For my final project, I wanted to create a game that presents a picture at the end with hidden images that represent events of 2020. The user is able to click two images to switch the locations of the images, and therefore unscramble the original scrambled image that loads. In order not to pressure the user or make the user create one “right answer”, I chose not to tell the user when the image is “correctly” unscrambled. Therefore, the game acts more like a pressure-free artistic adaptation of an image if they’d like.
// Susie Kim
// Final Project
// susiek@andrew.cmu.edu
// Section A
// set global variables
var imgLinks = [
"https://i.imgur.com/vGhpX7m.jpg", //1
"https://i.imgur.com/bNtGvLH.jpg", //2
"https://i.imgur.com/cWHfsai.jpg", //3
"https://i.imgur.com/hnFyXIy.jpg", //4
"https://i.imgur.com/06xo9FF.jpg", //5
"https://i.imgur.com/FJbeTn2.jpg", //6
"https://i.imgur.com/HZduFcW.jpg", //7
"https://i.imgur.com/fU2goSM.jpg", //8
"https://i.imgur.com/i4BVpfk.jpg", //9
"https://i.imgur.com/dvCAEzm.jpg", //10
"https://i.imgur.com/t7FzJVW.jpg", //11
"https://i.imgur.com/MVcrDnK.jpg", //12
"https://i.imgur.com/QUBppYU.jpg", //13
"https://i.imgur.com/IPIsgqD.jpg", //14
"https://i.imgur.com/Inkq9Nc.jpg", //15
"https://i.imgur.com/FJotQ09.jpg"]; //16
gridRows = 4;
gridCols = 4;
sqSize = 100;
images = [];
puzzles = [];
ids = [];
shuffledIds = [];
clickedImgs = [];
numClicks = 0;
// preload image links into images array
function preload() {
for (var i=0; i < 16; i++) {
images[i] = loadImage(imgLinks[i]);
}
}
function setup() {
createCanvas(500, 500);
background(145, 168, 209);
// write instructions text ontop and bottom
fill(255);
text('complete the puzzle to see the image :)', 150, 35);
text('click 2 pictures to switch their location!', 150, 475);
push();
// write instructions text on left and right
translate(250, 250);
rotate(radians(90));
text('2020 in review', -35, 225);
rotate(radians(180));
text('2020 in review', -35, 225);
pop();
// create a set of shuffled ids:
for (var i = 0; i < 16; i++) {
ids[i] = i;
}
shuffledIds = shuffle(ids);
// make 16 objects with object properties
for (var i = 0; i < gridRows; i++) {
for (var j = 0; j < gridCols; j++) {
puzzles[j+i*4] = makePuzzle(50+j*100, 50+i*100, shuffledIds[j+i*4], j+i*4);
}
}
}
function draw() {
// draw the grid of shuffled images
for (var i = 0; i < gridRows; i++) {
for (var j = 0; j < gridCols; j++) {
puzzles[j+i*4].display();
}
}
}
// create an object that holds the images' properties
function makePuzzle(xlocation, ylocation, idNum, idNum2) {
var makeImg = { x1: xlocation,
y1: ylocation,
shuffId: idNum,
origId: idNum2,
display: makeImage }
return makeImg;
}
// function that creates the image
function makeImage() {
image(images[this.shuffId], this.x1, this.y1, 100, 100);
}
// if mouse is clicked on two images, switch them
function mouseClicked() {
for (var i = 0; i < 16; i++) {
if (mouseX > puzzles[i].x1 & mouseX < puzzles[i].x1+100 && // boundaries of any image i
mouseY > puzzles[i].y1 && mouseY < puzzles[i].y1+100) {
clickedImgs.push(puzzles[i].origId); // push original Id value of clicked image into clickedImgs array
numClicks += 1;
}
}
// if 2 images are clicked, swap, and reset clicknum to 0
if (numClicks == 2) {
swapImages();
numClicks = 0;
}
}
// function that swaps the two images
function swapImages() {
// store the x and y values in a temporary variable
var tempX = puzzles[clickedImgs[0]].x1;
var tempY = puzzles[clickedImgs[0]].y1
// switch the x and y values
puzzles[clickedImgs[0]].x1 = puzzles[clickedImgs[1]].x1;
puzzles[clickedImgs[0]].y1 = puzzles[clickedImgs[1]].y1;
puzzles[clickedImgs[1]].x1 = tempX;
puzzles[clickedImgs[1]].y1 = tempY;
// clear the clickedImgs array
clickedImgs.pop();
clickedImgs.pop();
}
Some examples of imagery I used was a basketball for Kobe Bryant’s death, a fire printed bag for the California and Australia wildfires, a tiger poster for Tiger King, and an Oscar for Parasite winning Best Picture.
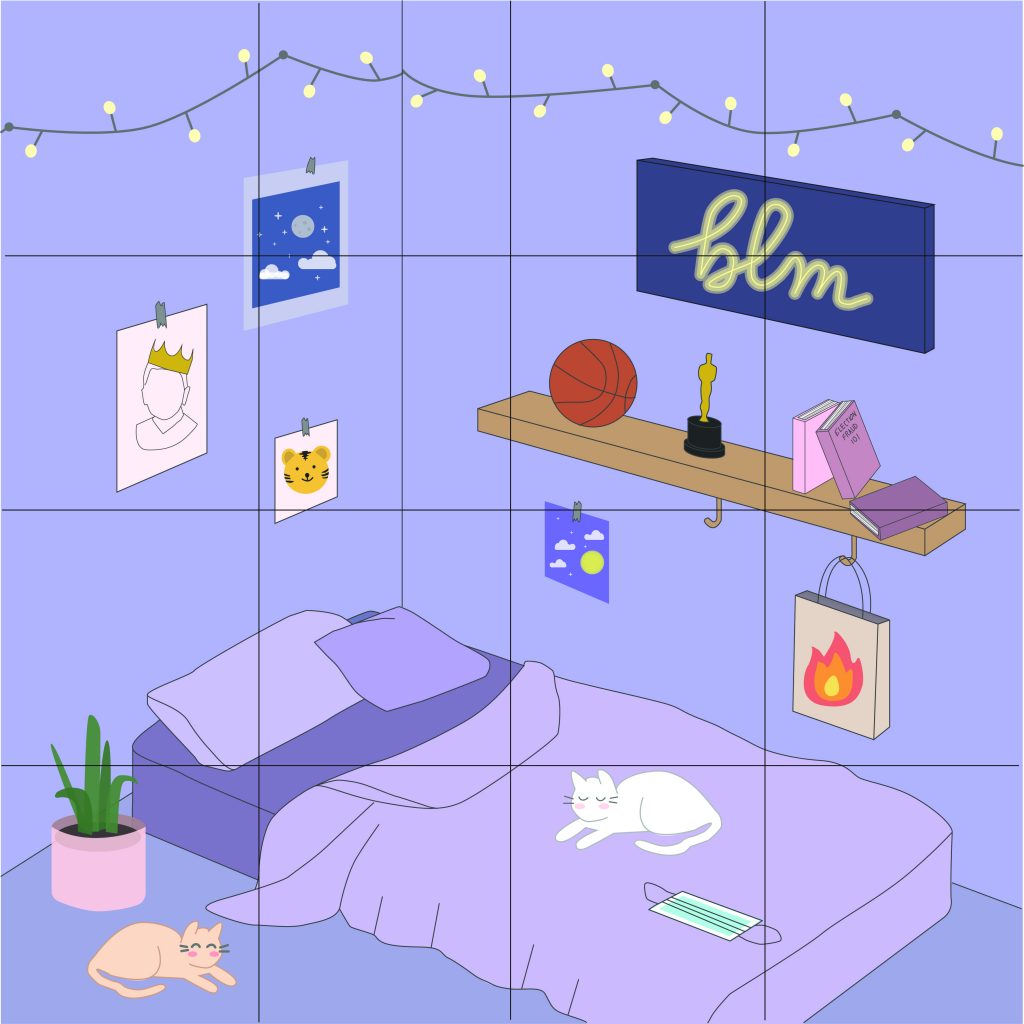