For this week’s project, I really wanted to get away from the craziness of the semester and portray the calming view from the beach house my cousins and I used to go to. I have really vivid memories of sitting on the porch, eating fruit while watching the fireflies and water wade in and out at nighttime: something I wish I could be doing right now.
sketchDownload
// Susie Kim
// susiek@andrew.cmu.edu
// Section A
// Project 11
// set global variables
var landSpeed = 0.001;
var landFlow = 0.005;
var firefly = [];
var fWidth = 50;
var fLeft = 27 - fWidth;
// load in images for background
function preload() {
feet = loadImage('https://i.imgur.com/VLK5brn.png');
fruit = loadImage('https://i.imgur.com/NNwEjtA.png');
cloud = loadImage('https://i.imgur.com/m6zIO0u.png');
}
function setup() {
createCanvas(400, 480);
background(29, 51, 81);
imageMode(CENTER);
// create fireflies at random locations on canvas
for (var i = 0; i < 7; i++) {
var xLocation = random(20, 380);
var yLocation = random(0, 360);
firefly[i] = makeFireflies(xLocation, yLocation);
}
}
function draw() {
background(29, 51, 81);
image(cloud, width/2, height/2-65, width-20, height-20); // background cloud image
// blue water
fill(147, 169, 209);
noStroke();
rect(0, 200, 400, 200);
// call upon functions to draw land, foreground, and update fireflies
land();
createForeground();
updateFirefly();
}
// draws front foreground porch, feet, and fruit dish
function createForeground() {
fill(188, 158, 130);
strokeWeight(1);
stroke(124, 87, 61);
// create porch base
rect(0, 360, 400, 120);
rect(0, 350, 400, 10);
rect(0, 0, 30, 355);
rect(370, 0, 30, 355);
// insert self-drawn images of feet laying on porch and fruit
image(feet, 100, 405, 150, 150);
image(fruit, 300, 410, 125, 125);
}
// draws undulating sand landscape
function land() {
fill(249, 228, 183);
stroke(255);
strokeWeight(8);
beginShape();
vertex(0, height);
// undulating line of sandscape
for (var x = 0; x < width; x++) {
var a = (x*landFlow) + (millis()*landSpeed);
var y = map(noise(a), 0, 1, height*12/20, height*11/20);
vertex (x, y);
}
vertex(width, height);
endShape();
}
// draws fireflies
function drawFirefly() {
fill(255, 245, 0, 100);
noStroke();
push();
translate(this.x1, this.y1); // allows fireflies to move depending on function
scale(this.fireflySize); // allows fireflies to be scalable depending on make function
ellipse(50, 50, 10, 10);
ellipse(50, 50, 5, 5);
pop();
}
// summons fireflies at given location with variables
function makeFireflies(xlocation, ylocation) {
var makeFirefly = {x1: xlocation,
y1: ylocation,
fireflySize: random(0.25, 2),
speed: -1,
move: moveFirefly,
draw: drawFirefly}
return makeFirefly;
}
// makes fireflies move across the page
function moveFirefly() {
this.x1 += this.speed;
if (this.x1 <= fLeft) {
this.x1 += width - fLeft;
}
}
// updates the array of fireflies
function updateFirefly() {
for (i = 0; i < firefly.length; i++) {
firefly[i].move();
firefly[i].draw();
}
}
Here is the main sketch that I did for this project:
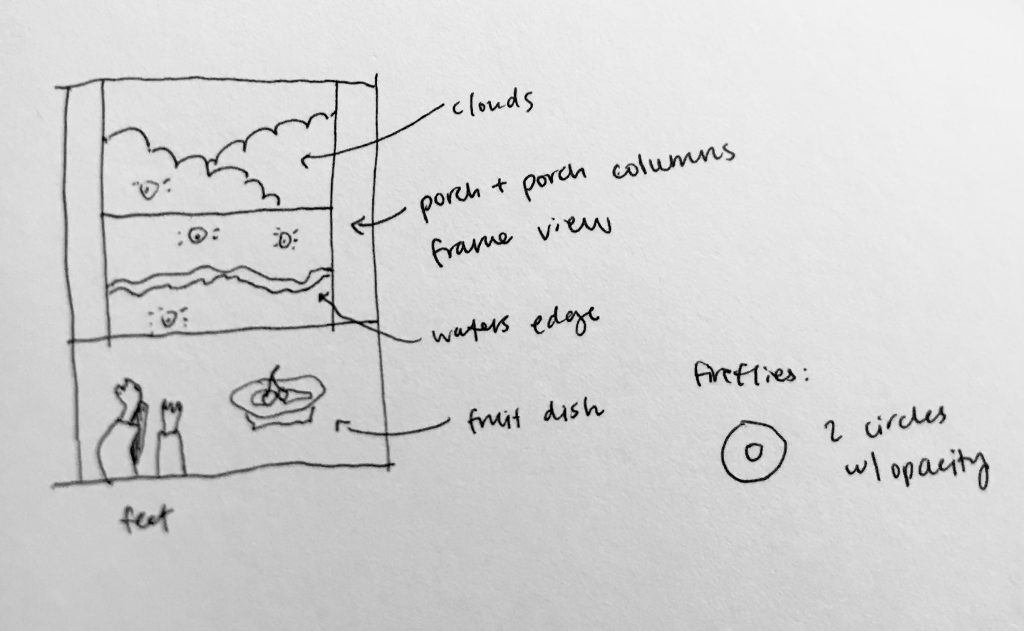