//Shannon Ha
//sha2@andrew.cmu.edu
// Section D
//Project 10 Sonic-Sketch
var underlyingImage;
function preload() { // call loadImage() and loadSound() for all media files here
scissorSnd = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/scissors-2/");
combingSnd = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/combing2-2/");
hairdryerSnd = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/hairdrying-2/");
spraySnd = loadSound(" https://courses.ideate.cmu.edu/15-104/f2019/spray-2/");
tadaSnd = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/mirror-2/");
spraySndB = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/spray2-2/");
combingSndB = loadSound("https://courses.ideate.cmu.edu/15-104/f2019/combing-hair-2/")
var saloonImg = "https://i.imgur.com/PqazkIh.jpg";
underlyingImage = loadImage(saloonImg);
}
function setup() {
createCanvas(500, 500);
useSound();
}
function soundSetup() { // setup for audio generation
scissorSnd.setVolume(2);
combingSnd.setVolume(5);
hairdryerSnd.setVolume(1);
spraySnd.setVolume(1);
tadaSnd.setVolume(1);
}
function draw() {
background(0);
image(underlyingImage, 0, 0); // stock photo of the saloon
}
function mousePressed() {
//makes the sound of hair combing play when the mouse is between the set of XY coordinates boundaries of comb images.
if (mouseX > 80 & mouseX < 160 & mouseY > 255 & mouseY < 360){
combingSndB.play();
}
else{
combingSndB.pause(); //pauses sound when mouse is clicked outside of the XY coordinate boundaries.
}
//makes the sound of spray play when the mouse is between the set of XY coordinate boundaries of spray image.
if (mouseX > 10 & mouseX < 160 & mouseY > 130 & mouseY < 240){
spraySndB.play();
}
else{
spraySndB.pause(); //pauses sound when mouse is clicked outside of the XY coordinate boundaries.
}
//makes the sound of hairdrying play when the mouse is between the set of XY coordinate boundaries of spray image.
if (mouseX > 70 & mouseX < 190 & mouseY > 30 & mouseY < 130){
hairdryerSnd.play();
}
else{
hairdryerSnd.pause();//pauses sound when mouse is clicked outside of the XY coordinate boundaries.
}
//makes the sound of combing play when the mouse is between the set of XY coordinate boundaries of combing image.
if (mouseX > 210 & mouseX < 320 & mouseY > 20 & mouseY < 110){
combingSnd.play();
}
else{
combingSnd.pause();//pauses sound when mouse is clicked outside of the XY coordinate boundaries.
}
//makes the sound of spray play when the mouse is between the set of XY coordinate boundaries of spray image.
if (mouseX > 330 & mouseX < 460 & mouseY > 20 & mouseY < 150){
spraySnd.play();
}
else{
spraySnd.pause(); //pauses sound when mouse is clicked outside of the XY coordinate boundaries.
}
if (mouseX > 330 & mouseX < 470 & mouseY > 150 & mouseY < 200){
scissorSnd.play();
}
else{
scissorSnd.pause();
}
if (mouseX > 330 & mouseX < 450 & mouseY > 205 & mouseY < 325){
tadaSnd.play();
}
else{
tadaSnd.pause();
}
}
This week I decided to use base my project on the sounds that you would hear at the hair salon. I found a copyright-free image that visualizes the steps the one goes through at a hair salon and I think adding the audio was a good way to show the procedure of getting your hair cut. I don’t know why my sketch won’t load on WordPress but it works so my localhost. Attached below is the image that I used for this project.
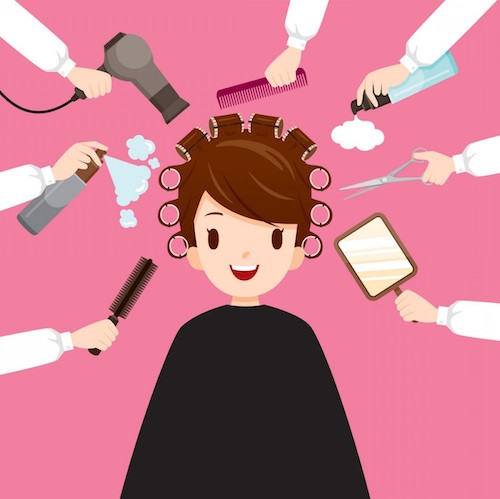
*1 grace day used for this project.