sketch
var y = 430 //start y position of house
var c = []; //random colors for balloons
var bx = []; //balloon random x coordinate
var by = [];//balloon random y coordinate
var dy = []; // cloud random x coordinate
var dx = []; //cloud random y coordinate
var tx = []; // star random x coordinate
var ty = []; //star random y coordinate
function setup() {
createCanvas(480, 480);
//for every minute, randomly colored and located balloon will pop up
for (i=0; i<= 60; i++){
c[i] = color(random(255), random(255), random(255))
bx[i] = random(160, 320)
by[i] = random((y-30), (y-175))
}
//during the daytime, for every hour randomly located cloud will pop up
for (d = 0; d<=23; d++){
dx[d] = random(25, width-25)
dy[d] = random(25, height-25)
}
//during nighttime, for every hour randomly located star will pop up
for (s=0; s<=23; s++){
tx[s] = random(75, 3*width-25)
ty[s] = random(75, 3*height-25)
}
}
function draw() {
//during nighttime, use dark sky and stars
if (hour() < 12){
background(7, 46, 110);
star();
}
// during daytime, use day sky and clouds
else {
background(111, 165, 252)
cloud();
}
//for every second, house will move up four units
translate(0, -4*second())
house();
}
function house(){
//base house
noStroke();
fill(61, 58, 50)
rect(width/2-28, y-20, 60, 20)
fill(209, 203, 88)
noStroke();
rect(width/2 - 25, y, 25, 50)
fill(240, 240, 144)
square(width/2, y, 50)
triangle(width/2, y, width/2+50, y, width/2 + 25, y-20)
strokeWeight(10)
stroke(84, 84, 66)
line(width/2, y, width/2 +25, y-20)
line(width/2 +50, y, width/2+25, y-20)
rect(width/2 + 22, y+25, 5, 15)
//for each minute, balloon tied to house will pop up
for (i=0; i< minute(); i++){
push();
//balloon string
stroke(255)
strokeWeight(1)
line(width/2, y-20, bx[i], by[i])
//balloons
fill(c[i]);
noStroke()
ellipse(bx[i], by[i], 20)
print(i)
pop();
}
}
function cloud(){
//for each hour, cloud will pop up
for (var d = 0; d<hour(); d++){
fill(255);
strokeWeight(3)
stroke(200)
ellipse(dx[d], dy[d], 100, 20);
ellipse(dx[d] + 20, dy[d] - 10, 40, 15)
}
}
function star(){
//for each hour, star will pop up
for (var s = 0; s<hour(); s++){
push();
stroke(255, 223, 107)
strokeWeight(3)
fill(255, 255, 217)
beginShape();
scale(0.3)
vertex(tx[s]+35, ty[s]+3)
vertex(tx[s] +46, ty[s]+22)
vertex(tx[s]+68, ty[s]+28)
vertex(tx[s] + 54, ty[s] +45)
vertex(tx[s] + 56,ty[s] + 67)
vertex(tx[s]+35, ty[s]+58)
vertex(tx[s] + 14, ty[s]+67)
vertex(tx[s] + 16, ty[s]+45)
vertex(tx[s]+2, ty[s]+28)
vertex(tx[s]+24, ty[s]+22)
endShape(CLOSE);
pop()
}
}
Over quarantine, I rewatched a bunch of Pixar movies, and was inspired by the movie “Up” for my clock. The house moves upwards for every second, the balloons show the minutes, and the clouds or stars in the background show the hour. In general, I had difficulty making the arrays refresh with the time.
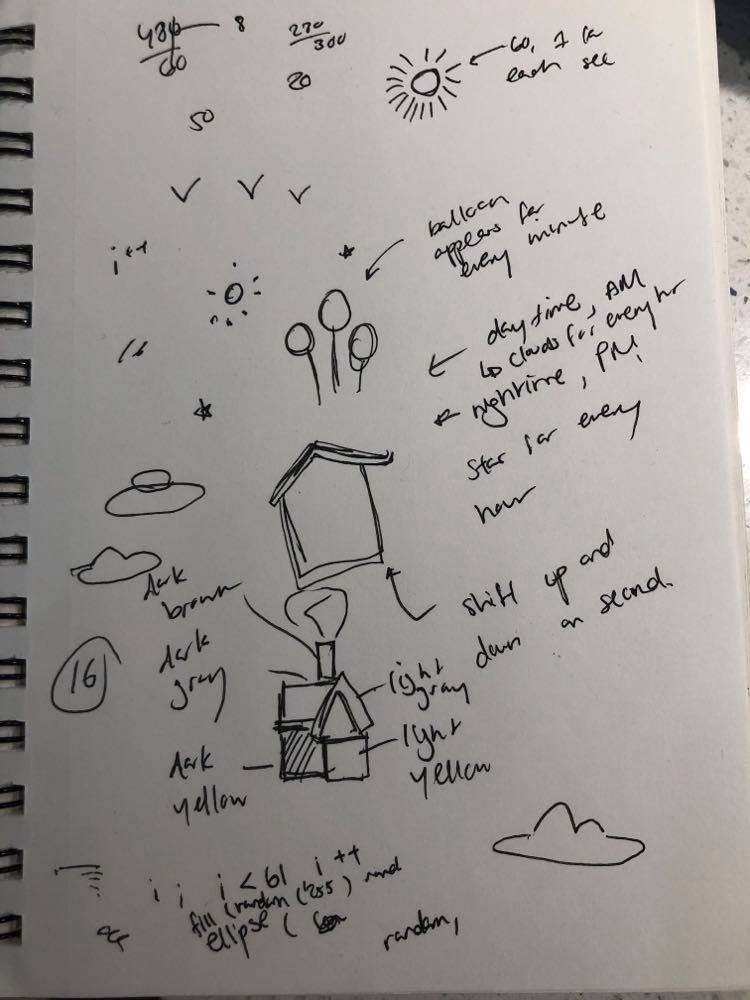