HCP
//Hayoon Choi
//hayoonc
//Section C
let img;
var click = 0; //initial click status
function preload() {
img = loadImage('https://i.imgur.com/5Ka0n6z.jpg?1');
}
function setup() {
createCanvas(330, 440);
imageMode(CENTER);
noStroke();
background(255);
img.loadPixels();
}
function draw() {
//pointilizing the picture
let pointillize = map(mouseX, 0, width, 3, 7);
let x = floor(random(img.width));
let y = floor(random(img.height));
let pix = img.get(x, y);
fill(pix);
if (click == 0){ //drawing pumpkin initially
pumpkin(x, y, pointillize, pointillize);
} else if (click == 1){ //drawing ghost after one click
ghost(x, y, pointillize, pointillize);
} else { //drawing bat after two clicks
bat(x, y, pointillize, pointillize);
}
}
function pumpkin(x, y){
//drawing the pumpkin
push();
translate(x, y);
rect(5, -10, 2, 6, 70);
ellipse(12, 0, 5, 9);
ellipse(0, 0, 5, 9)
ellipse(3, 0, 5, 11);
ellipse(9, 0, 5, 11);
ellipse(6, 0, 5, 12);
pop();
}
function ghost(x, y){
//drawing the ghost
push();
translate(x, y);
ellipse(0, 0, 9, 10);
ellipse(-5, 3, 5, 2);
ellipse(5, 3, 5, 2);
beginShape();
curveVertex(-4.5, 0);
curveVertex(-4.5, 0);
curveVertex(-3, 4);
curveVertex(-1, 6);
curveVertex(2, 9);
curveVertex(6, 11);
curveVertex(5, 8);
curveVertex(4, 4);
curveVertex(4.5, 0);
curveVertex(4.5, 0);
endShape();
pop();
}
function bat(x, y){
//drawing the bat
push();
translate(x, y);
ellipse(0, 0, 7, 10);
wing(); //calling the right wing
push()
scale(-1, 1); //flipping the wing to draw the left wing
wing();
pop();
triangle(2, -3, 1.9, -6, 0, -4.6);
triangle(-2, -3, -1.9, -6, 0, -4.6);
pop();
}
function wing(){
//drawing the wing
beginShape();
curveVertex(3, -2);
curveVertex(3, -2);
curveVertex(6, -2);
curveVertex(11, -3);
curveVertex(13, 0);
curveVertex(11, 0);
curveVertex(10, 0.3);
curveVertex(10, 2);
curveVertex(8, 1);
curveVertex(6.7, 0.7);
curveVertex(6, 2);
curveVertex(5, 1);
curveVertex(4.3, 0.7);
curveVertex(3.6, 0.9);
curveVertex(3, 2);
curveVertex(3, 2);
endShape();
}
function mousePressed() {
//making the shapes to change after mouse click
if (click == 2) {
click = 0;
} else {
click += 1;
}
if (mouseButton === RIGHT) { //right click restarts the process
background(255);
}
}
Since it’s Halloween, I decided to make all shapes Halloween related. The shapes change when the mouse clicks on the canvas and it restarts the process if the mouse right clicks.
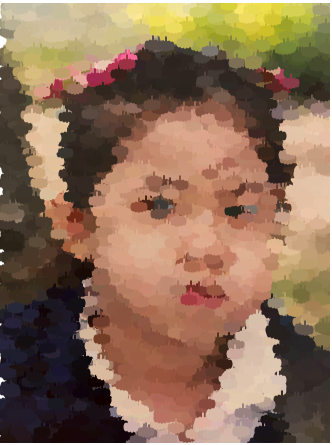
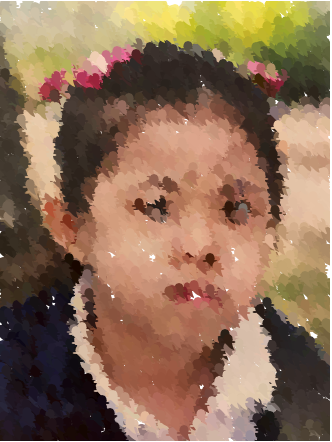
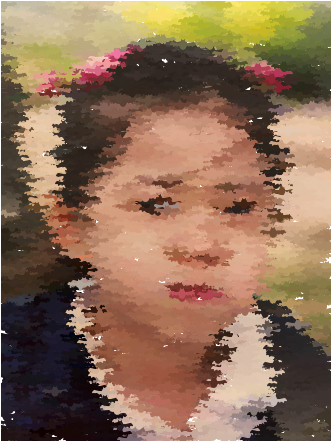