/// <reference path="../TSDef/p5.global-mode.d.ts" />
var lineLength = 75;
var x = 120;
var y = 240;
var branchAngle = 0;
var strokeGreenColor = 255;
var branchThickness = 10;
var bailout = 10;
var cloudScale=[0.5, 0.75, 1, 1.25, 1.5];
var move;
var b=25;
var h;
function setup() {
createCanvas(480, 480);
stroke(0, strokeGreenColor, 0);
strokeWeight(branchThickness);
}
function draw() {
//the color of the sky changes depending on what hour it is, if it's before noon, the color turns bluer and after noon it gets darker
h=hour();
if (h<12){
background(51, 15, 20.8*h);
} else {
background(51, 15, 255-20.8*(h-12));
}
push();
//the clouds are the minute hand, they move to the left depending on what minute it is
move = map(minute(), 0, 60, 0, width);
translate(move, 0)
drawClouds();
pop();
push();
translate(width / 2, height);
translate(0, 120-2*second());
//makes the tree spread out with seconds
branchAngle = map(second(), 0, 60, 1, 100);
drawTree(lineLength);
pop();
}
function drawClouds(){
//smallest cloud--cloud 1
push();
fill(250, 250);
noStroke();
scale(cloudScale[0]);
translate(500,100);
cloud();
pop();
//cloud 2
push();
fill(250, 250);
noStroke();
scale(cloudScale[1]);
translate(100, 250);
cloud();
pop();
//cloud 3
push();
fill(250, 250);
noStroke();
scale(cloudScale[2]);
translate(400, 350);
cloud();
pop();
//cloud 4
push();
fill(250, 250);
noStroke();
scale(cloudScale[3]);
translate(0, 50);
cloud();
pop();
//cloud 5
push();
fill(250, 250);
noStroke();
scale(cloudScale[4]);
translate(-50, 250);
cloud();
pop();
}
function cloud(){
ellipse(30, 10, 60, 40);
ellipse(40, 40, 90, 50);
ellipse(0, 40, 100, 50);
ellipse(0, 15, 55, 35);
}
function drawTree(lineLength) {
//Coloring leaves
if (lineLength < 15) {
strokeGreenColor = map(lineLength, 0, 150, 1, 255);
stroke(0, 150, 0, 150);
strokeWeight(9);
}
//Coloring the branch
if (lineLength > 15) {
strokeGreenColor = map(lineLength, 0, 150, 1, 255);
stroke(139, 69, 19, strokeGreenColor+100);
branchThickness = map(lineLength, 0, 150, 0, 25);
strokeWeight(branchThickness);
}
if (lineLength >= bailout) {
line(0, 0, 0, -lineLength * 2);
translate(0, -lineLength * 2);
//Right branch 1
push();
rotate(radians(branchAngle));
line(0, 0, 0, -lineLength);
drawTree(lineLength * 0.65);
pop();
//Left branch 1
push();
rotate(radians(-branchAngle));
line(0, 0, 0, -lineLength);
drawTree(lineLength * 0.65);
pop();
//Right branch 2
push();
rotate(radians(branchAngle * 0.5));
line(0, 0, 0, -lineLength);
drawTree(lineLength * 0.5);
pop();
//Left branch 2
push();
rotate(radians(-branchAngle * 0.5));
line(0, 0, 0, -lineLength);
drawTree(lineLength * 0.7);
pop();
}
}
For my project I decided to use a tree, clouds, and the sky as my clock. The tree grows and spreads out with what second it is, and I used the map function to change the angle proportionally to the seconds. The clouds move from left to right based on what minute it is, and at each hour the sky changes color. I wanted to use something organic like a plant to represent my clock but I originally sketched a vine, not a tree.
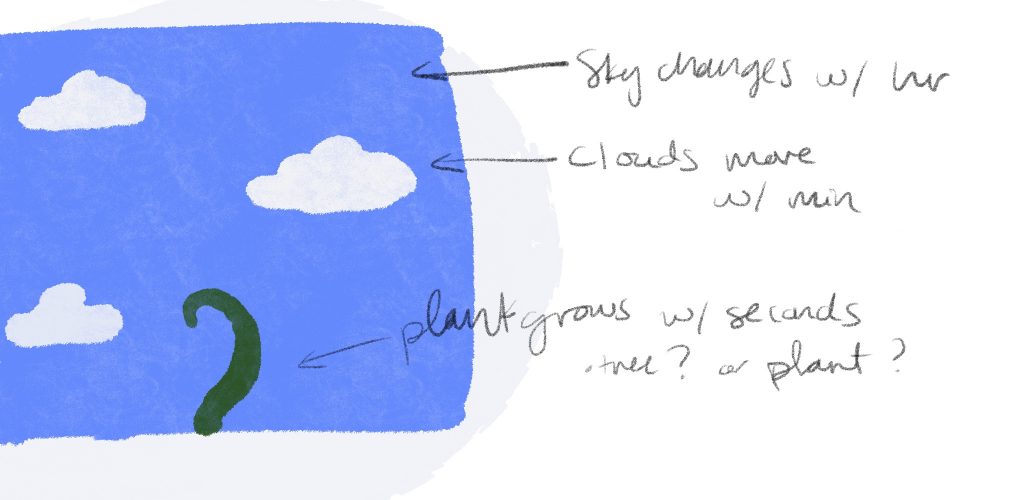
I decided to use a tree after seeing what someone made on GitHub. I was researching how to make plants that show growth in p5.js and found a tree which uses recursion to add branches. I used the same technique and changed the colors so it looked like how I wanted it to.