siminl-project3
// Simin Li
// Section C
// siminl@andrew.cmu.edu
// Project 3
var sqrMin = 20;
var sqrMax = 180; //limitations to size of square
var vanishX = 320;
var vanishY = 240;
var X1 = 200;
var Y1 = 120;
var A1 = 20; //coordinates and length of top left large square
var X2 = 440;
var Y2 = 120;
var A2 = 20; //coordinates and length of top right large square
var X3 = 200;
var Y3 = 360;
var A3 = 20;//coordinates and length of bottom left large square
var X4 = 440;
var Y4 = 360;
var A4 = 20;//coordinates and length of bottom right large square
var light = 255; //control brightness of large suqares and background
function setup() {
createCanvas(640, 480);
}
function draw() {
colorMode(RGB);
background(255 - light);//changes the color of the background with respect to distance from
//vanishing points to center of canvas
rectMode(CENTER);
noCursor();//remove mouse
var from14 = color(255,255 ,0);//"Yellow"; first base color for squares 1 and 4
var to14 = color(65,105,225);//"RoyalBlue"; second base color for squares 1 and 4
var from23 = color( 0,191,255); //"Deepskyblue"; first base color for squares 2 and 3
var to23 = color(255,255 ,0);//"Yellow"; second base color for squares 2 and 3
A1 = map(dist(vanishX,vanishY,X1,Y1),0, dist(X1,Y1,640,480),sqrMin,sqrMax);
var a1 = A1 * 2 / 3; //length of small squares is always a third of the large squares
A2 = map(dist(vanishX,vanishY,X2,Y2),0, dist(X2,Y2,0,480),sqrMin,sqrMax);
var a2 = A2 * 2 / 3;
A3 = map(dist(vanishX,vanishY,X3,Y3),0, dist(X3,Y3,640,0),sqrMin,sqrMax);
var a3 = A3 * 2 / 3;
A4 = map(dist(vanishX,vanishY,X4,Y4),0, dist(X4,Y4,0,0),sqrMin,sqrMax);
var a4 = A4 * 2 / 3;
// length of sqaures is correlated to the distance from square center to farthest corner
light = map(dist(vanishX,vanishY,width / 2,height / 2),0, dist(width / 2,height / 2,0,0),0,255);
//changes the color of the background with respect to distance from vanishing points to center of canvas
fill(light);
rect(X1,Y1,A1,A1);
rect(X2,Y2,A2,A2);
rect(X3,Y3,A3,A3);
rect(X4,Y4,A4,A4); // draw large squares
vanishX = min( max(0,mouseX),640);
vanishY = min(max(0,mouseY), 480);//vanish point of one point perspective
var x1 = (2 * X1 + vanishX) / 3;
var y1 = ((vanishY - Y1) / (vanishX - X1)) * (x1 - X1) + Y1; //position of small squares are at
// a third of vanishing point and center of large square
var X1L = X1 - A1 / 2;
var X1R = X1 + A1 / 2;
var Y1T = Y1 - A1 / 2;
var Y1B = Y1 + A1 / 2;// coordinates of 4 corners of large squares(used later to draw lines)
var x1L = x1 - a1 / 2;
var x1R = x1 + a1 / 2;
var y1T = y1 - a1 / 2;
var y1B = y1 + a1 / 2; // coordinates of 4 corners of small squares
strokeWeight(4);
line(X1L, Y1T, x1L, y1T);//top left connect
line(X1R, Y1T, x1R, y1T);//top right connect
line(X1L, Y1B, x1L, y1B);//bottom left connect
line(X1R, Y1B, x1R, y1B);//bottom right connect
var x2 = (2 * X2 + vanishX) / 3;
var y2 = ((vanishY - Y2) / (vanishX - X2)) * (x2 - X2) + Y2;
var X2L = X2 - A2 / 2;
var X2R = X2 + A2 / 2;
var Y2T = Y2 - A2 / 2;
var Y2B = Y2 + A2 / 2;
var x2L = x2 - a2 / 2;
var x2R = x2 + a2 / 2;
var y2T = y2 - a2 / 2;
var y2B = y2 + a2 / 2;
strokeWeight(4);
line(X2L, Y2T, x2L, y2T);//top left connect
line(X2R, Y2T, x2R, y2T);//top right connect
line(X2L, Y2B, x2L, y2B);//bottom left connect
line(X2R, Y2B, x2R, y2B);//bottom right connect
var x3 = (2 * X3 + vanishX) / 3;
var y3 = ((vanishY - Y3) / (vanishX - X3)) * (x3 - X3) + Y3;
var X3L = X3 - A3 / 2;
var X3R = X3 + A3 / 2;
var Y3T = Y3 - A3 / 2;
var Y3B = Y3 + A3 / 2;
var x3L = x3 - a3 / 2;
var x3R = x3 + a3 / 2;
var y3T = y3 - a3 / 2;
var y3B = y3 + a3 / 2;
strokeWeight(4);
line(X3L, Y3T, x3L, y3T);//top left connect
line(X3R, Y3T, x3R, y3T);//top right connect
line(X3L, Y3B, x3L, y3B);//bottom left connect
line(X3R, Y3B, x3R, y3B);//bottom right connect
var x4 = (2 * X4 + vanishX) / 3;
var y4 = ((vanishY - Y4) / (vanishX - X4)) * (x4 - X4) + Y4;
var X4L = X4 - A4 / 2;
var X4R = X4 + A4 / 2;
var Y4T = Y4 - A4 / 2;
var Y4B = Y4 + A4 / 2;
var x4L = x4 - a4 / 2;
var x4R = x4 + a4 / 2;
var y4T = y4 - a4 / 2;
var y4B = y4 + a4 / 2;
strokeWeight(4);
line(X4L, Y4T, x4L, y4T);//top left connect
line(X4R, Y4T, x4R, y4T);//top right connect
line(X4L, Y4B, x4L, y4B);//bottom left connect
line(X4R, Y4B, x4R, y4B);//bottom right connect
colorMode(RGB);
inter1 = lerpColor(from14, to14,dist(vanishX,vanishY,X1,Y1) / dist(X1,Y1,height,width));// far right corner is(640,480)
inter4 = lerpColor(from14, to14,dist(vanishX,vanishY,X4,Y4) / dist(X4,Y4,0,0));// far left corner is(0,0)
inter2 = lerpColor(from23, to23,dist(vanishX,vanishY,X2,Y2) / dist(X2,Y2,0,height));//far left corner is(0,640)
inter3 = lerpColor(from23, to23,dist(vanishX,vanishY,X3,Y3),0, dist(X3,Y3,width,0)); //fills squares based
//their distance from their furthest corner(according to x, y position)
//far right corner is(640,0)
fill(inter1);
rect(x1,y1,a1,a1);
fill(inter4);
rect(x4,y4,a4,a4);
fill(inter2);
rect(x2,y2,a2,a2);
fill(inter3);
rect(x3,y3,a3,a3); //draw and fill small squares
push(); //draw rotating flower to replace cursor
translate(mouseX,mouseY);
scale(2, 1); //stretched horizontally to 2 times
rotate(millis() / 1000) ;
noStroke();
fill(255);
ellipse(0,0,20,4);
ellipse(0,0,4,20);//white petals
push();
rotate(PI/4.0);
ellipse(0,0,20,4);
ellipse(0,0,4,20);//white petals that are rotated 45 degrees
fill("Gold");
ellipse(0,0,5,5);//center
pop();
pop();
}
The idea for this project was inspired by learning about one point perspective, two point perspective and three point perspective images in drawing class. In one point perspective images all lines on the yz plane intersect at one point called the vanishing point. the rest of the lines are parallel. The vanishing point in this dynamic drawing is the flower and the boxes are leaves.
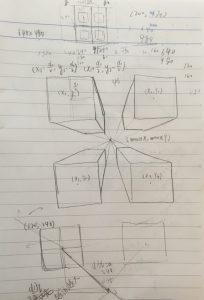
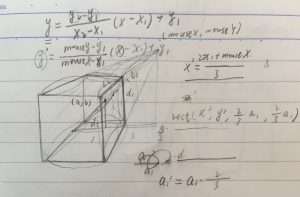