/*
Alice Fang
Section E
acfang@andrew.cmu.edu
Project-03
*/
/*
When mouseX is > width/2,
-magenta circle shrinks while cyan circle grows
-blue circle rotates clockwise, yellow circle couterclockwise
When mouseX is < width/2,
-magenta circle grows while cyan circle shrinks
-blue circle rotates counterclockwise, yellow circle clockwise
When mouseX is within the red circle
-green circle rotates clockwise
-green circle becomes smaller
-green circle rotates more quickly
-things become dark! goodbye light
Sometimes there is a delay with the growth/shrink of magenta and cyan circles-
neither I nor Prof. Dannenberg know exactly why 🙁 */
//circle diameters
var yellowD = 100;
var cyanD = 80;
var redD = 240;
var greenD = 80;
var magentaD = 320;
var blueD = 160;
var scale1 = 5; //constant rate of growth for cyan/magenta circles
var scale2 = -5; //constant rate of shrinking for cyan/magenta circles
//rotataion speed
var angle1 = 0; //blue circle
var angle2 = 0; //yellow circle
var angle3 = 0; //green circle (mouseX inside red circle)
var angle4 = 0; //green circle (mouseX outside red circle)
var angle5 = 0; //cyan circle
var angle6 = 0; //magenta circle
function setup() {
createCanvas(640, 480);
noStroke();
}
function draw() {
background(255);
if (dist(160, 280, mouseX, mouseY) < redD/2) {
background(0); //switch background to black
} else {
background(255);
}
var cyanS = constrain(cyanD, 40, 500); //limit growth of cyan
var magentaS = constrain(magentaD, 40, 500); //limit growth of magenta
if (mouseX > width / 2) {
cyanD += scale1; //cyan increases in size
magentaD += scale2; //magenta decreases in size
angle1 += 5; //blue rotates clockwise
angle2 -= 3; //yellow rotates counterclockwise
}
if (mouseX < width /2) {
cyanD += scale2; //cyan decrease in size
magentaD += scale1; //magenta increase in size
angle1 -= 3; //blue rotates counterclockwise, decreases speed
angle2 += 5; //yellow rotates clockwise, increases speed
}
//cyan circle
push();
translate(width/2, height/2);
rotate(radians(angle5));
angle5 -= 1; //rotation speed
fill('rgba(0, 255, 255, 0.5)');
ellipse(200, 160, cyanS, cyanS);
pop();
//magenta circle
push();
translate(width/2, height/2);
rotate(radians(angle6));
angle6 += 1; //rotation speed,
fill('rgba(255, 0, 255, 0.5)');
ellipse(80, 40, magentaS, magentaS);
pop();
//red circle
if (dist(160, 280, mouseX, mouseY) < redD/2) { //if mouse is inside red circle
fill('rgba(0, 0, 0, 0.7)'); //become black
ellipse(160, 280, redD, redD);
} else {
fill('rgba(255, 0, 0, 0.7)');
ellipse(160, 280, redD, redD);
}
//blue circle
push();
translate(360, 240);
rotate(radians(angle1));
ellipseMode(CORNER);
fill('rgba(0, 0, 255, 0.5)');
ellipse(0, 0, blueD, blueD);
pop();
//yellow circle
push();
translate(360, 240);
rotate(radians(angle2));
ellipseMode(CORNER);
fill('rgba(255, 255, 0, 0.7)');
ellipse(80, 80, yellowD, yellowD);
pop();
//green circle
if (dist(160, 280, mouseX, mouseY) < redD/2) { //if mouse is inside red circle
push();
translate(160, 280);
rotate(radians(angle3));
angle3 += 8; //rotation speed, clockwise
fill('rgba(0, 255, 0, 0.7)');
ellipse(180, 0, greenD/2, greenD/2);
pop();
} else {
push();
translate(160, 280);
rotate(radians(angle4));
angle4 += -1; //rotation speed, counterclockwise
fill('rgba(0, 255, 0, 0.7)');
ellipse(180, 0, greenD, greenD);
pop();
}
textSize(20);
fill(255);
if (dist(160, 280, mouseX, mouseY) < redD/2) { //if mouse is inside red circle
text("goodbye!", mouseX, mouseY);
} else {
text("hello!", mouseX, mouseY);
}
}
For this project, I was inspired by the RGB overlay. Originally, I wanted to set a condition where if two circles overlapped, the intersection would fill white, as would happen if you overlay-ed real RGB values. In the end, I decided to create something that was bright and bubbly, playing with opacity and color.
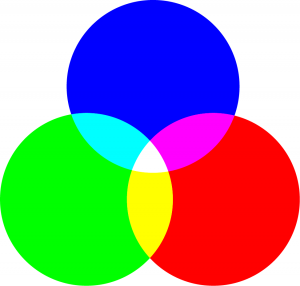
Also, a quick note. I sort of mentioned this in the comments, but nothing is random. Everything is dependent on the position of mouseX relative to the canvas (width/2), or to the red circle. The growth of the cyan and magenta circles lag a little, but will respond.