This is a random portrait generator that creates random robot faces! The hardest part of this was writing a loop that could let each mouse click cause the program to cycle through several faces at a time.
sketch
/*
* Eric Zhao
* ezhao2@andrew.cmu.edu
* Section D
*
* Random Face Generator. Randomizes face shape, eye color and size, nose shape,
* and presence of antenna. Operates on click and rolls through several faces
* per click.
*/
let w = 0; //face width
let h = 0; //face height
let topRadius = 0; //face curvature
let botRadius = 0; //face curvature
let eyeRadius = 0; //eye size
let index = 15; //loop index
let noseCircle = 0; //controls shape of nose
let faceColor = 0; //grayscale face color value
let eyeR = 0; //eye Red value
let eyeG = 0; //eye Green value
let eyeB = 0; //eye Blue value
function setup() {
createCanvas(600, 600);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
frameRate(30);
rectMode(CENTER);
}
function draw() {
/* This draw command runs when the mouse is clicked, generating 15 portraits and stopping
on the last one. Given that each face needs random values, this function sets the variables
instead of the mousePressed function. */
//setting variables randomly
w = random(width/4, width/2); //next 4 lines generate random rectangle dimensions
h = random(w/2, w*1.5);
topRadius = random(0, width/8);
botRadius = random(width/20, width/3);
eyeRadius = random(w/8, h/4);
noseCircle = int(random(int(0), int(2)));
faceColor = random (100, 200);
eyeR = random(30, 256);
eyeG = random(30, 256);
eyeB = random(30, 256);
//setting fills and strokes
background(185, 240, 185);
noStroke();
fill(faceColor);
rect(width/2, height/2, w, h, topRadius, topRadius, botRadius, botRadius); //draws rectangle
fill(eyeR, eyeG, eyeB)
ellipse((width/2) - (w/5), (height/2) - (h/5), eyeRadius, eyeRadius); //left eye
ellipse((width/2) + (w/5), (height/2) - (h/5), eyeRadius, eyeRadius); //right eye
noFill();
strokeWeight(5);
stroke(220);
if((eyeR + eyeG + eyeB) / 3 > 128) { //if the eye color is above a certain brightness, darken stroke
stroke(50)
}
arc((width/2) - (w/5), (height/2) - (h/5), eyeRadius * 0.8, eyeRadius * 0.8,
PI + QUARTER_PI, QUARTER_PI); //left reflection
arc((width/2) + (w/5), (height/2) - (h/5), eyeRadius * 0.8, eyeRadius * 0.8,
PI + QUARTER_PI, QUARTER_PI); //right reflection
stroke(220);
if (noseCircle === 1) { //this loop controls for nose shape and antenna
//draws a round nose and face profile
arc((width/2), (height/2) + (eyeRadius * 0.8) / 2, eyeRadius * 0.8, eyeRadius * 0.8,
HALF_PI, PI + HALF_PI);
line (width/2, (height/2) - (h/2), width/2, height/2);
line (width/2, (height/2) + (eyeRadius * 0.8), width/2, (height/2 + h/2));
stroke(faceColor);
line (width/2, (height/2) - (h/2), (width/2) + (w/4), (height/2) - (h/1.5)); //draws antenna stem
noStroke();
fill (255, 66, 66);
ellipse((width/2) + (w/4), (height/2) - (h/1.5), eyeRadius * 0.5, eyeRadius * 0.5); //draws antenna circle
} else {
//draws a triangle nose / face profile
line (width/2, (height/2) - (h/2), width/2, height/2 - eyeRadius);
line (width/2, height/2 - eyeRadius, (width/2) - (w/8), (height/2) + (h/4));
line ((width/2) - (w/8), (height/2) + (h/4), width/2, (height/2) + (h/4));
line (width/2, (height/2) + (h/4), width/2, (height/2 + h/2));
}
print(index.toString()); //prints loop index to console
index++;
if (index > 9) { //repeats the draw command 15 times and stops the loop afterwards
noLoop();
}
}
function mousePressed(){
index = 0;
loop();
}
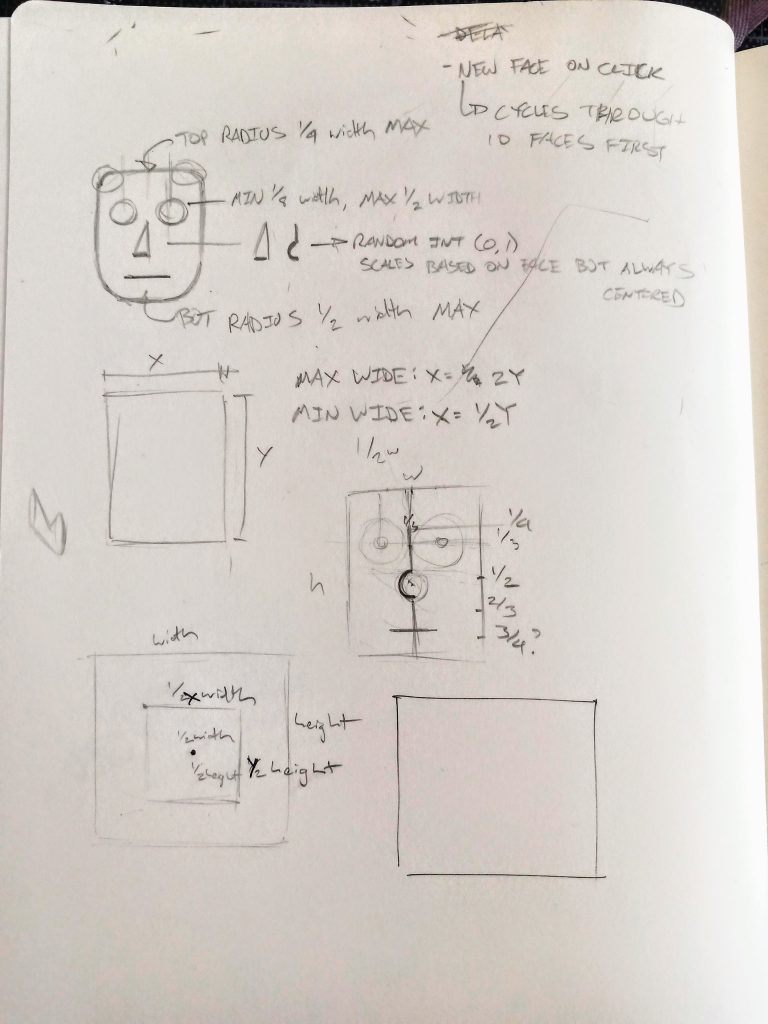