TEST CARD GENERATOR
The Test Card Generator (TCG) allows you to create a customizable Test pattern to use for testing outputs for a media installation. You can select HD or SD (1920×1080 or 1280×720) which will populate on your card. Then using the input fields you can type in what you’d like the card to say. Provided is a field for a title and labeling the output and input; a Projector using SDI, for example. You can also color the grid lines to further customize and is extremely helpful with multiple outputs. Un-checking the boxes will take away some of the graphic elements to further customize the look of the card. Lastly clicking save will save a PNG titled with the name and selected resolution to your downloads folder.
As a media designer, I’m often faced with ambitious projects and installations that call for multiple outputs and sources of media. In a room with wrap around projections seeing the same test pattern everywhere doesn’t help you understand how your system is working and what is media is being feed to what. A tool like TCG allows me to quickly test multiple output sources and customize the look based on version, show, or whatever I feel like I need shown.
Below are some of the customizable test cards I generated:
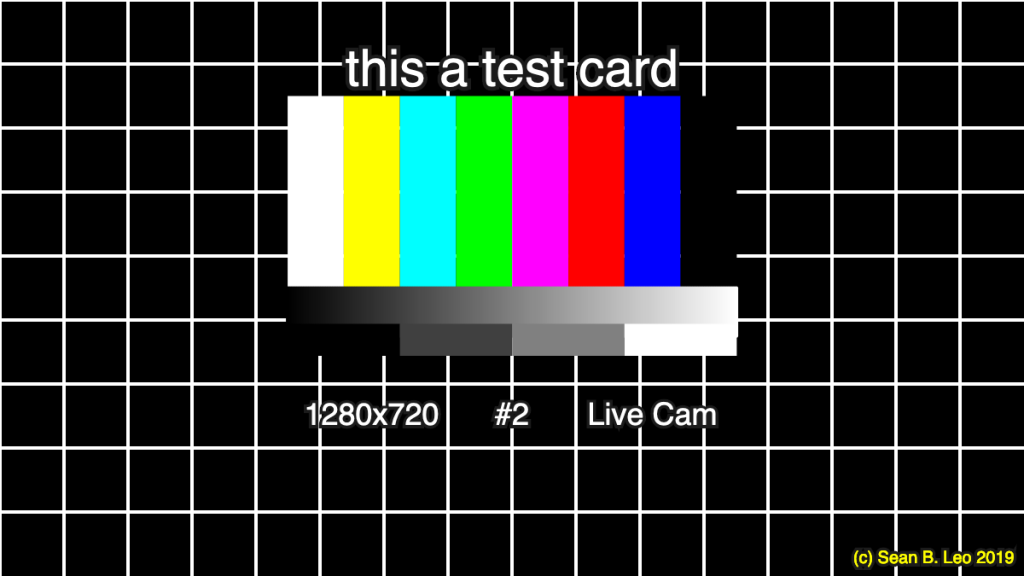
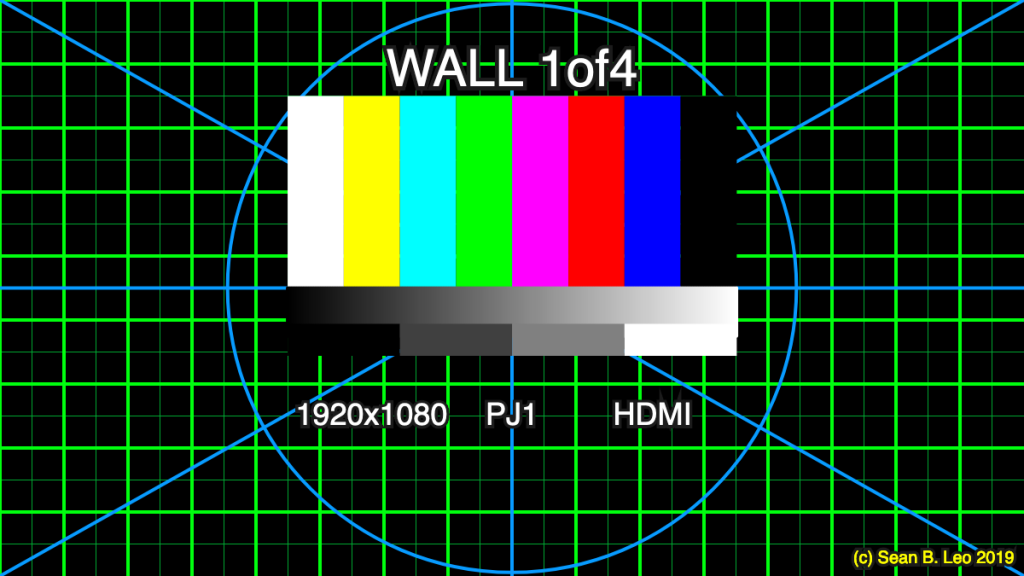
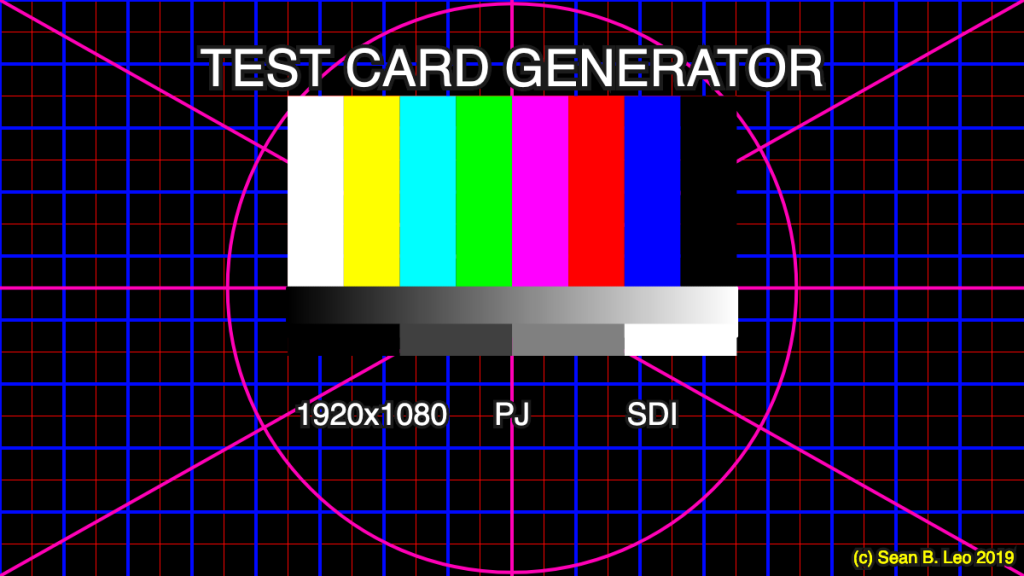
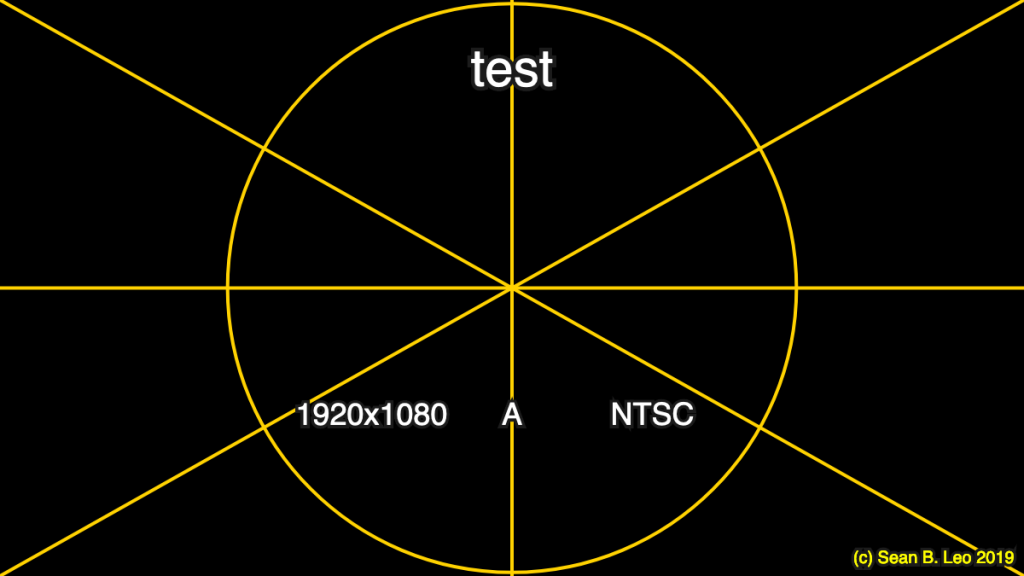
//Sean B. Leo
//sleo@andrew.cmu.edu
//Section C
//FINAL PROJECT
//TEST CARD GENERATOR___________(c) Sean B. Leo 2019
/*The TCG allows you to create a customizable Test pattern
to use for testing outputs for a media installion. You can
select HD or SD (1920x1080 or 1280x720) which will populate on your card.
Then using the input fields you can type in what you'd like the card to say.
Provided is a field for a title and labeling the output and input;
a Projector using SDI for example. You can also color the grid lines to further
customize and is extremly helpful with multiple outputs.
Un-checking the boxes will take away some of the graphic elements
to further customize the look of the card.
Lastly clicking save will save a PNG titled with the name and selected resolution
to your downloads folder.*/
//Global variables
//aspect ratio
var aspectX = 16;
var aspectY = 9;
// grid color variables
var colorInp1, colorInp2;
//gradient color variables
var c1, c2;
//grid slider
var gridSlider;
var spacing = 0;
//element dimensions
var colW;
var colH;
var rowW;
var rowH;
//radio varibile
var radio;
function setup() {
//radio options set for HD and SD
radio = createRadio();
radio.option('1920x1080');// HD
radio.option('1280x720');// SD
//canvas sized to fit within WP window
createCanvas(600, 337.5);
colorMode(RGB);
//Color picker input for elements
colorInp1 = createColorPicker('#ffffff'); //set to white at start
colorInp1.position(360,height+30);
colorInp2 = createColorPicker('#727272'); //set to gray at start
colorInp2.position(420,height+30);
colorInp3 = createColorPicker('#ffffff'); //set to white at start
colorInp3.position(480,height+30);
//black and white gradient color
c1 = color(0);
c2 = color(255);
//Title for test card
textInp1 = createInput('NAME YOUR TEST CARD');
textInp1.size(150, 20);
textInp1.position(0,height+30);
//label output (projector, screen, etc.)
textInp2 = createInput('OUTPUT');
textInp2.size(100, 20);
textInp2.position(150,height+30);
//label input (HDMI, DVI, SDI, etc.)
textInp3 = createInput('INPUT');
textInp3.size(100, 20);
textInp3.position(250,height+30);
//Save custom test card
button = createButton('Save Test Card');
button.position(420, height+60);
button.mousePressed(saveImage);
//graphic element sizing
colW = width/2.28/8;
colH = height/3;
barStartX = width/3.56;
barStartY = height/6;
//turn off or on elements
toggleGrid = createCheckbox('Grid', true);//set as on from start
toggleGrid.changed(toggleSet);
toggleGrid.position(0, height+60);
toggleLines = createCheckbox('Lines', true);
toggleLines.changed(toggleSet);
toggleLines.position(60, height+60);
toggleColor = createCheckbox('Color Bars', true);
toggleColor.changed(toggleSet);
toggleColor.position(120, height+60);
toggleGrad = createCheckbox('Black and White', true);
toggleGrad.changed(toggleSet);
toggleGrad.position(210, height+60);
}
function saveImage(){
radioXY = radio.value(); // read radio value as variable
var title = textInp1.value() +"_" + radioXY; //set file name from custom inputs
if (button.mousePressed()){
saveCanvas(title,'png');
}
}
function draw() {
background(0);
toggleSet();
textBlocks();
}
function toggleSet(){
//turn off or on element functions based on state of checkbox
if (toggleGrid.checked()) {
grid();
}
if (toggleLines.checked()) {
lines();
}
if (toggleColor.checked()) {
colorBars();
}
if (toggleGrad.checked()){
gradient();
}
}
function grid(){
//draw grid
var gridColorTop = colorInp1.color();//set grid color based on selection
var gridColorBot = colorInp2.color();//set grid color based on selection
var gridStrokeTop = 2;
var gridStrokeBot = .5
var x = spacing;
var y = spacing;
//bottom grid loop
for (var x = 0; x < width; x += width / (aspectX*2)) {
for (var y = 0; y < height; y += height / (aspectY*2)) {
stroke(gridColorBot);
strokeWeight(gridStrokeBot);
line(x, 0, x, height);
line(0, y, width, y);
}
}
//top grid
for (var x = 0; x < width; x += width / aspectX) {
for (var y = 0; y < height; y += height / aspectY) {
stroke(gridColorTop);
strokeWeight(gridStrokeTop);
line(x, 0, x, height);
line(0, y, width, y);
}
}
}
function lines(){
var lineColor = colorInp3.color();//set color based on selection
stroke(lineColor);
strokeWeight(2);
//diagonals
line(0, 0, width, height);
line(width, 0, 0, height);
noFill();
//cross lines
line(width/2, 0, width/2, height);
line(0, height/2, width, height/2);
//circle
ellipse(width/2, height/2, width/1.8);
}
function colorBars(){
//SMPTE color bars
noStroke();
fill('white');
rect(barStartX, barStartY, colW, colH);
fill('yellow');
rect(barStartX+colW, barStartY, colW, colH);
fill('cyan');
rect(barStartX+colW*2, barStartY, colW, colH);
fill(0, 255, 0);
rect(barStartX+colW*3, barStartY, colW, colH);
fill('magenta');
rect(barStartX+colW*4, barStartY, colW, colH);
fill('red');
rect(barStartX+colW*5, barStartY, colW, colH);
fill('blue');
rect(barStartX+colW*6, barStartY, colW, colH);
fill('black');
rect(barStartX+colW*7, barStartY, colW, colH);
}
function setGradient(x, y, w, h, c1, c2) {
//creates gradient
noFill();
for (var i = x; i <= x + w; i++) {
var inter = map(i, x, x + w, 0, 1);
var c = lerpColor(c1, c2, inter);
stroke(c);
line(i, y, i, y + h);
}
}
function gradient(){
//gradient block
//calls gradient and creates shape
setGradient(barStartX, height/2, colW*8, colH/4, c1, c2);
//grayscale blocks
noStroke();
fill(0);
rect(barStartX, height/1.779, colW*2, colH/6);
fill(255/4);
rect(barStartX+colW*2, height/1.779, colW*2, colH/6);
fill(255/2);
rect(barStartX+colW*4, height/1.779, colW*2, colH/6);
fill('white');
rect(barStartX+colW*6, height/1.779, colW*2, colH/6);
}
function textBlocks() {
radioXY = radio.value();
fill(255);
textSize(30);
textAlign(CENTER, CENTER);
stroke(27);
strokeWeight(5);//set against grid pattern for better legibility
//takes in first text value to display at top of canvas
text(textInp1.value(), width/2, barStartY-barStartY/4);
strokeWeight(4);
//textSize(18);
//takes in radio button value to set text on canvas
textSize(18);
text(radioXY, width/2 - colW*2.5, barStartY*4.35);
//second text value 'OUTPUT'
textSize(18);
text(textInp2.value(), width/2, barStartY*4.35);
//third text value 'INPUT
textSize(18);
text(textInp3.value(), width/2 + colW*2.5, barStartY*4.35);
//Signing my work
textSize(10);
fill('yellow');
text('(c) Sean B. Leo 2019', width-colW-20, height-10);
}