//Lan Wei
//Section D
//lanw@andrew.cmu.edu
//Project-03
var starXArray = [];
var starYArray = [];
var starRArray = [];
function setup() {
createCanvas(450, 450);
noStroke();
for (var i = 0; i < 200; i ++){
var starR = random(0.5, 2);
var starX = random(0, 2 * width);
var starY = random(0, 2 * height/3);
ellipse(starX, starY, starR, starR); // stars
starXArray.push(starX);
starYArray.push(starY); //store the positions of the stars in arrays
starRArray.push(starR); //store the size of the stars
print(starXArray);
}
}
function draw() {
background(5, 5, 32);
fill(136, 3, 11);
rect(0, 2 * height/3, width, height/3); //lower part of the city scene
//stars
for (var i = 0; i < 200; i ++){
fill(150);
ellipse(starXArray[i] - mouseX/7, starYArray[i], starRArray[i], starRArray[i]);
}
// buildings
push();
fill(136, 3, 11);
rect(-mouseX/2, 200, 50, 150);
rect(50-mouseX/2, 270, 30, 150);
rect(80-mouseX/2, 230, 70, 150);
rect(150-mouseX/2, 280, 90, 150);
rect(240-mouseX/2, 250, 50, 150);
rect(290-mouseX/2, 190, 50, 150);
rect(340-mouseX/2, 230, 20, 150);
rect(400-mouseX/2, 200, 50, 150);
rect(450-mouseX/2, 270, 30, 150);
rect(480-mouseX/2, 230, 70, 150);
rect(550-mouseX/2, 280, 90, 150);
rect(640-mouseX/2, 250, 50, 150);
rect(690-mouseX/2, 190, 50, 150);
rect(740-mouseX/2, 230, 20, 150);
pop();
//roller coaster
for (var x = 0; x < 5 * width/8; x += 0.1){ // the less thriller part
var xInSin = map(x, 0, width, 0, 4 * PI);
var y = 50 * sin(xInSin) + height/2 + 50;
fill(255);
ellipse (x, y, 1, 1);
if (x % 20 <= 0.1){ //vertical structure
push();
stroke(200);
strokeWeight(1);
line(x, height, x, y);
pop();
}
}
for (var x = 5 * width/8; x < width; x += 0.1){ //the thriller part
var xInSin = map(x, 0, width, 0, 4 * PI);
var y = 100 * sin(xInSin) + height/2;
ellipse (x, y, 1, 1);
if (x % 20 <= 0.1){ //vertical structure
push();
stroke(200);
strokeWeight(1);
line(x, height, x, y);
pop();
}
}
//carriage
if (mouseX < 5 * width/8){
y = 50 * sin(xInSin) + height/2 + 50;
fill(255, 255, 0);
var fakeX = map(mouseX, 0, width, 0, 4 * PI);
var rectY = 50 * sin(fakeX) + height/2 + 50
push();
rectMode(CENTER);
var ang = asin(sin(fakeX + PI/2));
translate(mouseX, rectY);
rotate(ang);
rect(0, 0, 20, 10);
pop();
}
if (mouseX >= 5 * width/8){
y = 100 * sin(xInSin) + height/2;
fill(255, 255, 0);
var fakeX = map(mouseX, 0, width, 0, 4 * PI);
var rectY = 100 * sin(fakeX) + height/2;
push();
rectMode(CENTER);
var ang = asin(sin(fakeX + PI/2));
translate(mouseX, rectY);
rotate(ang);
rect(0, 0, 20, 10);
pop();
}
}
I trird to make a scene of roller coaster at night, with the stars in the sky and buildings far away. The stars, buildings and roller coaster should move with different speeds (and probably different directions) with mouse moving. The hardest part is to make the roller coaster move along its track. The process is very fun.
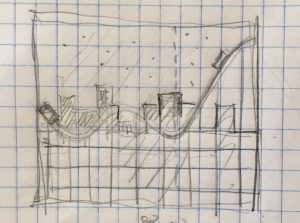