let portrait;
var radius = 0;
var angle = 0;
var framecount = 1;
var size = 0.5;
function preload() {
portrait = loadImage('https://i.imgur.com/EquKB8x.png');
}
function setup() {
createCanvas(480, 480);
background(0);
imageMode(CENTER);
portrait.loadPixels();
}
function draw() {
var centerX = width/2;
var centerY = height/2;
var circleX = radius * cos(radians(angle));
var circleY = radius * sin(radians(angle));
var clrX = random(0, width);
var clrY = random(0, height);
var clr = portrait.get(clrX, clrY);
//pointillize
for (var i= 0; i < 10; i++) {
fill(clr);
circle(clrX, clrY, size);
}
//top left spiral of hearts of image
noStroke();
var clr1 = portrait.get(circleX, circleY);
fill(clr1);
drawHeart(circleX, circleY, 15);
//bottom right spiral of hearts of image
push();
translate(centerX, centerY);
var clr2 = portrait.get(480-circleX, 480-circleY);
fill(clr2);
drawHeart(centerX-circleX, centerY-circleY, 15);
pop();
topInstagramBar();
bottomInstagramBar();
radius += 0.1;
angle += 7;
frameCount += 1;
if (frameCount > 13000) {
//"instagram tag" of my nickname where mouse is
fill(85);
triangle(mouseX, mouseY-10, mouseX+10, mouseY-20, mouseX-10, mouseY-20);
rect(mouseX-40, mouseY-40, 80, 20);
fill(255);
text('rishdish', mouseX-20, mouseY-27);
//stops when portrait is complete
noLoop();
}
}
function drawHeart(x, y, s) {
beginShape();
vertex(x,y);
bezierVertex(x-s/2, y-s/2, x-s, y+s/3, x, y+s);
bezierVertex(x+s, y+s/3, x+s/2, y-s/2, x, y);
endShape(CLOSE);
}
function mousePressed() {
//points get bigger as you click
size += 0.5;
if (size == 7) {
size = 0.5;
}
}
function topInstagramBar() {
//instagram bar
fill(240);
rect(0, 0, 480, 30);
//profile pic
fill(230, 210, 210);
circle(20, 15, 15);
stroke(195, 45, 140);
noFill();
circle(20, 15, 18);
noStroke();
fill(0);
text('15-104',35, 19);
//three dots in right hand corner
fill(10);
circle(455, 15, 3);
circle(460, 15, 3);
circle(465, 15, 3);
}
function bottomInstagramBar() {
//instagram bar
fill(240);
rect(0, 450, 480, 30);
//"like" icon
stroke(0);
fill(255);
drawHeart(20, 460, 15);
//"comment" icon
triangle(53, 471, 60, 471, 58, 467);
circle(50, 465, 16);
stroke(255);
circle(55, 468, 5);
//"share" icon
stroke(0);
triangle(75, 457, 92, 457, 80, 463);
triangle(80, 463, 92, 458, 82,472);
//"save"icon
beginShape();
vertex(454, 457);
vertex(454, 472);
vertex(460, 467);
vertex(466, 472);
vertex(466, 457);
endShape(CLOSE);
//image scroll through icons
noStroke();
fill(65, 141, 204);
circle(230, 465, 5);
fill(165);
circle(240, 465, 5);
circle(250, 465, 5);
}
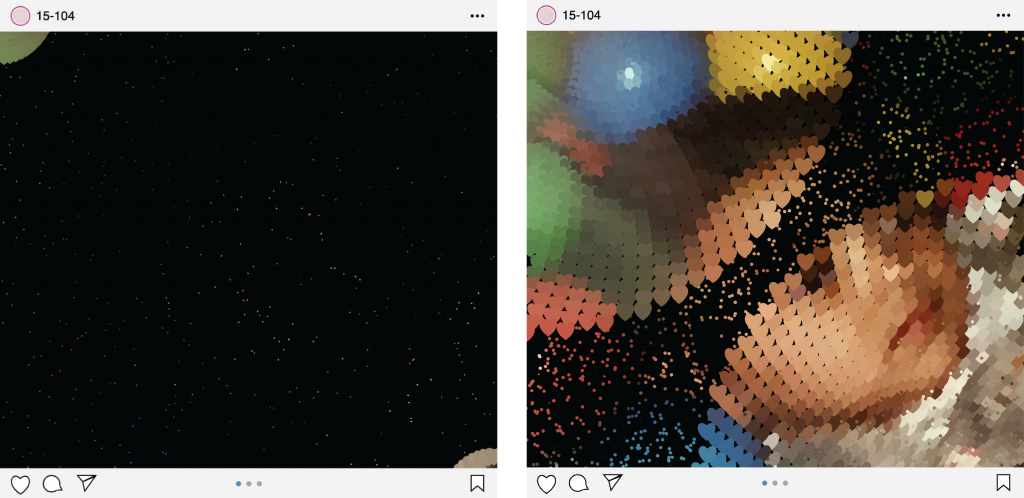
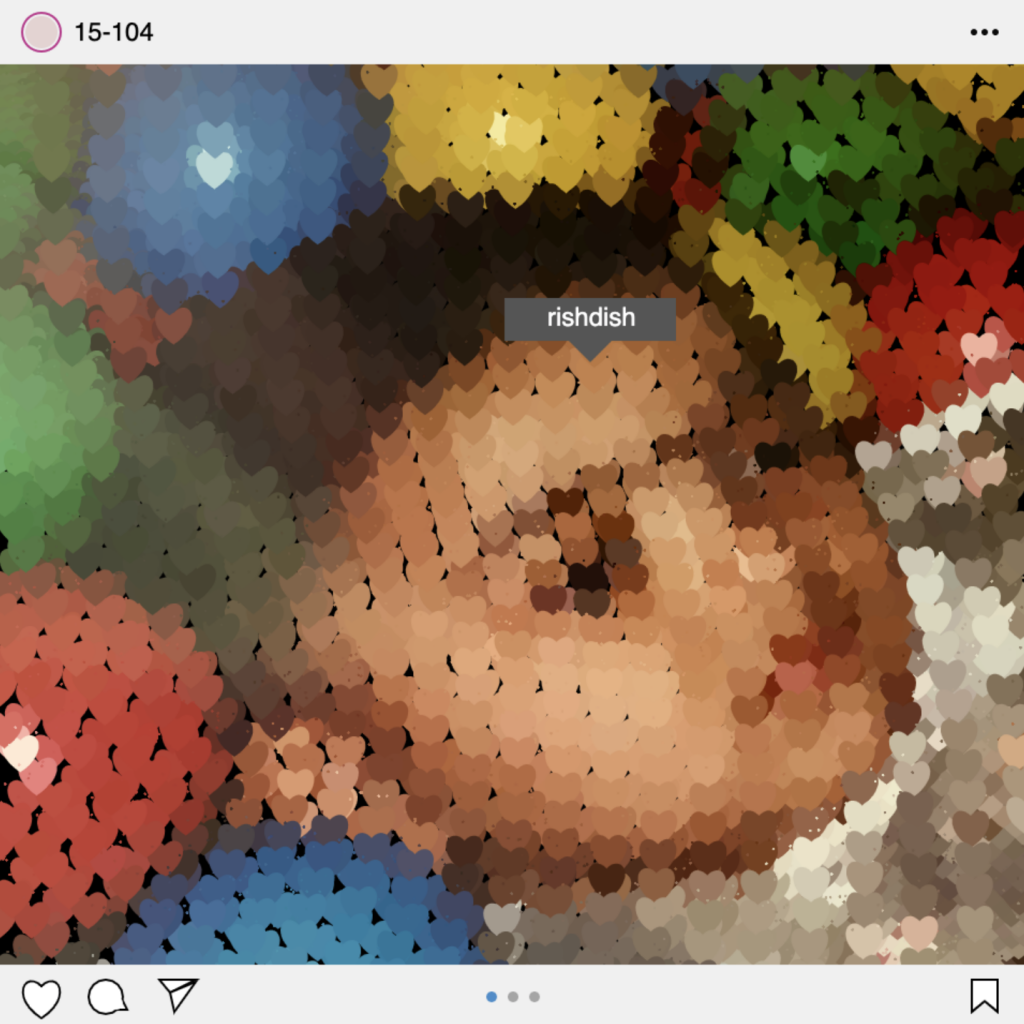
I used a photo of me as a baby for this. Once I had the two spirals of hearts running, I added the icons to make it look like an instagram post. When you click, the size of the points in the background increases. When the portrait is done running, an Instagram tag shows up where the mouse is with my childhood nickname.