// Yoo Jin Shin
// Section D
// yoojins@andrew.cmu.edu
// Project 10: Generative Landscape
var buildings = [];
var terrainSpeed = 0.0003;
var terrainSpeed2 = 0.0002;
var terrainSpeed3 = 0.0001;
var terrainDetail = 0.003;
var terrainDetail2 = 0.005;
var terrainDetail3 = 0.006;
function setup() {
createCanvas(480, 450);
// create an initial collection of buildings
for (var i = 0; i < 10; i++){
var rx = random(width);
buildings[i] = makeBuilding(rx);
}
frameRate(10);
}
function draw() {
background(255);
terrain3();
terrain2();
terrain();
building();
}
// blue terrain in front
function terrain() {
push();
var R = random(5, 40);
var G = random(5, 40);
fill(R, G, 80);
stroke(255);
strokeWeight(6);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail) + (millis() * terrainSpeed);
var y = map(noise(t), 0, 1, 0, height);
vertex(x, y);
}
endShape();
pop();
}
// purple terrain in middle
function terrain2() {
push();
var R = random(40, 60);
var G = random(0, 30);
fill(R, G, 80);
stroke(255);
strokeWeight(3);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail2) + (millis() * terrainSpeed2);
var y = map(noise(t), 0, 2, 0, height);
vertex(x, y);
}
endShape();
pop();
}
// pink terrain in back
function terrain3() {
push();
var G = random(0, 40);
var B = random(90, 100);
fill(120, G, B);
stroke(255);
strokeWeight(1);
beginShape();
for (var x = 0; x < width; x++) {
var t = (x * terrainDetail3) + (millis() * terrainSpeed3);
var y = map(noise(t), 0, 3, 0, height);
vertex(x, y);
}
endShape();
pop();
}
function building() {
push();
translate(0.1 * width, 0.1 * height);
scale(0.8);
displayHorizon();
updateAndDisplayBuildings();
removeBuildingsThatHaveSlippedOutOfView();
addNewBuildingsWithSomeRandomProbability();
pop();
}
function updateAndDisplayBuildings(){
// Update the building's positions, and display them.
for (var i = 0; i < buildings.length; i++){
buildings[i].move();
buildings[i].display();
}
}
function removeBuildingsThatHaveSlippedOutOfView(){
var buildingsToKeep = [];
for (var i = 0; i < buildings.length; i++){
if (buildings[i].x + buildings[i].breadth > 0) {
buildingsToKeep.push(buildings[i]);
}
}
buildings = buildingsToKeep;
}
function addNewBuildingsWithSomeRandomProbability() {
// With a very tiny probability, add a new building to the end.
var newBuildingLikelihood = 0.007;
if (random(0,1) < newBuildingLikelihood) {
buildings.push(makeBuilding(width));
}
}
function buildingMove() {
this.x += this.speed;
}
// draw alien buildings with changing colors
function buildingDisplay() {
var floorHeight = 20;
var bHeight = this.nFloors * floorHeight;
var B = random(100, 255);
var R = random(100, 255);
fill(R, 255, B);
stroke(0);
strokeWeight(2);
push();
translate(this.x, height - 40);
ellipse(0, -bHeight + 20, this.breadth, bHeight * 2);
// draw windows
stroke(0);
strokeWeight(2);
fill(0);
for (var i = 0; i < this.nFloors; i++) {
rect(-5, -15 - (i * floorHeight + 10), 10, 10);
}
pop();
}
function makeBuilding(birthLocationX) {
var bldg = {x: birthLocationX,
breadth: round(random(30, 80)),
speed: -2,
nFloors: round(random(3, 7)),
move: buildingMove,
display: buildingDisplay}
return bldg;
}
function displayHorizon(){
noStroke();
fill(0, 43, 7);
rect(-200, height - 150, width + 300, height - 150);
}
I tried creating an alien world generative landscape. The sky portion of the landscape has three terrains varying in color and speed, looking somewhat like clouds. The buildings vary in size and color, looking almost like cacti. This project was rather challenging, but I would definitely experiment more with generative landscapes in the near future and try creating more elaborate ones.
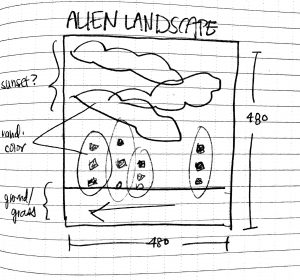