sketch
/*Aadya Bhartia
Section A
abhartia@cmu.edu*/
//initializing arrays to hold x and y position of candy based on hours
var candyX = [0,0,25,-25,-8,43,-42,15,-21,40,-45,6,12];
var candyY = [0,47,40,40,18,18,18,12,2,-5,-10,-15,-20];
var minX = 90;
var minY = 90;
var secX = 260;
var secY = 380;
var hr = 0;
function setup() {
createCanvas(500, 500);
background(255, 217, 206);
}
function draw() {
background(255, 217, 206);
var s = second();
var m = minute();
var h = hour();
var angle = 0;
var secR = s/60;
var minR = m/60;
//seconds
fill(139, 170, 173,90);
noStroke();
ellipse(secX, secY, 177*secR); //circle increasing in size for seconds
stroke(64, 78, 92);
strokeWeight(1/20*s);
noFill();
arc(secX,secY,200, 200, 0 , 2*PI * secR);
stroke(146, 55, 77);
strokeWeight(1/6*s); //stroke gets thicker based on seconds
noFill();
arc(secX,secY,184, 184, 0 ,2*PI * secR);
fill(221, 117, 150);
noStroke();
rect(secX+82,secY-8,200,10); //bar for seconds
//minutes
fill(221, 117, 150);
noStroke();
rect((minX-3),(minY +62),6,400);//base for the candy ball to count minutes
push();
noFill();
strokeWeight(1/5*m);
stroke(113, 10, 53);
translate(minX,minY);
ellipse(0, 0, 140); //base for the candy ball to count minutes
stroke(0);
strokeWeight(1);
//hour
hr = h%12; // number of candy in the ball count the hours
candy(hr);
pop();
//minutes counted with hearts in a 6 by 10 grid
var ctr = [];
var counter = 0;
for(var i = 0;i<60;i++){ //creating an array to store all possible minutes
ctr.push(i);
}
for(var x = 1;x<7;x++){
for(var y = 1;y<=10;y++){
push();
translate(320,8);
if(counter == m){
fill(255); //white colour for current minute
heart(25*x,25*y);
}
else{
fill(221, 117, 150,random(100,200));
heart(25*x,25*y);
}
pop();
counter+=1;
}
}
}
//candy helps count the hours
function candy(hr){
for(var j = 1; j<=hr;j++){//candy globe effect given by the predefined array counting the hours
fill(random(100,200),random(0,50),random(20,70));
ellipse(candyX[j],candyY[j],35);
}
}
function heart(minX,minY){ //creating hearts which count the minutes
ellipse(minX-4,minY,9);
ellipse(minX+4,minY,9);
triangle(minX-8,minY+2,minX+8,minY+2,minX,minY+10)
}
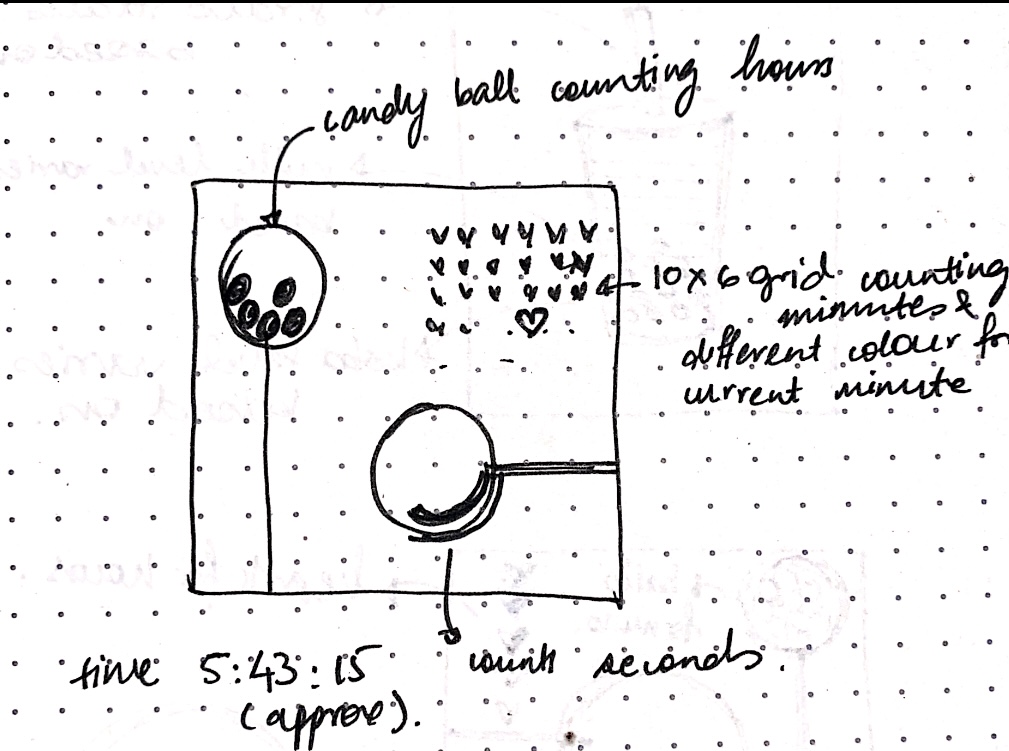