function setup() {
createCanvas(600, 600);
noLoop();
}
function draw() {
background(249, 220, 180);
//grid pattern background
for (var x = 0; x <= width; x += 20) {
for (var y = 0; y <= height; y += 20) {
push();
translate(x, y);
grid();
pop();
}
}
//columns of 4 eggs
for (var x = 56; x <= 560; x += 164) {
for (var y = 72; y <= 522; y += 150) {
push();
translate(x, y);
rotate(radians(-20));
egg();
pop();
}
}
//columns of 3 eggs
for (var x = 140; x <= 470; x += 164) {
for (var y = 150; y <= 450; y += 150) {
push();
translate(x, y);
rotate(radians(30));
scale(.85);
egg();
pop();
}
}
//columns of 3 bacons
for (var x = 50; x <= 546; x += 164) {
for (var y = 144; y <= 450; y += 150) {
push();
translate(x, y);
rotate(radians(20));
scale(.5);
bacon();
pop();
}
}
//columns of 4 bacons
for (var x = 140; x <= 472; x += 162) {
for (var y = 70; y <= 538; y += 152) {
push();
translate(x, y);
rotate(radians(-280));
scale(.7);
bacon();
pop();
}
}
}
function egg() {
fill(255); //white
noStroke();
ellipse(-11, -14, 40, 42);
ellipse(-7, 21, 50, 48);
beginShape();
curveVertex(4, -28);
curveVertex(4, -28);
curveVertex(23, 10);
curveVertex(9, 40);
curveVertex(9, 40);
endShape();
beginShape();
curveVertex(-27, -10);
curveVertex(-27, -10);
curveVertex(-10, -10);
curveVertex(-27, 10);
curveVertex(-27, 10);
endShape();
fill(255, 200, 100); //yolk
ellipse(-2, 5, 30);
noFill();
stroke(255);
strokeWeight(3);
arc(-2, 5, 20, 20, HALF_PI, PI);
}
function bacon() {
noFill();
strokeWeight(8);
strokeCap(SQUARE);
stroke(147, 73, 0);
bezier(35, -25, -40, -40, 40, 40, -35, 35);
push();
translate(13, 13);
bezier(35, -25, -40, -40, 40, 40, -35, 35);
pop();
}
function grid() {
rectMode(CENTER);
noStroke();
fill(255, 175, 148, 150);
rect(0, 0, 17, 17);
}
For this project, I was inspired by my water bottle, which has an eggs and bacon design on it. I decided to make my own pattern and have included some of my initial sketches below. I wanted to play with custom shapes, specifically curveVertex and bezier curves, as well as arcs. To add visual interest, I experimented with shifts in scale and rotation in creating an alternating pattern of eggs and bacon.
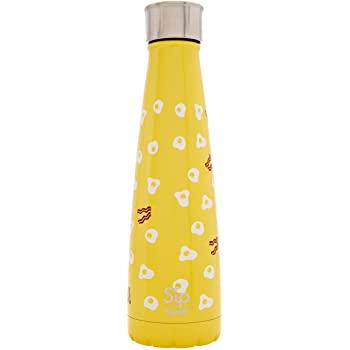
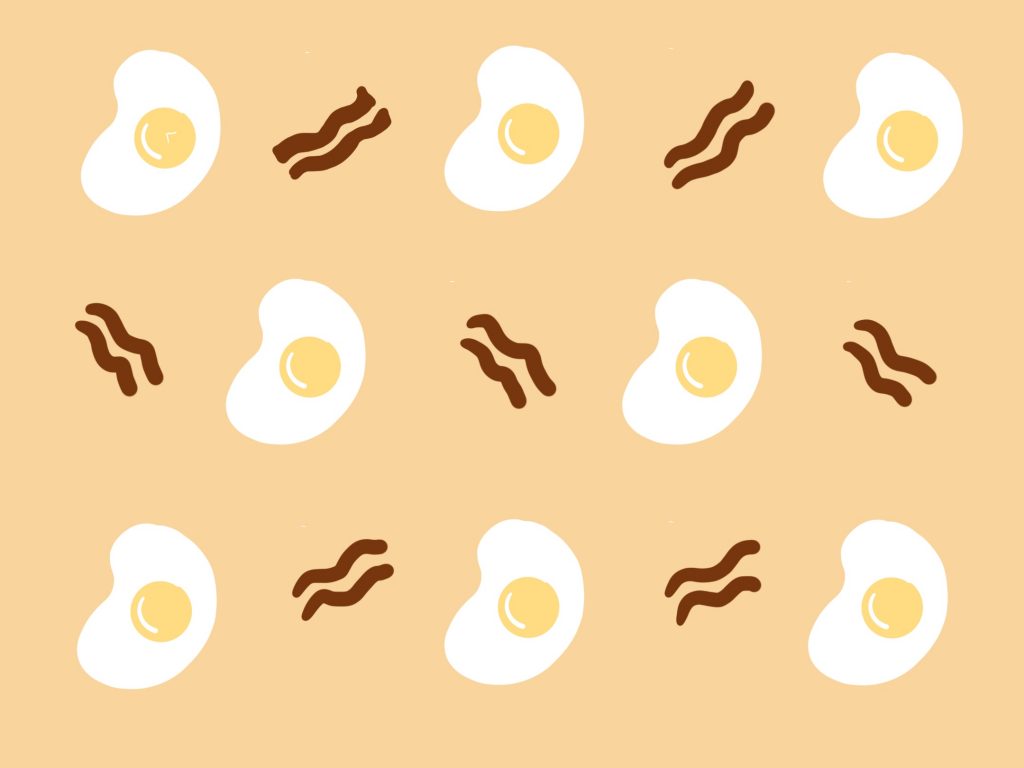
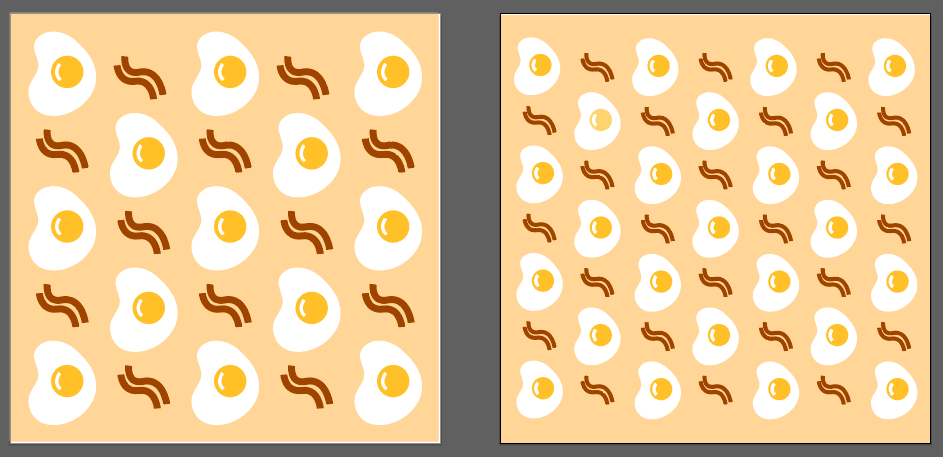