For this project I wanted to create a hospital scene to demonstrate time, and how lucky we are to experience time and have a beating heart. The IV drip represents minutes with markers indicating mid and quarter points. The heart monitor represents seconds, and the window scene outside indicates if it is morning or evening. Additionally, I threw in some moving clouds and spinning stars to make the window view more dynamic! Below are also pictures of how it looks during day and night time.
graanak-06
//Graana Khan
// Section B
// graanak@andrew.cmu.edu
// Porject-06
/* Seconds is shown by the heart monitor, minutes are shown by the blood bag IV decreasing,
and hours are shown by whether the window displays a nighttime or daytime scene. */
function setup() {
createCanvas(480, 480);
background(220);
var x = 0;
}
function draw() {
let h = hour();
let m = minute();
background(237, 237, 239);
noStroke();
fill(56, 46, 35);
rect(0, 407, 481, 73);
//window frame
noStroke();
fill(190, 195, 198);
rect(164, 268, 258, 20);
rect(175, 30, 235, 241);
//iv drip
fill(216);
rect(18, 457, 80, 5, 10);
rect(56, 127, 5, 333, 10);
rect(59, 134, 48, 5, 10);
rect(103, 135, 4, 9, 10);
//iv drip bag and wire
stroke(225, 224, 252);
strokeWeight(2);
noFill();
rect(88.5, 144, 34, 62.5, 10);
strokeWeight(1);
fill(147, 6, 6);
beginShape();
curveVertex(103, 206);
curveVertex(103, 206);
curveVertex(103, 293);
curveVertex(106, 322);
curveVertex(128, 342);
curveVertex(182, 357);
curveVertex(291, 357);
curveVertex(292, 353);
curveVertex(182, 353);
curveVertex(129, 338);
curveVertex(109, 319);
curveVertex(107, 293);
curveVertex(107, 206);
curveVertex(107, 206);
endShape();
//iv blood with changing minutes
noStroke();
fill(147, 6, 6);
rect(90, 145+m, 30, 60-m, 10);
// iv bag lines showing mid points of the minutes
stroke(225, 224, 252);
strokeWeight(2);
line(89, 160, 93, 160);
line(89, 175, 99, 175);
line(89, 190, 93, 190);
//window appearance
if(h < 18 & h > 5){
daytime();
} else{
nighttime();
}
//table
noStroke();
fill(255);
rect(92, 308, 6, 141, 10);
rect(338, 308, 6, 141, 10);
rect(92, 305, 252, 12, 10);
//flower vase
stroke(50, 132, 50);
strokeWeight(2);
line(312, 302, 312, 243);
stroke(225, 224, 252);
strokeWeight(1);
noFill();
rect(298, 263, 28, 46, 7);
//water
fill(187, 201, 249, 100);
noStroke();
rect(298, 280, 28, 29, 7);
//flower
noStroke();
fill(244, 132, 196);
ellipse(307, 244, 11, 2);
ellipse(318, 244, 11, 2);
push();
translate(308, 247);
rotate(radians(-40));
ellipse(0, 0, 11, 2);
pop();
push();
translate(317, 247);
rotate(radians(40));
ellipse(0, 0, 11, 2);
pop();
push();
translate(310, 249);
rotate(radians(-70));
ellipse(0, 0, 11, 2);
pop();
push();
translate(314, 249);
rotate(radians(70));
ellipse(0, 0, 11, 2);
pop();
push();
translate(308, 242);
rotate(radians(20));
ellipse(0, 0, 11, 2);
pop();
push();
translate(317, 242);
rotate(radians(-20));
ellipse(0, 0, 11, 2);
pop();
push();
translate(310, 239);
rotate(radians(60));
ellipse(0, 0, 11, 2);
pop();
push();
translate(320, 260);
rotate(radians(-60));
ellipse(0, 0, 11, 2);
pop();
fill(255, 223, 87);
ellipse(312, 244, 4, 3);
//heart monitor
fill(255);
rect(143, 202, 135, 103, 20);
fill(49, 49, 51);
rect(147, 206, 128, 97, 20);
fill(194, 255, 69);
rect(240, 290, 19, 8, 10);
fill(72, 72, 255);
ellipse(233, 294, 8, 8);
//hospital bed
fill(180, 178, 191);
rect(73, 364, 310, 16, 20);
rect(73, 331, 15, 45, 20);
push();
translate(450, 268);
rotate(radians(45));
rect(10, 0, 18, 134, 20);
fill(199, 221, 239);
rect(-17, 15, 27, 117, 40);
pop();
fill(199, 221, 239)
rect(89, 337, 285, 27, 20);
fill(180, 178, 191);
rect(80, 368, 5, 93, 10);
rect(378, 368, 5, 93, 10);
stroke(216);
strokeWeight(3);
fill(255);
ellipse(82, 461, 18, 18);
ellipse(380, 461, 18, 18);
//heart monitor seconds tracker
let sec = map(second(), 0, 60, 0, 120);
var x = sec;
heartmonitor(x);
}
function daytime(){
noStroke();
fill(213, 231, 247);
rect(186, 42, 211, 217);
fill(255, 255, 255, 150);
rect(210 + (mouseX/50), 55, 52, 21, 20);
rect(238 + (mouseX/50), 68, 39, 21, 10);
rect(331 + (mouseX/50), 86, 48, 28, 10);
rect(261 + (mouseX/50), 168, 38, 21, 10);
rect(244 + (mouseX/50), 179, 38, 21, 10);
fill(247, 221, 109, 100);
circle(292, 80, 63);
fill(247, 221, 109);
circle(292, 80, 50);
}
function nighttime(){
noStroke();
fill(12, 33, 48);
rect(186, 42, 211, 217);
noFill();
stroke(234, 234, 139, 200);
strokeWeight(2);
push();
translate(360, 75);
rotate(radians(mouseX));
line(0, -5, 0, 5);
line(-5, 0, 5, 0);
pop();
push();
translate(357, 166);
rotate(radians(mouseX));
line(0, -5, 0, 5);
line(-5, 0, 5, 0);
pop();
push();
translate(226, 121);
rotate(radians(mouseX));
line(0, -5, 0, 5);
line(-5, 0, 5, 0);
pop();
push();
translate(292, 80);
noStroke();
fill(255, 255, 255, 100);
circle(0, 0, 63);
fill(255);
circle(0, 0, 50);
pop();
}
function heartmonitor(x){
noStroke();
fill(247, 8, 162);
beginShape();
curveVertex(149 + x, 255);
curveVertex(149 + x, 255);
curveVertex(148 + x, 254);
curveVertex(147 + x, 255);
curveVertex(149 + x, 258);
curveVertex(151 + x, 255);
curveVertex(150 + x, 254);
curveVertex(150 + x, 254);
endShape();
}
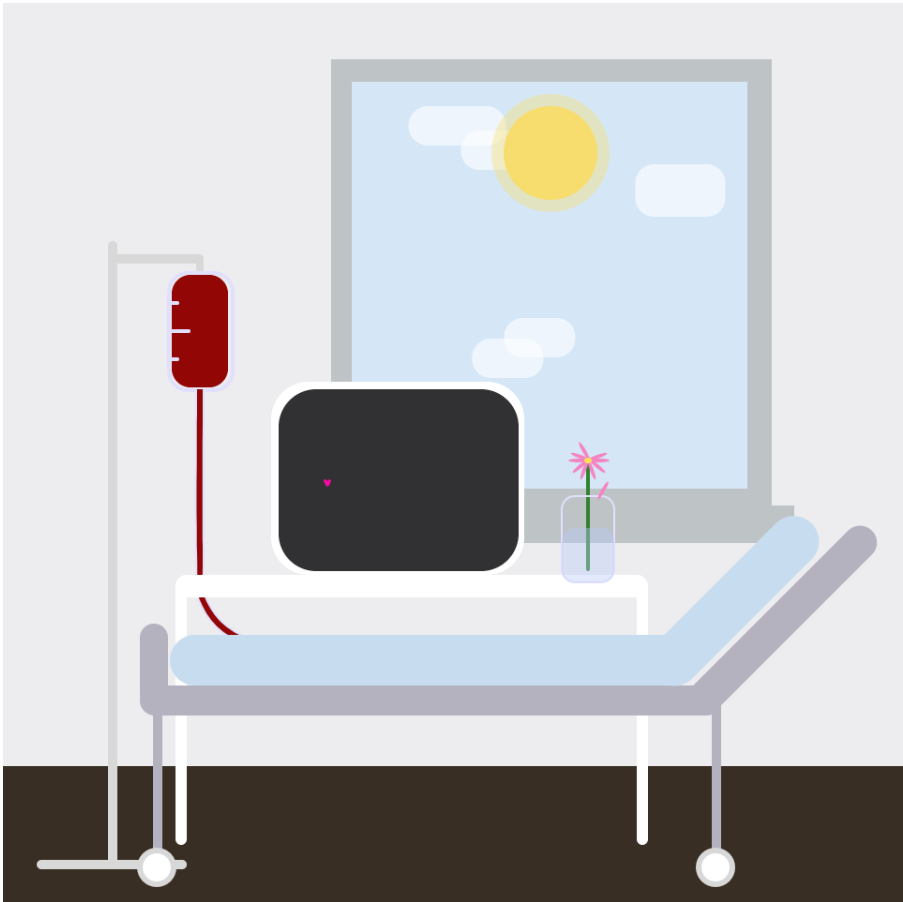
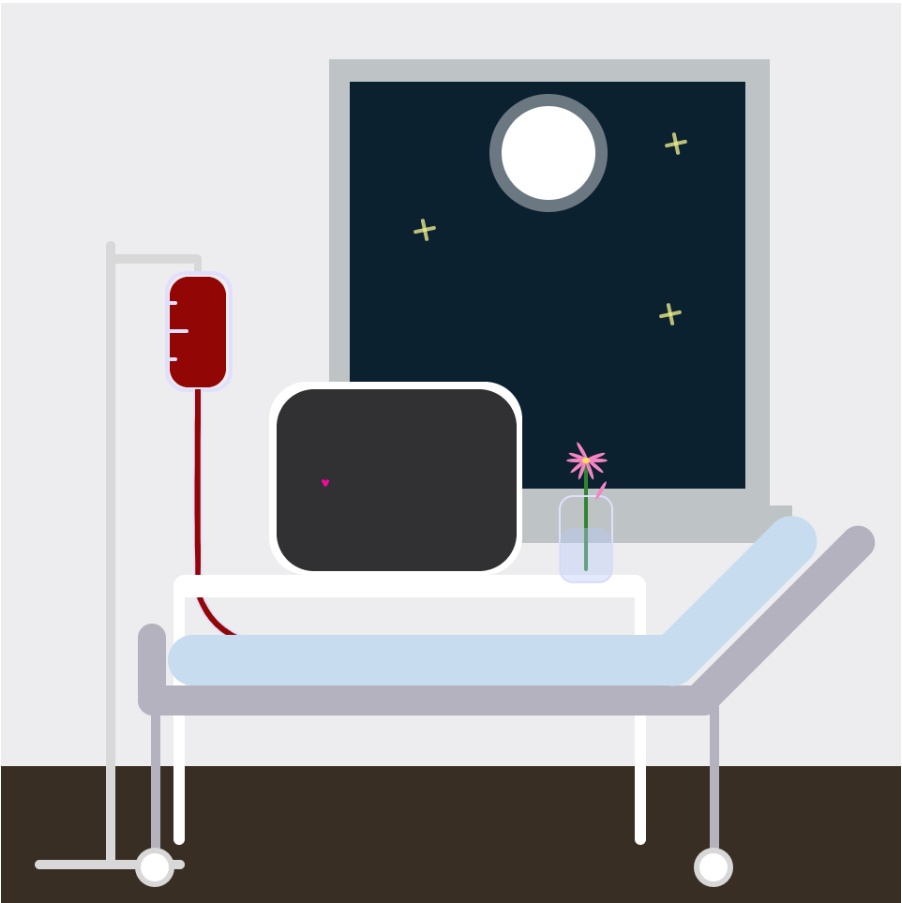