// Catherine Coyle
// ccoyle@andrew.cmu.edu
// section C
// project 9
var img;
var currColor;
var ix;
var iy;
var seenColors = [];
// basic image loading code
function preload() {
var imgurl = 'https://i.imgur.com/2TSW1Hy.png';
img = loadImage(imgurl)
}
function setup() {
createCanvas(480, 480);
background(0);
img.loadPixels();
}
function draw() {
// this basic pixel grabbing format was taken from the example on the website
var px = random(width);
var py = random(height);
ix = constrain(floor(px), 0, width-1);
iy = constrain(floor(py), 0, height-1);
currColor = img.get(ix, iy);
// will draw the shape/pixel with the right color
stroke(currColor);
drawShape(px, py);
}
// drawing the pixel at given location
function drawShape(x, y) {
var donutSize = calcSize();
strokeWeight(donutSize);
noFill();
ellipse(x, y, donutSize * 2, donutSize * 2);
}
// the size of the donuts is based on the frequency of
// pixels w the same color
function calcSize() {
var currColorBr = floor(brightness(currColor));
seenColors.push(currColorBr);
return constrain(countElements(currColorBr), 0, 20);
}
// idk if theres a java function that counts elements but
// i couldnt find one when i looked so i wrote one
function countElements(element) {
var c = 0;
for(var i = 0; i < seenColors.length; i++) {
if (seenColors[i] == element) {
c++;
}
}
// mod 10 to make them not get too big
return c % 10;
}
I thought this was a cool project! I chose my close friend Melissa as the subject because I think this is a really pretty picture of her that I took over the summer. She really likes donuts so I decided to make the ‘pixels’ all donut shaped. It felt too simple just doing that though so I wanted to add another aspect to it. I decided to have the sizes of the donuts be based on the frequency of the brightness of the pixels. It took a while because I found out that javascript doesn’t really have dictionaries which was disappointing so I just stored all the information in a list and wrote functions to count the frequency.
I am happy with how it turned out in the end!
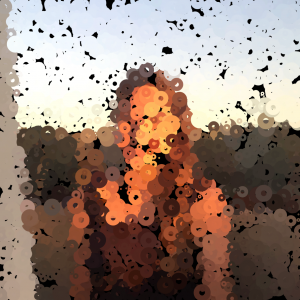
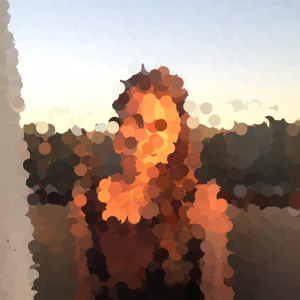
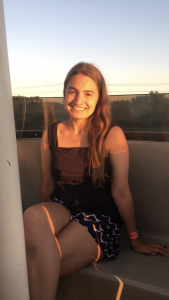