// Sarah Luongo
// sluongo
// sluongo@andrew.cmu.edu
// Project
/* This code aims to create the curve, butterfly curve, as explained on Wolfram
MathWorld. In addition to drawing the curve I played around with the fill color
and stroke depending on the X position of the mouse. */
// Setups canvas size and speed
function setup() {
createCanvas(480, 480);
frameRate(10);
}
// Draws the butterfly curve
function draw() {
var red = random(255); // Random shade of red
var green = random(255); // Random shade of green
var blue = random(255); // Random shade of blue
background(0); // Black background
translate(width/2, height/1.64); // Translate to the "center" of canvas
rotate(PI); // Rotate 180 degrees
if (mouseX < width/3) {
fill(red, green, blue); // Random fill color
stroke(0); // Black
// Draws butterfly curve 4 times
for (var i = 0; i < 4; i++) {
drawButterflyCurve(i);
}
} else if (mouseX >= width/3 & mouseX < 2*(width/3)) {
noFill();
stroke(red, green, blue); // Random stroke color
// Draws butterfly curve 4 times
for(var i = 0; i < 4; i++) {
drawButterflyCurve(i*2); // i*2 makes size of curve bigger
}
} else if (mouseIsPressed) {
background(225, 200, 255); // Purple background
noFill();
stroke(0); // Black
drawButterflyCurve(1); // To see original curve
} else {
noStroke();
fill(red, green, blue); // Random fill color
// Draws butterfly curve 4 times
for(var i = 0; i < 4; i++) {
drawButterflyCurve(i/3); // i/3 makes size of curve smaller
}
}
}
// Butterfly Curve
// https://mathworld.wolfram.com/ButterflyCurve.html
function drawButterflyCurve(j) {
var da = .01; // How round the "wings" are
var a = map(mouseX, 0, width, 0, width/9); // Constrain x size of butterfly curve
var b = map(mouseY, 0, height, 0, width/8); // Constrain y size of butterfly curve
// Creates the butterfly curve
beginShape();
for (var t = 0; t <= 24*PI; t += da) {
// The parametric equations found on the website commented above
var r = exp(cos(t))-2*cos(4*t)-pow(sin(t/12), 5);
var x = a * sin(t) * r * j;
var y = b * cos(t) * r * j;
vertex(x, y);
}
endShape();
}
When I saw this curve on Wolfram MathWorld, I was super excited because I had attempted to draw a butterfly in my last project. I think this curve is way cooler because there’s a lot more curves and more going on. So, it wasn’t just a boring (although I don’t find it so boring) Butterfly Curve, I added some if statements to duplicate the curves, change colors of the fill and stroke, but if this is too much for you you can also click the mouse in the bottom right to get the normal curve. Also, moving the mouse up and down and side to side within each if statement changes the size of the curves. If you move it left to right within a third of the width it kind of looks like the butterfly is flapping it’s wings.
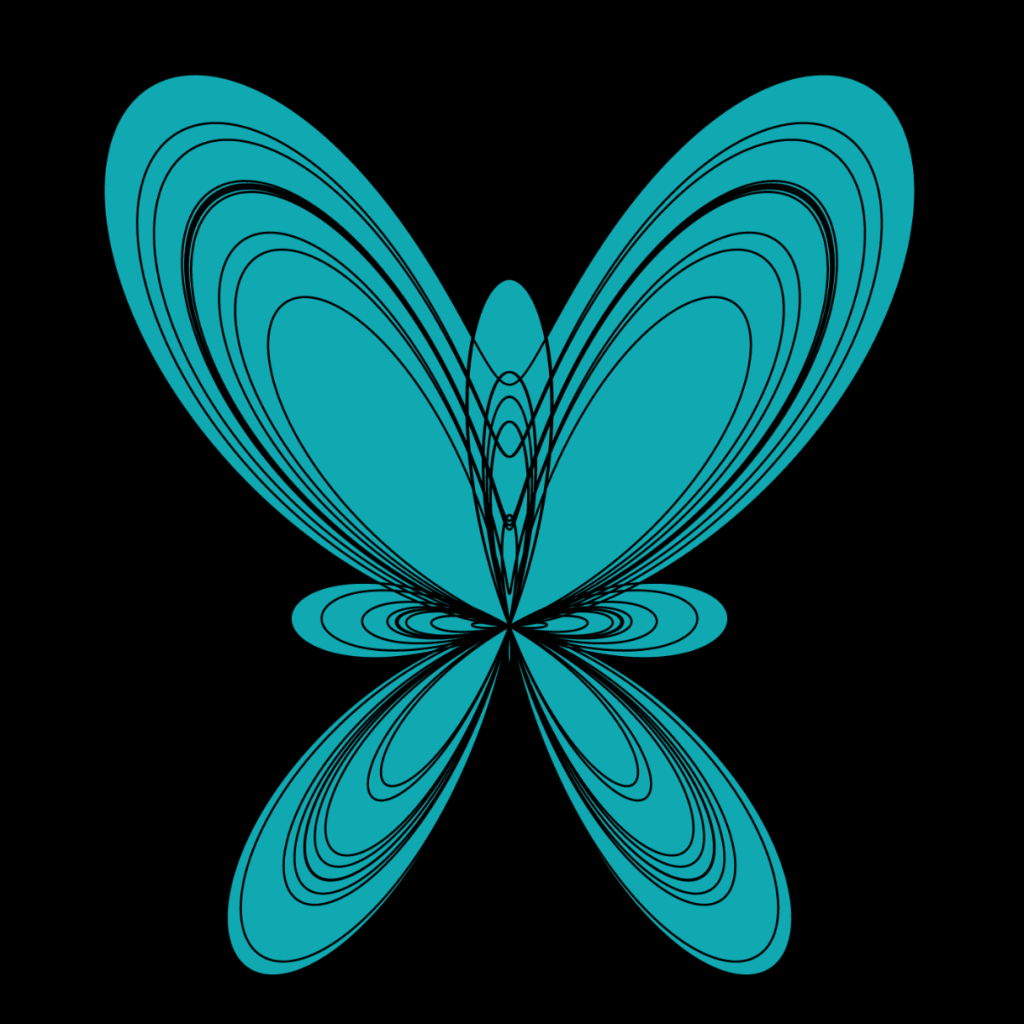
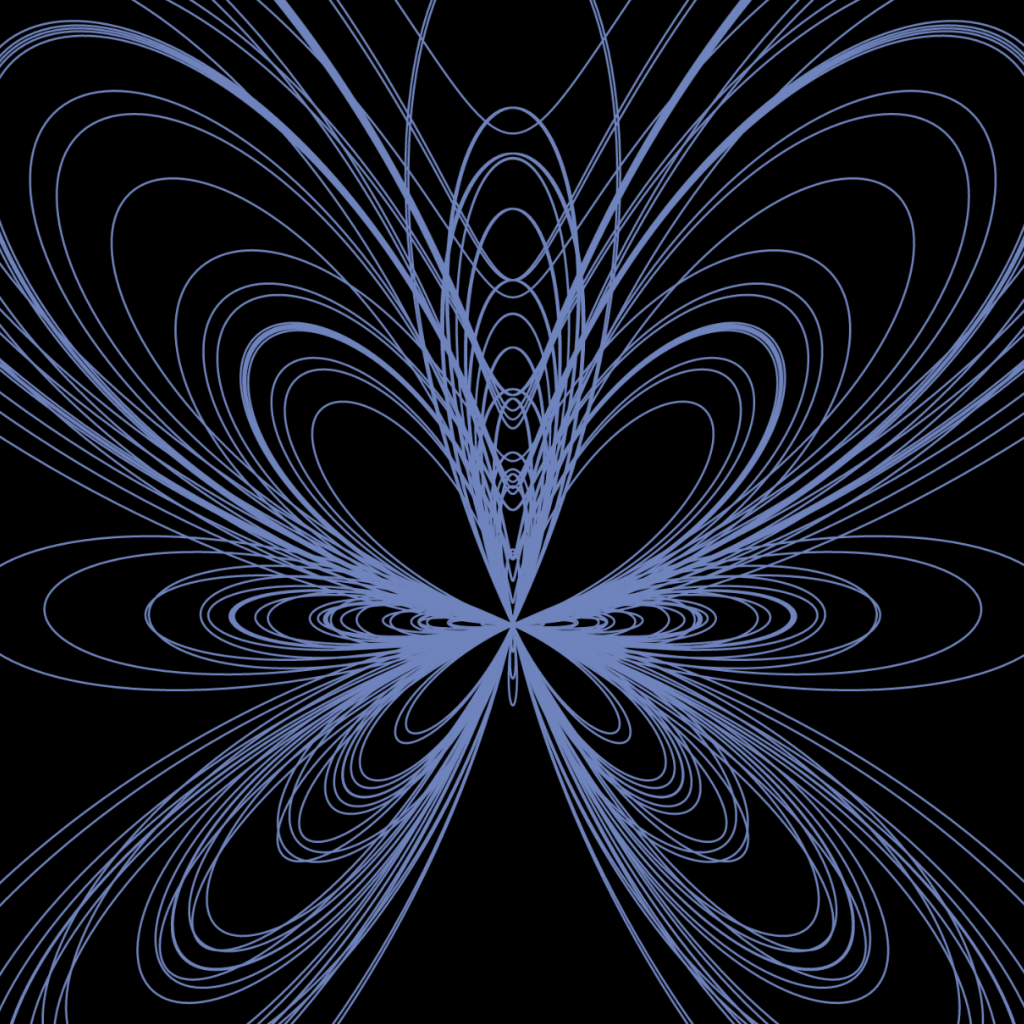
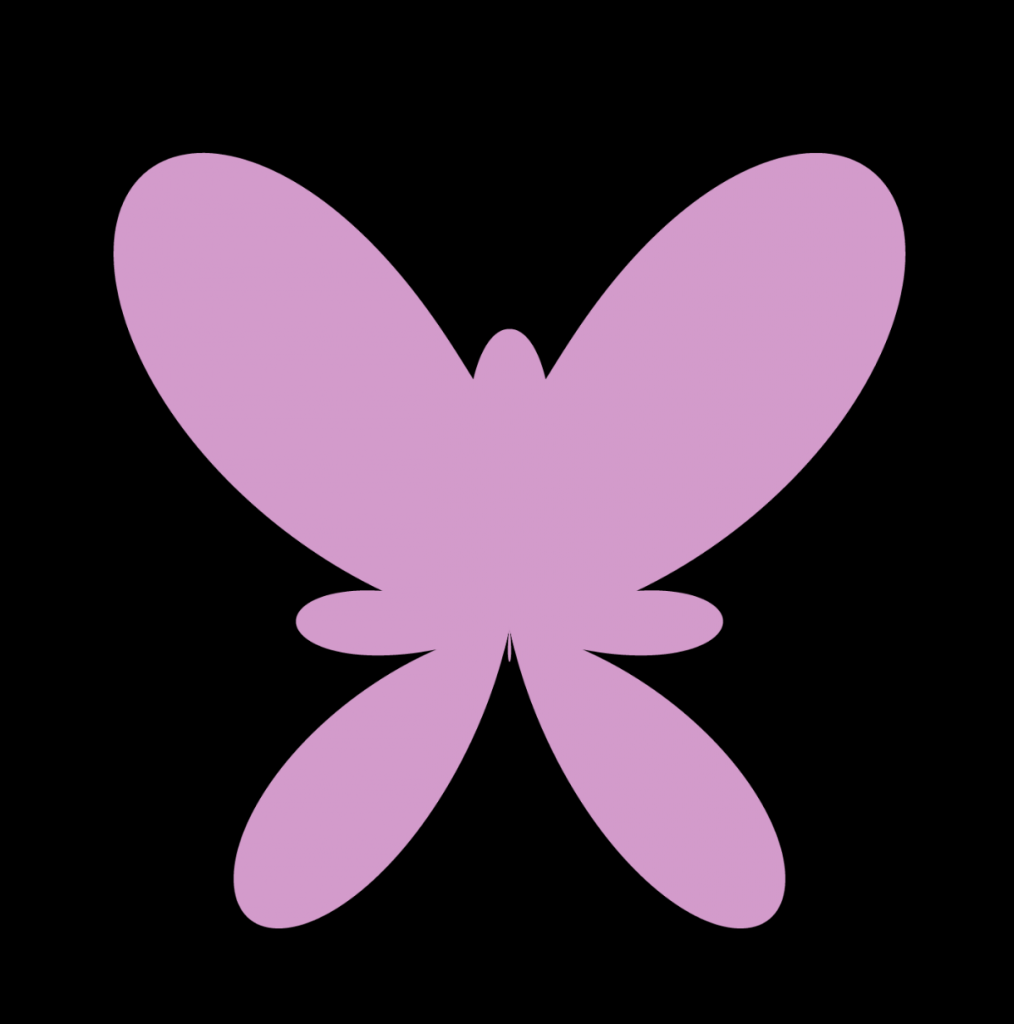
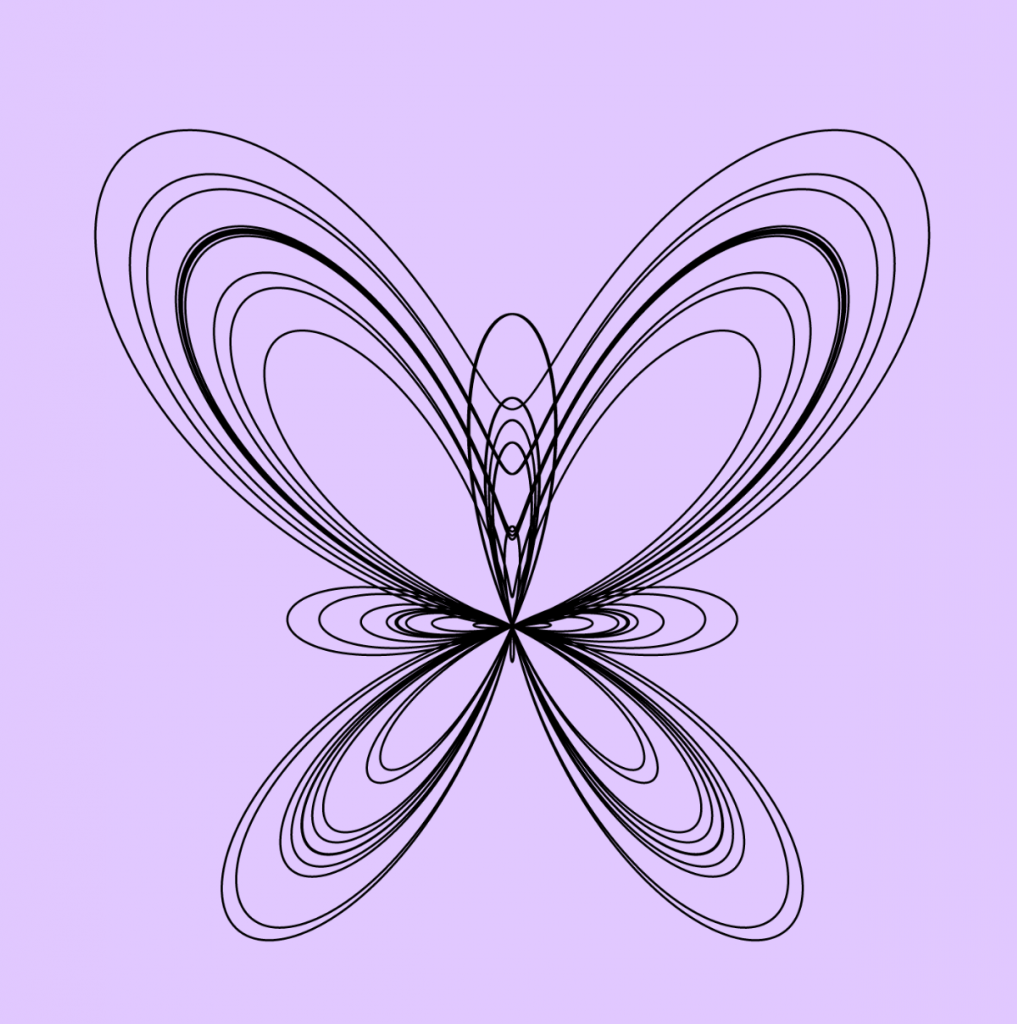