rjpark_wallpaper
var z = 150; // "zooming" the lines
var sw = z/20; // stroke weight of lines
var swl = z/100; // stroke weight of leaves
var l = z/10; // leaves placement on lines
var ls = z/5; // leaves size
function setup() {
createCanvas(600, 600);
background(190, 175, 155);
noLoop();
}
function draw() {
// set 1 of vertical lines (in even numbered columns)
// vx1 = variable for x coordinate movement
// vy1 = variable for y coordinate movement
for (var vx1 = 0; vx1 < width/10; vx1 ++) {
for (var vy1 = 0; vy1 < width/10; vy1 ++) {
stroke(90, 70, 60);
strokeWeight(sw);
line((z/2)+z*vx1, z*vy1, (z/2)+z*vx1, (z/2)+z*vy1);
}
}
// set 2 of vertical lines (in odd numbered columns)
// vx2 = variable for x coordinate movement
// vy2 = variable for y coordinate movement
for (var vx2 = 0; vx2 < width/10; vx2 ++) {
for (var vy2 = 0; vy2 < width/10; vy2 ++) {
stroke(120, 50, 20);
strokeWeight(sw);
line(z*vx2, (z/2)+z*vy2, z*vx2, z+z*vy2);
}
}
// set 1 of horizontal lines (in even numbered columns)
// hx1 = variable for x coordinate movement
// hy1 = variable for y coordinate movement
for (var hx1 = 0; hx1 < height/10; hx1 ++) {
for (var hy1 = 0; hy1 < height/10; hy1 ++) {
stroke(120, 50, 20);
strokeWeight(sw);
line((z/2)+z*hx1, (z/2)+z*hy1, z+z*hx1, (z/2)+z*hy1);
}
}
// set 2 of horizontal lines (in odd numbered columns)
// hx2 = variable for x coordinate movement
// hy2 = variable for y coordinate movement
for (var hx2 = 0; hx2 < height/10; hx2 ++) {
for (var hy2 = 0; hy2 < height/10; hy2 ++) {
stroke(90, 70, 60);
strokeWeight(sw);
line(z*hx2, z*hy2, (z/2)+z*hx2, z*hy2);
}
}
// set 1 of leaves on first xy coordinate of vertical lines (set 1)
for (var vx1 = 0; vx1 < width/10; vx1 ++) {
for (var vy1 = 0; vy1 < width/10; vy1 ++) {
fill(80, 110, 75);
noStroke();
ellipse((z/2)+z*vx1+l, z*vy1, ls, ls); // leaf body
triangle((z/2)+z*vx1+l, z*vy1-(ls/2), (z/2)+z*vx1+l+(ls/2), z*vy1, (z/2)+z*vx1+l+(ls/2), z*vy1-(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line((z/2)+z*vx1+(ls/5), z*vy1+(ls/3), (z/2)+z*vx1+l, z*vy1); // leaf veins
fill(130, 140, 90);
noStroke();
ellipse((z/2)+z*vx1, z*vy1-l, ls, ls); // leaf body
triangle((z/2)+z*vx1, z*vy1-l-(ls/2), (z/2)+z*vx1+(ls/2), z*vy1-l, (z/2)+z*vx1+(ls/2), z*vy1-l-(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line((z/2)+z*vx1-(ls/3), z*vy1-(ls/5), (z/2)+z*vx1, z*vy1-l); // leaf veins
}
}
// set 2 of leaves on first xy coordinate of horizontal lines (set 1)
for (var hx1 = 0; hx1 < width/10; hx1 ++) {
for (var hy1 = 0; hy1 < width/10; hy1 ++) {
fill(205, 185, 90);
noStroke();
ellipse((z/2)+z*hx1-l, (z/2)+z*hy1, ls, ls); // leaf body
triangle((z/2)+z*hx1-l-(ls/2), (z/2)+z*hy1, (z/2)+z*hx1-l, (z/2)+z*hy1+(ls/2), (z/2)+z*hx1-l-(ls/2), (z/2)+z*hy1+(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line((z/2)+z*hx1-(ls/5), (z/2)+z*hy1-(ls/3), (z/2)+z*hx1-l, (z/2)+z*hy1); // leaf veins
fill(250, 240, 190);
noStroke();
ellipse((z/2)+z*hx1, (z/2)+z*hy1+l, ls, ls); // leaf body
triangle((z/2)+z*hx1-(ls/2), (z/2)+z*hy1+l, (z/2)+z*hx1, (z/2)+z*hy1+l+(ls/2), (z/2)+z*hx1-(ls/2), (z/2)+z*hy1+l+(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line((z/2)+z*hx1+(ls/3), (z/2)+z*hy1+(ls/5), (z/2)+z*hx1, (z/2)+z*hy1+l); // leaf veins
}
}
// set 3 of leaves on first xy coordinate of vertical lines (set 2)
for (var vx2 = 0; vx2 < width/10; vx2 ++) {
for (var vy2 = 0; vy2 < width/10; vy2 ++) {
fill(80, 110, 75);
noStroke();
ellipse(z*vx2+l, (z/2)+z*vy2, ls, ls); // leaf body
triangle(z*vx2+l, (z/2)+z*vy2-(ls/2), z*vx2+l+(ls/2), (z/2)+z*vy2, z*vx2+l+(ls/2), (z/2)+z*vy2-(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line(z*vx2+(ls/5), (z/2)+z*vy2+(ls/3), z*vx2+l, (z/2)+z*vy2); // leaf veins
fill(130, 140, 90);
noStroke();
ellipse(z*vx2, (z/2)+z*vy2-l, ls, ls); // leaf body
triangle(z*vx2, (z/2)+z*vy2-l-(ls/2), z*vx2+(ls/2), (z/2)+z*vy2-l, z*vx2+(ls/2), (z/2)+z*vy2-l-(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line(z*vx2-(ls/3), (z/2)+z*vy2-(ls/5), z*vx2, (z/2)+z*vy2-l); // leaf veins
}
}
// set 4 of leaves on first xy coordinate of horizonal lines (set 2)
// replaces leaves on second xy coordinate of vertical lines (set 2) because first coordinate of first row of horizontal lines need leaves
for (var hx2 = 0; hx2 < height/10; hx2 ++) {
for (var hy2 = 0; hy2 < height/10; hy2 ++) {
fill(205, 185, 90);
noStroke();
ellipse(z*hx2-l, z*hy2, ls, ls); // leaf body
triangle(z*hx2-l-(ls/2), z*hy2, z*hx2-l, z*hy2+(ls/2), z*hx2-l-(ls/2), z*hy2+(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line(z*hx2-(ls/5), z*hy2-(ls/3), z*hx2-l, z*hy2); // leaf veins
fill(250, 240, 190);
noStroke();
ellipse(z*hx2, z*hy2+l, ls, ls); // leaf body
triangle(z*hx2-(ls/2), z*hy2+l, z*hx2, z*hy2+l+(ls/2), z*hx2-(ls/2), z*hy2+l+(ls/2)); // leaf tip
stroke(0);
strokeWeight(swl);
line(z*hx2+(ls/3), z*hy2+(ls/5), z*hx2, z*hy2+l); // leaf veins
}
}
}
I knew from the start that I wanted to implement nature and earth tones into my wallpaper. So, I created a simple, stair-shaped pattern for vines and pairs of leaves at each intersection of the stair-shaped lines.
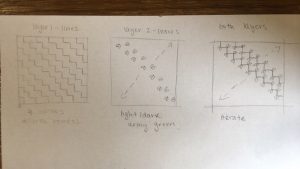
Although this looks like an easy pattern to code, there were a lot of different parts to consider. I had to iterate the vertical and horizontal lines both across and down; I had to make 4 double for-loops to make this happen. So, there are 2 sets of vertical and horizontal lines each. You can see the 2 sets by color (red-ish brown and dark brown). I also had to iterate the pairs of leaves at one end of each vertical and horizontal line (2 sets for each), so, I also had to make 4 double for-loops to make this happen.
Lastly, I created global variables that make it easier for the user to change the dimensions of the shapes in the wallpaper. Of those global variables, only one has to be changed because the others are dependent on that one global variable, z. If the user changes z, the entire wallpaper will either “zoom” in or out.