// Bridget Doherty, bpdohert, 104 Section C
// draw at least 3 string art shapes
var dx1;
var dy1;
var dx2;
var dy2;
// line density
var numLines = 40;
// starting line values
var x1 = 0;
var y1 = 0;
var x3 = 0;
var y3 = 300;
// second line values
var x2 = 400;
var y2 = 300;
var x4 = 400;
var y4 = 0;
var mouseClick = 0;
function setup() {
createCanvas(400, 300);
background('black');
blendMode(EXCLUSION);
}
function draw() {
drawCircles();
string1();
string2();
string3();
noLoop();
}
function drawCircles() {
for (i = 20; i < width; i+= 40) {
fill(250);
noStroke();
circle(i*1.3, i, 40);
}
}
function string1() {
for(i = 0; i <= height; i += 10) {
stroke('green');
line(width/2.5, height/2, 0, i);
line(width/2.5, height/2, width, i);
}
for(i = 0; i <= width; i += 10){
stroke('yellow');
line(width/3 + width/2, height/2, 0, i);
line(width/3 + width/2, height/2, width, i);
}
}
function string2() {
stroke('blue');
dx1 = (300-50)/numLines;
dy1 = (0)/numLines;
dx2 = (50-300)/numLines;
dy2 = (300-300)/numLines;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
}
function string3() {
stroke('cyan');
dx1 = (300-50)/numLines;
dy1 = (0)/numLines;
dx2 = (50-300)/numLines;
dy2 = (300-300)/numLines;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
}
}
Category: Uncategorized
LO-04
I get to talk about my favorite coding platform Max again! I found this pretty interesting granular synthesis patch, where the creator set up a patch where you can take samples of music playing and loop them back quickly, and still be locked into the set tempo (not sure if this is algorithmically read off of the sound or input by the person running the patch) when you release it. Makes for some pretty cool audio effects. The creator demonstrates this using a sort of folk-world-choral piece, with mostly vocals and drums/percussion, but it would be really interesting to use this on a multitrack recording of an orchestra or other multi-part ensemble, to play with different parts of the music while the rest of the orchestration continues underneath it. This video is from 2013, and the software has improved a lot since then, so I would be really interested to play with the patch in a newer version of Max and see how I could clean it up in presentation mode so it could possibly be used in a live performance format.
Looking Outward-04
Francesco Mauro
Section D
I looked into the music of Robert Henke, and enjoyed it. The music has a wide dramatic sound, and invokes some sort of primal feeling. It feels immersive and vast. One of his songs sounded like you were standing on the edge of that one cavern from planet earth looking down. He is a minimalist from Germany, and it matches well with his computational music making. The absolute minimalism makes me feel like I’m listening for the truth within each sound. The control he obviously has on each sound allows slight movements to feel like massive musical sweeps. He speaks about his creation involving a tweaking and tuning of algorithms looking for patterns that are pleasing. Looking for an unexpected effect within the rigid parameters. A very approachable method.
Lines: A face
For this project, I tried to make a face out of simple lines. When constructing faces, it is always interesting to see how simplistic or complicated you could make it through the assumptions of what is considered a face.
var dx1;
var dy1;
var dx2;
var dy2;
var numLines = 50;
function setup() {
createCanvas(400, 400);
background(200);
line(50, 50, 150, 300);
line(300, 300, 350, 100);
line(10,380,180,300);
line(280,260,370,390);
dx1 = (150-50)/numLines;
dy1 = (300-50)/numLines;
dx2 = (350-300)/numLines;
dy2 = (100-300)/numLines;
}
function draw() {
fill(0);
triangle(200,0,140,100,180,110);
triangle(180,140,200,120,280,145);
triangle(190,145,200,160,240,150);
var x1 = 50;
var y1 = 50;
var x2 = 300;
var y2 = 300;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
} for (var i = -150; i <= numLines * 2; i += 4) {
line(x1, y1, x2, y2);
x1 += dx1 / 2;
y1 += dy1 / 2;
x2 += dx2 / 2;
y2 += dy2 / 2;
//eyelashes i think
} for (var i = 1; i <= numLines * 2; i += 4) {
line(x2 * 5, y1 , x1 -50, y2 + 40);
var x1 = 0;
var x2 = 200;
var y1 = 300;
var y2 = 350;
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
//random lines for texture
} for (var i = 0; i <= numLines; i += 2) {
line(x1, y1 - 300, x2, y2 - 300);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
//hair
} for (var i = 0; i <= numLines; i += 2) {
x2 = 80;
y2 = 30;
line(x1, y1 - 300, x2, y2 - 300);
x1 -= dx1;
y1 -= dy1;
x2 += dx2;
y2 += dy2; }
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2; }
x1 = 10;
y1 = 380;
x2 = 280;
y2 = 260;
for (var i = 0; i <= numLines; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2; }
noLoop();
}
04-Blog Post
Sonumbra was made as an interactable space in 2006 by Loop.pH, and utilized the movements of the audience. This entire project not only was so beautiful to look at, but also so admirable in how they treated the entire aesthetic of the place, taking into account the multiple variables of a setting, including sound, placement, sizing, and lighting. I suppose the algorithms took in the movements of the visitors and paired them with the fiber lights that made up the structure as well as computer-generated sound. The creator did an excellent job creating beauty in the structure that could have otherwise been rather chaotic or ugly-looking.
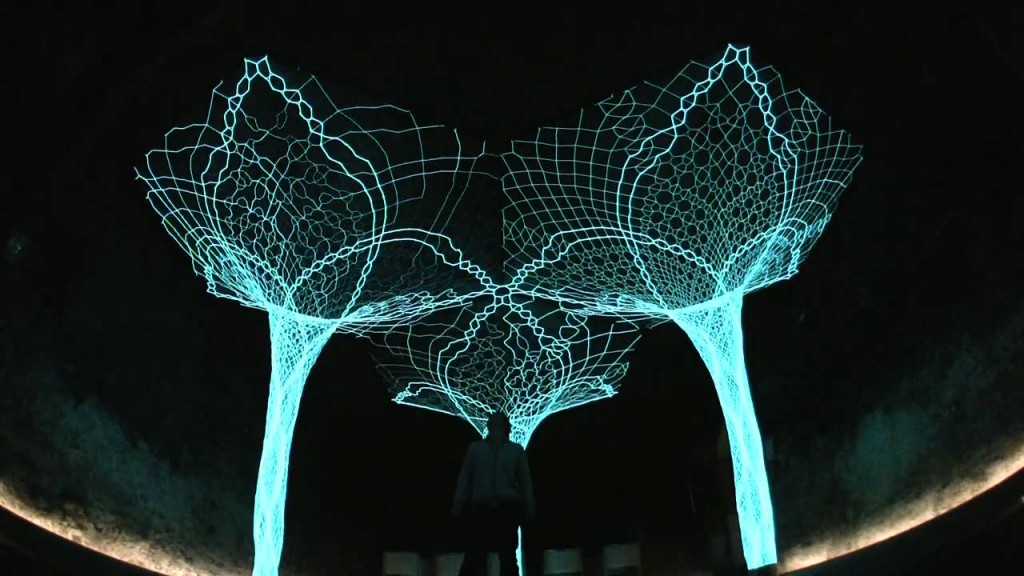
Link here
Project-04: String Art-Section B
All Seeing Eye.
/*
* Evan Stuhlfire
* estuhlfi@andrew.cmu.edu
* Section B
*
* Project-04: String Art
* This program uses geometric shapes to create
* string art.
*/
function setup() {
createCanvas(400, 300);
background(73, 80, 87); //davys grey from coolors.com
}
function draw() {
// draw the string lines for the frame in light blue
stringLeftLower(204, 255, 255, 55);
stringLeftUpper(204, 255, 255, 55);
stringRightUpper(204, 255, 255, 55);
stringRightLower(204, 255, 255, 55);
// move origin of canvas to center
translate(width/2, height/2);
// draw the circle design with light blue
stringCircle(204, 255, 255);
noLoop();
}
/* draw the string pattern at the lower left */
function stringLeftLower(r, g, b, t) {
var numLines = 40;
var x1 = 0; // start at top right
var y1 = 0; // top right
var y2 = height;
var x2 = xInc = (width/numLines);
var yInc = (height/numLines);
stroke(r, g, b, t);
// iterate over each line to draw
for (index = 0; index < numLines; index ++) {
line(x1, y1, x2, y2);
y1 += yInc;
x2 += xInc;
}
}
/* draw the string pattern at the upper left */
function stringLeftUpper(r, g, b, t) {
// set vars to start at lower left and draw up
var numLines = 40;
var x1 = 0;
var y1 = height; // lower left
var y2 = 0;
var x2 = xInc = width/numLines;
var yInc = height/ numLines;
stroke(r, g, b, t);
// iterate over each line to draw
for (index = 0; index < numLines; index ++) {
line(x1, y1, x2, y2);
y1 -= yInc; // move up the canvas
x2 += xInc; // move across the canvas
}
}
/* draw the string pattern at the upper right */
function stringRightUpper(r, g, b, t) {
var numLines = 40;
var x1 = xInc = width/numLines;
var x2 = width;
var y1 = 0;
var y2 = 0;
var yInc = height/ numLines;
stroke(r, g, b, t);
// iterate over each line to draw
for (index = 0; index < numLines; index ++) {
line(x1, y1, x2, y2);
y2 += yInc; // move down the canvas
x1 += xInc; // move right across the canvas
}
}
/* draw the string pattern at the lower right */
function stringRightLower(r, g, b, t) {
// set variable
var numLines = 40;
var x1 = width; // right side
var x2 = 0;
var xInc = width/numLines;;
var yInc = height/numLines;
var y1 = height - yInc; // bottom right
var y2 = height;
stroke(r, g, b, t); // set color and transparency
// iterate over each line to draw
for (index = 0; index < numLines; index ++) {
line(x1, y1, x2, y2);
y1 -= yInc; // move up the canvas
x2 += xInc; // move right across the canvase
}
}
/* draw the center string circle */
function stringCircle(r, g, b) {
// 36 spokes on the circle design
var circlePoints = 36;
var angle = 0;
var rotDeg = 0;
// iterate over each spoke
for (index = 0; index < circlePoints; index++) {
// save settings
push();
// map the angle to the perimeter of the circle
angle = map(index, 0, circlePoints, 0, TWO_PI);
// convert angle to x y coordinates
var radius = 90;
var circleX = radius * cos(angle);
var circleY = radius * sin(angle);
// move origin to the starting point of the circle
translate(circleX, circleY);
// rotate each spoke to the origin
rotate(radians(rotDeg));
// variables for drawing string design
var circleX2 = -radius * 2;
var circleY2 = 0;
var smallCircleDiam = 10;
var offset = 15;
// draw small circles at end of spokes
stroke(r, g, b, 255);
circle(0, 0, smallCircleDiam * .2);
noFill();
circle(0, 0, smallCircleDiam); // outline
// set stroke color and decrease transparency to
// see more detail.
stroke(r, g, b, 125);
// draw three lines from each perimeter point to
// create spokes
line(0, 0, circleX2, circleY2);
line(0, 0, circleX2, circleY2 + offset);
line(0, 0, circleX2, circleY2 -offset);
// extend lines off of spokes
stroke(r, g, b, 50);
line(0, 0, offset * 8, circleY2);
line(0, 0, offset * 8, circleY2 + offset);
line(0, 0, offset * 8, circleY2 -offset);
// call function to draw the background circles with
// transparancey
backgroundCircles(index, offset, r, g, b, 80);
pop(); // restore settings
rotDeg += 10; // rotate 10 degrees 36/360
}
}
/* draw the background circles with design */
function backgroundCircles(index, offset, r, g, b, t) {
// save settings
push();
stroke(r, g, b, t); // light blue with transparency
// rest origin, space circles out
translate(25, 0);
// draw small inner circle on even spoke
if (index % 2 == 0) {
circle(0, 0, 20);
circle(110, 0, 70);
} else {
var diam = offset * 4; // set diameter
// draw bigger circle on odd spoke
circle(offset * 3, 0, diam);
// string design of four circles inside each
// bigger circle
var shiftValue = 10;
circle(offset * 3, -shiftValue, diam/2);
circle(offset * 3, shiftValue, diam/2);
circle(offset * 3 + shiftValue, 0, diam/2);
circle(offset * 3 - shiftValue, 0, diam/2);
}
pop();// restores settings
}
Project 4 – String Art
Clicking on the canvas allows the user to iterate through the 3 string art drawings, while holding the left mouse down shows all 3 of them overlaid on each other.
// Tsz Wing Clover Chau
// Section E
function setup() {
createCanvas(400, 300);
background(255);
}
var counter = 0;
var showCounter = 0;
var m = 1;
var n = 10;
var n2 = n*2;
function draw() {
noFill();
var cx = width/2;
var cy = height/2;
var dy1 = (height/n2)*m; // dimensions for drawing 1
var dx1 = (width/n2)*m;
var dx2 = width/(2*n); // dimensions for drawing 2
var dy2 = height/(6*n);
var dx3 = width/(6*n);
var dy3 = height/(2*n);
var size1 = min(width, height)*(4/5); // dimensions for drawing 3
var sx1 = width/5;
var sy1 = height/10;
var sx2 = width*4/5;
var sy2 = height*9/10;
var dx4 = size1/(2*n);
var dy4 = dx4;
var size2 = min(width, height)*(3/5);
var sx3 = (width-size2)/2;
var sy3 = (height-size2)/2;
var sx4 = width-sx3;
var sy4 = height - sy3
var dx5 = size2/(2*n);
var dy5 = dx5;
if (counter %3 == 0){ // drawing 1
background(255);
for (var i = 0; i <= n2; i++){
stroke(231, 111, 81);
line(dx1*i, 0, width, dy1*i);
stroke(233, 196, 106);
line(width, dy1*i, width-(dx1*i), height);
stroke(42, 157, 143);
line(width - (dx1*i), height, 0, height - (dy1*i));
stroke(38, 70, 83);
line(0, height-(dy1*i), dx1*i, 0);
}
} else if (counter % 3 == 1){ // drawing 2
background(255);
for (var j = 0; j <= n; j++){
stroke(38, 70, 83);
line(cx+(dx2*j), cy+(dy2*j), width*(2/3)-(dx3*j), dy3*j);
line(cx-(dx2*j), cy-(dy2*j), width*(2/3)-(dx3*j), dy3*j);
stroke(233, 196, 106);
line(cx-(dx2*j), cy-(dy2*j), (width/3)+(dx3*j), height-(dy3*j));
line(cx+(dx2*j), cy+(dy2*j), (width/3)+(dx3*j), height-(dy3*j));
}
} else { // drawing 3
background(255);
for (var k = 0; k < n; k++){
stroke(42, 157, 143);
line(cx-(dx5*k), cy+(dy5*k), sx3+(dx5*k), sy4);
line(cx-(dx5*k), cy+(dy5*k), sx3, sy4-(dy5*k));
line(cx+(dx5*k), cy-(dy5*k), sx4-(dx5*k), sy3);
line(cx+(dx5*k), cy-(dy5*k), sx4, sy3+(dy5*k));
line(cx, sy3, sx4, sy3);
line(sx4, sy3, sx4, cy);
line(cx, sy4, sx3, sy4);
line(sx3, cy, sx3, sy4);
stroke(244, 162, 97);
line(cx-(dx4*k), cy-(dy4*k), sx1+(dx4*k), sy1);
line(cx-(dx4*k), cy-(dy4*k), sx1, sy1+(dy4*k));
line(cx+(dx4*k), cy+(dy4*k), sx2, sy2-(dy4*k));
line(cx+(dx4*k), cy+(dy4*k), sx2-(dx4*k), sy2);
line(cx, sy1, sx1, sy1);
line(sx1, sy1, sx1, cy);
line(cx, sy2, sx2, sy2);
line(sx2, cy, sx2, sy2);
}
}
showAll();
if (showCounter > 15){ // all 3 drawings
background(255);
stroke(38, 70, 83);
for (var i = 0; i <= n2; i++){
line(dx1*i, 0, width, dy1*i);
line(width, dy1*i, width-(dx1*i), height);
line(width - (dx1*i), height, 0, height - (dy1*i));
line(0, height-(dy1*i), dx1*i, 0);
}
for (var k = 0; k <= n; k++){
stroke(142, 202, 230);
line(cx+(dx2*k), cy+(dy2*k), width*(2/3)-(dx3*k), dy3*k);
line(cx-(dx2*k), cy-(dy2*k), width*(2/3)-(dx3*k), dy3*k);
stroke(42, 157, 143);
line(cx-(dx2*k), cy-(dy2*k), (width/3)+(dx3*k), height-(dy3*k));
line(cx+(dx2*k), cy+(dy2*k), (width/3)+(dx3*k), height-(dy3*k));
stroke(233, 196, 106);
line(cx-(dx5*k), cy+(dy5*k), sx3+(dx5*k), sy4);
line(cx-(dx5*k), cy+(dy5*k), sx3, sy4-(dy5*k));
line(cx+(dx5*k), cy-(dy5*k), sx4-(dx5*k), sy3);
line(cx+(dx5*k), cy-(dy5*k), sx4, sy3+(dy5*k));
line(cx, sy3, sx4, sy3);
line(sx4, sy3, sx4, cy);
line(cx, sy4, sx3, sy4);
line(sx3, cy, sx3, sy4);
stroke(231, 111, 81);
line(cx-(dx4*k), cy-(dy4*k), sx1+(dx4*k), sy1);
line(cx-(dx4*k), cy-(dy4*k), sx1, sy1+(dy4*k));
line(cx+(dx4*k), cy+(dy4*k), sx2, sy2-(dy4*k));
line(cx+(dx4*k), cy+(dy4*k), sx2-(dx4*k), sy2);
line(cx, sy1, sx1, sy1);
line(sx1, sy1, sx1, cy);
line(cx, sy2, sx2, sy2);
line(sx2, cy, sx2, sy2);
}
}
}
function mouseClicked(){
counter ++;
}
function showAll(){
if (mouseIsPressed){
showCounter ++;
} else {
showCounter = 0;
}
}
Project 4: String Art
/*
* Andrew J Wang
* ajw2@andrew.cmu.edu
* Section A
*
* This program draws line arts
*/
var maxNum = 30;
function setup() {
createCanvas(400, 300);
background(0);
}
function draw() {
background(0);
//using the 3 functions for 3 different shapes
backgroundStrings (50);
stroke(255);
circleStrings(200,150,200,500);
stroke(255,0,0,255);
circleStrings(200,150,100,58);
push();
fill(255);
ellipse(200,150,100);
pop();
stroke(255,0,0,255);
hexagonStrings(200,150,100,5,10);
}
function circleStrings(Cx,Cy,Cd,Cl) {
for (var k = 0; k < maxNum; k++)
{
//grabing points on the circle
y=sin((k*(360/maxNum))*Math.PI/180)*(Cd/2)+Cy;
x=cos((k*(360/maxNum))*Math.PI/180)*(Cd/2)+Cx;
//making lines that is perpandicular to the radius with adjustable lengths
p1X = x + cos(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p1Y = y + sin(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p2X = x - cos(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
p2Y = y - sin(-(Math.PI/2-(k*(360/maxNum))*Math.PI/180))*Cl;
line (p2X,p2Y,p1X,p1Y);
}
}
function hexagonStrings(Hx,Hy,Hd,num,seg) {
//make arrays
const array = [];
var angle = 360/num;
for (var k = 0; k < num; k++)
{
array[k] = new Array();
y=sin(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)+Hy;
x=cos(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)+Hx;
line (Hx,Hy,x,y);
for (var s = 0; s<seg; s++)
{
//3D array grabing every points on the line of the star
array[k][s] = new Array();
var y1=sin(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)*s/seg+Hy;
var x1=cos(-Math.PI/2+(k*angle)*Math.PI/180)*(Hd/2)*s/seg+Hx;
array[k][s][0] = x1;
array[k][s][1] = y1;
}
}
//cross connecting those lines using for loop
for (var k = 0; k < num-1; k++)
{
for (var s = 0; s<seg; s++)
{
line(array[k][s][0],array[k][s][1],array[k+1][seg-1-s][0],array[k+1][seg-1-s][1]);
}
}
for (var s = 0; s<seg; s++)
{
line(array[num-1][s][0],array[num-1][s][1],array[0][seg-1-s][0],array[0][seg-1-s][1]);
}
}
function backgroundStrings (num) {
for (var k=0; k<num; k++)
{
//cross connecting with for loop
stroke(255/num*k,0,0,255);
line (k*width/num,0,width,k*height/num);
line (width-k*width/num,height,0,height-k*height/num);
line (k*width/num,height,width,height-k*height/num);
line (width-k*width/num,0,0,k*height/num);
}
}
Looking Outwards-04-Section B
Experiments in Musical Intelligence, EMI or EMMY, is a program that analyzes musical compositions and generates entirely new compositions that emulate the sound, style, mood, and emotion of the original. Written by composer, author, and scientist, David Cope, this project allows entirely new compositions to be algorithmically generated in the style of any composer. Compositions have been generated in the styles of Bach, Beethoven, Chopin, and many more, including Cope himself. In fact, Cope’s original inspiration for the software project was writer’s block. He was stuck and wanted to identify his own compositional style.
Although the software is data driven and bases its compositions on works by the original composer, it never repeats or copies the original work. The compositions generated are unique. Cope’s software deconstructs the original works; then records their time signatures. The final step runs the data through a recombinant algorithm for which Cope holds a patent.
This project was truly revolutionary for its time. It inspires questions about creativity and the mind. Originally written in the LISP programming language in the 1980s, it has been modified to use AI techniques as they have advanced. Interestingly, the generative compositions have been used in a type of Turing Test. One particular test set out to see if audiences could identify which of three compositions was actually composed by Bach, which was an emulated composition written by a human, and which was generated by a computer. Audiences chose the EMMY generated composition as the actual Bach. Perhaps EMMY is the first piece of software to pass the Turing Test.
To learn more about David Cope and EMMY click here and here.
Week 04 Blog – Brian Eno
Just as a song has a beginning, so too must it come to an end. Or so I thought before discovering the world of generative music several years ago. Popularized by musician Brian Eno in the late 70s, this genre defies norms by challenging the idea that a song or an album needs to end. Rather, this type of ambient music has the ability to generate and grow on its own, ever-expanding, yet unfortunately, there hasn’t been a way to release never-ending music to the public until recently. The project I wish to discuss today is the app that Eno released in 2016, called “Reflection”. “Reflection” is a culmination of all his work with computer-generated colored light displays and installation art, and most importantly, it has the capacity to play an endless loop of music that will never repeat itself. The app is designed by Eno and uses his secret programming methods to produce the music, as well as the visual light displays that he coded himself using a basic Arduino board. Eno considered himself more of a scientist than an artist and loves the accessibility that science offers, and tries to convey this accessibility in his music, which he approaches in a very physically intuitive manner, trying to isolate the things that “work” and continue to do them. In his project “Reflection” he uses code that randomizes the notes played, as well the delays, volume of notes, and rhythm of the piece. The code he creates also allows him to use a function to correct any notes that clash with the key. The code then generates the music and once it begins playing, Eno adds additional rules to form the music into how he likes it, though he never imagines the result beforehand.