“Flight Patterns” by Aaron Koblin explores the cultural trends and relationships between humans and technology with the use of U.S. flight data. He used data from the U.S. Federal Aviation Administration which resulted in an animation that shows the frequent routes in popular western cities and traffic over certain geographical areas. I was initially drawn to this piece because I had initially thought it was randomly generated from its close up shots, but it was when the image zoomed out, did I see it in its entirety. This phantom like quality mixed with various colors and patterns illustrates a wide range of aircraft events and results in a mesmerizing depiction of our country. I enjoyed this time-lapse animation of the American air-traffic patterns because it parallels with our idea of what a recorded time lapse footage of these flight patterns may look like in real life.
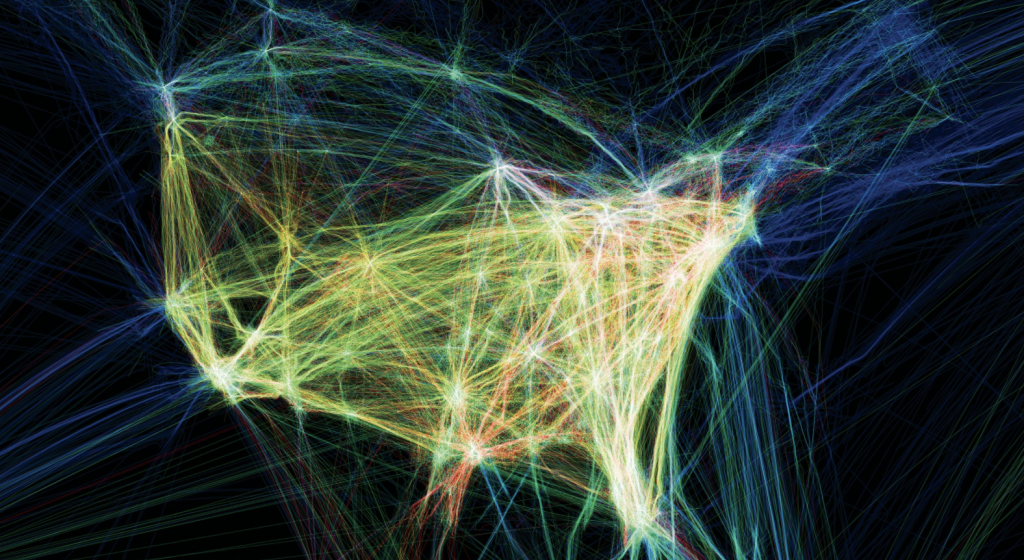