I really enjoyed creating this even though it took me a while to understand objects, I feel the birds and pagoda along with the colour tones were extremely soothing!
sketch
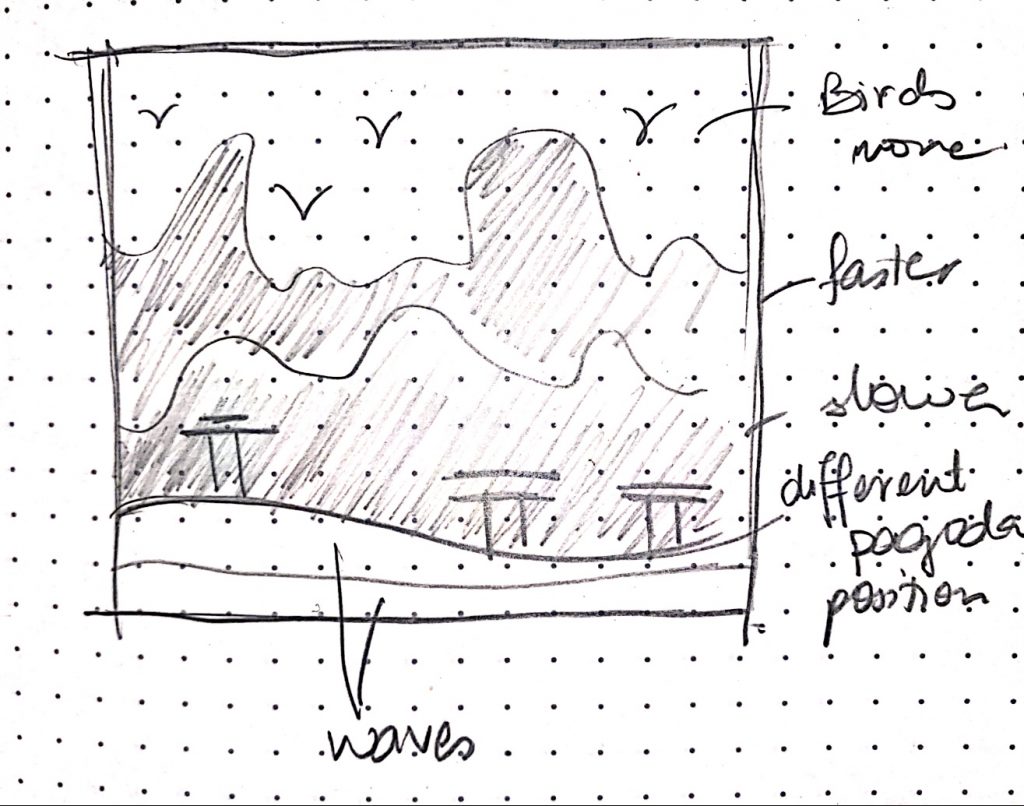
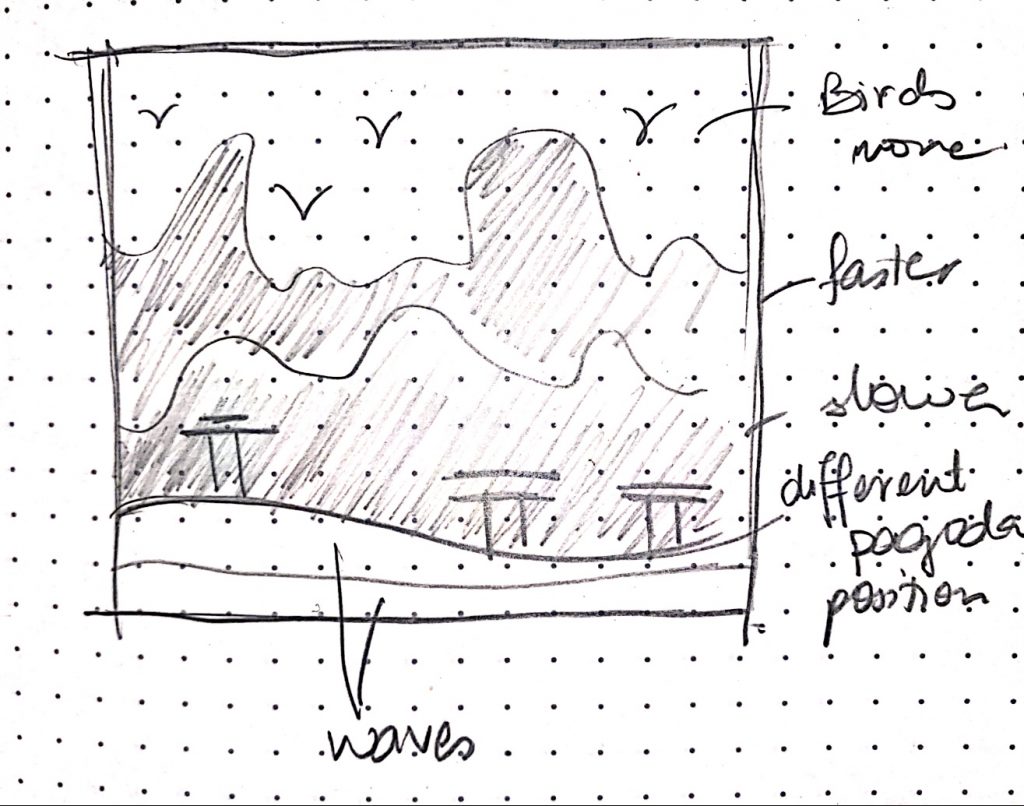
//Aadya BHartia
//Section A
//abhartia@andrew.cmu.edu
/* the program shows a river and mountains as well as pagodas, the birds and pagodas along with the
variation in landscape help the visuals become more interesting and dynamic */
//variables to store colurs
var c1;
var c2;
//storing birds in an array
var birds = [];
//pagoda in an array
var pagodas = [];
//water speed
var waterSpeed = 0.0001;
function setup() {
createCanvas(400, 400);
c1 = color(218, 239, 252);
c2 = color(240, 198, 196);
frameRate(15);
//create an initial collection of birds
for(var i = 0; i<5; i++){
var birdX = random(width);
var birdY = random(0, height/3);
birds[i] = drawBird(birdX, birdY);
}
//create an initial collection of pagodas
for(var k = 0; k<2; k++){
var pagodaX = random(width);
var pagodaY = random(250, 300);
pagodas[k] = drawPagoda(pagodaX, pagodaY);
}
}
function draw() {
gradient(c1, c2);
drawMountain2(); //background mountains
drawMountain();//foreground mountains
//pagoda update functions
pagodaUpdate();
pagodaAppear();
pagodaDisappear();
//water level
drawWater();
drawWater2();
//birds in the sky
birdUpdate();
birdAppear();
birdDisappear();
}
//background gradient from blue to light orange
function gradient(c1,c2){
noFill();
noStroke();
for(var i = 0; i<height; i++){
var a = map(i, 0, height, 0, 1);
var c = lerpColor(c1,c2,a);
stroke(c);
line(0, i, width, i);
}
}
//function to display water in foreground
function drawWater(){
var water1 = 0.001;
var speedW1 = 0.0003;
noStroke();
fill(189, 210, 222);
beginShape();
for(var i = 0; i<width; i++){
var x = i*water1 + millis()*speedW1;
var y = map(noise(x), 0, 1, 260, 300);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function drawWater2(){
//function to display second shade water in foreground
var water2 = 0.001;
var speedW2 = 0.0001;
fill(163, 198, 218);
beginShape();
for(var i = 0; i<width; i++){
var x = i*water2 + millis()*speedW2;
var y = map(noise(x), 0, 1, 290, 350);
vertex(i, y);
}
vertex(width, height);
vertex(0, height);
endShape();
}
function drawMountain(){
var mount1 = 0.014;
var speedM1 = 0.0008;
//foreground mountain
stroke(249, 224, 226);
beginShape();
for(var i = 0; i<width; i++){
var x = i*mount1 + millis()*speedM1;
var y = map(noise(x), 0,1.5,height/6, height);
line(i, y, i, height);
}
endShape();
//shading for foreground mountain
for(var k = width/2; k<4*width/5; k++){
var z = map(k, width/2,4*width/5,0, 255);
stroke(246, 188, 192,z);
line(0, k, width, k);
}
}
function drawMountain2(){
var mount2 = 0.009;
var speedM2 = 0.001;
//background mountain
stroke(246, 188, 192);
beginShape();
for(var i = 0; i<width; i++){
var x = i*mount2 + millis()*speedM2;
var y = map(noise(x), 0,1.5,20, 2*height/3);
line(i, y, i, height);
}
endShape();
//shading for background mountain
for(var k = width/4; k<2*width/3; k++){
var z = map(k, width/4,2*width/3,0, 255);
stroke(249, 224, 226,z);
line(0, k, width, k);
}
}
//pagodas
function pagodaMove(){
//update speed of every pagoda
this.x1 -= this.speed;
}
function pagodaMake(){
noFill();
stroke(80);
strokeWeight(2);
//making the pagoda
line(this.x1, (this.y1+50) , this.x1, (this.y1-40));
line(this.x1+30, (this.y1+50) , this.x1 +30, (this.y1-40));
strokeWeight(4);
line(this.x1, (this.y1-35), this.x1+30, (this.y1-35));
strokeWeight(6);
line(this.x1-5, (this.y1-42), this.x1+35, (this.y1-42));
}
function pagodaUpdate(){
// Update the pagodas's positions, and display them.
for(var i = 0; i< pagodas.length; i++){
pagodas[i].shift();
pagodas[i].display();
}
}
function pagodaAppear(){
//with a tiny probablilty adding pagodas
if(random(0,1)<0.007){
var pagodaX = width;
var pagodaY = random(250, 300 );
pagodas.push(drawPagoda(pagodaX, pagodaY));
}
}
function pagodaDisappear(){
//removing pagodas which have reached the edge
var pagodasKeep = [];
for(var i = 0; i<pagodas.length; i++){
if(pagodas[i].x1 + pagodas[i].bredth > 0){
pagodasKeep.push(pagodas[i]);
}
}
pagodas = pagodasKeep;
}
function drawPagoda(pagodaX, pagodaY){
var pagoda = { x1: pagodaX, y1: pagodaY,
speed: 4, bredth: 30,
shift: pagodaMove, display: pagodaMake}
return pagoda;
}
//birds
function drawBird(birdX, birdY){
var bird = { x: birdX, y: birdY,
velocity: random(4,8), size: random(6,10),
move: birdMove, show: birdMake}
return bird;
}
function birdMove(){
//update speed of every birds
this.x -= this.velocity;
this.y -= this.velocity/random(5,10);
}
function birdMake(){
noFill();
stroke(80);
strokeWeight(1);
arc(this.x, this.y, this.size, this. size/2, PI, TWO_PI);
arc(this.x+ this.size, this.y, this.size, this. size/2 ,PI, TWO_PI);
}
function birdUpdate(){
// Update the birds's positions, and display them.
for(var i = 0; i< birds.length; i++){
birds[i].move();
birds[i].show();
}
}
function birdAppear(){
//with a tiny probablilty adding birds
if(random(0,1)<0.1){
var birdX = width;
var birdY = random(0, height/3 );
birds.push(drawBird(birdX, birdY));
}
}
function birdDisappear(){
//removing birds which have reached the edge
var birdsKeep = [];
for(var i = 0; i<birds.length; i++){
if(birds[i].x + birds[i].size > 0){
birdsKeep.push(birds[i]);
}
}
birds = birdsKeep;
}