Category: Project-11-Composition
Yiran Xuan – Project 11 – Composition
This sketch is based off of my favorite drawing tool in FireAlpaca, the Mirror Brush. It initializes two different turtles that move to the mouse’s position when the mouse is clicked. Buttons on the keyboard can affect the pen’s path; pressing ‘p’ will toggle the penUp and penDown, ‘w’ and ‘t’ will widen and thin the pen tracks respectively, while ‘r’, ‘b’, ‘g’, ‘l’ change the color of the tracks.
(Completed with 2-day extension)
/*
Yiran Xuan
Section A
yxuan@andrew.cmu.edu
Project 11
*/
//Goal: Create mirroring paint tool that uses the turtle graphics
var brushturtle; //turtle that corresponds to mouse movements
var mirrorturtle; //turtle that mirrors brushturtle
var targetx = 0; //x coord of where brush turtle should go
var targety = 0; //y coord of both turtles
var mirrorx = -targetx;
var brushweight = 5;
function setup(){
createCanvas(480, 480);
strokeWeight(6);
translate(240, 0);
brushturtle = makeTurtle(0, 0); //initialize turtles
mirrorturtle = makeTurtle(0,0);
brushturtle.setColor('black');
mirrorturtle.setColor('black');
mirrorturtle.setWeight(brushweight);
}
function draw(){
translate(240, 0); //needs to be constantly retranslated so that 0 line is down the middle of canvas
brushturtle.setWeight(brushweight);
mirrorturtle.setWeight(brushweight);
brushturtle.goto(targetx, targety); //transport brush
mirrorturtle.goto(mirrorx, targety); //transport mirror
}
function mouseClicked() {
targetx = mouseX - 240; //mouse coords ignores translate, so needs compensation
targety = mouseY;
mirrorx = -targetx;
}
function keyPressed(){
if(key == 'p'){ //pressing 'p' switches between pen position
penUporDown();
}
if(key == 'w'){
penWider();
}
if(key == 't'){
penThinner();
}
if(key == 'r'){ //changing pen colors
brushturtle.setColor('red');
mirrorturtle.setColor('red');
}
if(key == 'g'){
brushturtle.setColor('green');
mirrorturtle.setColor('green');
}
if(key == 'b'){
brushturtle.setColor('blue');
mirrorturtle.setColor('blue');
}
if(key == 'l'){
brushturtle.setColor('black');
mirrorturtle.setColor('black');
}
}
function penUporDown(){ //lifts or puts down pen
if(brushturtle.penIsDown){
brushturtle.penUp();
mirrorturtle.penUp();
}
else if(brushturtle.penIsDown == false){
brushturtle.penDown();
mirrorturtle.penDown();
}
}
function penWider(){ //widens penstroke
brushweight+= 0.5;
}
function penThinner(){ //thins penstroke
if(brushweight > 1){
brushweight -= 0.5;
}
}
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft,
right: turtleRight,
forward: turtleForward,
back: turtleBack,
penDown: turtlePenDown,
penUp: turtlePenUp,
goto: turtleGoTo,
angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo,
angleTo: turtleAngleTo,
setColor: turtleSetColor,
setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
Robert Oh- Project 11- Composition
//Robert Oh
//Section C
//rhoh@andrew.cmu.edu
//Project-11-Composition
var turtle;
var fx = 240;
var fy = 240;
var diffX = 100;
var diffY = 100;
function setup() {
createCanvas(480, 480);
frameRate(30);
noStroke();
}
function draw() {
background(130, 255, 246);
fishDisplay(fx, fy);
diffX = mouseX - fx;
diffY = mouseY - fy;
if (diffX > 1 || diffX < -1){
fx = fx + (diffX)/30;
}
if (diffY > 1 || diffY < -1){
fy = fy + (diffY)/30
}
}
// drawing each fish
function fishDisplay(x, y) {
turtle = makeTurtle(x, y);
turtle.penDown();
turtle.face(270);
turtle.setColor(100);
turtle.setWeight(3);
//body
for (var i = 0; i < 360; i ++){
turtle.forward(.7);
turtle.right(1);
}
turtle.face(0);
turtle.penUp();
turtle.forward(80);
//tail
turtle.penDown();
turtle.face(30);
turtle.forward(40);
turtle.face(270);
turtle.forward(40);
turtle.face(150);
turtle.forward(40);
turtle.penUp()
turtle.face(180);
turtle.forward(60);
turtle.face(270);
turtle.forward(25);
//eye
turtle.penDown();
turtle.face(0);
for (var i = 0; i < 180; i ++){
turtle.forward(.2);
turtle.right(2);
}
}
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
For this project, I went back to data exam 1 once again and borrowed the fish design we had drawn. I wanted to revisit the data exam and had the fish follow the mouse cursor. Overall, I had a lot of fun recreating the fish (but with turtle-style drawing).
Victoria Reiter – Project 11 – Composition
/*
Victoria Reiter
Section B
vreiter@andrew.cmu.edu
Assignment-10-b
*/
// makes turtle
var turtle;
// angle for the pretty plant design thing!
var chooseAngle = 200;
//makes plus shape
function plus() {
turtle.penDown();
turtle.forward(5);
turtle.left(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
turtle.left(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
turtle.left(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
turtle.left(90);
turtle.forward(5);
turtle.right(90);
turtle.forward(5);
}
function setup() {
createCanvas(600, 400);
background(80, 0, 255);
// makes turtle at center of canvas
turtle = makeTurtle(width / 2, height / 2);
turtle.setColor(color(202, 255, 112, 80));
turtle.setWeight(.25);
turtle.penUp();
}
function draw() {
plus(20, 30);
for (var i = 0; i < 100; i++) {
var distt = mouseX / 7;
turtle.penUp();
turtle.forward(distt);
plus();
turtle.penUp();
// returns turtle to center so it progressively moves greater distances
turtle.back(distt);
// rotates by golden angle
turtle.left(chooseAngle);
}
}
// --------------------------------
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
///GRACE DAY TAKEN///
For this week’s project I took inspiration from the “golden angle” and natural angles of plants and things in nature from last week. I wanted to know what it would be like if I manipulated it to work through an angle of my choosing, and other aspects of my choosing, such as a plus shape instead of hexagons. I made the stroke weight very fine and the opacity more sheer to add added effects. I also introduced animation by incorporating variation with mouseX.
I think this design is interesting because as you move the mouse, it looks as though there is a flower itself in the middle rotating around and around, but really it’s just the shapes building on themselves. How interesting!
((This is unrelated but I went to the Children’s Museum today and saw the Letter Rain mentioned in the Deliverables for the week and that wE. cOdED. so I thought that was cool! Here’s a quick picture just for fun hehehe))
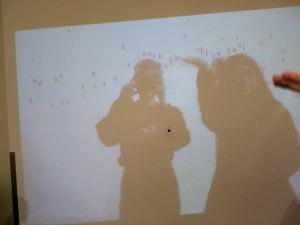
Yingying Yan – Project 11 Composition
/*
Yingying Yan
Section E
yingyiny@andrew.cmu.edu
Project-11
*/
//make an array so i can draw more than one flower
var ttl = [2,2,2,2,2,2,2,2,2,2,2,2,2,2,2];
function setup() {
createCanvas(480, 240);
background(0);
frameRate(10);
//set up the make turtle
for(var i = 0; i < ttl.length; i++) {
ttl[i] = makeTurtle(random(width), random(height));
}
}
function draw() {
for(var i = 0; i < ttl.length; i++) {
ttl[i].setWeight = (random(0.1,5));
ttl[i].setColor = (random(150), random(200), random(255));
//start drawing the flowers
ttl[i].penDown();
var dd = 4
ttl[i].forward (dd);
ttl[i].right(dd);
ttl[i].right(dd);
ttl[i].forward(dd);
ttl[i].left(dd)
ttl[i].forward(dd * i);
ttl[i].left(150);
}
}
//restart the drawing
function mousePressed(){
background(0)
}
//////////////////////////////////////////////////////////////
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
Xiaoying Meng – Project 11 Composition
click to change colors
//Xiaoying Meng
//B
//xiaoyinm@andrew.cmu.edu
//Project 11
var myTurtle;
var R = [255,200,150,100,50,0];
var G = [255,200,150,100,50,0];
var B = [0,50,100,150,200,255];
var i=0;
function triangles(){
for (var i = 0; i < 3; i++){
myTurtle.penDown();
myTurtle.forward(5);
myTurtle.left(120);
}
}
function setup() {
createCanvas(480, 400);
background(0);
}
function mousePressed(){
//change colors
i = i+1;
if (i===5){
i=0;
}
}
function draw() {
myTurtle = makeTurtle(480-mouseX,400-mouseY);
myTurtle.setColor(color(R[i],G[i],B[i]));
myTurtle.penUp();
for (var x=0; x< 100; x++){
myTurtle.penUp;
//go out
var dist = x*2;
myTurtle.forward(dist);
//draw
triangles();
//go back
myTurtle.penUp();
myTurtle.left(180);
myTurtle.forward(dist);
myTurtle.left(180);
//rotate angle based on location of mouseX
var a =180*(3-sqrt(5))-mouseX/100;
myTurtle.left(a);
}
}
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
I wanted to create something interactive with the turtles. Allowing the users to draw with them. But to add some interesting factors, I made the turtle go the opposite direction of the mouse and the “pen” rotate according to mouseX.
Katherine Hua – Project 11 – Composition
/* Katherine Hua
Section A
khua@andrew.cmu.edu
Project-11-Composition */
//global variables
var ttl1;
var ttl2;
var ttl3;
var ttl4;
var ttl5;
function setup() {
createCanvas(480, 480);
background(35);
//starting positions of turtle
ttl1 = makeTurtle(267, 302);
ttl2 = makeTurtle(260, 280);
ttl3 = makeTurtle(280, 407);
ttl4 = makeTurtle(267, 277);
ttl5 = makeTurtle(278, 360);
strokeCap(PROJECT);
strokeJoin(MITER);
frameRate(1);
}
function draw() {
//turtle 5
ttl5.setColor('skyblue');
ttl5.setWeight(1);
for (var n = 0; n < 10; n ++) {
ttl5.forward(30);
ttl5.left(55);
ttl5.forward(50);
ttl5.right(15);
if (n % 9 === 0) {
ttl5.forward(3);
}
}
//turtle 1
ttl1.setColor('pink');
ttl1.setWeight(1);
for (var i = 0; i < 20; i ++) {
ttl1.forward(70);
ttl1.left(120);
ttl1.forward(100);
ttl1.right(10);
}
//turtle 3
ttl3.setColor('yellow');
ttl3.setWeight(1);
for (var k = 0; k < 10; k ++) {
ttl3.forward(50);
ttl3.left(55);
ttl3.forward(60);
ttl3.right(15);
if (k % 9 === 0) {
ttl3.forward(5);
}
}
// turtle 4
ttl4.setColor('orange', 20);
ttl4.setWeight(1);
for (var m = 0; m < 10; m ++) {
ttl4.forward(20);
ttl4.left(110);
ttl4.forward(50);
ttl4.right(15);
}
//turtle 2
ttl2.setColor('skyblue');
ttl2.setWeight(1);
for (var j = 0; j < 10; j ++) {
ttl2.forward(1);
ttl2.left(55);
ttl2.forward(10);
ttl2.right(15);
if (j % 9 === 0) {
ttl2.forward(5);
}
}
}
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
At first I wanted to create a sunflower through this, but in the end I got carried away and began adding more and more layers to the design.
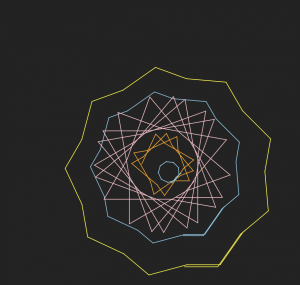
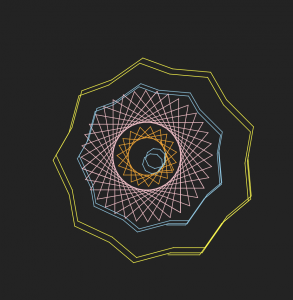
Yingyang Zhou-Project-11-Composition
//Yingyang Zhou
//yingyanz@andrew.cmu.edu
//Project 11
//section A
var turtle;
var treeX = 300;
var treeY = 400;
function setup() {
createCanvas(600, 400);
background(224, 216, 208);
frameRate(1);
}
function draw(){
turtle = makeTurtle(treeX, treeY);
turtle.setColor(color(84, 60, 43));
turtle.setWeight(7);
turtle.penUp();
var trunkRandomDegree = random(5);
var trunkRandomHeight = random(minTrunk,maxTrunk);
turtle.penDown();
turtle.left(90+trunkRandomDegree);
turtle.forward(trunkRandomHeight);
turtle.right(90+trunkRandomDegree);
turtle.penUp();
noLoop();
turtle.setColor(color(119, 166+random(-20,20), 119));
branch(turtle, floor(random(1, 5)), 10, 3, random(5), random(5));
}
var height = 400;
var minTrunk = height/4;
var maxTrunk = height/3;
var minBranch = height/10;
var maxBranch = height/3;
var minDegree = 3;
var maxDegree = 45;
function branch(turtle, number, length, width, left, right){
var number = floor(random(1, 5));
var length = 7;
var width = 0.5;
for(var i = 0; i < number; i++){
turtle.penDown();
turtle.left(90+random(minDegree,maxDegree));
turtle.forward(length);
branch(turtle, number/i, length, width, random(5), random(5));
length = length/i;
width = width/i;
}
turtle.penDown();
turtle.left(90+trunkRandomDegree);
turtle.forward(-trunkRandomHeight/2);
turtle.penDown();
turtle.left(random(minDegree))
turtle.left(random(minDegree,maxDegree));
turtle.forward();
for(var i = 0; i < 6; i++){
turtle.penDown();
turtle.forward(5);
turtle.right(60);
}
turtle.penUp();
}
function mousePressed(){
treeX = mouseX;
treeY = mouseY;
// setup();
draw();
}
///////////////////////////////////////////////////////////////////////
function turtleLeft(d){this.angle-=d;}function turtleRight(d){this.angle+=d;}
function turtleForward(p){var rad=radians(this.angle);var newx=this.x+cos(rad)*p;
var newy=this.y+sin(rad)*p;this.goto(newx,newy);}function turtleBack(p){
this.forward(-p);}function turtlePenDown(){this.penIsDown=true;}
function turtlePenUp(){this.penIsDown = false;}function turtleGoTo(x,y){
if(this.penIsDown){stroke(this.color);strokeWeight(this.weight);
line(this.x,this.y,x,y);}this.x = x;this.y = y;}function turtleDistTo(x,y){
return sqrt(sq(this.x-x)+sq(this.y-y));}function turtleAngleTo(x,y){
var absAngle=degrees(atan2(y-this.y,x-this.x));
var angle=((absAngle-this.angle)+360)%360.0;return angle;}
function turtleTurnToward(x,y,d){var angle = this.angleTo(x,y);if(angle< 180){
this.angle+=d;}else{this.angle-=d;}}function turtleSetColor(c){this.color=c;}
function turtleSetWeight(w){this.weight=w;}function turtleFace(angle){
this.angle = angle;}function makeTurtle(tx,ty){var turtle={x:tx,y:ty,
angle:0.0,penIsDown:true,color:color(128),weight:1,left:turtleLeft,
right:turtleRight,forward:turtleForward, back:turtleBack,penDown:turtlePenDown,
penUp:turtlePenUp,goto:turtleGoTo, angleto:turtleAngleTo,
turnToward:turtleTurnToward,distanceTo:turtleDistTo, angleTo:turtleAngleTo,
setColor:turtleSetColor, setWeight:turtleSetWeight,face:turtleFace};
return turtle;}
I enjoyed doing this project and the randomness turned out a little surprising but I like it! The idea is when you click on the canvas, there would be a tree grow from where you clicked.
Romi Jin – Project-11-Composition
/*
Romi Jin
Section B
rsjin@andrew.cmu.edu
Project-11
*/
function setup() {
createCanvas(480, 480);
background("black");
}
function draw() {
var myTurtle = makeTurtle(width/2, height/2);
myTurtle.penDown();
myTurtle.setColor("white");
myTurtle.setWeight(0.05);
strokeJoin(MITER);
strokeCap(PROJECT);
var step = 1; //different patterns
for (i = 0; i <= 150; i++) {
//flower drawing
myTurtle.forward(step);
myTurtle.left(step);
myTurtle.forward(step);
myTurtle.left(step);
myTurtle.forward(step);
myTurtle.left(step);
myTurtle.forward(step);
myTurtle.left(step);
//angle
myTurtle.right(200);
//spacing
myTurtle.forward(i*3);
}
}
/////////////////////////////////////////////////////////////
//turtle code
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
For this project, I decided to play around with angles and lines and see what kind of shapes I could make with different patterns and sizing. I eventually ended up with a flower / pinwheel, drawn spiraling out to a certain angle. It was interesting to see the difference between using turtle graphics to draw this versus not using it.
Jessica Timczyk – Project 11
// Jessica Timczyk
// Section D
// jtimczyk@andrew.cmu.edu
// Project-
var turtle
function setup() {
createCanvas(480, 480);
background(0);
frameRate(10);
}
function draw() {
turtle = makeTurtle(mouseX, mouseY); // make turtle variables
angle = turtle.angleTo(mouseX, mouseY);
turtle.right(angle);
turtle.forward(40);
turtle.setWeight(6) // setting the specifications for the turtles lines
strokeJoin(MITER);
strokeCap(PROJECT);
turtle.setColor(random(255)); //random colors
turtle.penDown(); // make swirl shape
turtle.forward(3);
turtle.left(90);
turtle.forward(50);
turtle.right(90);
turtle.forward(50);
turtle.right(90);
turtle.forward(37.5);
turtle.right(90);
turtle.forward(25);
turtle.right(90);
turtle.forward(12.5);
turtle.right(90);
turtle.forward(12.5);
turtle.left(90);
turtle.forward(12.5);
turtle.left(90);
turtle.forward(25);
turtle.left(90);
turtle.forward(37.5);
turtle.left(90);
turtle.forward(47);
turtle.goto(mouseX,mouseY);
}
function turtleLeft(d) {
this.angle -= d;
}
function turtleRight(d) {
this.angle += d;
}
function turtleForward(p) {
var rad = radians(this.angle);
var newx = this.x + cos(rad) * p;
var newy = this.y + sin(rad) * p;
this.goto(newx, newy);
}
function turtleBack(p) {
this.forward(-p);
}
function turtlePenDown() {
this.penIsDown = true;
}
function turtlePenUp() {
this.penIsDown = false;
}
function turtleGoTo(x, y) {
if (this.penIsDown) {
stroke(this.color);
strokeWeight(this.weight);
line(this.x, this.y, x, y);
}
this.x = x;
this.y = y;
}
function turtleDistTo(x, y) {
return sqrt(sq(this.x - x) + sq(this.y - y));
}
function turtleAngleTo(x, y) {
var absAngle = degrees(atan2(y - this.y, x - this.x));
var angle = ((absAngle - this.angle) + 360) % 360.0;
return angle;
}
function turtleTurnToward(x, y, d) {
var angle = this.angleTo(x, y);
if (angle < 180) {
this.angle += d;
} else {
this.angle -= d;
}
}
function turtleSetColor(c) {
this.color = c;
}
function turtleSetWeight(w) {
this.weight = w;
}
function turtleFace(angle) {
this.angle = angle;
}
function makeTurtle(tx, ty) {
var turtle = {x: tx, y: ty,
angle: 0.0,
penIsDown: true,
color: color(128),
weight: 1,
left: turtleLeft, right: turtleRight,
forward: turtleForward, back: turtleBack,
penDown: turtlePenDown, penUp: turtlePenUp,
goto: turtleGoTo, angleto: turtleAngleTo,
turnToward: turtleTurnToward,
distanceTo: turtleDistTo, angleTo: turtleAngleTo,
setColor: turtleSetColor, setWeight: turtleSetWeight,
face: turtleFace};
return turtle;
}
I really enjoyed doing this project. I had originally intended for the swirls to make a continuous line across the screen but after multiple tries I couldn’t seem to get them to follow each other. I think using turtle to create visuals is very fun and makes it a lot easier because it allows me to create individual shapes and patterns, like these swirls that can be moved all together without having to individually put in each value. next time I would like to try to figure out to create these patterns but have them be all connected with each other in a nice line.