For this week’s project, I decided to abstractly depict a photo of my friend and I using the idea of a bitmap and pixels. I was inspired by various 8-bit art pieces that I had found online, and wanted to make my own interactive interpretation!
Depending on the mouse’s position, you can move around to see the pixels bigger, which ‘magnifies’ and makes clearer whatever part you are toggling over! Additionally, if you click the up and down arrow, you can see the “bitmap pixels” increase or decrease in number, respectively!
sketchDownload
// Susie Kim
// susiek@andrew.cmu.edu
// Section A
// Project 09
// set variables
var img;
var bitMap;
var grid = 19;
// load image
function preload() {
var imgURL = "https://i.imgur.com/7Vy4Fqz.jpg";
img = loadImage(imgURL);
}
function setup() {
createCanvas(480, 380);
background(255);
img.resize(480, 380); // resize image to be canvas size
img.loadPixels(); // load image pixels
}
function draw() {
background(255);
drawBits(); // call upon drawBits function
}
function drawBits() {
noStroke();
rectMode(CENTER);
// make grid of rectangles, with each growing bigger or smaller depending on mouse position
for (x = 0; x < 480; x += grid) {
for (y = 0; y < 380; y += grid) {
var dis = dist(mouseX, mouseY, x, y); // measure distance between mouse pos and each square bit
var bitMap = map(dis, 0, 480, 11+(grid*.05), 3+(grid*.05)); // map width of each rectangle bit dependent on distance
var bitColor = img.get(x,y); // get color for each rectangle bit
fill(bitColor);
rect(x, y, bitMap+(grid*.35), bitMap+(grid*.35)); // draw grid of rectangles with width adjusting
}
}
}
function keyPressed() {
// lock maximum "pixel" size at 23
if (grid > 23) {
grid = 23;
}
// lock minimum "pixel" size at 15
if (grid < 15) {
grid = 15;
}
// make "pixels" bigger with each click of up arrow, and smaller with click of down arrow
if (keyCode === UP_ARROW) { // bigger
clear();
grid += 1;
} else if (keyCode === DOWN_ARROW) { // smaller
clear();
grid -= 1
}
}
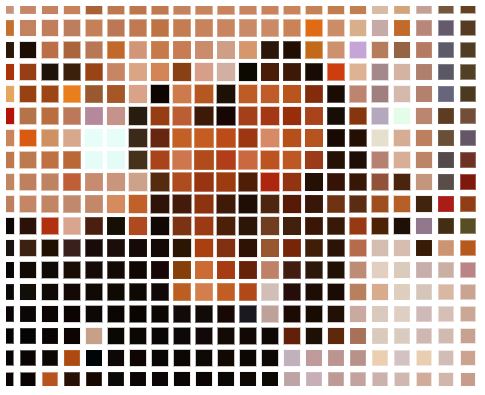
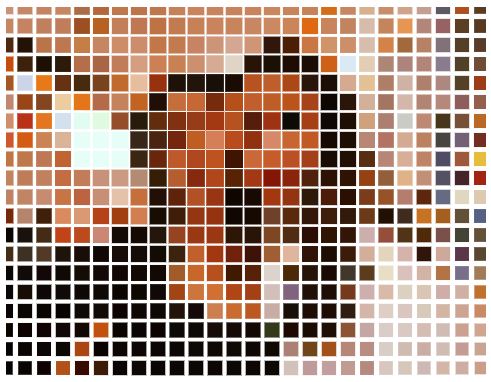
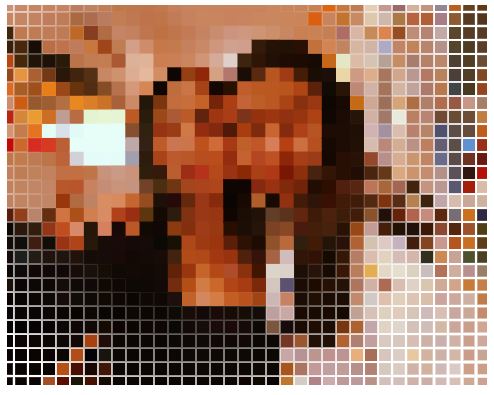
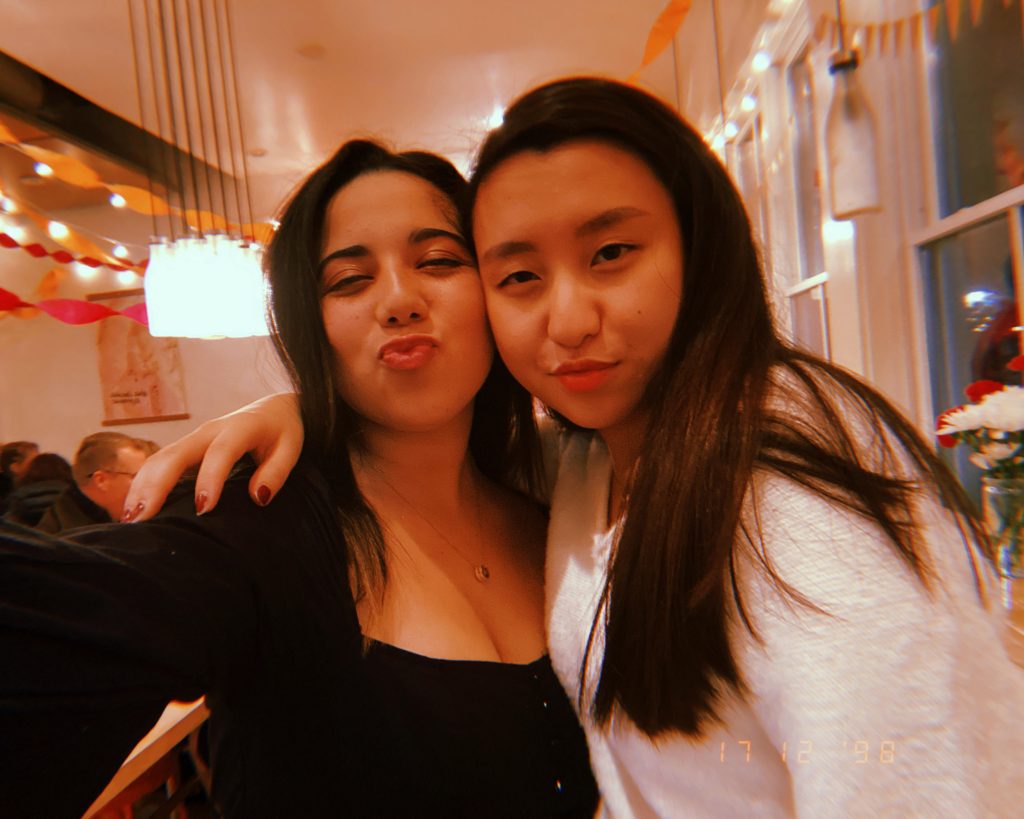