stringartjessa
var dx1;
var dy1;
var dx2;
var dy2;
var count = 50;
function setup() {
createCanvas(400, 400);
background(0);
dx1 = (400-200)/count;
dy1 = (0-200)/count;
dx2 = (400-400)/count;
dy2 = (400-400)/count;
rectMode(CENTER);
}
function draw() {
//divide the canvas into quadrants
fill(255, 255, 255, 25); //set fill to transparent white
rect(300,0,width/2, height); //draw square in top right
rect(0,300,width, height/2); //draw square in bottom left
push();
push();
push();
translate(-200,0) //move the origin to the left
//shape 1
stroke('cyan')
var x1 = 200;
var y1 = 200;
var x2 = 400;
var y2 = 200;
for (var i = 0; i <= count; i += 1) {
line(x1, y1, x2, y2);
x1 += dx1;
y1 += dy1;
x2 += dx2;
y2 += dy2;
noLoop();
}
pop() //return origin position
//shape 2
stroke('white')
da1 = (200-0)/count;
db1 = (200-0)/count;
da2 = (400-0)/count;
db2 = (200-400)/count;
var a1 = 0;
var b1 = 0;
var a2 = 400;
var b2 = 400;
for (var i = 0; i <= count; i += 1) {
line(a1, b1, a2, b2);
a1 += da1;
b1 += db1;
a2 += da2;
b2 += db2;
noLoop();
}
push(); //save current canvas properties
rotate(radians(45)); //rotate canvas 45 degrees
//shape 3
stroke('magenta')
dc1 = (0-400)/count;
dd1 = (0-0)/count;
dc2 = (0-200)/count;
dd2 = (400-200)/count;
var c1 = 400;
var d1 = 0;
var c2 = 200;
var d2 = 200;
for (var i = 0; i <= count; i += 1) {
line(c1, d1, c2, d2);
c1 += dc1;
d1 += dd1;
c2 += dc2;
d2 += dd2;
noLoop();
}
pop(); //restore canvas properties before drawing shape 3
rotate(radians(-20)) //rotate canvas 20 degrees counterclockwise
translate(-78,58) //move canvas to the left and down so top point of shape 4 meets center of canvas
//shape 4
stroke('cyan')
de1 = (200-400)/count;
df1 = (200-400)/count;
de2 = (0-200)/count;
df2 = (400-200)/count;
var e1 = 400;
var f1 = 400;
var e2 = 200;
var f2 = 200;
for (var i = 0; i <= count; i += 1) {
line(e1, f1, e2, f2);
e1 += de1;
f1 += df1;
e2 += de2;
f2 += df2;
noLoop();
}
pop(); //reset canvas properties to before shape 3 was drawn
//shape 5
stroke('magenta');
dg1 = (200-400)/count;
dh1 = (0-0)/count;
dg2 = (200-400)/count;
dh2 = (400-100)/count;
var g1 = 400;
var h1 = 0;
var g2 = 400;
var h2 = 200;
for (var i = 0; i <= count; i += 1) {
line(g1, h1, g2, h2);
g1 += dg1;
h1 += dh1;
g2 += dg2;
h2 += dh2;
noLoop();
}
translate(-15,135) //move canvas to the left and down
rotate(radians(-44)) //rotate canvas 44 degrees counterclockwise
//shape 6
stroke('white')
dj1 = (400-200)/count;
dk1 = (200-0)/count;
dj2 = (200-400)/count;
dk2 = (400-200)/count;
var j1 = 200;
var k1 = 0;
var j2 = 400;
var k2 = 200;
for (var i = 0; i <= count; i += 1) {
line(j1, k1, j2, k2);
j1 += dj1;
k1 += dk1;
j2 += dj2;
k2 += dk2;
}
noLoop();
}
It took me a while to figure out how to manipulate the loops to make the shapes I wanted, so I made some general sketches to guide the composition and the guide lines. Ultimately, I had to transform the canvas and make slight modifications throughout the code to get the visual effect I wanted, but this would have been impossible to do without loops.
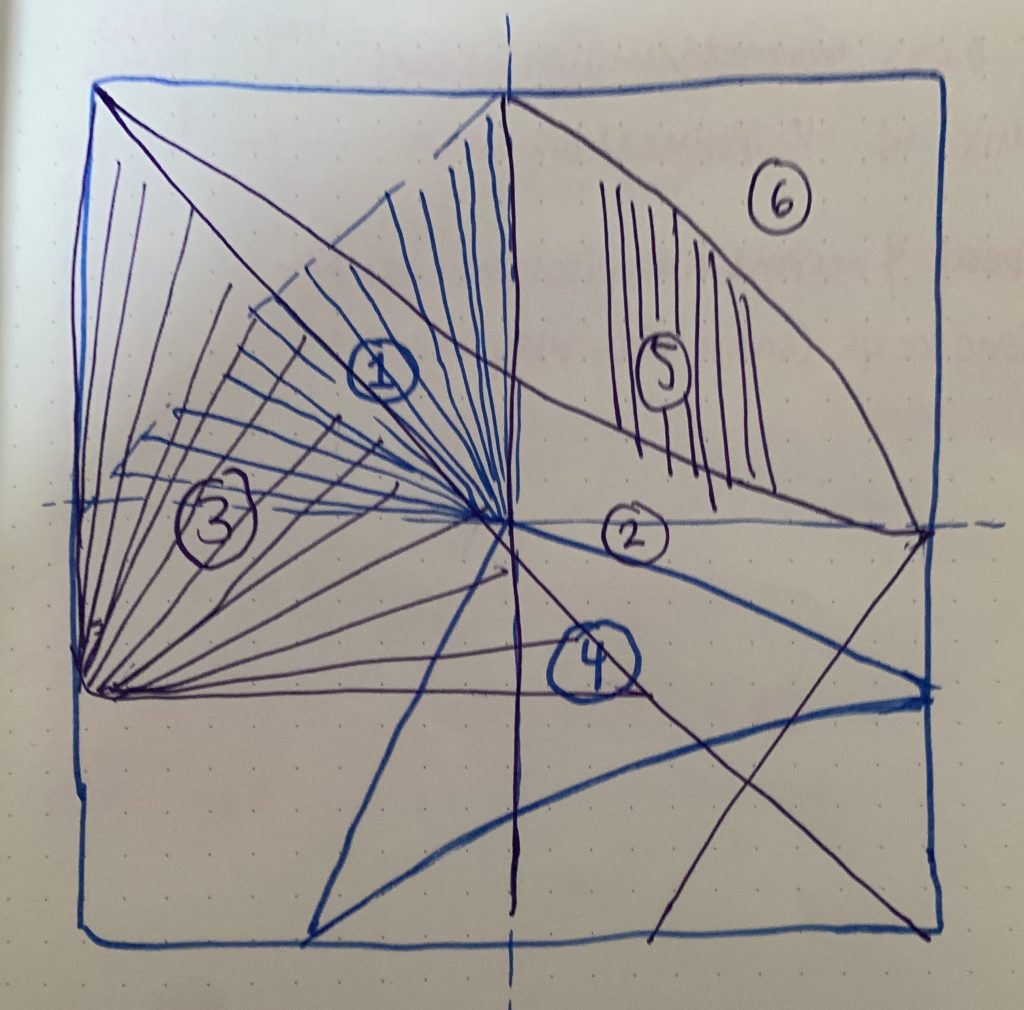