/*
Victoria Reiter
Section B
vreiter@andrew.cmu.edu
Project-06-Abstract Clocks
*/
function setup() {
createCanvas(480, 260);
// declares variables later used for time
var h;
var m;
var s;
// declares value for opacity
var alphaValue;
}
function draw() {
// describes appearance of text
textFont('Colonna MT');
textAlign(CENTER);
// sets background color
background(209, 252, 235);
// variables for time
h = hour();
m = minute();
s = second();
// sizes for the images that will represent time aspects
var hourShapeWidth = width / 36;
var minuteShapeWidth = width / 200;
var secondShapeWidth = width / 170;
// makes a loop to count the hours, drawing forks, highlighting
// the fork that represents the current hour
for (var i = 0; i < 24; i++) {
if (i !== h) {
fill(25, 125, 75);
fork(i * (hourShapeWidth + 6) + 7, 65);
} else {
fill(255, 255, 0);
fork(i * (hourShapeWidth + 6) + 7, 65);
}
}
// makes a loop to count the minutes, drawing dining glasses
// and highlighting the glass that represents the current minute
var glassX = k * (minuteShapeWidth + 5.5) + 6
var glassY = height / 20;
angle = 0;
increment = 5;
for (var k = 0; k < 60; k++) {
if (k !== m) {
fill(150, 150, 255);
glass(k * (minuteShapeWidth + 5.5) + 6, 35);
} else {
fill(255, 255, 0);
glass(k * (minuteShapeWidth + 5.5) + 6, 35);
}
}
// makes a loop to count the seconds, drawing spoons and highlighting
// the spoon that represents the current minute
for (var j = 0; j < 60; j++) {
if (j !== s) {
fill(150, 155, 155);
spoon(j * (secondShapeWidth + 5) + 5, 15);
} else {
fill(255, 255, 0);
spoon(j * (secondShapeWidth + 5) + 5, 15);
}
}
// lines that divide the clock into representative "time zones" for meals
stroke(203, 158, 255, 150);
line(width / 4 + 1, 0, width / 4 + 1, height);
line(width / 2 - 3, 0, width / 2 - 3, height);
line(3 * width / 4 - 7, 0, 3 * width / 4 - 7, height);
// if the time is between midnight and 6am, it is breakfast time
if (h >= 0 & h < 6) {
noStroke();
alphaValue = 255;
fill(226, 140, 59, alphaValue);
textSize(17);
textStyle(BOLD);
text("Breakfast Time!", 60, 230);
scale(.5);
breakfast(57, 255);
} else {
noStroke();
alphaValue = 110;
fill(226, 140, 59, alphaValue);
textSize(17);
textStyle(NORMAL);
text("Breakfast Time!", 60, 230);
scale(.5);
breakfast(57, 255);
}
// if the time is between 6am and noon, it is lunch time
if (h >= 6 & h < 12) {
alphaValue = 255;
fill(48, 155, 24, alphaValue);
textSize(40);
textStyle(BOLD);
text("Lunch Time!", 357, 462);
scale(1.2, 1.5);
lunch(250, 180);
} else {
alphaValue = 110;
fill(48, 155, 24, alphaValue);
textSize(40);
textStyle(NORMAL);
text("Lunch Time!", 357, 462);
scale(1.2, 1.5);
lunch(250, 180);
}
// if the time is between noon and 6pm, it is dinner time
if (h >= 12 & h < 18) {
alphaValue = 255;
fill(204, 89, 71, alphaValue);
textStyle(BOLD);
textSize(30);
text("Dinner Time!", 495, 310);
scale(.8);
dinner(550, 225);
} else {
alphaValue = 110;
fill(204, 89, 71, alphaValue);
textSize(30);
textStyle(NORMAL);
text("Dinner Time!", 495, 310);
scale(.8);
dinner(550, 225);
}
// if the time is between 6pm and midnight, it is time for dessert !!
if (h >= 18 & h < 24) {
alphaValue = 255;
fill(224, 0, 160, alphaValue);
textStyle(BOLD);
text("Time for Dessert!!!", 870, 387);
scale(.575, .5);
dessert(1500, 468);
} else {
alphaValue = 110;
fill(224, 0, 160, alphaValue);
textStyle(NORMAL);
text("Time for Dessert!!!", 870, 387);
scale(.575, .5);
dessert(1500, 468);
}
}
// draws a spoon
function spoon(x, y) {
ellipse(x, y, 6, 8);
rect(x - 1, y, 2, 12);
}
// draws a drinking glass
function glass(x, y) {
arc(x, y, 8, 8, - 3 * PI / 16, PI + 3 * PI / 16, CHORD);
rect(x - 1, y, 2, 10);
rect(x - 2, y + 10, 5, 1);
}
// draws a fork
function fork(x, y) {
rect(x, y, 16, 5);
rect(x, y - 10, 2, 10);
rect(x + 5, y - 10, 2, 10);
rect(x + 10, y - 10, 2, 10);
rect(x + 14, y - 10, 2, 10);
triangle(x, y + 5, x + 16, y + 5, x + 8, y + 10);
rect(x + 6, y + 7, 4, 15);
}
// draws breakfast foods
function breakfast(x, y) {
eggs(x, y);
bacon(x + 75, y - 50);
}
// draws lunch foods
function lunch(x, y) {
sandwich(x, y);
apple(x + 95, y);
}
// draws dinner foods
function dinner(x, y) {
mashedPotatoes(x + 80, y + 90);
chicken(x, y);
}
// draws dessert
// function iceCream is put into this function just for ease of naming,
// since "dessert" better represents the meal of this specific time zone
// than does simply "ice cream"
function dessert (x, y) {
iceCream(x, y);
}
// draws two sunny-side up eggs
function eggs(x, y) {
noStroke();
fill(255, 255, 255, alphaValue);
ellipse(x, y, 100);
ellipse(x + 20, y + 75, 100);
fill(255, 155, 0, alphaValue);
ellipse(x + 15, y, 35);
ellipse(x + 45, y + 85, 35);
}
// draws two strips of bacon
function bacon(x, y) {
fill(255, 50, 50, alphaValue);
rect(x, y, 40, 180);
rect(x + 60, y, 40, 180);
fill(255, 150, 150, alphaValue);
rect(x + 25, y, 8, 180);
rect(x + 85, y, 8, 180);
}
// draws a sammich/sammie
function sandwich(x, y) {
fill(165, 130, 41, alphaValue);
ellipse(x - 5, y, 50);
ellipse(x + 35, y, 50);
rect(x - 20, y, 65, 75, 10);
fill(0, 255, 0, alphaValue);
quad(x - 25, y - 25, x + 15, y - 35, x + 20, y + 10, x - 10, y + 7);
quad(x - 20, y, x - 33, y + 25, x + 5, y + 45, x + 5, y);
quad(x - 20, y + 30, x - 14, y + 70, x + 20, y + 55, x + 10, y)
fill(255, 255, 0, alphaValue);
rect(x - 6, y - 22, 60, 95);
fill(255, 0, 0, alphaValue);
ellipse(x + 15, y + 15, 45, 85)
fill(165, 130, 41, alphaValue);
ellipse(x + 20, y - 5, 50);
ellipse(x + 55, y - 5, 50);
rect(x + 5, y - 5, 65, 75, 10);
}
// draws an apple
function apple(x, y) {
fill(102, 79, 22, alphaValue);
rect(x, y, 7, 20);
fill(230, 0, 0, alphaValue);
ellipse(x + 2, y + 55, 80, 70);
fill(0, 195, 0, alphaValue);
ellipse(x + 9, y + 17, 20, 6);
}
// draws a chicken drumstick
function chicken(x, y) {
fill(255, alphaValue);
rect(x - 10, y, 20, 125);
ellipse(x - 17, y + 120, 30);
ellipse(x + 13, y + 120, 30);
fill(165, 130, 41, alphaValue);
ellipse(x, y, 100, 125);
triangle(x, y + 40, x - 20, y + 75, x + 20, y + 75);
}
// draws a nice scoop of mashed potatoes with a slab of butter
function mashedPotatoes(x, y) {
fill(0, 0, 255, alphaValue);
ellipse(x, y, 200, 15);
fill(255, alphaValue);
arc(x, y, 100, 100, PI, 0);
fill(255, 255, 0, alphaValue);
rect(x - 15, y - 52, 50, 30, 10);
}
// draws a yummy ice cream cone
function iceCream(x, y) {
fill(25, 255, 255, alphaValue);
ellipse(x, y + 50, 150);
fill(255, 25, 235, alphaValue);
ellipse(x, y - 50, 150);
fill(191, 158, 74, alphaValue);
triangle(x - 80, y + 80, x + 80, y + 80, x, y + 220);
fill(245, 0, 0, alphaValue);
ellipse(x + 15, y - 125, 35);
fill(185, 0, 0, alphaValue);
rect(x + 15, y - 150, 2, 20);
fill(0, alphaValue);
quad(x - 20, y - 30, x - 14, y - 38, x - 12, y - 9, x - 18, y - 25);
quad(x - 40, y - 70, x - 34, y - 60, x - 30, y - 74, x - 28, y - 65);
quad(x + 20, y - 50, x + 29, y - 60, x + 35, y - 44, x + 28, y - 55);
quad(x + 60, y - 20, x + 54, y - 25, x + 57, y - 14, x + 65, y - 25);
fill(132, 25, 255, alphaValue);
ellipse(x - 15, y + 33, 10);
ellipse(x - 55, y + 25, 15);
ellipse(x - 25, y + 55, 8);
ellipse(x + 40, y + 25, 10);
ellipse(x + 25, y + 65, 12);
}
My clock for this week reflects my own biological clock…for when to eat meals! If it were truly accurately, every time of day would be “Time for Dessert!!!,” but for the sake of the project, I’ve also included breakfast, lunch, and dinner.
At the top are spoons to count the seconds, glasses for the minutes, and forks for the hours. The opacity of the meals also change, and are fully opaque when it is time to eat them!
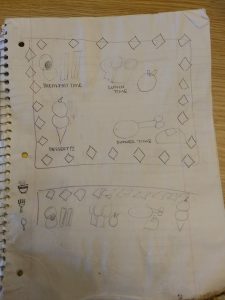
I assigned foods that are typical to each meal [in the US anyway], like some nice bacon and eggs, a sandwich and apple, a chicken drumstick and mashed potatoes with butter, and ice cream. If I ever feel both hungry and confused I will turn to this clock for meal suggestions!