// Emily Franco
// efranco
// Section C
//color only randomized when page is loaded
var x = 0;
//-----SLIDER VARS-----
//stores latest mouseX position for slider
var xPos;
//stores past x positions
var pastXPos=0;
//bar height
var barH = 20;
//bar height
var barWidth = 10;
//tracks if mouse has been pressed
var pressed = 0;
//-----DEFAULT FACE VARS----
var eyeWidth = 16;
var eyeHeight = 24;
function setup() {
createCanvas(640, 480);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
//reference for position of face elements
var y_ref_pos = width/2.5;
strokeWeight(0);
background (138,176,162);
//header
fill(0);
textSize (20);
text ('Slide the arrow to pick a face for me.',10,barH+barWidth+20);
//----EMOTION METER----
//meter sliderer mark
fill("black");
triangle (((width/5)*2)+(width/10),barH-2,((width/5)*2)+(width/10)-3,barH-7,((width/5)*2)+(width/10)+3,barH-7);
if (mouseIsPressed){
//draw over 1st triangle background
background (138,176,162);
triangle (xPos=mouseX,barH-2,mouseX-3,barH-7,mouseX+3,barH-7);
pressed = 1;
}else if (pressed==1){
//draw over 1st triangle background
background (138,176,162);
triangle (xPos,barH-2,xPos-3,barH-7,xPos+3,barH-7);
}
//meter
fill (85,180,220); //blue
//very happy
rect(0,barH,(width/5),barWidth);
//happy
fill(193,230,90); //green
rect(width/5,barH,(width/5),barWidth);
//meh...
fill(225,181,37); //yellow
rect((width/5)*2,barH,(width/5),barWidth);
//shock
fill(252,65,18); //red
rect((width/5)*3,barH,(width/5),barWidth);
//angry
fill(137,5,5); //dark red
rect((width/5)*4,barH,(width/5),barWidth);
//--------HAIR-------
//back hair
fill (104, 66, 17); //dark brown
ellipse (width/2, y_ref_pos+28,260,400);
//--------CLOTHES-------
fill (220, 96, 46); //orange
arc((width/2)-32+44,y_ref_pos+158,280,70,Math.PI,0);
//shirt
rect((width/2)-87,y_ref_pos+140,181,180);
//------DEFALUT FACE-----
strokeWeight (.25);
//base ears
fill (238, 217, 197); //beige
ellipse ((width/2)-106,y_ref_pos,32,60);
ellipse ((width/2)+106,y_ref_pos,32,60);
//neck
fill (238, 217, 197);//beige
ellipse((width/2)+1, y_ref_pos+130,90,60);
strokeWeight (0);
rect((width/2)-44, y_ref_pos+90,90,40);
//base face
stroke("black");
strokeWeight (.5);
ellipse (width/2,y_ref_pos,200,232);
if (pressed == 1){
//nose
strokeWeight (0);
fill (229, 155, 99); //orange
triangle (width/2,y_ref_pos-20,(width/2)-20,y_ref_pos+40, width/2,y_ref_pos+38);
}
//-----EXPRESSIONS----
//mouse position over emotion meter changes face expression
//VERY HAPPY
if (xPos<width/5){
//outter eye
strokeWeight (0.25);
fill (242,239,234); //white
stroke (58,37,22); //dark brown
circle ((width/2)-46,y_ref_pos-20,eyeWidth+40);
circle ((width/2)+46,y_ref_pos-20,eyeWidth+40);
//eye pupil
fill (58,37,22); //dark brown
circle ((width/2)-46,y_ref_pos-20,eyeWidth+30);
circle ((width/2)+46,y_ref_pos-20,eyeWidth+30);
//eye highlight
fill (242,239,234); //white
circle ((width/2)-46,y_ref_pos-20,eyeWidth);
circle ((width/2)+46,y_ref_pos-20,eyeWidth);
//eye small highlights
fill (242,239,234); //white
ellipse ((width/2)-56,y_ref_pos-30,eyeWidth-10);
ellipse ((width/2)+56,y_ref_pos-30,eyeWidth-10);
//mouth
strokeWeight (1);
stroke("black");
fill (233, 161, 135); //pink
arc((width/2)-2,y_ref_pos+55,80,50,0,3.15);
line ((width/2)+38,y_ref_pos+55,(width/2)-42,y_ref_pos+55);
//cheeks
fill (233, 161, 135); //pink
strokeWeight (0);
circle((width/2)+54,y_ref_pos+30,40);
circle((width/2)-60,y_ref_pos+30,40);
}
//HAPPY
else if (xPos<(width/5)*2 & xPos>=width/5){
//eyes
fill (58,37,22); //dark brown
ellipse ((width/2)-44,y_ref_pos-20,eyeWidth,eyeHeight);
ellipse ((width/2)+44,y_ref_pos-20,eyeWidth,eyeHeight);
//mouth
strokeWeight (1);
stroke("black");
noFill();
arc((width/2)-2,y_ref_pos+70,20,14,0,3);
//cheeks
fill (233, 161, 135); //pink
strokeWeight (0);
circle((width/2)+44,y_ref_pos+30,40);
circle((width/2)-50,y_ref_pos+30,40);
}
//MEH
else if (xPos<(width/5)*3 & xPos>=(width/5)*2){
//mouth
strokeWeight (1);
stroke("black");
line ((width/2)+40,y_ref_pos+65,(width/2)-40,y_ref_pos+65);
//cheeks
fill (233, 161, 135); //pink
strokeWeight (0);
circle((width/2)+44,y_ref_pos+30,40);
circle((width/2)-50,y_ref_pos+30,40);
//outter eye
fill (58,37,22); //dark brown
circle ((width/2)-46,y_ref_pos-20,eyeWidth);
circle ((width/2)+46,y_ref_pos-20,eyeWidth);
}
//SHOCK
else if (xPos<(width/5)*4 & xPos>=(width/5)*3){
//eyes
fill (58,37,22); //dark brown
ellipse ((width/2)-44,y_ref_pos-30,eyeWidth+6,eyeHeight*2);
ellipse ((width/2)+44,y_ref_pos-30,eyeWidth+6,eyeHeight*2);
//mouth
strokeWeight (1);
stroke("black");
fill (233, 161, 135); //pink
arc((width/2)-2,y_ref_pos+95,40,90,3.15,0);
line((width/2)+18,y_ref_pos+95,(width/2)-22,y_ref_pos+95);
//cheeks
fill (233, 161, 135); //pink
strokeWeight (0);
circle((width/2)+60,y_ref_pos+30,40);
circle((width/2)-60,y_ref_pos+30,40);
}
//ANGRY
else if (xPos>(width/5)*4){
//eyes
fill (58,37,22); //dark brown
arc((width/2)-50,y_ref_pos-20,50,25,0,3.15);
arc((width/2)+50,y_ref_pos-20,50,25,0,3.15);
//eyebrows
strokeWeight (3);
stroke(58,37,22); //dark brown
line ((width/2)-75,y_ref_pos-35,(width/2)-25,y_ref_pos-25);
line ((width/2)+75,y_ref_pos-35,(width/2)+25,y_ref_pos-25);
//mouth
strokeWeight (2);
stroke("black");
noFill();
arc((width/2)-2,y_ref_pos+80,30,40,3.1,0);
}
//------BODY-----
//shoulders
strokeWeight (0);
fill (238, 217, 197); //beige
circle((width/2)-120, y_ref_pos+182,80);
circle((width/2)+126, y_ref_pos+180,80);
//arms
rect((width/2)-160,y_ref_pos+180,80,140);
rect((width/2)+86,y_ref_pos+180,80,140);
//-----DETAILS----
//earings
fill (111, 115, 210); //purple
square ((width/2)-114,y_ref_pos+30,16);
square ((width/2)+100,y_ref_pos+30,16);
//bangs
push();
strokeWeight(0);
fill (104, 66, 17); //brown
rotate (-0.9);
ellipse (width/2-230, y_ref_pos+140,40,150);
rotate (1.7);
ellipse (width/2-5, y_ref_pos-330,40,150);
pop();
//hairclip
//random color generated in first loop and only changes when page is reloaded
x=x+30;
if (x==30) {
stroke(r=random(200),g=random (200),b=random(200));
}else{
stroke(r,g,b);
}
strokeWeight(4);
line(width/2+50,y_ref_pos-60,(width/2)+80,y_ref_pos-80);
//shirt details
strokeWeight(8);
stroke(r,g,b);
point(width/2, y_ref_pos+200);
}
Category: SectionC
Project – 03
Blog – 03
I am most inspired by the work of Amanda Ghassaei. She has been making groundbreaking artwork for years and continues to push computational art’s limits. One project of hers that I admire is her locked letters project which she worked on in 2021. Letter-locking is the practice where a letter becomes its own envelope through a series of complex folds. Ghassaei uses x-ray microtomography and a fascinating “virtual unfolding” method to read sealed letters without even opening them. Although I am not familiar with microtomography, according to her website she uses “3D reconstruction of the folded packet using data collected by a high-resolution microtomography scanner” to create her artwork. Additionally, I really admire her work on 3D printing a record player(2012). I commend this because it is a rarity in the 3D printing realm, and it actually produces music just like any other vinyl! According to her website, she imported raw audio data, did some geometrical calculations, and eventually exported this geometry directly to a 3D printable file format. Check her work out below!
Locked Letters (2021)
https://amandaghassaei.com/projects/locked_letters/
3D Printed Record (2012)
https://amandaghassaei.com/projects/3D_printed_record/
By: Katie Makarska
anabelle’s project 03
This is my project! Try making the sky change from sundown to midnight, see the moon change phases, and the stars glitter! You can try to pet the cat as well <3
// kayla anabelle lee (kaylalee)
// section c
// project 03 ^^
moonX = 200;
moonY = 150;
outline = 55;
inline = 50;
let direction = 0.8;
let speed = 1;
let starDiam = 20;
let redValue = 231;
let greenValue = 157;
let blueValue = 193;
let skyScraperX = 300;
let skyScraperY = 100;
let happyMouthX = 60;
let happyMouthY = 450;
let triangleWindowX = 225;
let triangleWindowY = 185;
let catDirection = 1;
let catAngle = 1;
let moonSpin = 0;
function setup () {
createCanvas(450, 600);
}
function draw() {
print(catAngle);
background(redValue, greenValue, blueValue);
// constrain the rbg values to the 2 sky colors i want
redValue = constrain(redValue, 46, 231);
greenValue = constrain(greenValue, 26, 157);
blueValue = constrain(blueValue, 71, 193);
// turns dusk when mouse on left, turns night when mouse on right
if (mouseX > width/2) {
redValue -= 3;
blueValue -= 3;
greenValue -= 3;
} if (mouseX < width/2) {
redValue += 3;
blueValue += 3;
greenValue += 3;
}
// moon cycle
if (mouseX <= 100) {
newMoon(35, 290, 30, 30);
} if (100 < mouseX & mouseX <= 200) {
crescentMoon(115, 110, 30, 30);
} if (200 < mouseX & mouseX <= 300) {
gibbousMoon(190, 50, 30, 30);
} if (300 < mouseX) {
fullMoon(300, 50, 30, 30);
}
// triangular building
stroke(164, 96, 175);
strokeWeight(1);
fill(119, 127, 199);
fill(230, 209, 242);
quad(95, 600, 220, 170, 390, 170, 390, 600);
// triangle building windows
// im sorry i couldnt use a forLoop for these as well -- whenever i tried, the program wouldnt load
triangleWindow(triangleWindowX,triangleWindowY);
triangleWindow(triangleWindowX - 5, triangleWindowY + 20);
triangleWindow(triangleWindowX - 10, triangleWindowY + 40);
triangleWindow(triangleWindowX - 15, triangleWindowY + 60);
triangleWindow(triangleWindowX - 20, triangleWindowY + 80);
triangleWindow(triangleWindowX - 25, triangleWindowY + 100);
triangleWindow(triangleWindowX - 30, triangleWindowY + 120);
triangleWindow(triangleWindowX - 35, triangleWindowY + 140);
triangleWindow(triangleWindowX - 40, triangleWindowY + 160);
triangleWindow(triangleWindowX - 45, triangleWindowY + 180);
triangleWindow(triangleWindowX - 50, triangleWindowY + 200);
triangleWindow(triangleWindowX - 55, triangleWindowY + 220);
triangleWindow(triangleWindowX - 60, triangleWindowY + 240);
triangleWindow(triangleWindowX - 65, triangleWindowY + 260);
// skyscraper
fill(217, 196, 236);
stroke(164, 96, 175);
strokeWeight(1);
rect(300, 100, 150, 500);
rect(350, 45, 100, 55);
triangle(350, 40, 450, 0, 530, 40);
// skyscraper windows
for(skyScraperX = 300; skyScraperX < 440; skyScraperX += 40) {
for(skyScraperY = 100; skyScraperY < 650; skyScraperY +=40) {
push();
translate(20, 20);
skyWindow();
pop();
}
}
// happymouth building
stroke(164, 96, 175);
strokeWeight(1);
fill(204, 183, 229);
rect(40, 430, 195, 170);
rect(50, 380, 170, 40);
textSize(25);
fill(244, 244, 211);
stroke(119, 127, 199);
text("happyMouth()", 60, 405);
// happymouth windows
for(happyMouthX = 40; happyMouthX < 200; happyMouthX += 60) {
for(happyMouthY = 420; happyMouthY < 600; happyMouthY += 30) {
push();
translate(15, 30);
happyWindow();
pop();
}
}
// if mouse on happymouth sign, stars pulse AND crescent moon rotates
if (dist(135, 390, mouseX, mouseY) < 30) {
starrySky();
starDiam += direction * speed;
if (starDiam > 30) {
direction = -direction;
starDiam = 30;
} if (starDiam < 0) {
direction = -direction;
starDiam = 0;
}
} else starrySky();
// rooftop building
fill(177, 156, 216);
stroke(64, 96, 175);
quad(185, 550, 270, 420, 430, 420, 410, 550);
fill(190, 169, 223);
rect(185, 550, 225, 50);
quad(410, 600, 410, 550, 430, 420, 430, 600);
// cat perch and cat
fill(117, 80, 166);
rect(0, 180, 150, 60);
cat(130, 120, 10, 10)
triangle(43, 69, 39, 78, 45, 78);
triangle(74, 82, 64, 84, 72, 93);
push();
rotate(radians(catAngle*catDirection));
push();
scale(0.7);
rotate(radians(-20));
catTail(-50, 250, catAngle*catDirection);
pop();
pop();
// cat tail animation
if (dist(60, 50, mouseX, mouseY) < 50) {
push();
rotate(radians(catAngle*catDirection));
push();
scale(0.7);
rotate(radians(-20));
catTail(-50, 250, catAngle*catDirection);
pop();
pop();
catAngle -= 1;
if (catAngle < -10) {
catAngle = -10;
}
} if (dist(40, 120, mouseX, mouseY) < 50) {
catAngle += 1;
if (catAngle > 10){
catAngle = 10;
}
}
}
// my catalogue of functions
function cat(x, y, w, h) {
fill(50);
noStroke();
ellipse(x/2, y/2, 50, 50);
ellipse(x/2 + 17, y/2 + 5, 25, 28);
ellipse(x/2 - 20, y/2 + 65, 70, 100);
arc(x/2 - 20, y/2 + 120, 90, 70, radians(180), radians(0));
triangle(x/2 + 5, y/2 - 15, x/2 + 20, y/2 - 15, x/2 + 13, y/2 - 35);
triangle(x/2 - 5, y/2 - 15, x/2 - 20, y/2 - 15, x/2 - 13, y/2 - 35);
bezier(x/2 - 20, y/2 + 5, x/2 - 30, y/2 + 30, x/2 - 30, y/2 + 20, x/2 - 40, y/2 + 25);
bezier(x/2 + 10, y/2 + 20, x/2 + 5, y/2 + 30, x/2 + 5, y/2 + 40, x/2 + 13, y/2 + 55);
}
function catTail(x, y, catAngle) {
noStroke();
beginShape();
curveVertex(x, y);
curveVertex(x, y);
curveVertex(x - 5, y + 40);
curveVertex(x + 20, y + 100);
curveVertex(x + 80, y + 140);
curveVertex(x + 150, y + 150);
curveVertex(x + 160, y + 140);
curveVertex(x + 145, y + 120);
curveVertex(x + 70, y + 100);
curveVertex(x + 40, y + 50)
curveVertex(x + 30, y);
curveVertex(x + 30, y);
endShape();
}
function triangleWindow(triangleWindowX, triangleWindowY) {
fill(244, 244, 211);
quad(triangleWindowX, triangleWindowY, triangleWindowX -5, triangleWindowY + 15, triangleWindowX + 200, triangleWindowY + 15, triangleWindowX + 200, triangleWindowY);
}
function happyWindow() {
rect(happyMouthX, happyMouthY, 50, 15);
}
function skyWindow() {
fill(244, 244, 211);
rect(skyScraperX, skyScraperY, 20, 20);
}
function newMoon(newX, newY, w, h) {
outline = 55;
inline = 50;
stroke(255);
fill(redValue, greenValue, blueValue);
ellipse(newX, newY, outline, outline);
ellipse(newX, newY, inline, inline);
}
function crescentMoon(crescentX, crescentY, w, h) {
outline = 55;
inline = 50;
fill(255);
ellipse(crescentX, crescentY, inline, inline);
fill(redValue, greenValue, blueValue);
noStroke();
rect(115, 80, outline/2, outline)
stroke(255);
noFill();
ellipse(crescentX, crescentY, outline, outline);
fill(redValue, greenValue, blueValue);
noStroke();
ellipse(crescentX, crescentY, inline/2, inline);
}
function gibbousMoon(gibbousX, gibbousY, w, h) {
outline = 55;
inline = 50;
noStroke();
fill(255);
ellipse(gibbousX, gibbousY, inline, inline);
fill(redValue, greenValue, blueValue);
noStroke();
fill(redValue, greenValue, blueValue);
rect(190, 24, outline/2, outline);
stroke(255);
noFill();//Outline
ellipse(gibbousX, gibbousY, outline, outline);
fill(255)
arc(gibbousX, gibbousY, inline/2, inline, PI/2, -PI/2);
}
function fullMoon (fullX, fullY, w, h) {
outline = 55;
inline = 50;
noFill();
stroke(255);
ellipse(fullX, fullY, outline, outline);
fill(255);
ellipse(fullX, fullY, inline, inline);
}
function starrySky() {
noStroke();
fill(253, 253, 150);
circle(140, 30, starDiam/5);
circle(275, 136, starDiam/7);
circle(61, 303, starDiam/4);
circle(167, 38, starDiam/6);
circle(10, 34, starDiam/7);
circle(163, 147, starDiam/3);
circle(292, 121, starDiam/8);
circle(154, 328, starDiam/5);
circle(360, 15, starDiam/2);
circle(19, 386, starDiam/6);
}
// ALL moon functions were made by brantschen at https://editor.p5js.org/brantschen/sketches/bGH-klhrY
// cat mechanics inspired by jiatong li
LookingOutwards-03
In collaboration, multiple designers, including Arturo Tedeschi, Michael Pryor, Pavlina Vardoulaki, and Matteo Silverio, designed a suspension lamp called HorisON. Inspiration for this lamp draws on the contrast between high-tech and traditional handcrafting. These designers speculate that future design will not depend on the constant technological improvement of products and their production, but rather on an integration of the high-tech with humanistic characteristics, such as uniqueness in imperfection. The lamp is made up of two main parts a 3D printed, parametric form that diffuses LEDs inside and a handcraft shell, made in Murano in Italy. During the day, when the lamp is off the shell hides the inner coils and becomes the centerpiece of the lamp. When one, the lamp’s 3D printed core becomes the centerpiece. By emphasizing the combination of human and high-tech, I believe these designers are bridging a very quickly growing gap of disinterest and devaluing of items brought by mass production. Both the handcrafted and paramedic design are objects deserving of appreciation and awe but each is perceived differently. The handcraft part tells a story of craftsmanship and offers people the opportunity to appreciate the skill of the artist while the parametric design offers interest as an unusual item that represents the modern.
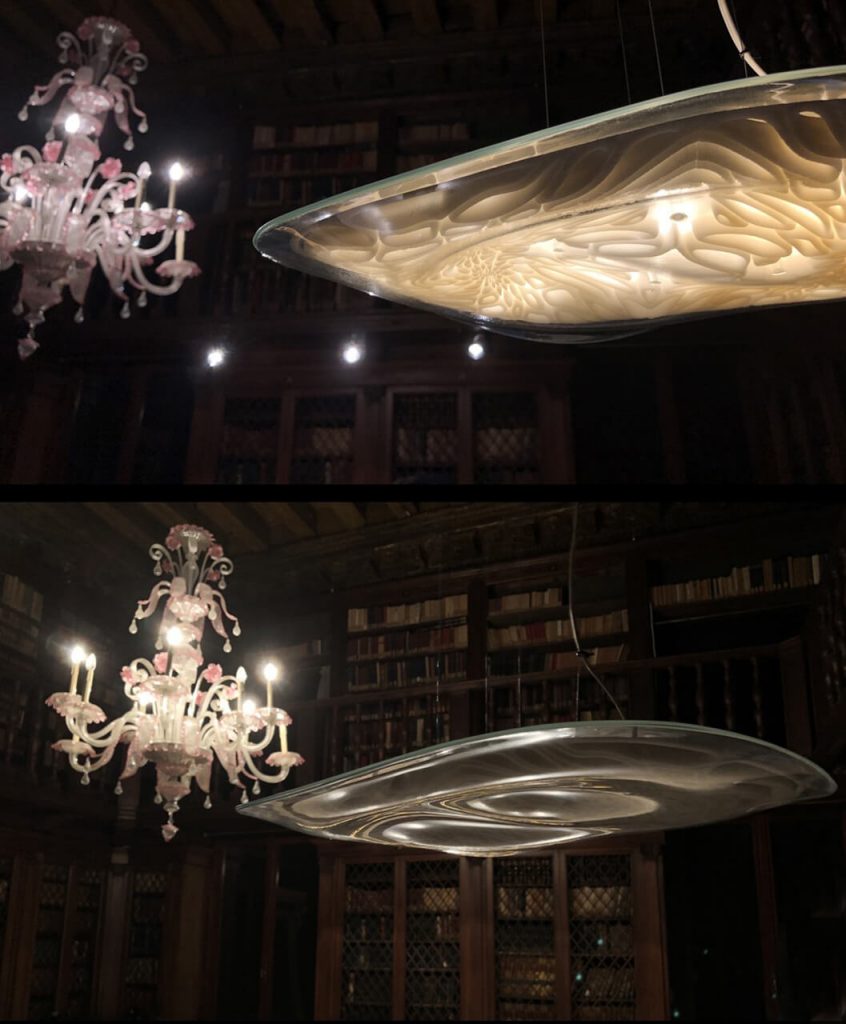
Looking Outwards-03
If you have read my first blog post, you will remember that I am an advocate for fashion design, especially if the usage of advanced technology are involved. Through this class, I have been able to discover a variety of different computational design artists. In this blog, I would like to highlight Nervous System‘s famous and innovative 3D printed Kinematic fabric dress, which has been featured in an exhibition at The Museum of Modern Art in New York.
Nervous System has created their first dress using Kinematics, a unique 3D printing system that creates complex, flexible forms of articulated modules. They first use WebGL, a JavaScript API for rendering 2D and 3D graphics, to write computer programs based on processes and patterns inspired by nature. The team also uses 3D scanning and a physics simulation to fold a piece of fabric into the machine for fabrication, and later is printed with SLS technology. The idea is that once the fabric piece is removed from the machine, it can unfold into a dress.
I greatly admire the intricate detail and the thousands of unique interlocking elements that were incorporated into the dress. I believe it showcases the future of both sustainably made garments and fashion in general, with digital fabrication making any designer’s wildest ideas come true. Nervous System mainly draws inspiration from natural phenomena, and I can see how they implement the complexities of nature in their designs. Their design platform pushes fashion and computational fabrication in a way where they are able to express and customize their concepts with CAD software and 3D printing to create evolutional and affordable wearable art.
Looking Outward – 03
Work Title: Momentum, 2008, Hollywood Hills
Artist: Matt McConnell
https://www.mattmcconnell.com/momentum https://www.youtube.com/watch?v=puuTwNM3-sI&t=49s
Designed by McConnell Studio are these interactive kinetic sculptures with sound elements embedded in them. The concept is to reflect the millipede’s motion of walking. When millipedes move, their legs move in a wave-like motion from back to forth. As the first pair of legs begin to go down, the second pair of legs are already coming up. I think this idea of biomimicry — taking what we observe in nature and making it become part of the art piece —is a valuable design process. Because the structure and form of the artwork are not decided randomly, it already has a clear algorithm methodology such as repetition, arraying, rotating, etc. Fundamental functions that can potentially generate these patterns include loops, mapping, and bezier curves. There is also sound incorporated into this artwork, which is probably caused by the movement of each curve. So each curve segment is given a pitch, and when the curve is in motion in a particular direction, the sound plays with a combination of different sound waves.
Project 03 Dynamic Drawing
For this project, I wanted some kind of flower scene. I had a lot of fun figuring out how to make it seem like they can grow based on the position of the mouse, along with making the cloud interactive both with the sun and the mouse. I added the spinning parts to try the rotate and push & pop methods and figure out how they work.
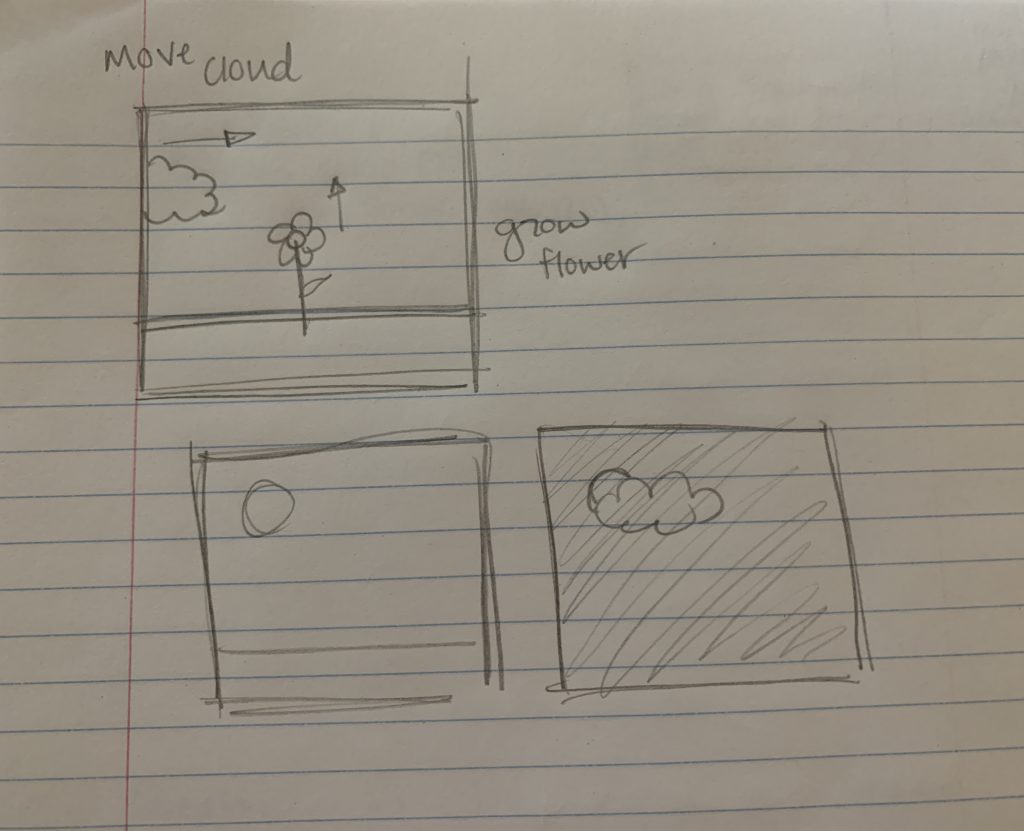
// Rachel Legg / rlegg / Section C
var x;
var y;
var offset = 5;
var fOne;
var fThree;
var edge = 599;
var angle = 0;
function setup() {
createCanvas(600, 450);
background(255);
x = 1/5 * width;
}
function draw() {
//if mouse is on right side, it is night , or else it is day
if (mouseX <= width / 2){
background(135, 206, 235); //dark blue
noStroke();
ellipse(75, 75, 40);
fill("yellow");
noStroke();
ellipse(75, 75, 40);
}else{
background(0, 0, 102);
fill(255);
noStroke();
ellipse(75, 75, 40);
fill(0, 0, 102);
ellipse(80, 70, 40);
}
//ground
fill(111, 78, 55); //brown
noStroke();
rect(0, 4/5 * height, width, 4/5 * height);
fill("green"); //grass
rect(0, 4/5 * height, width, 20);
//flower 1
//flower stem grows and shrink based on mouse location
if(mouseX < 200 & mouseY <= (4/5 *height)){
stroke("green");
line(1/4 * width, 4/5 * height, 1/4 * width, mouseY);
fill(255);
noStroke();
fOne = 1/4 * width;
circle(fOne, mouseY - 17, 30);
circle(fOne - 20, mouseY - 5, 30);
circle(fOne + 20, mouseY - 5, 30);
circle(fOne - 12, mouseY + 13, 30);
circle(fOne + 12, mouseY + 13, 30);
fill("yellow");
stroke("pink");
strokeWeight(4);
circle(1/4 * width, mouseY, 20);
//flower spinds when not interacting
}else{
strokeWeight(4);
stroke("green");
line(1/4 * width, 4/5 * height, 1/4 * width, 2/3 * height);
fill(255);
noStroke();
push();
translate(150, 300);
rotate(radians(angle));
circle(0, -17, 30);
circle(-20, -5, 30);
circle(20, -5, 30);
circle(-12, 13, 30);
circle(12, 13, 30);
fill("yellow");
stroke("pink");
strokeWeight(4);
circle(0, 0, 20);
pop();
angle += 2
}
//flower 2
if(mouseX < 400 & mouseX > 200 && mouseY <= (4/5 *height)){
stroke("green");
line(width / 2, 4/5 * height, width / 2, mouseY);
fill(250, 172, 382);
noStroke();
circle(width / 2, mouseY, 40, 40);
fill(77, 208, 99);
circle(width / 2, mouseY, 25, 25);
fill(255, 51, 51);
circle(width / 2, mouseY, 10, 10);
}else{
strokeWeight(4);
stroke("green");
line(width / 2, 4/5 * height, width / 2, 2/3 * height);
fill(250, 172, 382);
noStroke();
circle(width / 2, 2/3 * height, 40, 40);
fill(77, 208, 99);
circle(width / 2, 2/3 * height, 25, 25);
fill(255, 51, 51);
circle(width / 2, 2/3 * height, 10, 10);
}
//flower 3
if(mouseX > 400 & mouseY <= (4/5 *height)){
stroke("green");
line(3/4 * width, 4/5 * height, 3/4 * width, mouseY);
noStroke();
fThree = 3/4 * width;
fill(0, 128, 255);
circle(fThree + 20, mouseY - 17, 25);
circle(fThree - 20, mouseY - 17, 25);
circle(fThree + 20, mouseY + 17, 25);
circle(fThree - 20, mouseY + 17, 25);
fill(0, 51, 102);
ellipse(fThree, mouseY - 17, 20, 30);
ellipse(fThree - 20, mouseY, 30, 20);
ellipse(fThree + 20, mouseY, 30, 20);
ellipse(fThree, mouseY + 17, 20, 30);
fill(255, 153, 204);
circle(3/4 * width, mouseY, 30);
fill(255, 51, 153);
stroke(204, 204, 255);
strokeWeight(4);
circle(3/4 * width, mouseY, 20);
}else{
strokeWeight(4);
stroke("green");
line(3/4 * width, 4/5 * height, 3/4 * width, 2/3 * height);
fill(255);
noStroke();
noStroke();
push();
translate(450, 300);
rotate(radians(angle));
fill(0, 128, 255);
circle(20, -17, 25);
circle(-20, -17, 25);
circle(20, 17, 25);
circle(-20, 17, 25);
fill(0, 51, 102);
ellipse( 0, -17, 20, 30);
ellipse(-20, 0, 30, 20);
ellipse(20, 0, 30, 20);
ellipse(0, 17, 20, 30);
fill(255, 153, 204);
circle(0, 0, 30);
fill(255, 51, 153);
stroke(204, 204, 255);
strokeWeight(4);
circle(0, 0, 20);
pop();
angle += 2
}
//cloud follows mouse back and forth
if (mouseX > x) {
x += 0.5;
offset = -5;
}
if (mouseX < x) {
x -= 0.5;
offset = 5;
}
if (x > 599){ //constrain to frame
x = 599;
}
fill(255);
noStroke();
ellipse(x, 100, 100);
ellipse(x + 50, 110, 70);
ellipse(x - 50, 110, 70);
//if cloud covers sun, screen gets darker
var shadeCover = 20;
if (x <= 125 & x >= 30){
background(0, 0, 102, shadeCover);
}
}
Looking Outward – 03
Digital Grotesque is a creation of architecture where the artist, Benjamin Dillenburger, uses algorithms to generate the geometry, and then uses sand printing to create the builds. The 3D printed sandstone parts (which are made up of sandstone and resin) can then be connected together to make up the completed piece, and it is huge. It is essentially printing architecture!! The artist uses a computer to generate very intricate, complex parts, and it is absolutely amazing to me that the computer was able to execute this amount of detail at such a large scale without needing any intervention. I also really admire the use of texture that is able to come through. Dillenburger shares that the precision is down to millimeters and that this kind of detail could never be drawn by hand as there are 260 million facets and 30 billion voxels. I often have thought about how modern buildings have lost their detailed quality, and this technology makes me wonder how the future of architecture and style could change with generative processes and technology.
Title: Digital Grotesque (2013)
Artist: Benjamin Dillenburger
Link: http://benjamin-dillenburger.com/grotto/
The Foldable Fractal
The project I found that I admire is “Foldable Fractal 2.0” by David Dessens who is a generative artist. This project was from 2008.
I admire the visual look of it a lot. It is mesmerizing and beautiful to just look at. It is also gives off clock vibes to me, and makes me think of time passing or gears turning, even though the sculpture does not move. The design of it is simple as well, it seems to just be a pattern of hexagons that repeat within each other to create the structure. I admire how peaceful it seems too. It is a design that invites the viewer to stare and dissect.
The algorithms that were used to generate this art was through using a recursive algorithm that is based on a lindermayer system. It uses a recursive algorithm of a pentagon shape after folding a pentagonal dodecahedron. I believe the artist used a program called Generator.x 2.0 to build the algorithm and sculpture.
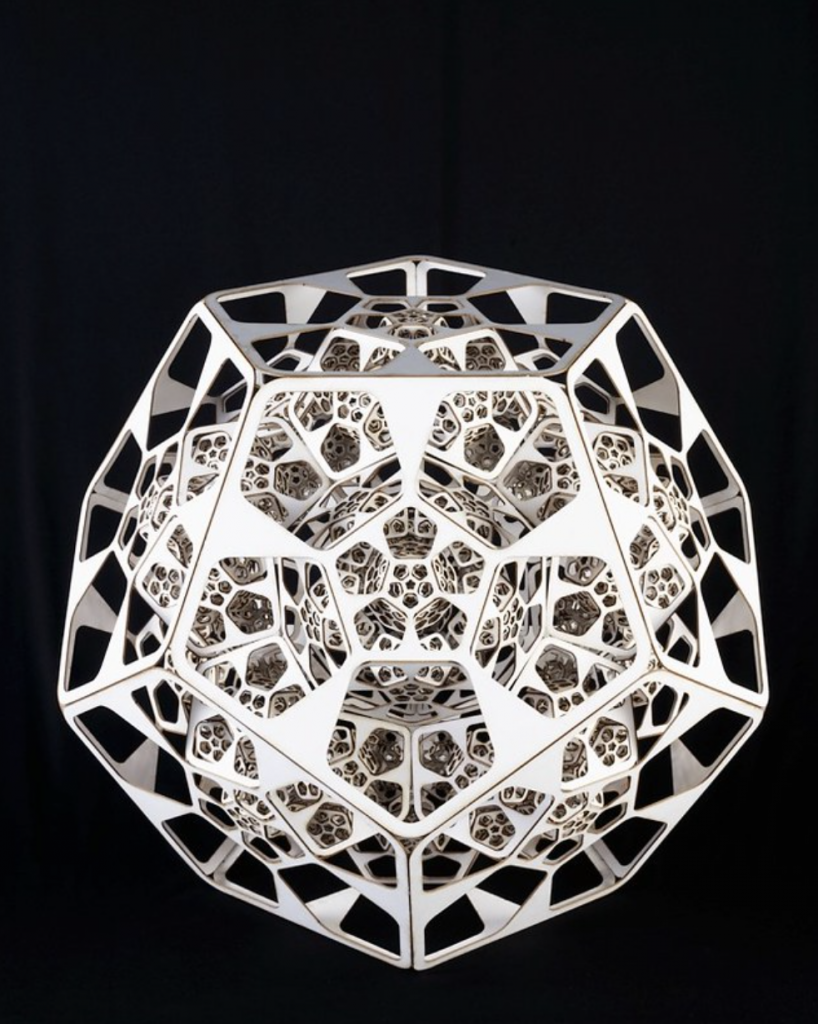