function setup() {
createCanvas(200, 300);
background(1);
}
function draw() {
rect(width/2, height/2, 50, 50);
fill("red")
}
Category: Project-01-Face
Zee Salman – Project – 01
function setup() {
createCanvas(200,300);
}
function draw(){
rect(10,10,40,40);
fill('pink')
}
Joseph Zhang – Project – 01
//https://p5js.org/reference/
// setup() runs once
// every program should have program setup
function setup(){
createCanvas(600,600);
background('#D1C3BA');
noStroke();
//black hair
fill('#191919');
ellipse(300,274,218.6,288);
translate(291.6,161.5);
rotate(-6.1);
ellipse(0,0,138.9,74.1);
rotate(6.1);
translate(-291.6,-161.5);
//ears + neck
fill('#C69B7B');
ellipse(195,312,34,58);
ellipse(404,312,34,58);
rect(243,397,114,142);
//face + facial features
fill('#E0BA99');
ellipse(300,307,208,274);
rect(196,312,208,91);
triangle(196, 404, 300, 467, 404, 404);
rect(300,371.5,80,27);
//nose + mouth
fill('#C69B7B');
triangle(284, 350, 300, 322.6, 316, 350);
rect(284,344,32,12);
ellipse(300,385,80,46);
//upper mouth
fill('#E0BA99');
rect(260,358,80,27);
//eyes and body
fill('#191919');
ellipse(247,299,22,22);
ellipse(353,299,22,22);
ellipse(300,644,360,274);
// ears
fill('#DDDDDD');
ellipse(186,332,8,8);
ellipse(414,332,8,8);
}
Besides understanding the functions available with using p5.js, one of the biggest takeaways I received was understanding the importance of sequencing code properly. Certain lines of code would mess the entire visual composition of the portrait if not put in the right place. I realized that much of the learning process came from working, crashing, revising, and rebuilding.
Jina Lee – Project – 01
Before starting this code, I made an Adobe Illustrator file. The reference made it easier for me to understand where the coordinates were. When I first started, I couldn’t mentally imagine the where the shapes were on the canvas, but as I added more elements it got easier.
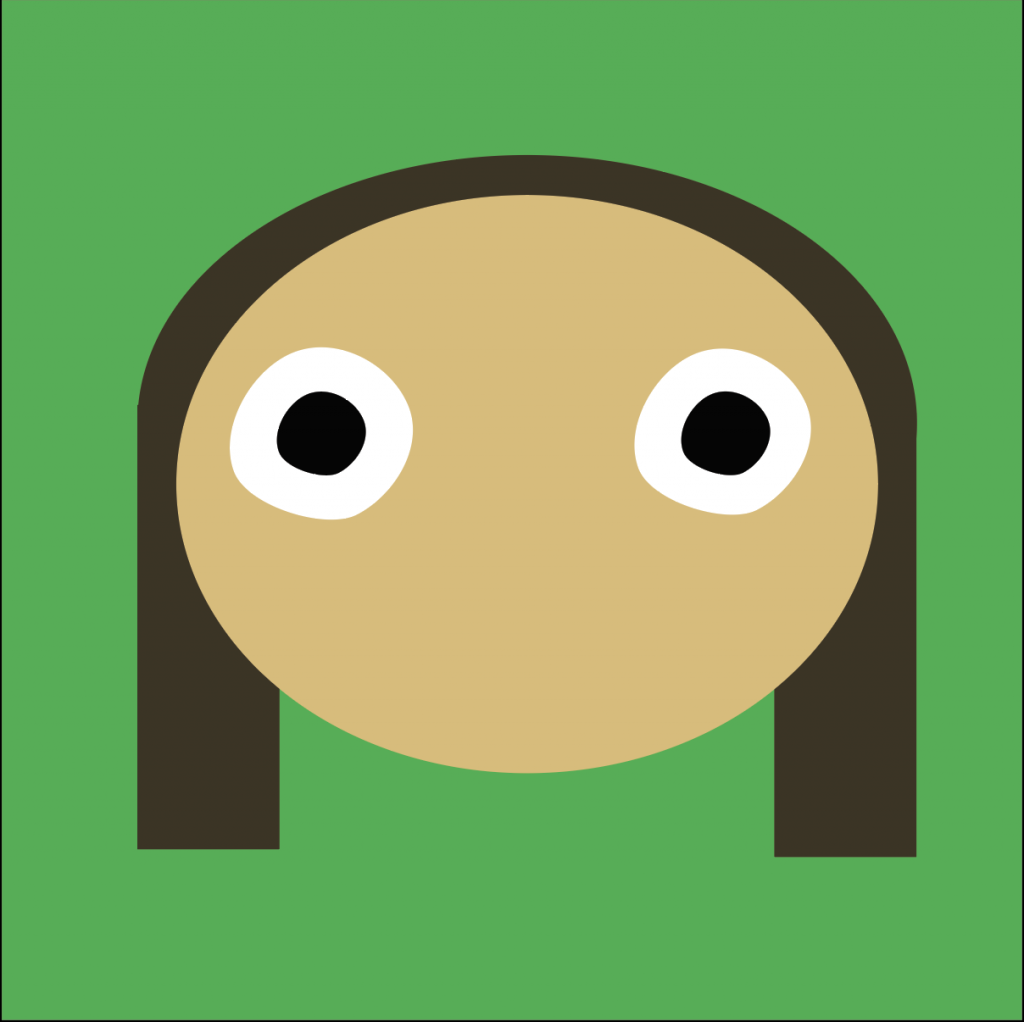
function setup() {
//Jina Lee
//Section E
//jinal2@andrew.cmu.edu
//Project-01(Self-Portrait)
createCanvas(400, 400);
background('rgb(46, 175, 74)');
//hair
noStroke();
fill('rgb(61, 52, 35)');
rect(50, 160, 80, 150);
noStroke();
fill('rgb(61, 52, 35)');
rect(270, 160, 80, 150);
noStroke();
fill('rgb(61, 52, 35)');
ellipse(200, 160, 300, 210);
//face
noStroke();
fill('rgb(201, 171, 112)');
rect(163.5, 270, 71.2, 40);
noStroke();
fill('rgb(221, 188, 115)');
ellipse(200, 180, 250, 210);
noStroke();
fill('rgb(221, 188, 115)');
ellipse(70, 180, 30, 40);
noStroke();
fill('rgb(221, 188, 115)');
ellipse(330, 180, 30, 40);
//shirt
noStroke();
fill('rgb(118, 185, 219)');
arc(200, 401, 250, 200, PI, 0, CHORD);
noStroke();
fill('rgb(201, 171, 112)');
arc(199, 301, 71, 65, 0, PI, OPEN);
//eyes
noStroke();
fill('white');
ellipse(130, 160, 60, 60);
noStroke();
fill('white');
ellipse(275, 160, 60, 60);
noStroke();
fill('black');
ellipse(275, 160, 30, 30);
noStroke();
fill('black');
ellipse(130, 160, 30, 30);
//earrings
noStroke();
fill('rgb(183, 183, 183)');
arc(340, 170, 10, 10, 0, PI + QUARTER_PI, PIE);
noStroke();
fill('white');
ellipse(335, 190, 5, 5);
noFill();
stroke('rgb(183, 183, 183)');
strokeWeight(2);
arc(330, 199, 10, 10, 0, PI);
noFill();
stroke('rgb(183, 183, 183)');
strokeWeight(2);
arc(70, 199, 10, 10, 0, PI);
//mouth
noStroke();
fill('white');
ellipse(200, 230, 50, 50);
noStroke();
fill('black');
arc(200, 230, 50, 50, 0, PI, PIE);
stroke('black');
strokeWeight(4);
line(200, 250, 200, 206.5);
//baby hairs
noFill();
stroke('rgb(61, 52, 35)');
strokeWeight(10);
line(110, 60, 130, 80);
noFill();
stroke('rgb(61, 52, 35)');
strokeWeight(10);
line(130, 60, 130, 80);
}
function draw() {
}
William Su – Project – 01
function setup() {
createCanvas(200, 200);
}
function draw() {
rect(10,10,80,20);
}
Deklin Versace – Project – 01
function setup() {
createCanvas(200, 600);
}
function draw() {
rect(10,10,70,400);
}
Gretchen Kupferschmid-Project-01
Mihika Bansal-Project- 01
function setup() {
createCanvas(200, 300);
}
function draw() {
rect(10,10,40,40);
fill("red");
}
Alice Cai Project Week 1
function setup() {
createCanvas(200,200);
}
function draw () {
rect(10,10,40,40);
fill("black");
}
Caroline Song – Project 01
function setup (){
createCanvas(200,300);
}
function draw(){
fill("red");
rect(10,10,40,40);
}