I created a wallpaper with illustrations of birds, rainbows, and clouds. Recently, I saw two parrots outside my window (it was odd because I live in the middle of an urban city). I was worried they might not survive the cold weather, but they flew away as my family opened the window to bring them in. I wanted to create visuals of the scene I saw that day in my wallpaper.
sketch
//Stefanie Suk
//15-104 Section D
var positionbx = 10; //starting position x for blue bird
var positionby = 10; //starting position y for blue bird
var offsetbx = 120; //spacing x for blue bird
var offsetby = 140; //spacing y for blue bird
var positionyx = 75; //starting position x for yellow bird
var positionyy = 75; //starting position y for yellow bird
var offsetyx = 120; //spacing x for yellow bird
var offsetyy = 140; //spacing y for yellow bird
var positionrx = 10; //starting position x for rainbow
var positionry = 95; //starting position y for rainbow
var offsetrx = 120; //spacing x for rainbow
var offsetry = 140; //spacing y for rainbow
var positioncx = 70; //starting position x for cloud
var positioncy = 20; //starting position y for cloud
var offsetcx = 120; //spacing x for cloud
var offsetcy = 140; //spacing y for cloud
var positiondx = 0; //starting position x for dots
var positiondy = 0; //starting position y for dots
var offsetdx = 20; //spacing x for dots
var offsetdy = 20; //spacing y for dots
function setup() {
createCanvas(500, 600);
background(195, 213, 195);
noLoop();
}
function draw() {
for(var a=0; a<50; a++){ //column of grid
for(var b = 0; b<50; b++){ //row of grid
push();
translate(positiondx + offsetdx*b, positiondy + offsetdy*a); //scale down
dots();
pop();
}
}
// blue bird grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positionbx + offsetbx*b, positionby + offsetby*a);
scale(0.5); //scale down
bluebird();
pop();
}
}
// yellow bird grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positionyx + offsetyx*b, positionyy + offsetyy*a);
scale(0.4); //scale down
yellowbird();
pop();
}
}
//rainbow grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positionrx + offsetrx*b, positionry + offsetry*a);
scale(0.5); //scale down
rainbow();
pop();
}
}
//cloud grid
for(var a=0; a<5; a++){ //column of grid
for(var b = 0; b<5; b++){ //row of grid
push();
translate(positioncx + offsetcx*b, positioncy + offsetcy*a);
cloud();
pop();
}
}
}
function bluebird() {
//body
noStroke();
fill(178, 232, 245);
ellipse(0, 0, 50, 50); //head
ellipse(0, 40, 60, 80);
fill(178, 232, 245); //body
push()
rotate(radians(30));
ellipse(48, 30, 10, 60) //tail
// white section of head
fill(250);
rotate(radians(220));
arc(0, 0, 45, 45, 0, QUARTER_PI);
pop();
//beak
fill(187, 201, 205);
ellipse(4, 0, 10, 10); //right grey circle
ellipse(-4, 0, 10, 10); //left grey circle
fill(157, 170, 173);
triangle(-6, 2, 6, 2, 0, 18); //dark grey beak
//eye
fill(10);
ellipse(10, -2, 7, 7); //right eye
ellipse(-10, -2, 7, 7); //left eye
//wings
fill(132, 163, 170);
ellipse(20, 40, 15, 40); //right dark blue wing
ellipse(-20, 40, 15, 40); //left dark blue wing
}
function yellowbird() {
//body
noStroke();
fill(245, 242, 125);
ellipse(0, 0, 50, 50); //head
ellipse(0, 40, 60, 80);
fill(245, 242, 125); //body
push()
rotate(radians(30));
ellipse(48, 30, 10, 60) //tail
// orange section of head
fill(255, 176, 117);
rotate(radians(220));
arc(0, 0, 45, 45, 0, QUARTER_PI);
pop();
//beak
fill(212, 208, 150);
ellipse(4, 0, 10, 10); //right greyish yellow circle
ellipse(-4, 0, 10, 10); //left greyish yellow circle
fill(151, 150, 131);
triangle(-6, 2, 6, 2, 0, 18); //dark greyish yellow beak
//eye
fill(10);
ellipse(10, -2, 7, 7); //right eye
ellipse(-10, -2, 7, 7); //left eye
//wings
fill(160, 212, 150);
ellipse(20, 40, 15, 40); //right green wing
ellipse(-20, 40, 15, 40); //left green wing
}
function rainbow() {
push();
noStroke();
fill(255, 92, 92);
rotate(radians(158));
arc(0, 0, 35, 35, 0, PI+QUARTER_PI, OPEN); //red layer
fill(255, 156, 92);
arc(0, 0, 30, 30, 0, PI+QUARTER_PI, OPEN); //orange layer
fill(255, 245, 92);
arc(0, 0, 25, 25, 0, PI+QUARTER_PI, OPEN); //yellow layer
fill(160, 240, 125);
arc(0, 0, 20, 20, 0, PI+QUARTER_PI, OPEN); //green layer
fill(125, 193, 240);
arc(0, 0, 15, 15, 0, PI+QUARTER_PI, OPEN); //blue layer
fill(175, 125, 240);
arc(0, 0, 10, 10, 0, PI+QUARTER_PI, OPEN); //purple layer
pop();
}
function cloud() {
noStroke();
fill(255);
ellipse(0, 0, 20, 20);
ellipse(-10, 5, 15, 15);
ellipse(12, 4, 17, 17);
ellipse(22, 5, 10, 10); //white ellipses left to right
}
function dots() {
noStroke();
fill(255, 255, 255, 6);
ellipse(0, 0, 5, 5); //dots for background
}
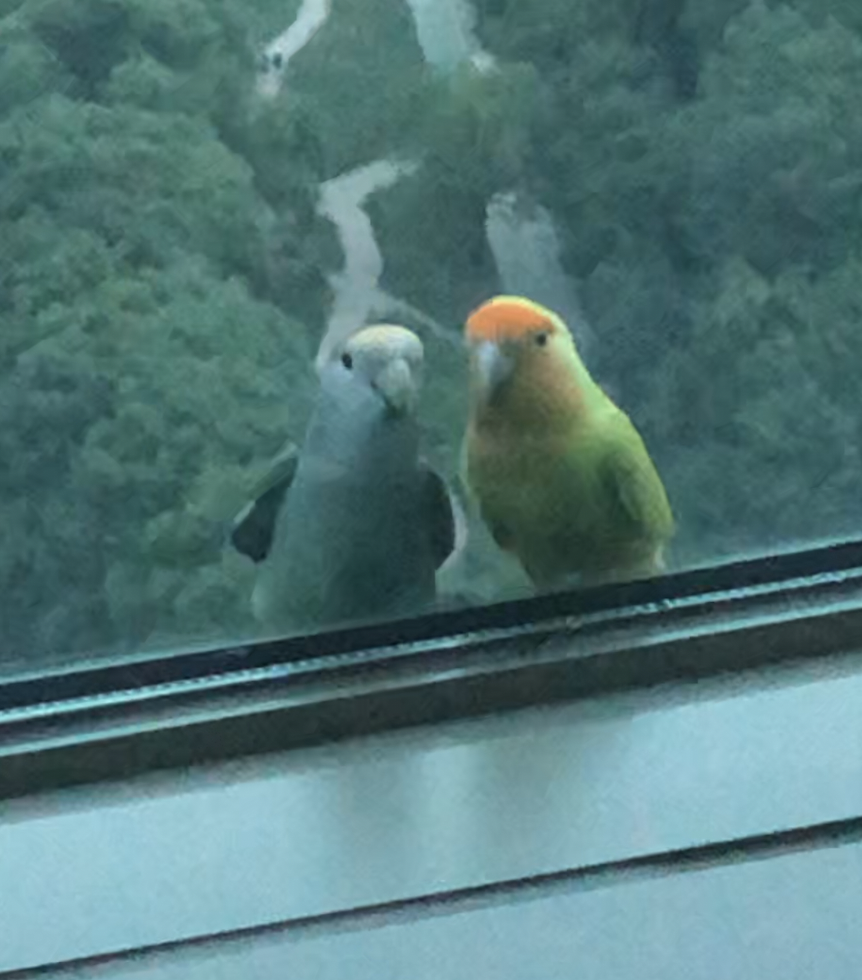