For this project, I wanted to draw a single object but in different ways. I took the functions from the website provided in the project brief and decided to draw a heart by plugging in functions for “x” and “y” and using the “beginShape()” function. I first began by using a “for loop” to draw a circle by creating a local variable that adds in increments for every loop. By altering this variable, I was able to draw the heart in different ways.
Click to change colors:
sketch
function setup() {
createCanvas(400, 480);
background(220);
text("p5.js vers 0.9.0 test.", 10, 15);
}
function draw() {
background(0);
translate(width / 2, height / 2);
//heart one
push();
translate(-100, -130);
noFill();
strokeWeight(0.5);
stroke(255);
beginShape();
for(var a = 0; a < TWO_PI; a += 0.3) { //this function draws a circle before the equation is put in
if(mouseIsPressed){
stroke(random(255), random(255), random(255)); //color change
}
//drawing the first heart
var r = constrain(mouseX / 180, 1, 70); //movement of mouse
var x = r * 16 * pow(sin(a), 3);
var y = -r * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(x, y);
//drawning the second heart
var b = r/2 * 16 * pow(sin(a), 3);
var c = -r/2 * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(b, c);
}
endShape(CLOSE);
pop();
//heart two
push();
translate(100, -130);
noFill();
strokeWeight(0.5);
stroke(255);
beginShape();
for(var a = 0; a < TWO_PI; a += 0.2) {
if(mouseIsPressed){
stroke(random(255), random(255), random(255)); //color change
}
//drawing the first heart
var r = constrain(mouseX / 180, 1, 70); //movement of mouse
var x = r * 16 * pow(sin(a), 3);
var y = -r * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(x, y);
//drawing the second heart
var b = r/2 * 16 * pow(sin(a), 3);
var c = -r/2 * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(b, c);
}
endShape(CLOSE);
pop();
//heart three
push();
translate(-100, 0);
noFill();
strokeWeight(0.5);
stroke(255);
beginShape();
for(var a = 0; a < TWO_PI; a += 0.1) {
if(mouseIsPressed){
stroke(random(255), random(255), random(255)); //color change
}
//drawing the first heart
var r = constrain(mouseX / 180, 1, 70);
var x = r * 16 * pow(sin(a), 3);
var y = -r * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(x, y);
//drawing the second heart
var b = r/2 * 16 * pow(sin(a), 3);
var c = -r/2 * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(b, c);
}
endShape(CLOSE);
pop();
//heart four
push();
translate(100, 0);
noFill();
strokeWeight(0.5);
stroke(255);
beginShape();
for(var a = 0; a < TWO_PI; a += 0.08) {
if(mouseIsPressed){
stroke(random(255), random(255), random(255)); //color change
}
//drawing the first heart
var r = constrain(mouseX / 180, 1, 70);
var x = r * 16 * pow(sin(a), 3);
var y = -r * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(x, y);
//drawing the second heart
var b = r/2 * 16 * pow(sin(a), 3);
var c = -r/2 * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(b, c);
}
endShape(CLOSE);
pop();
//heart five
push();
translate(-100, 130);
noFill();
strokeWeight(0.5);
stroke(255);
beginShape();
for(var a = 0; a < TWO_PI; a += 0.06) {
if(mouseIsPressed){
stroke(random(255), random(255), random(255)); //color change
}
//drawing the first heart
var r = constrain(mouseX / 180, 1, 70);
var x = r * 16 * pow(sin(a), 3);
var y = -r * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(x, y);
//drawing the second heart
var b = r/2 * 16 * pow(sin(a), 3);
var c = -r/2 * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(b, c);
}
endShape(CLOSE);
pop();
//heart six
push();
translate(100, 130);
noFill();
strokeWeight(0.5);
stroke(255);
beginShape();
for(var a = 0; a < TWO_PI; a += 0.04) {
if(mouseIsPressed){
stroke(random(255), random(255), random(255)); //color change
}
//drawing the first heart
var r = constrain(mouseX / 180, 1, 70);
var x = r * 16 * pow(sin(a), 3);
var y = -r * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(x, y);
//drawing the second heart
var b = r/2 * 16 * pow(sin(a), 3);
var c = -r/2 * (13 * cos(a) - (5 * cos(2 * a)) - 2 * cos(3 * a) - cos(4 * a));
vertex(b, c);
}
endShape(CLOSE);
pop();
}
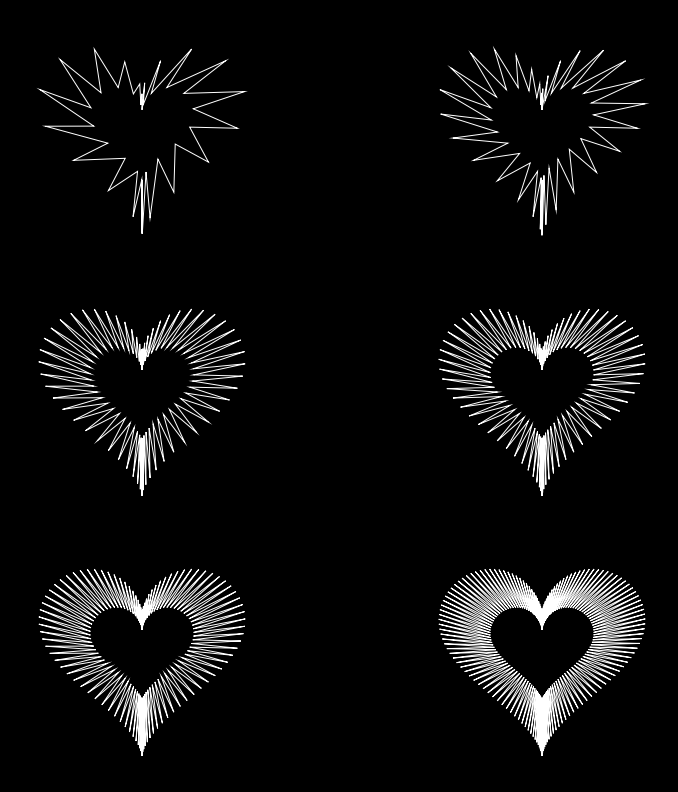
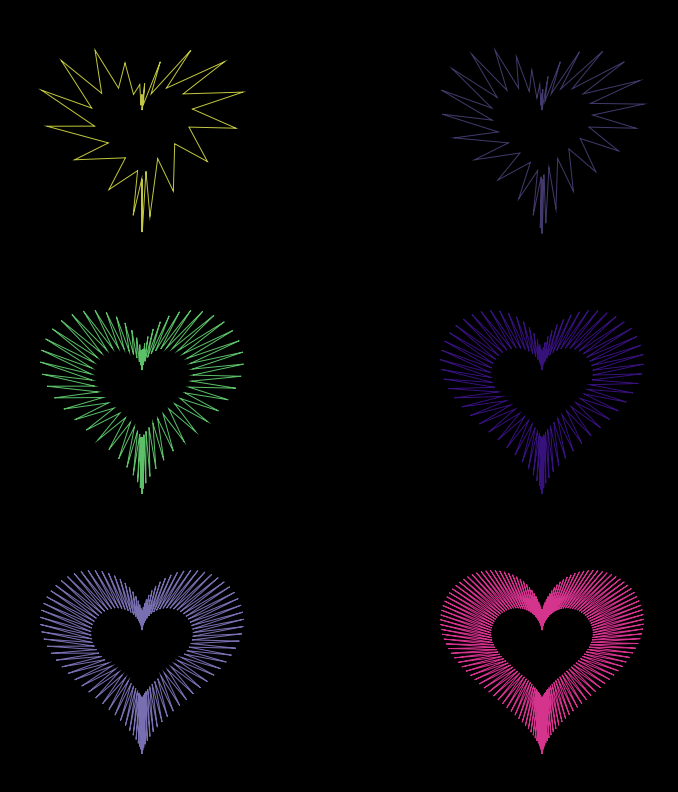