For my project, I wanted to incorporate these curves so that it served a purpose to the image. I did not want to simply create curves and just submit random shapes. I thought that the curve I wanted to use would suit the appearance of fireworks very well. It was difficult at first to figure out how to create these fireworks because I originally wanted them to burst and then disappear/fall down as real fireworks do. However, I decided to just have the fireworks growing and changing colors because I wanted the curves to constantly be seen. I based my project off of a cartoon image of a Fourth of July fireworks celebration, and that is why I put a person on a picnic blanket on the grass so that it would make more sense. I think the easiest part for me was making the city skyline and the person’s body because I have continuously layered shapes in all my projects. I think the most difficulty I had was creating the fireworks, while also making them different enough so that it actually seemed like real unpredictable fireworks. As mouseX or mouseY moves, colors of the fireworks can change, and the size and patterns move based on the mouse movement.
//Annie Kim
//anniekim@andrew.cmu.edu
//SectionB
//anniekim-07-project ~ CURVES ~
xStar = []; //array for stars
yStar = [];
function setup() {
createCanvas(480, 440);
background(10, 23, 45); //dark blue sky
for (i = 0; i < 60; i ++) {
xStar[i] = random(0, 480); //randomly placing stars
yStar[i] = random(0, 240);
}
frameRate(10);
}
function draw() {
//moon
fill(245);
noStroke();
circle(360, 50, 40);
fill(10, 23, 45);
circle(350, 50, 40);
var s = second(); //stars popping up every second
for (i = 0; i < s; i ++) {
fill(255);
ellipse(xStar[i], yStar[i], 2, 2); //stars
}
//orange OR yellow firework
push();
translate(250, 80);
if (mouseX > 240) { //mouseX right half -> yellow
stroke(218, 218, 100);
} else { //mouseX left half -> orange
stroke(218, 100, 100);
}
firework1();
pop();
//purple or pink firework
push();
translate(450, 45);
if (mouseY < 240) { //mouseY top half -> pink
stroke(135, 105, 170);
} else { //mouseY bottom half -> purple
stroke(105, 85, 255);
}
firework2();
pop();
//blue firework
push();
translate(30, 50);
stroke(136, 194, 223);
firework3();
pop();
//pink and red firework
push();
translate(200, 200);
if (mouseX < 240) { //mouseX left half -> pink
stroke(180, 70, 180);
} else { //mouseX right half -> red
stroke(255, 120, 150);;
}
firework4();
pop();
//green or dark green firework
push();
translate(440, 180);
if (mouseX > 240) { //mouseX right half -> dark green
stroke(155, 165, 130);
} else {
stroke(205, 255, 180);
}
firework5();
pop();
//~~~~~CITY~~~~~
//buildings in black
fill(0);
noStroke();
rect(0, 260, 480, 40); //base
rect(0, 220, 20, 80);
rect(145, 200, 15, 100);
rect(225, 230, 15, 70);
rect(310, 200, 25, 100);
//city buildings FILLED BY YELLOW
fill(250, 250, 160);
stroke(0);
strokeWeight(8);
rect(20, 240, 30, 60);
rect(50, 210, 25, 90);
rect(75, 230, 20, 70);
rect(95, 250, 30, 50);
rect(125, 220, 20, 80);
rect(160, 215, 20, 85);
rect(180, 200, 20, 100);
rect(200, 200, 25, 100);
rect(240, 250, 30, 50);
rect(270, 230, 40, 70);
rect(335, 220, 15, 80);
rect(350, 240, 30, 60);
rect(380, 190, 20, 110);
rect(400, 220, 20, 80);
rect(420, 230, 40, 70);
rect(460, 260, 20, 40);//rightmost building
//START OF WINDOW MAKING(separated by building in code)
strokeWeight(8);
stroke(0);
//building #1
line(62, 215, 62, 300);
line(55, 230, 70, 230);
line(55, 245, 70, 245);
line(55, 263, 70, 263);
line(55, 280, 70, 280);
//building #2
strokeWeight(4);
line(270, 240, 310, 240);
line(270, 250, 310, 250);
line(270, 260, 310, 260);
line(270, 270, 310, 270);
line(270, 280, 310, 280);
line(270, 290, 310, 290);
//building #3
line(410, 220, 410, 295);
line(400, 240, 420, 240);
line(400, 260, 420, 260);
line(400, 280, 420, 280);
//building #4
line(425, 230, 425, 295);
line(430, 230, 430, 295);
line(435, 230, 435, 295);
line(440, 230, 440, 295);
line(445, 230, 445, 295);
line(450, 230, 450, 295);
line(455, 230, 455, 295);
line(420, 250, 460, 250);
line(420, 270, 460, 270);
line(420, 290, 460, 290);
//building #5
strokeWeight(6);
line(180, 215, 200, 215);
line(180, 225, 200, 225);
line(180, 235, 200, 235);
line(180, 245, 200, 245);
line(180, 255, 200, 255);
line(180, 265, 200, 265);
line(180, 275, 200, 275);
line(180, 285, 200, 285);
//building #6
strokeWeight(3);
line(200, 232, 225, 232);
line(200, 267, 225, 267);
line(212.5, 200, 212.5, 295);
//END OF WINDOW MAKING
//grass
fill(42, 83, 47);
noStroke();
rect(0, 299, 480, 141);
//picnic blanket
fill(220, 125, 145); //pink color
stroke(185, 100, 100); //darker pink color outline
quad(150, 340, 310, 340, 330, 400, 130, 400); //blanket
//PERSON SITTING ON BLANKET
//arms
fill(230, 200, 170);
noStroke();
rect(170, 340, 8, 30);
rect(212, 340, 8, 30);
//pants
fill(104, 104, 55);
noStroke();
ellipse(185, 380, 45, 25);
ellipse(205, 380, 45, 25);
//shirt
fill(234, 234, 131);
noStroke();
rect(177.5, 313, 35, 57);
ellipse(195, 369, 38, 9);
rect(170, 320, 10, 20);
rect(210, 320, 10, 20);
//shoulders
circle(177.5, 321, 15);
circle(212.5, 321, 15);
//sleeve lines
stroke(200, 200, 100);
strokeWeight(2);
line(178, 327, 178, 340);
line(212, 327, 212, 340);
//neck
fill(200, 170, 140);
noStroke();
rect(190, 305, 10, 8);
//head shape
fill(230, 200, 170);
ellipse(195, 295, 23, 28);
//hair
fill(80, 45, 25);
ellipse(195, 285, 25, 20);
ellipse(191, 290, 20, 30);
triangle(195, 288, 207, 288, 195, 303);
//ear
fill(230, 200, 170);
stroke(200, 180, 140);
strokeWeight(1);
ellipse(200, 295, 4, 7);
}
function firework1() { //yellow-orange firework
var x;
var y;
var a = map(mouseY, 0, height, 0, 20);
var h = map(mouseY, 0, height, 0, 20);
var b = a / 4;
var angle = 360;
beginShape();
for (var i = 0; i < angle; i ++) {
strokeWeight(0.2);
noFill();
var q = map(i, 0, 180, 0, TWO_PI);
x = (a - b) * cos(q) + (h) * cos(((a + b)/ b) * q);
y = (a - b) * sin(q) + (h) * sin(((a + b)/ b)* q);
vertex(x, y);
}
endShape(CLOSE);
}
function firework2() { //purple-pink firework
var x;
var y;
var a = map(mouseY, 0, height, 0, 35);
var h = map(mouseY, 0, height, 0, 35);
var b = a / 8;
var angle = 360;
beginShape();
for (var i = 0; i < angle; i ++) {
strokeWeight(0.2);
noFill();
var q = map(i, 0, 180, 0, TWO_PI);
x = (a - b) * cos(q) + h * cos(((a + b)/ b) * q / 2);
y = (a - b) * sin(q) + h * sin(((a + b) / b) * q / 2);
vertex(x, y);
}
endShape(CLOSE);
}
function firework3() { //blue firework
var x;
var y;
var a = map(mouseX, 0, 240, 0, 20);
var h = map(mouseY, 0, 240, 0, 20);
var b = a / 15;
var angle = 360;
beginShape();
for (var i = 0; i < angle; i ++) {
strokeWeight(0.08);
noFill();
var q = map(i, 0, 180, 0, TWO_PI);
x = (a - b) * cos(q) + h * cos(((a + b)/ b) * q);
y = (a - b) * sin(q) + h * sin(((a + b) / b) * q);
vertex(x, y);
}
endShape(CLOSE);
}
function firework4() { //pink-red firework
var x;
var y;
var a = map(mouseX, 0, 400, 0, 50);
var h = map(mouseY, 0, 400, 0, 40);
var b = a / 20;
var angle = 360;
beginShape();
for (var i = 0; i < angle; i ++) {
strokeWeight(0.2);
noFill();
var q = map(i, 0, 180, 0, TWO_PI);
x = (a - b) * cos(q) + h * cos(((a + b)/ b) * q);
y = (a - b) * sin(q) + h * sin(((a + b) / b) * q);
vertex(x, y);
}
endShape(CLOSE);
}
function firework5() { //green-dark green firework
var x;
var y;
var a = map(mouseX, 0, 240, 0, 18);
var h = map(mouseX, 0, 240, 0, 18);
var b = a / 7;
var angle = 360;
beginShape();
for (var i = 0; i < angle; i ++) {
strokeWeight(0.4);
noFill();
var q = map(i, 0, 180, 0, TWO_PI);
x = (a - b) * cos(q) + h * cos(((a + b)/ b) * q);
y = (a - b) * sin(q) + h * sin(((a + b) / b) * q);
vertex(x, y);
}
endShape(CLOSE);
}
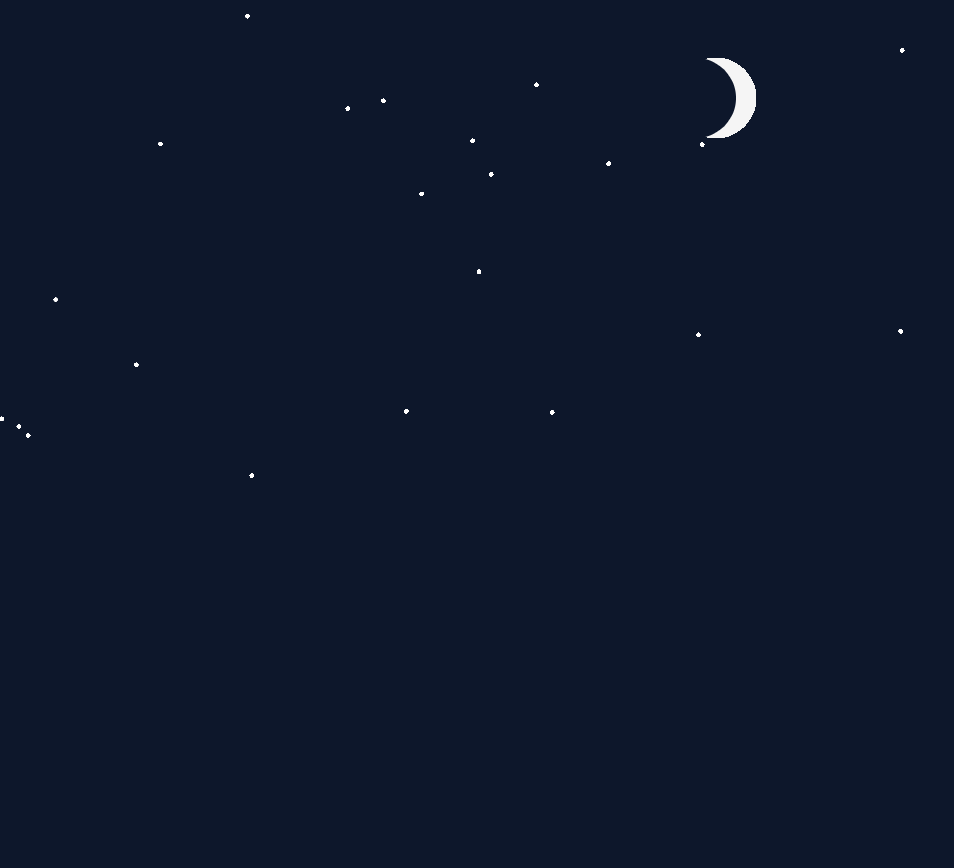
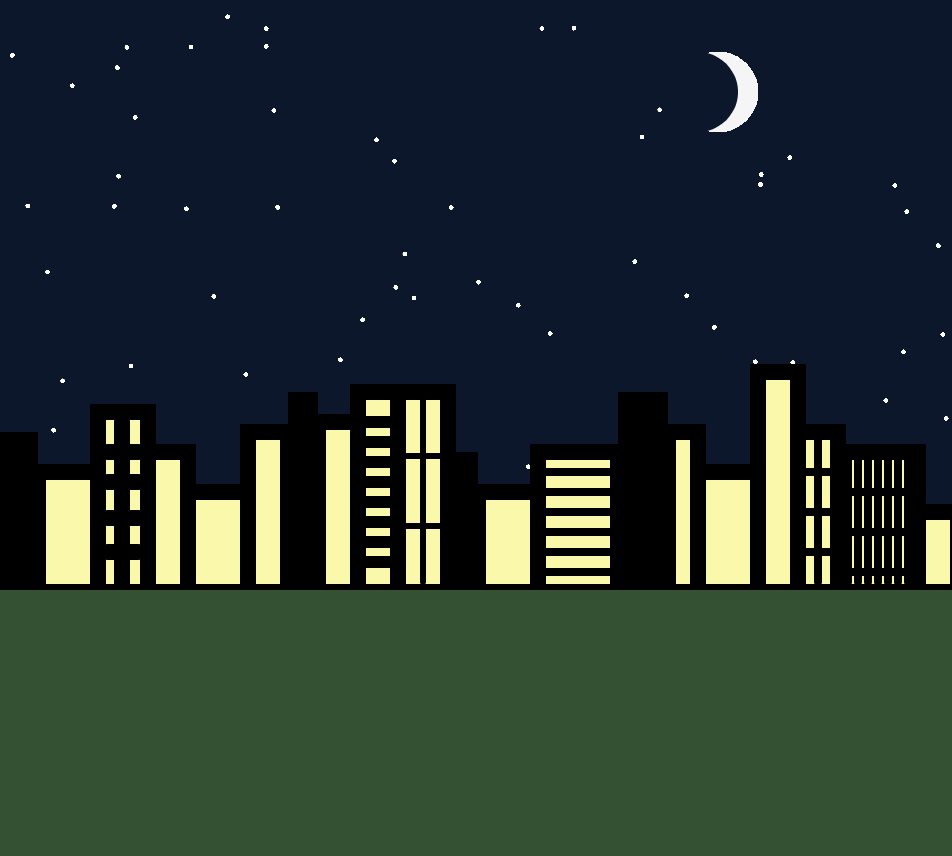
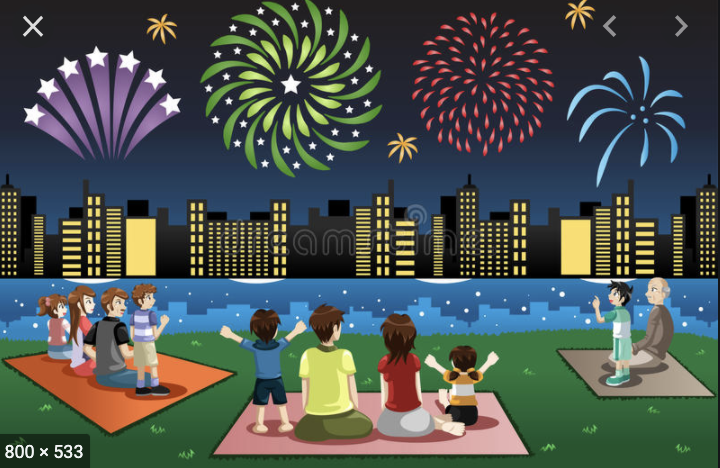