
fill(49, 54, 56, 140);
ellipse(theta, row, bigDot); // draw dots at intersections
// draw little flowers
drawLittleFlowers(theta, row);
}
}
}
}
noLoop();
}
function drawLife(theta, row) {
// draw the circle of life
// set variable for circle dimensions and offset angles
var bigDiam = 110;
var diam = 30; // set smaller circle diameter
var ellipseOffset = 20;
var rad = diam/2;
var angle1 = 30;
var angle2 = 5 * angle1;
var angle3 = 45;
var oneEighty = 180;
var angleOffset = 2;
var diamOffset = 5;
push(); // save settings
translate(theta, row); // reposition origin
stroke(49, 54, 56, 90); // lighten inner circles
// draw larger ellipse
ellipse(0, 0, bigDiam, bigDiam - ellipseOffset);
// draw center circle
circle(0, 0, diam);
noFill();
// draw layers of circles with drawMoreCircles with
// distance from center, diameter, and angle of offset
// inner layer of circles
drawMoreCircles(rad, diam, oneEighty/2);
drawMoreCircles(rad, diam, angle1);
drawMoreCircles(rad, diam, angle2);
// second layer of circles
drawMoreCircles(diam, diam, oneEighty/2);
drawMoreCircles(diam, diam, angle1);
drawMoreCircles(diam, diam, angle2);
drawMoreCircles(diam - diamOffset, diam, oneEighty);
drawMoreCircles(diam - diamOffset, diam, 0);
drawMoreCircles(diam - diamOffset, diam, angleOffset * angle1);
drawMoreCircles(diam - diamOffset, diam, 2 * angleOffset * angle1);
// third layer of circles
// adjustments to offset diam and angle
drawMoreCircles(diam + rad - diamOffset, diam, oneEighty -
(5 * angleOffset));
drawMoreCircles(diam + rad - diamOffset, diam, -5 * angleOffset)
pop(); // retore settings
fill(120); // solid grey
// draw center flower dot
ellipse(theta, row, dot);
}
function drawMoreCircles(expand, diam, angle) {
// draw two circles at opposite angles defines by the
// distance from the center, diameter, and offset angle
circle(expand * cos(radians(angle)), expand * sin(radians(angle)), diam);
if(angle != 180 & angle != 0){
circle(expand * cos(radians(-angle)), expand *
sin(radians(-angle)), diam);
}
}
function drawLittleFlowers(theta, row) {
var rowOffset = 75;
var petalLength = 15;
var petalWidth = 4;
var petals = 6;
var angle = 30;
var angleIncrease = 60;
var diam = 30;
var accentOffset1 = 7;
var accentOffset2 = 10;
var ellipseOffset = 10;
// set color for flower petals
stroke(49, 54, 56, 100);
fill(224, 223, 214);
// draw circle to contain flower
ellipse(theta, row + rowOffset, diam + ellipseOffset, diam);
circle(theta, row + rowOffset, diam);
// draw the petals
for(var i = 0; i < petals; i++){
push(); // save settings
translate(theta, row + rowOffset);
rotate(radians(angle));
ellipse(petalLength/2, 0, petalLength, petalWidth);
// draw accent lines with offsets
line(petalLength + accentOffset1, 0, petalLength + accentOffset2, 0);
pop(); // restore settings
angle += angleIncrease;
}
// draw the center dot
fill(120); // solid grey
ellipse(theta, row + rowOffset, dot);
}
Project 5: Wallpaper
Shirley P5
//Xinyi Du
//15104 Section B
//xinyidu@andrew.cmu.edu
//Project-05
var w = 100;
var h = 100;
function setup() {
createCanvas(600, 600);
}
function draw() {
background(0, 58, 100);
for (var col=0; col<3; col++) {
for (var row=0; row<10; row++) {
stroke(200, 223, 82); //green
rotateEllipsee(w+3*w*col, h-100+h*row);
stroke(221,160,221); //pink
fill(221-90,160-90,221-90); //pink
circleLine2(w+3*w*col, h-100+h*row);
}
}
for (var col=0; col<3; col++) {
for (var row=0; row<10; row++) {
stroke(221,160,221); //pink
rotateEllipsee(w+100+3*w*col, 0+h*row);
stroke(200, 223, 82); //green
fill(200-90, 223-90, 82-90); //green
circleLine2(w+100+3*w*col, 0+h*row);
}
}
for (var col=0; col<3; col++) {
for (var row=0; row<10; row++) {
stroke(200, 223, 82); //green
circless(-20+3*w*col, h/2+h*row);
stroke(221,160,221); //pink
circless(20+3*w*col, -50+h/2+h*row);
}
}
}
//draw the small circles and lines
function circleLine(x, y) {
strokeWeight(1.5);
push();
translate(x, y);
line(60/2-5, 0, 60/2+20*sqrt(2), 0);
ellipse(60/2+20*sqrt(2)-10/2, 0, 10, 10);
rotate(radians(25)); //rotate to get the second pair of ellipse and line 25 degrees above
line(60/2-5, 0, 60/2+20*sqrt(2)-5, 0);
ellipse(60/2+20*sqrt(2)-10/2-5, 0, 10, 10);
rotate(radians(-50)); //rotate to get the third pair of ellipse and line 25 degrees below
line(60/2-5, 0, 60/2+20*sqrt(2)-5, 0);
ellipse(60/2+20*sqrt(2)-10/2-5, 0, 10, 10);
pop();
}
//draw the ellipses center on (x,y)
function ellipsee(x, y) {
strokeWeight(1.5);
//mirror the small circles and lines though rotating them 18 degrees
circleLine(x, y);
push();
translate(x, y);
rotate(radians(180));
circleLine(0, 0);
pop();
//white ellipses
fill(0, 58, 100);
ellipse(x, y, 60, 39);
ellipse(x, y, 40, 26);
ellipse(x, y, 20, 13);
}
//roatate the ellipses drawn in the previous function
function rotateEllipsee(x, y) {
fill(0, 58, 100);
push();
translate(x, y);
rotate(radians(-45));//rotate -45 degrees
ellipsee(0, 0);
pop();
}
//draw the vertical lines and circles
function circleLine2(x, y) {
strokeWeight(2);
//fill(221,160,221);
line(x, y-w, x, y-w+50);
line(x, y-w+50, x, y-w+50+h);
line(x, y-w+50+h, x, y-w+50+h+50);
ellipse(x, y-w+50, 12);
ellipse(x, y-w+50+h, 12);
}
//draw the vertical circles
function circless(x, y) {
strokeWeight(1.5);
noFill();
ellipse(x, y, 25);
ellipse(x, y, 10);
ellipse(x, y-25, 7);
ellipse(x, y+25, 7);
ellipse(x, y-42, 7);
ellipse(x, y+42, 7);
}
Looking Outwards 05: 3D Computer Graphics, Section B
Mikael Hvidtfeldt Christensen is a generative artist with a background in physics and computational chemistry. He generates 3D images in a project called Syntopia. His works are varied and experimental. He keeps a blog and a Flickr account of his finished works and his experiments. I admire his sharing the results of his experiments with the world. Even though they are not completed works they are inspiring and show the iterative process that goes into creating a project.
Most of Christensen’s images are geometric renderings of complex shapes and building designs; however he has also created color swapping algorithms and texturizing art. The images from these projects take existing images and sort the colors into layers or add disturbing textures, such as lizard scales to lettuce.
Christensen is passionate about complex systems and has written his own software to generate and render his images. His software, Structure Synth, is available for download and can be found here. It is written in C++, OpenGL, and Qt 4.
Looking Outwards 05
This piece of 3D artwork is by Jakub Javora. He is a 3D artist with a background in studying at the Academy of Fine Arts and over 10 years of experience as a matte painter and concept artist in the movie and commercial industries. His typical works represent lifelike paintings and scenery depictions. He is well-known in the industry and currently works as a concept artist for Paranormal. I admire his creative ability to combine fantasy scenery and characters with reality. He uses the software Maya to create these experimental parallaxes that are usually in the form of 5-10 second GIFs. In one of his interviews, he mentioned that he takes inspiration from nature a lot and also just in his daily life. It’s important to surround yourself with inspiration.
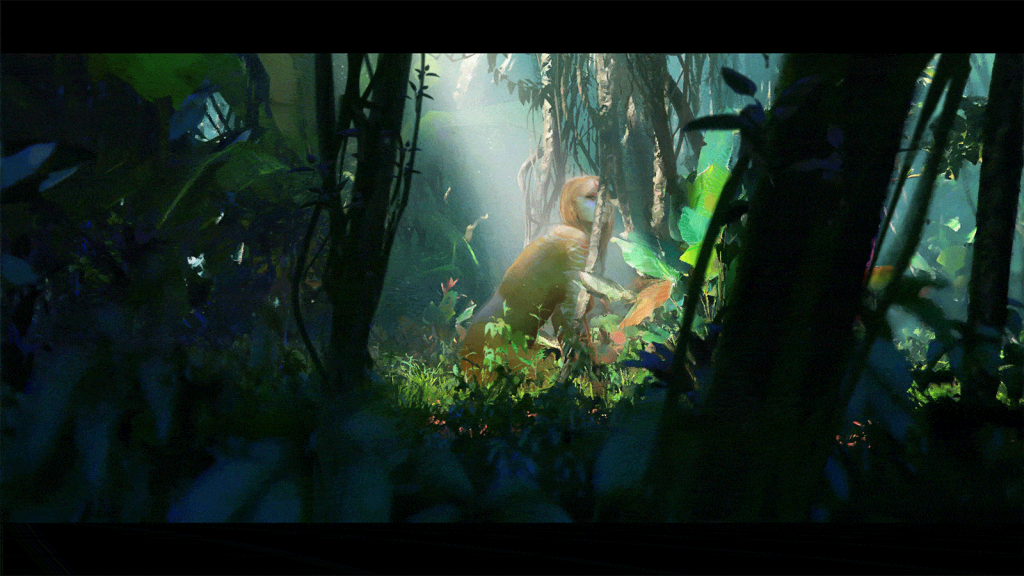
Project 05 – Wallpaper – srauch
Here is my palm frond wallpaper. Vibes.
//sam rauch, section B, srauch@andrew.cmu.edu, project 05
//this code makes a wallpaper pattern with overlapping palm leaves
function setup() {
createCanvas(400, 400);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(16, 28, 48);
//drawing pattern
var direction = 1 //flips direction leaves are facing as they're drawn in the j loop
for (var i = 0; i <= width+110; i+=110){
for (var j = 0; j<= height+100; j+=70){
stem(i,j, 45*direction);
direction = direction*-1;
}
}
noLoop();
}
function portLeaf(a,b,radius, spin){ //make left leaves. must be called at 0,0
push();
translate(a,b);
rotate(radians(spin));
noStroke();
push(); //bottom half
fill(20, 50, 23);
translate(a-(cos(radians(70))*radius), b-(sin(radians(70))*radius));
arc(0, 0, radius*2, radius*2, radians(70), radians(110), CHORD);
pop();
push(); //top half
fill(37, 87, 42);
translate(a+(cos(radians(250))*radius), b-(sin(radians(290))*radius));
arc(0,0, radius*2, radius*2, radians(250), radians(290), CHORD);
pop();
pop();
}
function starLeaf(a,b,radius,spin) { //make right leaves. must be called at 0,0
push();
translate(a,b);
rotate(radians(spin));
noStroke();
push(); //bottom half
fill(20, 50, 23);
translate(a+(cos(radians(70))*radius), b-(sin(radians(70))*radius));
arc(0, 0, radius*2, radius*2, radians(70), radians(110), CHORD);
pop();
push(); //top half
fill(37, 87, 42);
translate(a-(cos(radians(250))*radius), b-(sin(radians(290))*radius));
arc(0,0, radius*2, radius*2, radians(250), radians(290), CHORD);
pop();
pop();
}
function stem(stemX, stemY, spin) {//make a stem, and place leaves along it
push();
translate(stemX, stemY);
rotate(radians(spin));
//leaves at the tip
portLeaf(0,0,50,60);
starLeaf(0,0,50,-60);
portLeaf(0,0,50,80);
starLeaf(0,0,50,-80);
//put leaves along the stem
var radius = 45;
for(var i = 0; i<100; i+=10){
push();
translate(0,i);
portLeaf(0,0,radius, 30);
starLeaf(0,0,radius,-30);
radius += 3;
pop();
}
//line for stem
stroke(20, 60, 25);
strokeWeight(3);
line(0,0, 0, 100);
pop();
}
Project 05
Inspired by the “Evil Eye”, project 5 illustrates a patterned wallpaper with celestial elements and different colored eyes. I enjoyed designing the pattern 🙂
//Jenny Wang
//Section B
//jiayiw3@andrew.cmu.edu
//Project-05
function setup() {
createCanvas(600, 600);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background("lightyellow");
//eye1 nested loop
for(var x = 100; x < width; x+=200){
for(var y = 75; y < height; y += 150){
eye1(x,y);
}
}
//eye2 nested loop
for(var x = -200; x < width+200; x+=200){
for(var y = -147; y < height+200; y += 149){
eye2(x,y);
}
}
//star nested loop
for(var x = -100; x < width+180; x+=200){
for(var y = -150; y < height+140; y += 150){
fill(4,158,177);
star1(x,y);
}
}
//moon nested loop
for(var x = -200; x < width+200; x+=200){
for(var y = 75; y < height; y += 150){
moon(x,y);
}
}
}
function eye1(x,y){
//outside shape
fill("white");
stroke(1,35,133);
strokeWeight(3);
beginShape();
vertex(x-45,y);
bezierVertex(x-5,y-30,x+5,y-30,x+45,y);
bezierVertex(x+5,y+30,x-5,y+30,x-45,y)
endShape();
//innershape
fill(255,234,172);//yellow
noStroke();
beginShape();
vertex(x-35,y);
bezierVertex(x,y-20,x,y-20,x+35,y);
bezierVertex(x,y+20,x,y+20,x-35,y)
endShape();
//iris outer
noStroke();
fill(4,158,177);
ellipse(x,y,43,43);
//array lines
stroke(255,234,172);
strokeWeight(2);
line(x,y+17,x,y-17);
line(x+17,y,x-17,y);
line(x-14,y-14,x+14,y+14);
line(x+14,y-14,x-14,y+14);
//inner iris
noStroke();
fill(1,35,133);
ellipse(x,y,25,25);
fill("white");
ellipse(x,y,5,5);
}
function eye2(x,y){
//outside shape
fill("white");
stroke(4,158,177);
strokeWeight(2);
beginShape();
vertex(x-45,y);
bezierVertex(x-5,y-30,x+5,y-30,x+45,y);
bezierVertex(x+5,y+30,x-5,y+30,x-45,y)
endShape();
//innershape
fill(4,158,177);//lightblue
noStroke();
beginShape();
vertex(x-35,y);
bezierVertex(x,y-20,x,y-20,x+35,y);
bezierVertex(x,y+20,x,y+20,x-35,y)
endShape();
//iris outer
noStroke();
fill(255,234,172);
ellipse(x,y,43,43);
//array lines
stroke(4,158,177);
strokeWeight(2);
line(x,y+17,x,y-17);
line(x+17,y,x-17,y);
line(x-14,y-14,x+14,y+14);
line(x+14,y-14,x-14,y+14);
//inner iris
noStroke();
fill(1,35,133);
ellipse(x,y,25,25);
fill("white");
ellipse(x,y,5,5);
}
function star1(x,y){
noStroke();
beginShape();
vertex(x-3, y+2);
vertex(x, y+20);
vertex(x+3, y+2);
vertex(x+12, y);
vertex(x+3, y-2);
vertex(x, y-20);
vertex(x-3, y-2);
vertex(x-12, y);
endShape();
}
function moon(x,y){
fill(239,172,59);
noStroke();
beginShape();
vertex(x-15,y-5);
bezierVertex(x-5,y,x+5,y,x+15,y-5);
bezierVertex(x+5,y+10,x-5,y+10,x-15,y-5)
endShape();
}
Project -05: Wallpaper
I wanted my wallpaper to look 3D and look like if the light was shining from the top left side!
function setup() {
createCanvas(400, 390);
background(0);
}
function draw() {
rotateGrid(-50,0); //rotate's final grid
}
function drawTriangleOne(x,y) { //draws Triangle 1
strokeWeight(0.1);
fill(150); //Triangle 1
triangle(x, y, x + 50, y, x + 25, y + (sqrt(1875))/2);
fill(80)
triangle(x + 50, y, x + 25, y + sqrt(1875), x + 25, y + (sqrt(1875))/2);
fill(230)
triangle(0, 0, x + 25, y + (sqrt(1875))/2, x + 25, y + sqrt(1875));
}
function drawTriangleTwo(x,y) { //draws Triangle 2
fill(200); //Triangle 2
triangle(x + 50, y, x + 25, y + (sqrt(1875)), x + 50, y + (sqrt(1875))/2);
fill(150);
triangle(x + 50, y, x + 50, y + (sqrt(1875))/2, x + 75, y + sqrt(1875));
fill(80);
triangle(x + 25, y + (sqrt(1875)), x + 50, y + (sqrt(1875))/2, x + 75, y + sqrt(1875));
}
function drawFlipTriangleOne(x,y) { //Draws Triangle 3
push();
translate(-25, sqrt(1875));
drawTriangleTwo(x,y);
pop();
}
function drawFlipTriangleTwo(x,y) { //Draws Triangle 4
push();
translate(25, sqrt(1875));
drawTriangleOne(x,y);
pop();
}
function drawSingleRowTriangles(x,y) { //Draws First Row of Triangles
for (var i = 0; i < 8; i++) {
drawTriangleOne(x,y);
drawTriangleTwo(x,y);
translate(50,0);
}
}
function drawFourStartingTriangles(x,y) { //Groups First Four Starting Triangles
for (var i = 0; i < 9; i++) {
drawTriangleOne(x,y);
drawTriangleTwo(x,y);
drawFlipTriangleOne(x,y);
drawFlipTriangleTwo(x,y);
}
}
function drawTwoRowTriangles(x,y) { //Draws First 2 Rows of Triangles
push();
for (var i = 0; i < 11; i++) {
drawFourStartingTriangles(x,y);
translate(50,0);
}
drawTriangleOne(x,y);
drawFlipTriangleOne(x,y);
pop();
}
function drawTriangleGrid(x,y) { //Uses for loops to create entire Triangle Grid
push();
for (var i = 0; i < 6; i++) {
drawTwoRowTriangles(x,y);
translate(0, 2*sqrt(1875));
}
drawSingleRowTriangles(x,y);
drawTriangleOne(x,y);
drawTriangleTwo(x,y);
pop();
}
function rotateGrid(x,y) {
angleMode(DEGREES);
rotate(30);
translate(0,-200);
drawTriangleGrid(x,y);
}
Looking Outwards – 05: 3D Computer Graphics
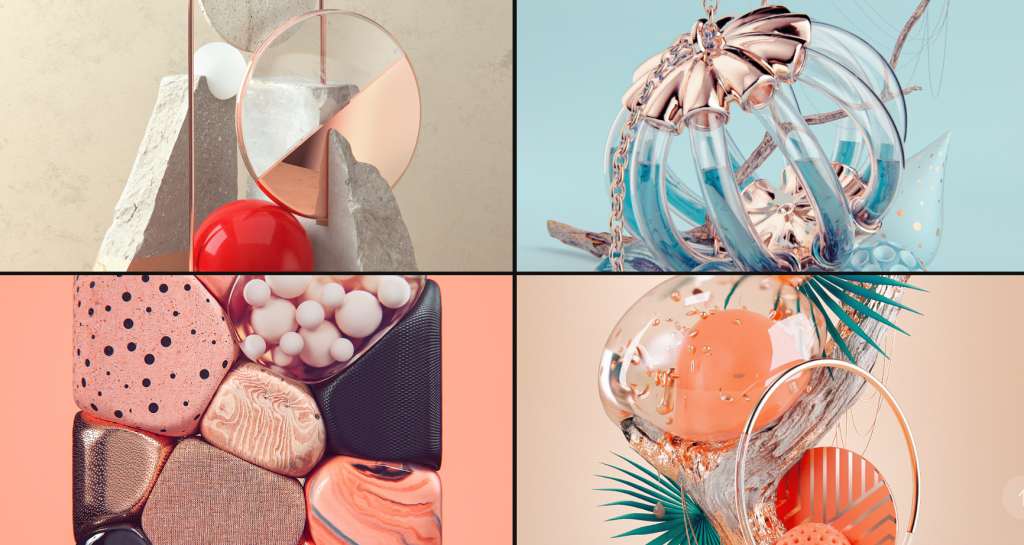
Roman Bratschi is the owner and creative director of his 3D designs made in Switzerland. He is also an experienced animation director, and has experienced his career as a graphic designer. His work is inspired by natural shapes, forms, and patterns. His techniques are expert in exposing their natural simplicity in the final piece. One thing I enjoy about his work is how all of his work reminds me of MacBook Wallpaper. Also, I love how organic some of his work is. Some of them resemble fruits and other vegetables, but they all have the same theme.