When looking through the curves, I thought it would be perfect to use them and create a flower with multiple layers. I decided to use the hypotrochoid and the epitrochoid curves with pastel-ish colors. As you move across x and y, the flower should grow in size relative to where mouseX and mouseY are. The background color also changes relative to the x and y positions of the mouse.
sketch
//Jessie Chen
//jessiech@andrew.cmu.edu
//D
//Project 07
//Curves
var nPoints = 100;
var angle = 0;
var radius = 200;
var r = 200;
var g = 170;
var b = 170;
function setup() {
createCanvas(480, 480);
frameRate(20);
}
function draw() {
var xcolorchange = map(mouseX, 0, width, 0, 50);
var ycolorchange = map(mouseY, 0, height, 0, 50);
//slight change in the background as mouse moves across x and y
background(r - ycolorchange, g + xcolorchange, b + ycolorchange);
//origin at the center of the canvas
translate(width/2, height/2);
//purple layer of petals
push(radians(angle));
rotate(radians(-angle))
angle += mouseX;
stroke(240, 192, 236);
//fill(152, 115, 148);
fill(207, 181, 210)
hypotrochoid(mouseX/2);
pop()
//pink layer of petals
push();
rotate(radians(angle));
angle += mouseY;
stroke(232, 164, 184);
fill(193, 116, 139);
epitrochoid(mouseX/3);
pop();
//yellow layer of petals
push();
rotate(radians(angle));
angle += mouseX;
stroke(239, 216, 142);
fill(193, 175, 116);
hypotrochoid(mouseX/4);
pop();
//green layer of petals
push();
rotate(radians(angle));
angle += mouseY;
stroke(216, 240, 192);
fill(164, 176, 148);
epitrochoid(mouseX/8);
pop();
//orange circles
push();
rotate(radians(angle));
angle += mouseX;
radius = mouseX/3;
fill(164, 209, 232);
circles(radius);
pop();
//blue circles
push();
rotate(radians(angle));
angle += mouseY;
radius = mouseX/2;
fill (233, 175, 109);
circles(radius);
pop();
//yellow circles
push();
rotate(radians(angle));
angle += mouseX;
radius = mouseX/15;
fill(239, 216, 142);
circles(radius);
pop();
}
function epitrochoid(size) {
var x;
var y;
var a = size;
var b = a/15;
var h = constrain(mouseY/30, a/5, b*5);
beginShape();
for(var i = 0; i<nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a + b) * cos(t) + h * cos(((a + b) / b) * t);
y = (a + b) * sin(t) + h * sin (((a + b) / b) * t);
vertex(x, y);
}
endShape(CLOSE);
}
function hypotrochoid(size) {
var x;
var y;
var a = size;
var b = a/15;
var h = constrain(mouseY/30, a/5, b*10);
var ph = mouseX/60;
beginShape();
for (var i = 0; i < nPoints; i++) {
var t = map(i, 0, nPoints, 0, TWO_PI);
x = (a - b) * cos(t) + h * cos(((a - b) / b) * t);
y = (a - b) * sin(t) + h * sin (((a - b) / b) * t);
vertex(x, y);
}
endShape(CLOSE);
}
//array of circles
function circles(radius) {
for (t = 0; t < 360; t = t + 30) {
var x = radius * cos(radians(t));
var y = radius * sin(radians(t));
stroke(255);
ellipse(x, y, mouseX/35, mouseX/35);
}
}
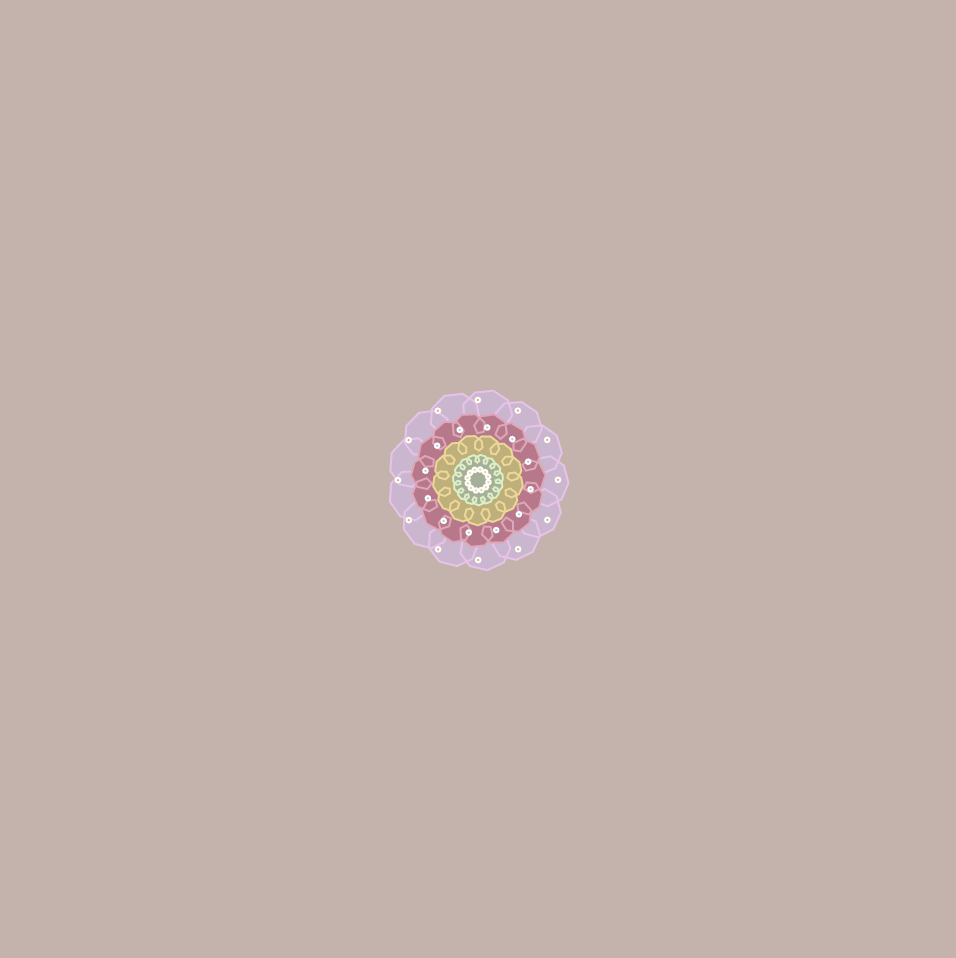
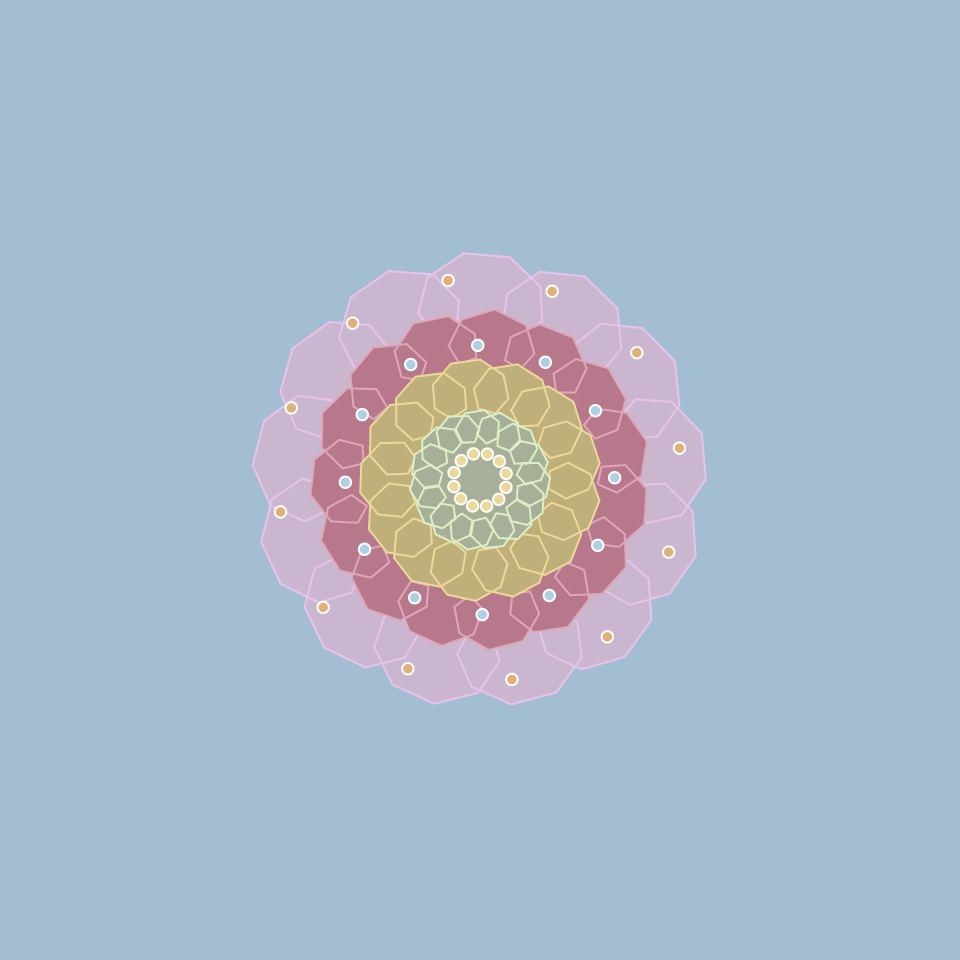
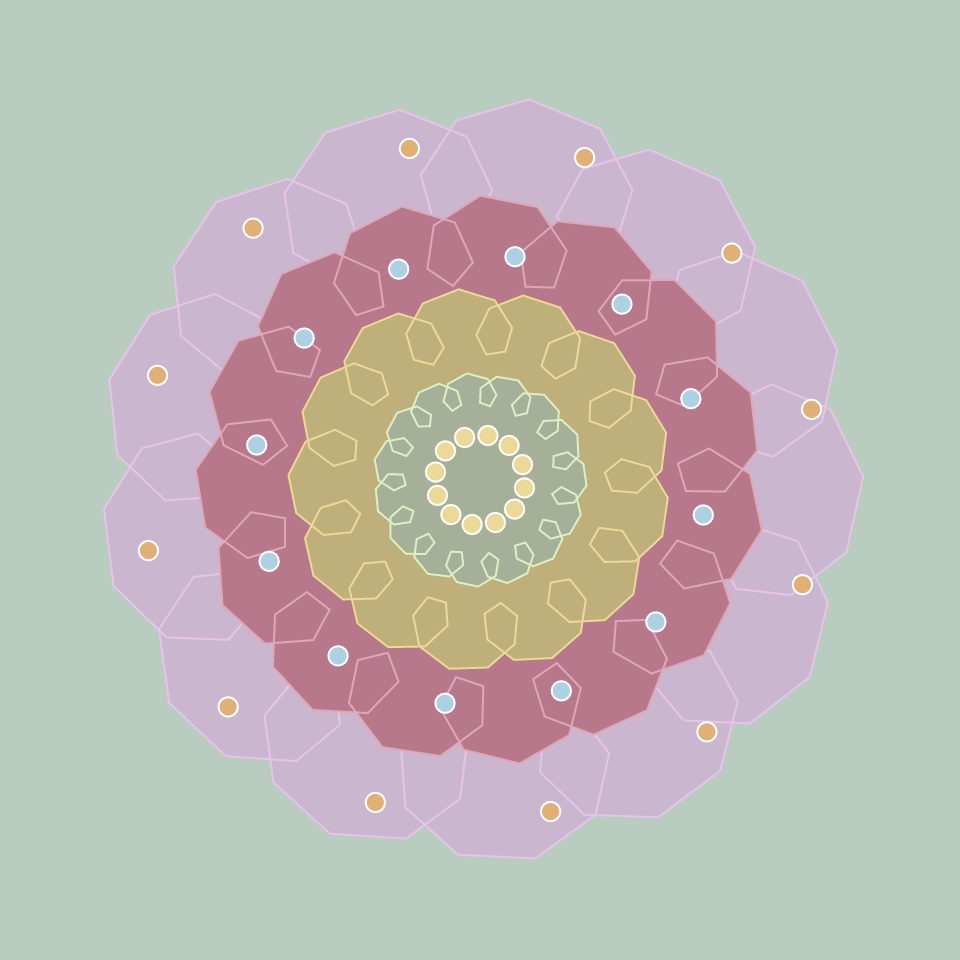