/* Katherine Hua
Section A
khua@andrew.cmu.edu
Project-07-Curves */
function setup() {
createCanvas(480, 480);
frameRate(10);
}
function draw() {
var r1 = map(mouseX, 0, width, 230, 250); // making variables to make the background dependent on mouseX or mouseY
var g1 = map(mouseY, 0, height, 220, 230);
var b1 = map(mouseX, 0, width, 225, 250);
background(r1, g1, b1); // setting background color
var nPoints = 200; // setting number of points on shape
var radius;
// legs
strokeWeight(5);
bezier(random(195, 205), 320, 150, 320, 240, 400, random(165, 175), 400); // left leg
bezier(random(275, 285), 320, 320, 320, 240, 400, random(300, 310), 400); // right lelg
// body
strokeWeight(5);
fill(50, 50, 50);
push();
translate(width/2, height/2);
beginShape();
radius = 200;
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var a = map(mouseX, 0, 480, 90, 110); //body will change sizes based on position of mouseX
var n = map(mouseX, 0, 480, 40, 90);
var px = (a/n)*(((n-1)*cos(theta))-(cos((n-1)*theta)));
var py = (a/n)*(((n-1)*sin(theta))-(sin((n-1)*theta)));
vertex(px + random(-5, 5), py + random(-5, 5));
} endShape(CLOSE);
pop();
// eyes
strokeWeight(1);
fill(255);
nPoints = 10;
// whites of left eye
push();
translate(width/2 - 40, height/2 + 10);
beginShape();
radius = 30;
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-5, 5), py + random(-5, 5));
} endShape(CLOSE);
pop();
// whites of right eye
push();
translate(width/2 + 40, height/2 + 10);
beginShape();
radius = 30;
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-5, 5), py + random(-5, 5));
} endShape(CLOSE);
pop();
// pupil of left eye
fill(0);
nPoints = 4;
push();
translate(width/2 - 40, height/2 + 10);
beginShape();
radius = 10;
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-5, 5), py + random(-5, 5));
} endShape(CLOSE);
pop();
// pupil of right eye
push();
translate(width/2 + 40, height/2 + 10);
beginShape();
radius = 10;
for (var i = 0; i < nPoints; i++) {
var theta = map(i, 0, nPoints, 0, TWO_PI);
var px = radius * cos(theta);
var py = radius * sin(theta);
vertex(px + random(-5, 5), py + random(-5, 5));
} endShape(CLOSE);
pop();
//arms
noFill();
strokeWeight(5);
//making arms grabbing hand curve different depending if arms are reaching below or above the midpoint
if (mouseY > width/2) {
bezier(random(145, 155), 240, random(115, 125), 240, mouseX - 80, mouseY + 40, mouseX-50, mouseY-50); //left arm
bezier(random(325, 335), 240, 360, random(235, 245), mouseX + 80, mouseY - 40, mouseX+50, mouseY-50); //right arm
} else if (mouseY < width/2) {
bezier(150, 240, 120, 240, mouseX - 80, mouseY + 40, mouseX-50, mouseY+50); //left arm
bezier(330, 240, 360, 240, mouseX + 80, mouseY - 40, mouseX+50, mouseY+50); //right arm
}
//star
rectMode(CENTER);
push();
translate(mouseX, mouseY); //star will follow position of the mouse
var r2 = map(mouseY, 0, height, 200, 230);
var g2 = map(mouseY, 0, height, 150, 180);
var b2 = map(mouseX, 0, width, 125, 175);
fill(r2, g2, b2);
stroke(r2, g2, b2);
rect(0, 0, 20, 20);
push();
rotate(PI/4);
rect(0,0, 20, 20);
pop();
pop();
}
My design is based off of one of my favorite childhood movies, “Spirited Away.” The character I created is supposed to be one of the coal spirit (or soot ball). Below is an image of how they look like:
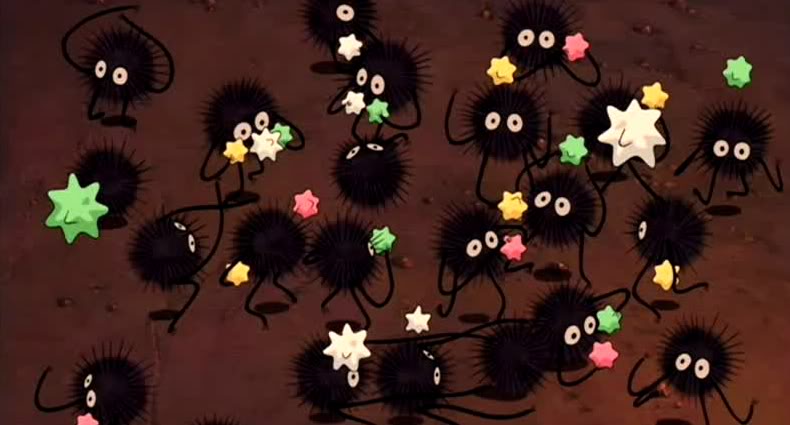
These sootballs are constantly moving and really like to eat these star candies so made a star candy follor the mouse and the sootball’s arms try to reach for the candy.