For my abstract clock, I was having a creative block and had trouble approaching what to make. I thought since most of my other projects have been pretty literal, I would make this one more abstract with a focus on shapes and how they can change based on hours, minutes, and seconds. I was thinking about how time is focused around growing, until it restarts and how that concept could be reflected into my clock. I decided to have “bubbles” that represent hours, minutes, and seconds organized in columns. I wanted these bubbles to slowly grow based on each of the timings. I also made it so the bubble slowly goes from transparent to opaque as seconds, minutes, and hours increase. Originally, I was thinking I wanted a shape inside the bubbles that would turn based on the time, but decided on a line to draw a better connection to a clock. I gave each bubble a line in the center that rotates based on the timing.
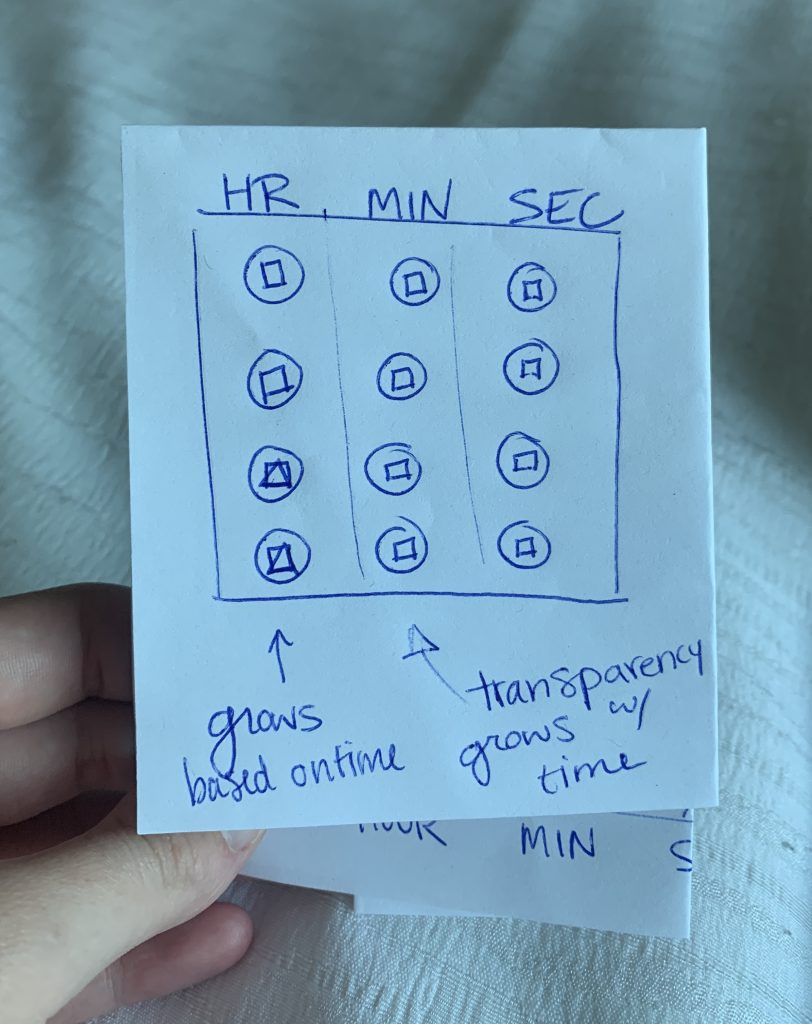
//Rachel Legg / rlegg / section C
var col;
function setup() {
createCanvas(480, 480);
}
function draw() {
//background color changes every hour
background(0);
frameRate(15);
//variables for time
var sec = second();
var min = minute();
var hr = hour();
strokeWeight(2);
//Have Bubbles expand with each increment
//line on each bubble to mimic a clock based on hr, min, sec
//Hr Row
for(col = 0; col <= 3/4 * height; col += 120){
//have hr be yellow
fill(255, 255, 0, 30 + hr); //have opacity grow with time
stroke(255, 255, 0);
ellipse(width/6, height/8 + col, 2 * hr);
//have line in center that moves with change in hr
push();
translate(width/6, height/8 + col);
rotate(radians(-90 + (hr * 15))); // 360/24 = 15
line(0, 0, 10, 10);
pop();
}
//Min Row
for(col = 0; col <= 3/4 * height; col += 120){
//have min be pink
fill(255, 0, 255, 30 + min); //have opacity grow with time
stroke(255, 0, 255);
ellipse(width/2, height/8 + col, 2 * min);
//have line in center that moves with change in min
push();
translate(width/2, height/8 + col);
rotate(radians(-90 + (min * 6))); // 360/60 = 6
line(0, 0, 10, 10);
pop();
}
//Sec Row
for(col = 0; col <= 3/4 * height; col += 120){
//have sec be blue
fill(0, 255, 255, 30 + sec); //have opacity grow with time
stroke(0, 255, 255);
ellipse(5/6 * width, height/8 + col, 2 * sec);
//have line in center that moves with change in sec
push();
translate(5/6 * width, height/8 + col);
rotate(radians(-90 + (sec * 6))); // 360/60 = 6
line(0, 0, 10, 10);
pop();
}
}