/*
Yingying Yan
Section E
yingyiny@andrew.cmu.edu
Project - 10
*/
var snowman = [];
function setup() {
createCanvas(480, 240);
for (var i = 0; i < 4; i++) {
var rx = random(width);
snowman[i] = makeSnowman(rx);
}
frameRate(10);
}
function draw() {
background("green");
//background
displayHorizon();
//snowman
updateAndDisplaySnowman();
removeSnowmanThatHaveSlippedOutOfView();
addNewSnowmanWithSomeRandomProbability();
}
function updateAndDisplaySnowman() {
for(var i = 0; i < snowman.length; i++) {
snowman[i].move();
snowman[i].display();
}
}
function removeSnowmanThatHaveSlippedOutOfView() {
var snowmanKeep = [];
for (var i = 0; i < snowman.length; i++) {
if(snowman[i].x + 50 > 0) {
snowmanKeep.push(snowman[i]);
}
}
snowman = snowmanKeep;
}
function addNewSnowmanWithSomeRandomProbability() {
var newSnowmanPercent = 0.006
if (random(0,1) < newSnowmanPercent) {
snowman.push(makeSnowman(width))
}
}
//move towards the left
function snowmanMove() {
this.x += this.speed;
}
//function that draws the snowman
function snowmanDisplay() {
push();
fill(255);
noStroke();
var sizeBottom = 35;
var sizeMiddle = 25;
var sizeTop = 20;
var yy = height-35;
//translate(this.x, height - 35);
translate(this.x, 0);
//bottom circle
ellipse(0, yy - sizeBottom / 2, sizeBottom, sizeBottom);
//middle circle
ellipse(0, yy - sizeBottom - sizeMiddle / 2 +5 , sizeMiddle, sizeMiddle);
// //top circle
// ellipse(0, yy - sizeBottom - sizeMiddle - sizeTop / 2 + 10, sizeTop, sizeTop);
push();
fill(0);
ellipse(0 - 5, yy - sizeBottom - sizeMiddle / 2 + 2, 2, 2);
ellipse(0 + 5, yy - sizeBottom - sizeMiddle / 2 + 2, 2, 2);
noFill();
stroke(0)
ellipse(0, yy - sizeBottom - sizeMiddle / 2 + 5, 4, 4);
line(15, yy - sizeBottom / 2, 30, yy - 40);
line(-15, yy - sizeBottom / 2, -30, yy - 40);
pop();
pop();
}
//define all the objects and variables
function makeSnowman(positionOg) {
var sman = {
x: positionOg,
//y: 380,
speed: -1.0,
move: snowmanMove,
display: snowmanDisplay
}
return sman;
}
//background
function displayHorizon() {
fill("lightblue");
rect(-1,-1, width + 1, height - 39);
}
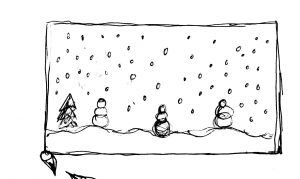
I wanted to render some snow scene because I love the snow. Unfortunately, I can barely finish the project. But I have a snowman! I mean lots of snowmen. I think this project is hard and really makes me think about “object”. I am still in the process of understanding the code. I think for my final project if I would do something similar, it will be much better than this.